Using the Zapier API to Push Data in Javascript
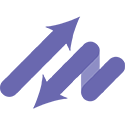
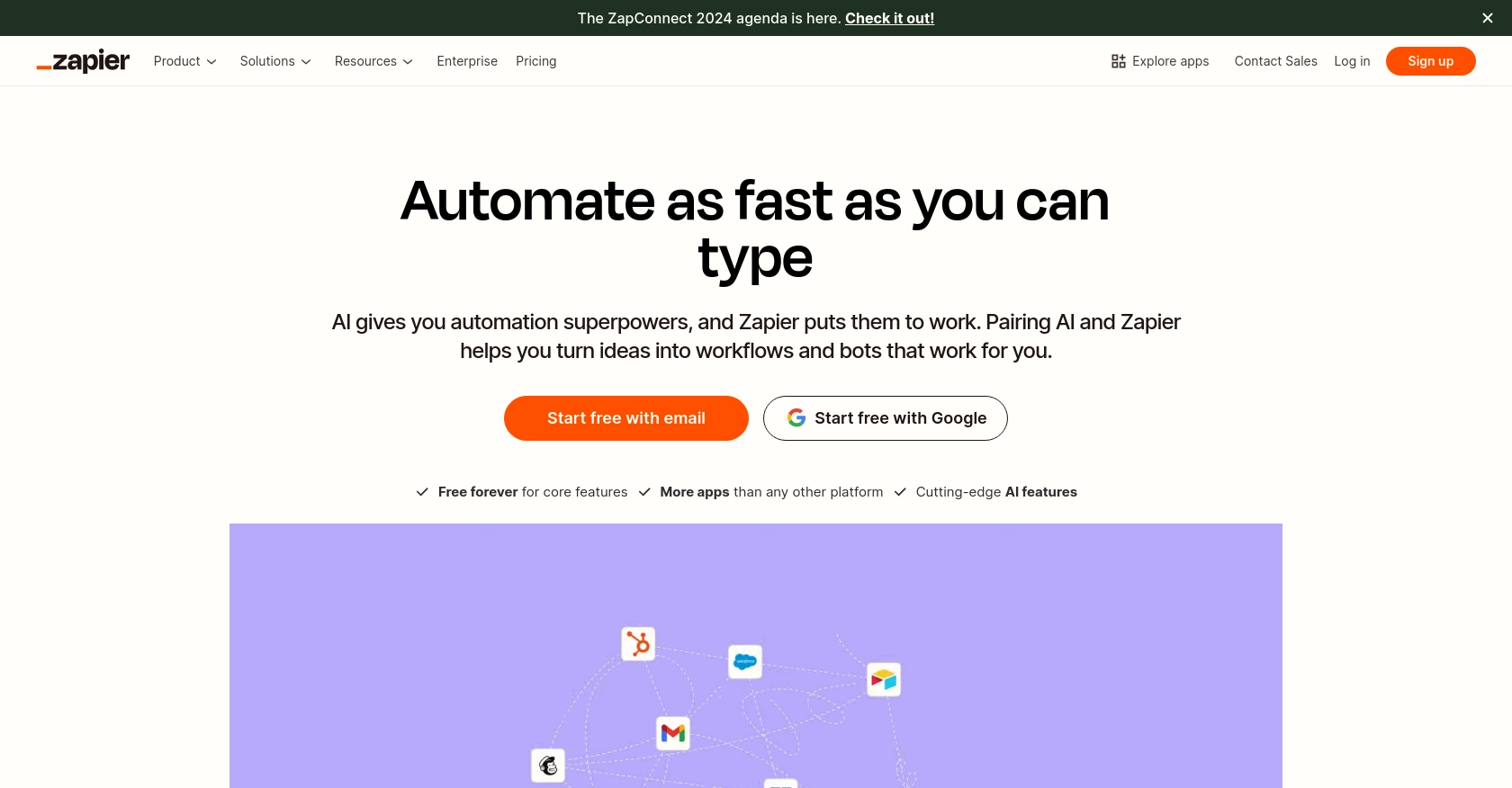
Introduction to Zapier API Integration
Zapier is a powerful automation tool that connects various apps and services, enabling seamless data transfer and workflow automation without the need for extensive coding. It supports a wide range of applications, making it a popular choice for businesses looking to streamline their operations.
Developers may want to integrate with the Zapier API to automate data transfer between different platforms. For example, you could use the Zapier API to push data from a custom application to a CRM system, ensuring that customer information is always up-to-date without manual intervention.
