Using the igloohome API to Create Pin (with Javascript examples)
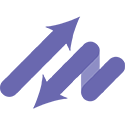
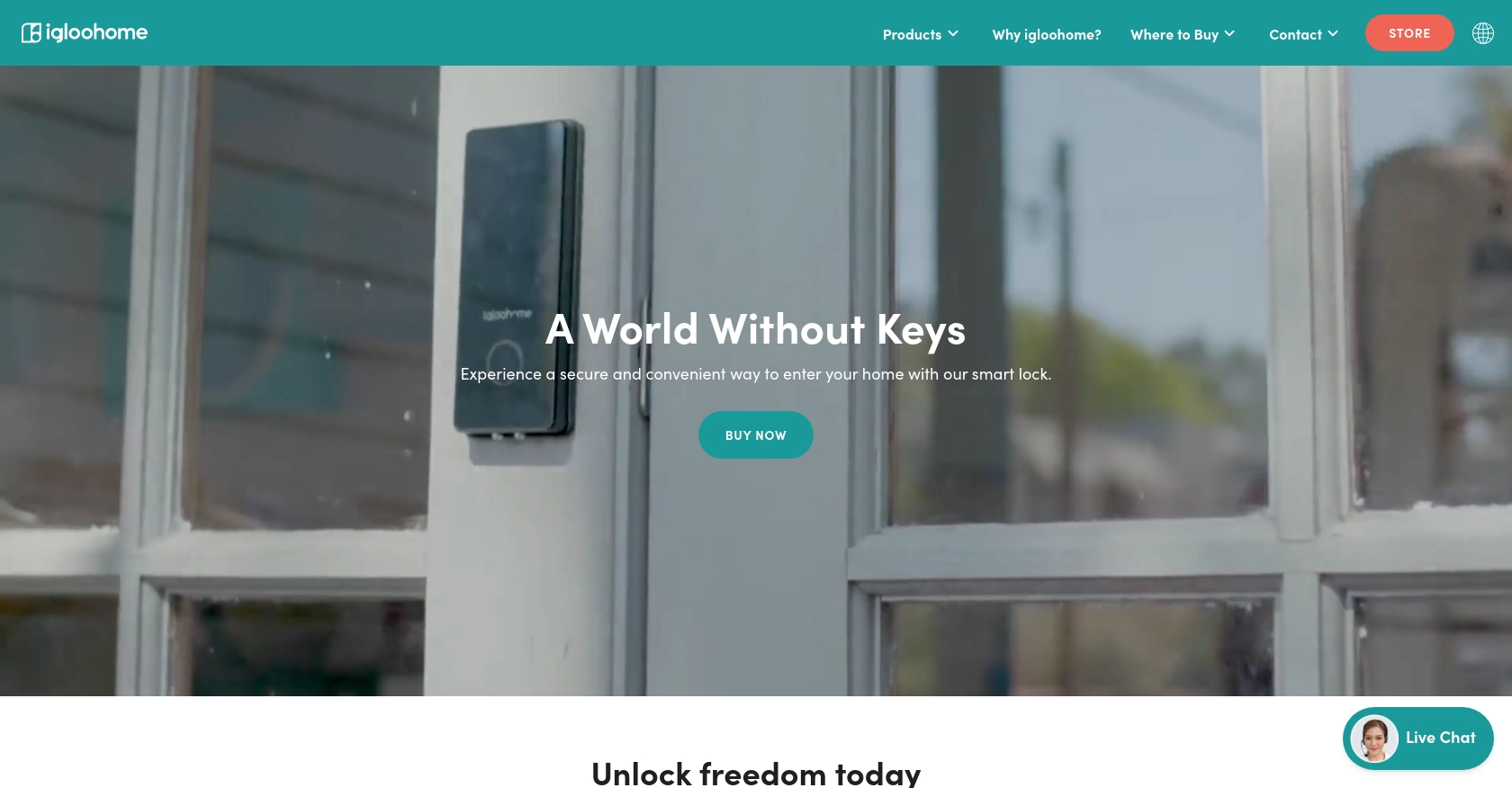
Introduction to igloohome API
igloohome is a leading provider of smart access solutions, offering a range of products that allow users to manage property access remotely. With its innovative technology, igloohome enables seamless and secure entry management for homes, offices, and vacation rentals.
