Using the Eventbrite API to Get Attendees (with PHP examples)
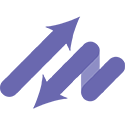
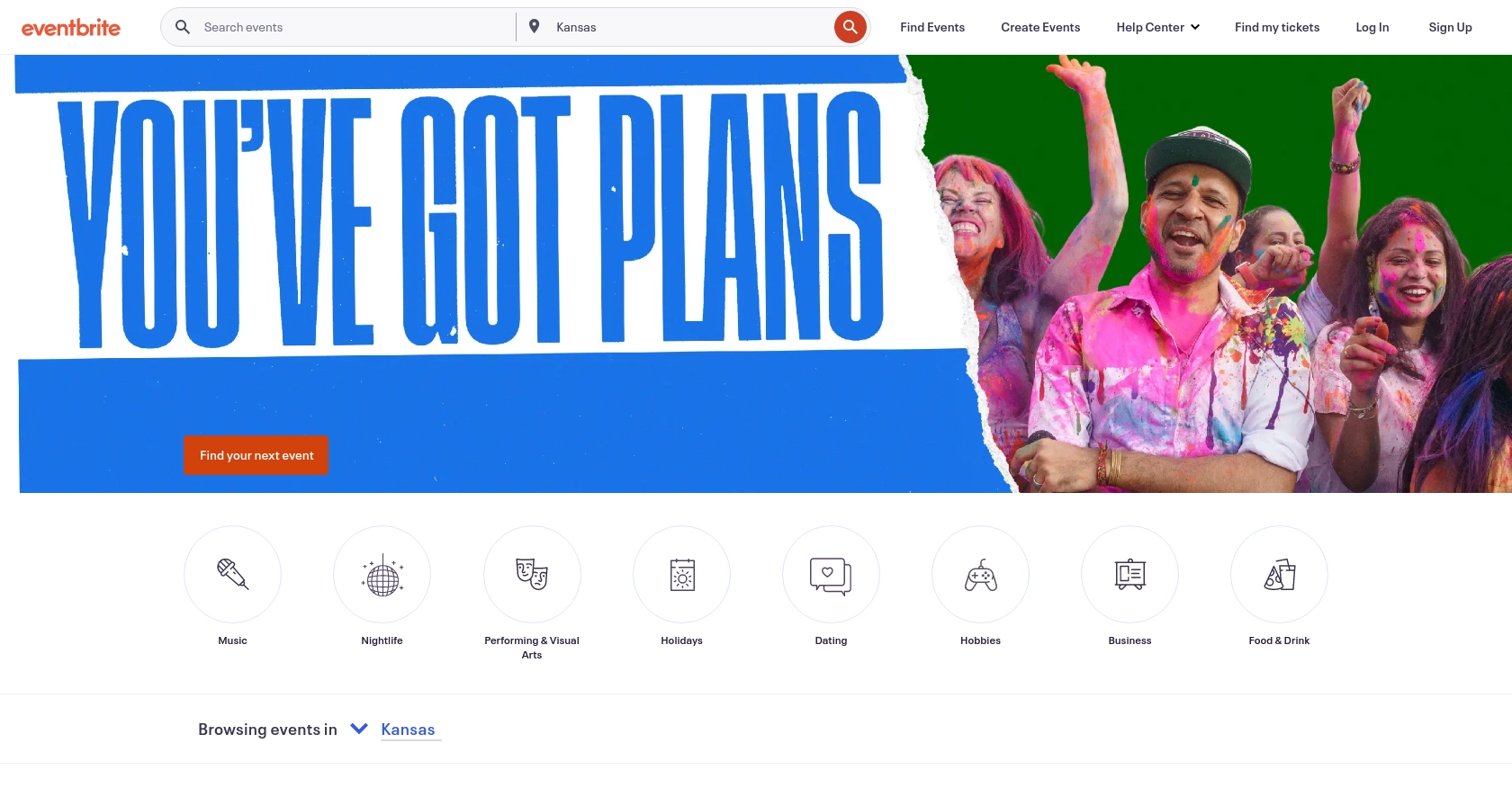
Introduction to Eventbrite API Integration
Eventbrite is a popular event management and ticketing platform that enables users to create, promote, and manage events. It offers a robust API that allows developers to interact with various aspects of the platform, including events, attendees, and ticketing information.