Setting Up Your Zapier Developer Account for API Integration
Before you can start using the Zapier API to push data with JavaScript, you'll need to set up a Zapier developer account. This account will allow you to create and manage integrations, test API calls, and authenticate your applications.
Creating a Zapier Developer Account
- Visit the Zapier sign-up page and create a free account if you haven't already.
- Once logged in, navigate to the Zapier Developer Platform.
- Click on "Create a New Integration" to start building your integration.
Configuring Authentication for Zapier API
Zapier supports various authentication methods, including API Key, OAuth v2, and Session Authentication. For this tutorial, we will focus on setting up OAuth v2, which is the preferred method for secure integrations.
- In the Zapier Developer Platform, select your integration and navigate to the "Authentication" section.
- Choose "OAuth v2" as your authentication method.
- Provide the necessary details such as Client ID, Client Secret, Authorization URL, and Token URL. These details are typically obtained from the service you are integrating with.
- Set up the scopes required for your integration to access the necessary data.
Testing Your Zapier Integration
Once your authentication is configured, it's important to test your integration to ensure everything is set up correctly.
- Navigate to the "Test" section in the Zapier Developer Platform.
- Use the provided tools to simulate API calls and verify that your authentication is working as expected.
- Check the response data to ensure it matches the expected output.
For more detailed guidance on setting up authentication, refer to the Zapier Authentication Documentation.
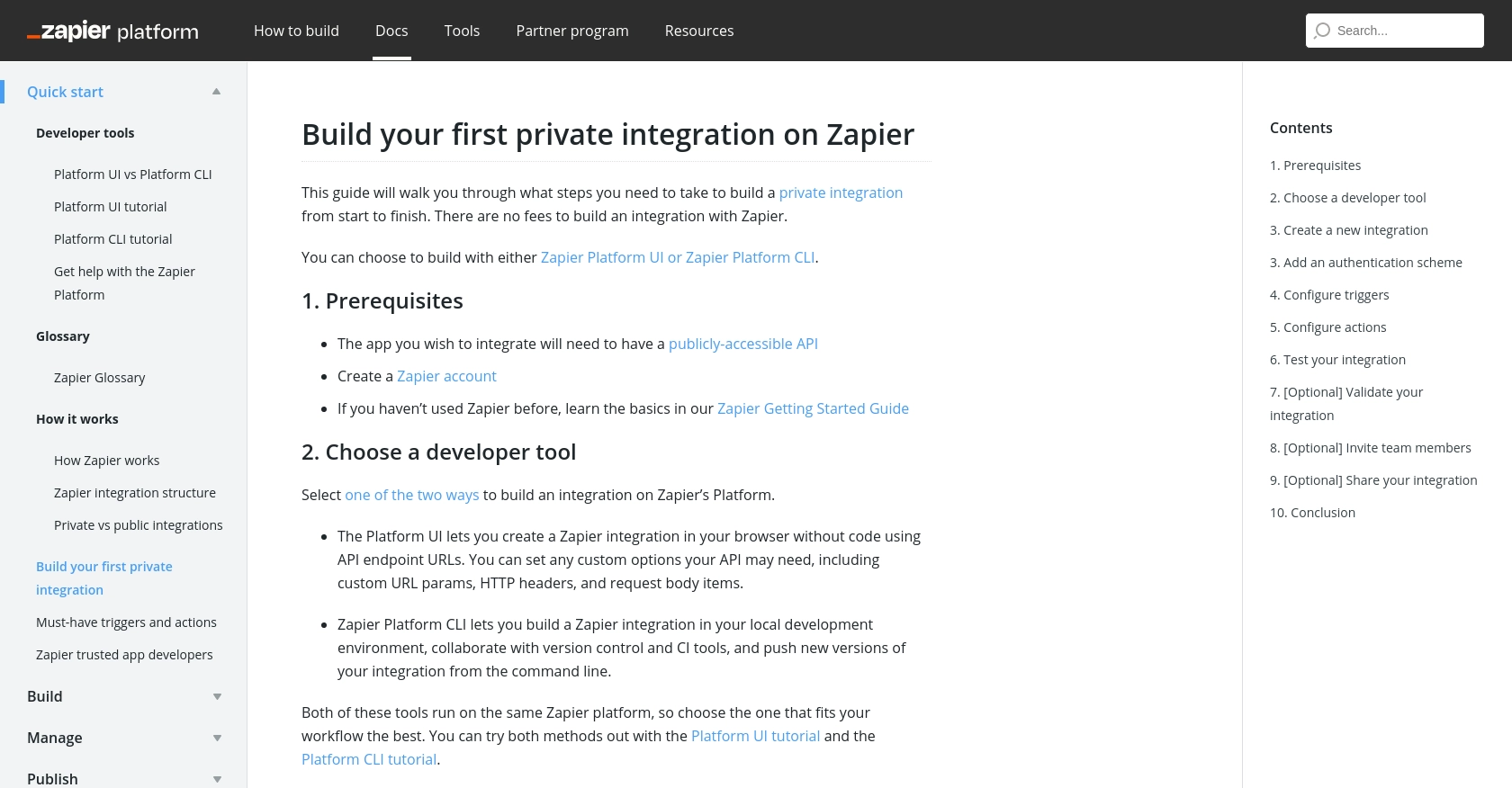
sbb-itb-96038d7
Making API Calls with Zapier in JavaScript
To push data using the Zapier API with JavaScript, you'll need to understand the basics of making HTTP requests and handling responses. This section will guide you through setting up your development environment, writing the necessary code, and verifying the success of your API calls.