Developers may want to integrate with the igloohome API to enhance their applications with smart access capabilities. By connecting with igloohome, developers can automate the creation of PIN codes, manage access logs, and provide users with a flexible and secure way to control entry to their properties.
For example, a property management software could use the igloohome API to generate one-time PIN codes for guests, ensuring secure and temporary access to rental properties. This integration can significantly improve the guest experience by providing a hassle-free check-in process.
Setting Up Your igloohome Test/Sandbox Account
To begin integrating with the igloohome API, you'll need to set up a test or sandbox account. This allows you to experiment with the API without affecting live data. Follow these steps to get started:
Register for an igloohome API Trial
First, sign up for a 30-day trial of the igloohome API. This trial provides access to all necessary features for testing and development.
- Visit the igloohome registration page.
- Fill out the registration form with your details and submit it.
- Check your email for a confirmation message and follow the instructions to activate your account.
Creating an igloohome App for OAuth Authentication
Since the igloohome API uses OAuth for authentication, you'll need to create an app to obtain the necessary credentials.
- Log in to your igloohome developer account.
- Navigate to the "Apps" section and click on "Create New App."
- Fill in the required information, such as the app name and description.
- Set the redirect URI to a valid URL where users will be redirected after authentication.
- Save your app, and note down the client ID and client secret provided. These will be used for API authentication.
Configuring OAuth Scopes for igloohome API Access
To ensure your app has the correct permissions, configure the OAuth scopes:
- In the app settings, locate the "Scopes" section.
- Select the necessary scopes for creating PIN codes and managing access.
- Save the changes to update your app's permissions.
With your igloohome test account and app set up, you're ready to start making API calls and integrating smart access features into your application.