Integrating with the Eventbrite API can be highly beneficial for developers looking to automate event management tasks. For example, you might want to retrieve a list of attendees for a specific event to sync with your CRM system, ensuring seamless communication and follow-up with participants.
This article will guide you through using PHP to interact with the Eventbrite API, focusing on retrieving attendee information efficiently. By the end of this tutorial, you'll be equipped to handle attendee data and integrate it into your applications.
Setting Up Your Eventbrite Developer Account and OAuth Authentication
Before you can start interacting with the Eventbrite API, you'll need to set up a developer account and configure OAuth authentication. This process will allow you to securely access Eventbrite's resources and retrieve attendee information.
Creating an Eventbrite Developer Account
To begin, you'll need to create a developer account on Eventbrite. Follow these steps:
- Visit the Eventbrite API platform and sign in with your Eventbrite account. If you don't have an account, you'll need to create one.
- Once logged in, navigate to the "Create App" section to register a new application. This will generate the necessary credentials for API access.
Configuring OAuth Authentication for Eventbrite API
The Eventbrite API uses OAuth 2.0 for authentication, which involves creating an app to obtain the client ID and client secret. Follow these steps to set up OAuth:
- In your Eventbrite developer account, go to the "Apps" section and click on "Create App."
- Fill in the required information, such as the app name, description, and redirect URI. The redirect URI is where users will be redirected after they authorize your app.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure, as they are essential for making authorized API requests.
Obtaining Access Tokens for API Requests
With your client ID and client secret, you can now obtain an access token to make API requests. Here's how:
- Direct users to the Eventbrite authorization URL, where they can grant your app permission to access their data.
- After authorization, users will be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the Eventbrite token endpoint, including your client ID, client secret, and authorization code.
Once you have the access token, you can use it to authenticate your API requests and retrieve attendee information.
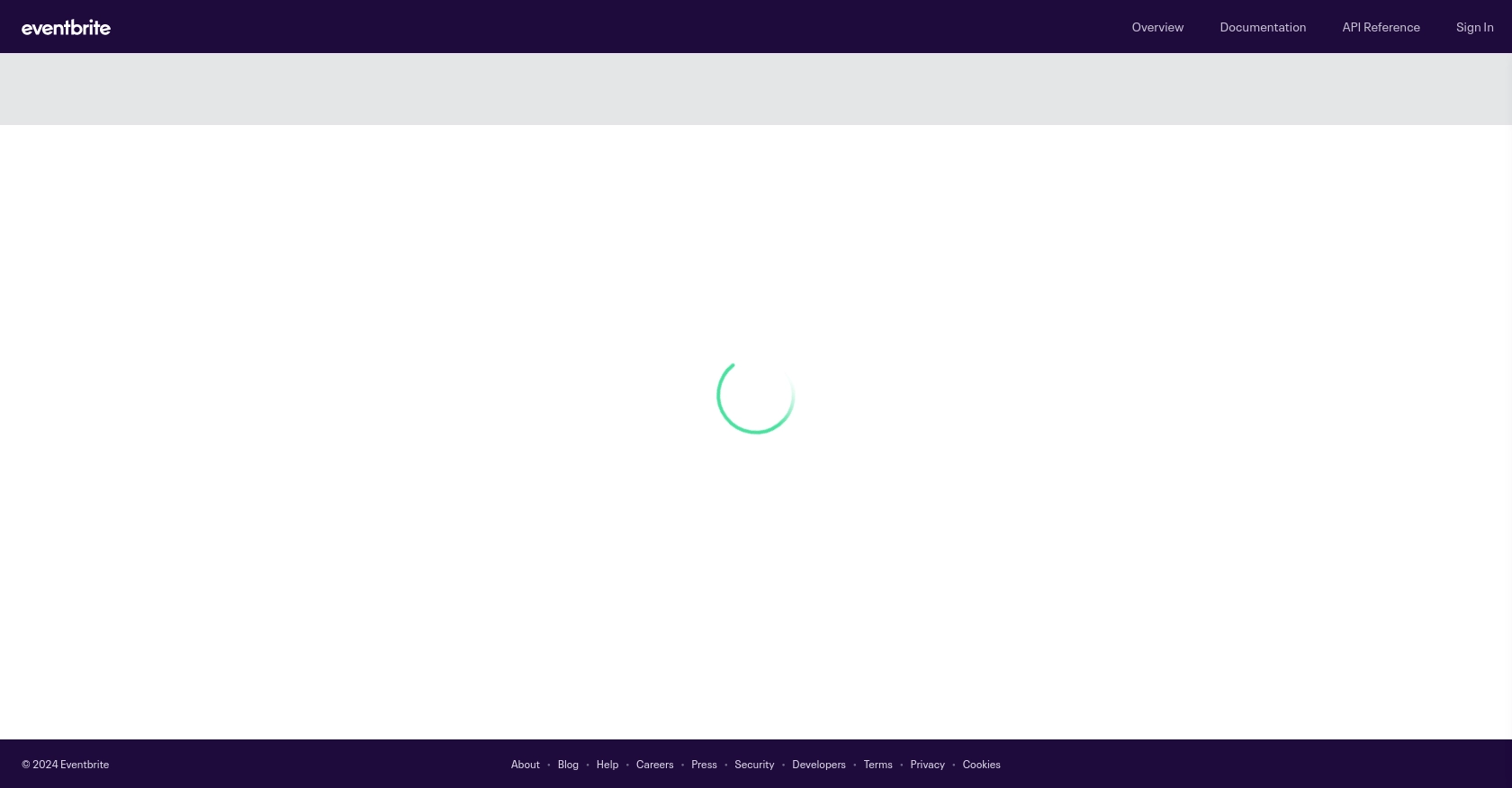
sbb-itb-96038d7
Making API Calls to Retrieve Eventbrite Attendees Using PHP
To interact with the Eventbrite API and retrieve attendee information, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment, installing necessary dependencies, and executing API calls to fetch attendee data.