Setting Up Your JavaScript Environment for Zapier API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Basic knowledge of JavaScript and HTTP requests.
To install the necessary dependencies, open your terminal and run:
npm install axios
The axios
library will help you make HTTP requests easily.
Writing JavaScript Code to Push Data to Zapier API
Now, let's write a simple script to push data to the Zapier API. Create a file named pushDataToZapier.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://hooks.zapier.com/hooks/catch/your_webhook_id/';
const headers = {
'Content-Type': 'application/json'
};
// Define the data to push
const data = {
name: 'John Doe',
email: 'john.doe@example.com'
};
// Make a POST request to the Zapier API
axios.post(endpoint, data, { headers })
.then(response => {
console.log('Data pushed successfully:', response.data);
})
.catch(error => {
console.error('Error pushing data:', error.response ? error.response.data : error.message);
});
Replace your_webhook_id
with the actual webhook ID provided by Zapier for your integration.
Verifying the Success of Your Zapier API Call
After running your script, you should verify that the data was successfully pushed to Zapier:
- Check the console output for a success message.
- Log into your Zapier account and navigate to the Zap that uses this webhook.
- Ensure that the data appears as expected in the Zap's task history.
If you encounter errors, refer to the error messages in the console and consult the Zapier Action Documentation for troubleshooting tips.
Handling Errors and Common Error Codes in Zapier API
When making API calls, it's crucial to handle potential errors gracefully. Here are some common error codes you might encounter:
- 400 Bad Request: Check your request syntax and data format.
- 401 Unauthorized: Verify your authentication credentials.
- 403 Forbidden: Ensure you have the necessary permissions.
- 404 Not Found: Confirm the endpoint URL is correct.
- 500 Internal Server Error: Retry the request or contact Zapier support if the issue persists.
For more detailed error handling strategies, refer to the Zapier Test Authentication Documentation.
Conclusion and Best Practices for Using Zapier API with JavaScript
Integrating with the Zapier API using JavaScript can significantly enhance your application's automation capabilities, allowing seamless data transfer between various platforms. By following the steps outlined in this guide, you can efficiently set up and manage your Zapier integrations.
Best Practices for Secure and Efficient Zapier API Integration
- Securely Store Credentials: Always store your API credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Zapier's rate limits to avoid throttling. Implement exponential backoff strategies for retrying failed requests.
- Standardize Data Formats: Ensure data consistency by standardizing formats before pushing data to Zapier, reducing errors and improving integration reliability.
- Monitor and Log API Calls: Keep track of your API interactions by logging requests and responses. This will help in troubleshooting and optimizing performance.
Streamlining Integration Development with Endgrate
While building integrations with Zapier can be powerful, it can also be time-consuming. Endgrate offers a solution to simplify this process by providing a unified API endpoint for multiple platforms, including Zapier. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zapier
- https://platform.zapier.com/quickstart/build-private-integration
- https://platform.zapier.com/build/auth
- https://platform.zapier.com/manage/sharing
- https://platform.zapier.com/build/trigger
- https://platform.zapier.com/build/action
- https://platform.zapier.com/build/test-auth
- https://platform.zapier.com/publish/integration-checks-reference
Ready to get started?