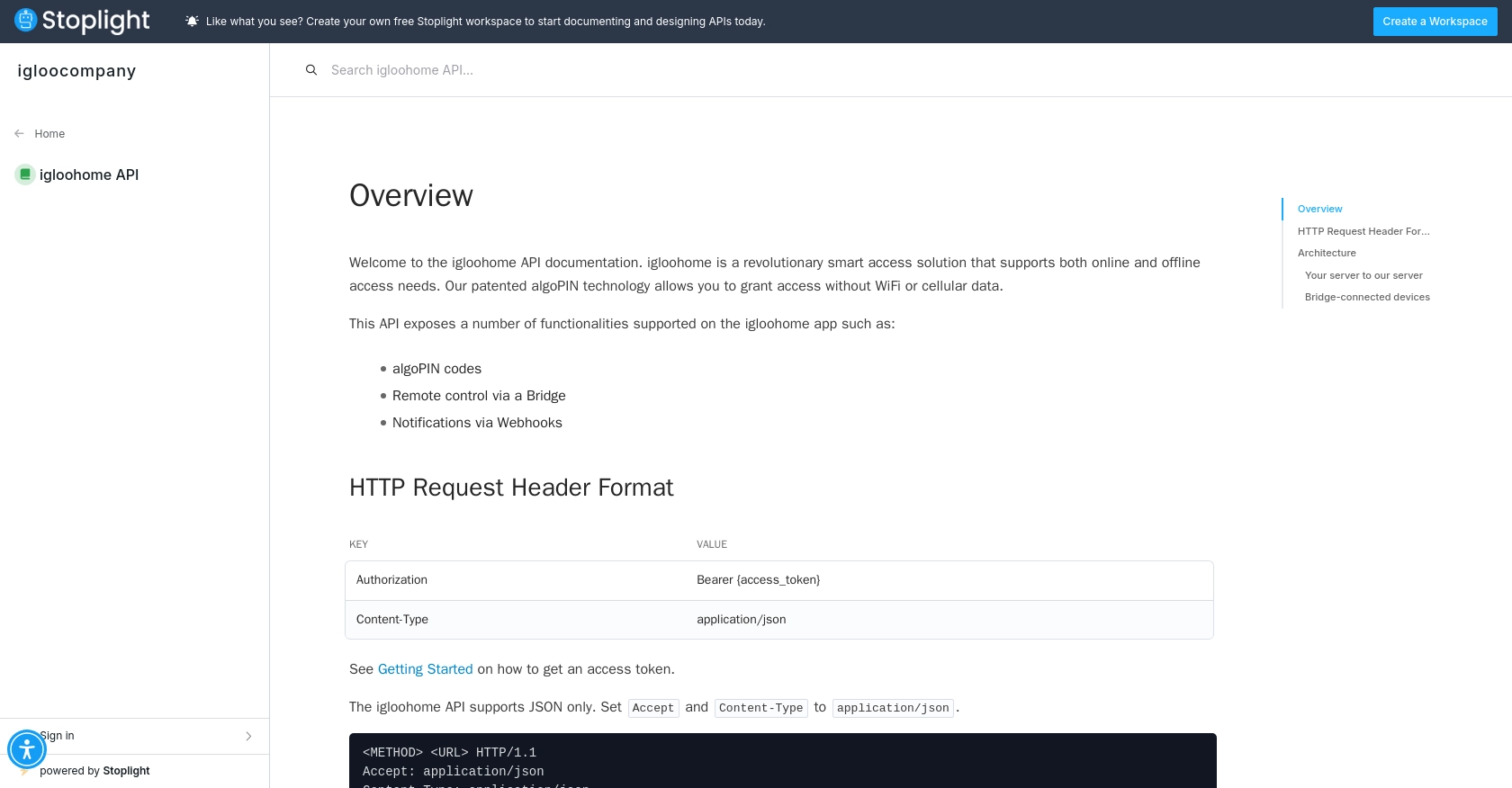
sbb-itb-96038d7
Making API Calls to Create a One-Time PIN Code with igloohome API Using JavaScript
To interact with the igloohome API and create a one-time PIN code, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for igloohome API Integration
Before you begin coding, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code.
- Basic knowledge of JavaScript and asynchronous programming.
Installing Necessary Dependencies for igloohome API Calls
You'll need to install the axios
library to handle HTTP requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Create a One-Time PIN Code with igloohome API
Now, let's write the code to make an API call to create a one-time PIN code:
const axios = require('axios');
// Replace with your client ID, client secret, and access token
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
const accessToken = 'YOUR_ACCESS_TOKEN';
// API endpoint for creating a one-time PIN code
const endpoint = 'https://api.igloohome.co/v1/pins';
// Function to create a one-time PIN code
async function createOneTimePin() {
try {
const response = await axios.post(endpoint, {
// Request body parameters
type: 'one_time',
duration: 60 // Duration in minutes
}, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('One-Time PIN Code Created:', response.data);
} catch (error) {
console.error('Error Creating PIN Code:', error.response ? error.response.data : error.message);
}
}
// Call the function to create a PIN
createOneTimePin();
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, and YOUR_ACCESS_TOKEN
with the credentials obtained during the OAuth setup.
Verifying API Call Success and Handling Errors
After running the code, you should see the newly created PIN code in the console output. To verify the success of your API call, check the response data for the PIN code details.
If an error occurs, the catch block will log the error message. Common errors include invalid credentials or insufficient permissions. Refer to the igloohome API documentation for more details on error codes and troubleshooting.
