How to Get Candidates with the Zoho Recruit API in Python
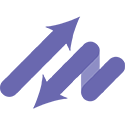
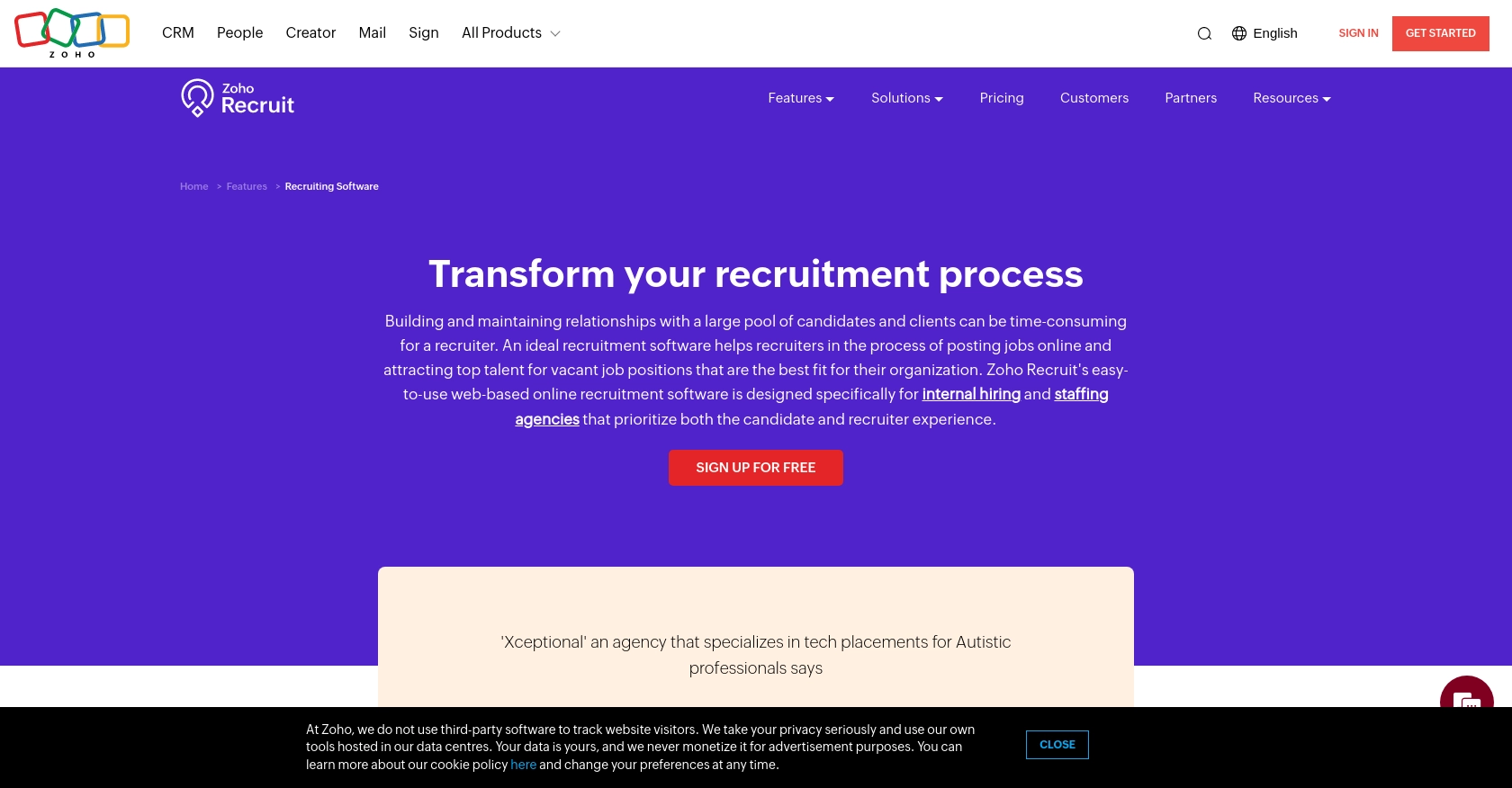
Introduction to Zoho Recruit
Zoho Recruit is a comprehensive recruitment software solution designed to streamline the hiring process for businesses of all sizes. It offers a suite of tools that facilitate candidate sourcing, resume management, and interview scheduling, making it a popular choice for staffing agencies and corporate HR departments.