Setting Up Your PHP Environment for Eventbrite API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
- The Guzzle HTTP client library for making HTTP requests
To install Guzzle, run the following command in your terminal:
composer require guzzlehttp/guzzle
Executing API Calls to Fetch Attendee Data from Eventbrite
With your environment set up, you can now write PHP code to interact with the Eventbrite API. Below is a sample script to retrieve attendees for a specific event:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$eventId = 'Your_Event_ID';
$response = $client->request('GET', "https://www.eventbriteapi.com/v3/events/{$eventId}/attendees/", [
'headers' => [
'Authorization' => "Bearer {$accessToken}",
'Accept' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$attendees = json_decode($response->getBody(), true);
foreach ($attendees['attendees'] as $attendee) {
echo "Name: " . $attendee['profile']['name'] . "\n";
echo "Email: " . $attendee['profile']['email'] . "\n";
}
} else {
echo "Failed to retrieve attendees. Status code: " . $response->getStatusCode();
}
Replace Your_Access_Token
and Your_Event_ID
with your actual access token and event ID.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of attendees printed in your terminal. To verify the request's success, ensure the status code is 200. If the request fails, the script will output the status code, helping you diagnose the issue.
Common error codes include:
- 401 Unauthorized: Check your access token.
- 404 Not Found: Verify the event ID.
- 429 Too Many Requests: You have hit the rate limit.
For more details, refer to the Eventbrite API documentation.
Conclusion: Best Practices for Eventbrite API Integration with PHP
Integrating with the Eventbrite API using PHP offers a powerful way to manage event data and automate processes. By following the steps outlined in this guide, you can efficiently retrieve attendee information and incorporate it into your applications.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always keep your client ID, client secret, and access tokens secure. Consider using environment variables or secure vaults to store sensitive information.
- Handle Rate Limiting: Be mindful of Eventbrite's rate limits to avoid disruptions. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. - Standardize Data: Transform and standardize attendee data to ensure consistency across your systems, making it easier to integrate with other platforms.
Streamlining Integrations with Endgrate
While integrating with the Eventbrite API is straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Eventbrite. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your integrations.
Read More
Ready to get started?