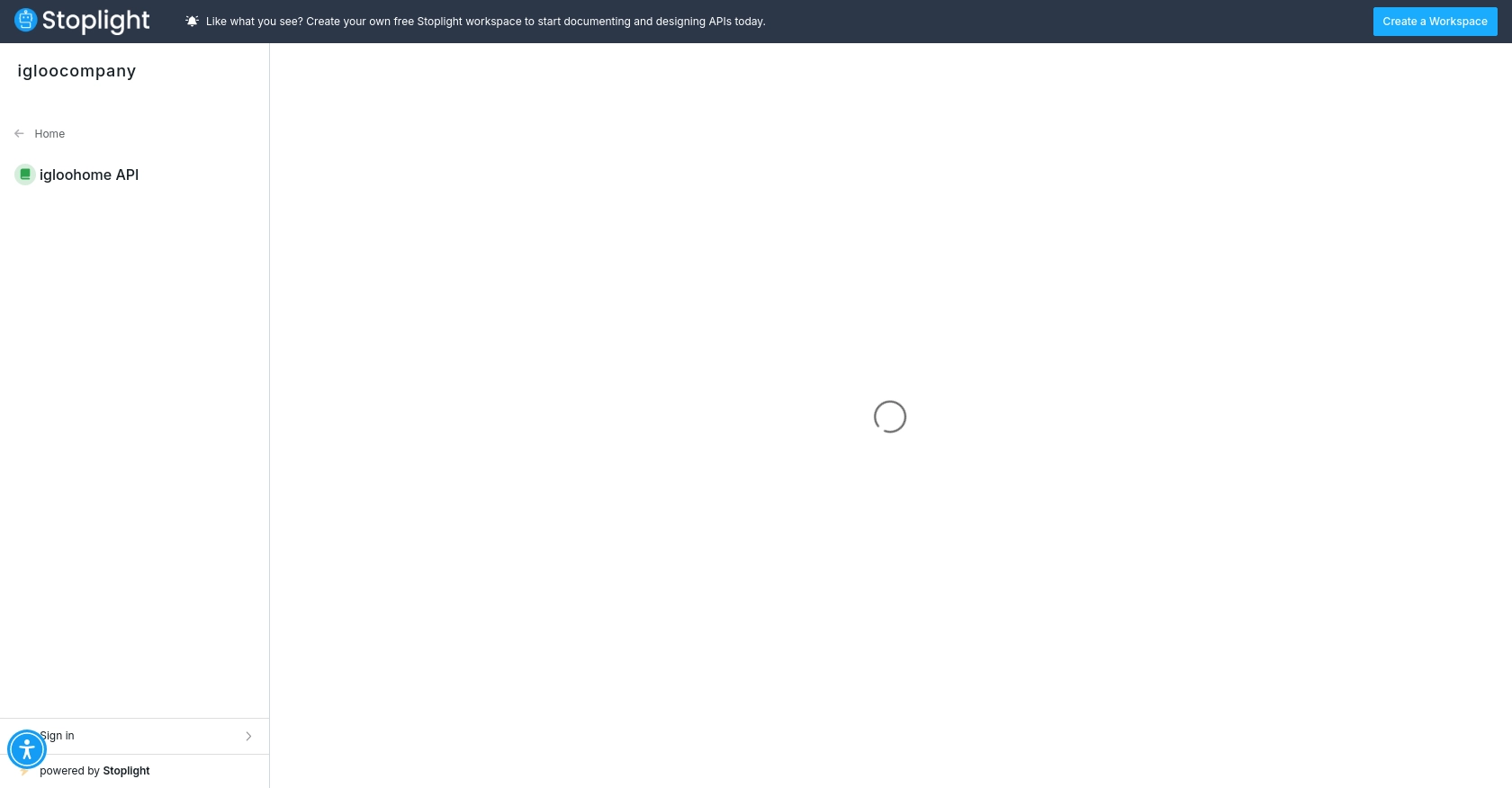
Conclusion and Best Practices for Using igloohome API with JavaScript
Integrating the igloohome API into your application can significantly enhance your smart access capabilities, providing users with secure and flexible entry management solutions. By following the steps outlined in this guide, you can efficiently create one-time PIN codes using JavaScript, ensuring a seamless experience for your users.
Best Practices for Secure and Efficient igloohome API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive from the API is standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and provide user-friendly messages when issues occur.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including igloohome. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website. Take advantage of an intuitive integration experience and streamline your development process today.
Read More
Ready to get started?