Integrating with the Zoho Recruit API allows developers to automate and enhance recruitment workflows. For example, you can use the API to retrieve candidate information and seamlessly integrate it into your internal systems, improving efficiency and reducing manual data entry.
Setting Up Your Zoho Recruit Test/Sandbox Account
Before you can begin interacting with the Zoho Recruit API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Zoho Recruit Account
If you don't already have a Zoho Recruit account, you can sign up for a free trial or use the free version. Visit the Zoho Recruit website and follow the instructions to create your account. Once your account is set up, log in to access the Zoho Recruit dashboard.
Registering a Zoho Recruit Application for OAuth Authentication
To interact with the Zoho Recruit API, you need to register your application to obtain the necessary credentials for OAuth authentication. Follow these steps:
- Go to the Zoho Developer Console.
- Select the appropriate client type for your application. For most web applications, choose "Web-Based".
- Enter the required details:
- Client Name: A name for your application.
- Homepage URL: The URL of your application's homepage.
- Authorized Redirect URIs: The URL where Zoho will redirect after successful authentication.
- Click Create to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating OAuth Tokens
With your application registered, you can now generate OAuth tokens to authenticate API requests:
- Direct the user to the Zoho authorization URL with the necessary scopes, such as
ZohoRecruit.modules.candidates.READ
. - Upon user consent, Zoho will redirect to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token and refresh token by making a POST request to Zoho's token endpoint.
For detailed steps on generating tokens, refer to the Zoho Recruit OAuth Overview.
Testing Your Setup
With your test account and OAuth tokens ready, you can now proceed to make API calls to Zoho Recruit. Ensure that you use the sandbox environment to verify your setup and experiment with API interactions safely.
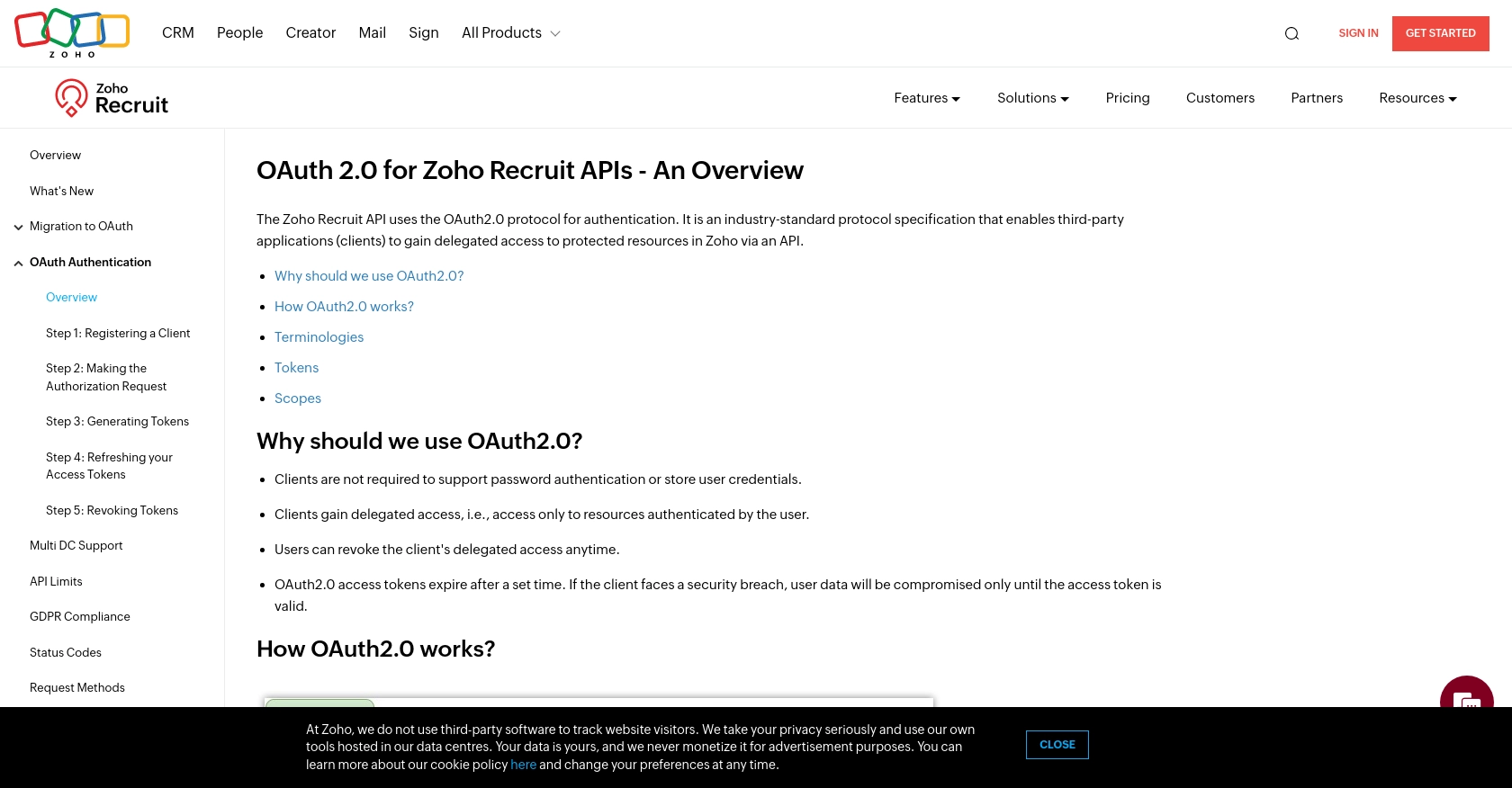
sbb-itb-96038d7
Making API Calls to Retrieve Candidates from Zoho Recruit Using Python
To interact with the Zoho Recruit API and retrieve candidate data, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through the process of setting up your Python environment, making the API call, and handling the response.
Setting Up Your Python Environment for Zoho Recruit API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Fetch Candidates from Zoho Recruit
Create a new Python file named get_candidates.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://recruit.zoho.com/recruit/v2/Candidates"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token"
}
# Make a GET request to the Zoho Recruit API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
candidates = response.json().get("data", [])
for candidate in candidates:
print(candidate)
else:
print(f"Failed to retrieve candidates: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth authentication process.
Executing the Python Script to Retrieve Candidate Data
Run the script from your terminal or command line:
python get_candidates.py
If successful, the script will print out the candidate data retrieved from Zoho Recruit. If there's an error, it will display the status code and error message.
Handling API Response and Errors from Zoho Recruit
It's crucial to handle potential errors when making API calls. Zoho Recruit may return various status codes indicating success or failure:
- 200 OK: The request was successful.
- 400 BAD REQUEST: The request is invalid.
- 401 AUTHORIZATION ERROR: Invalid API key provided.
- 403 FORBIDDEN: No permission to perform the operation.
- 429 TOO MANY REQUESTS: API request limit exceeded.
For more details on status codes, refer to the Zoho Recruit Status Codes Documentation.
Verifying API Call Success in Zoho Recruit Sandbox
After running your script, verify the data retrieval by checking the sandbox environment in Zoho Recruit. Ensure the candidates listed match the data returned by your API call.
By following these steps, you can efficiently retrieve candidate data from Zoho Recruit using Python, streamlining your recruitment processes and enhancing your application's capabilities.
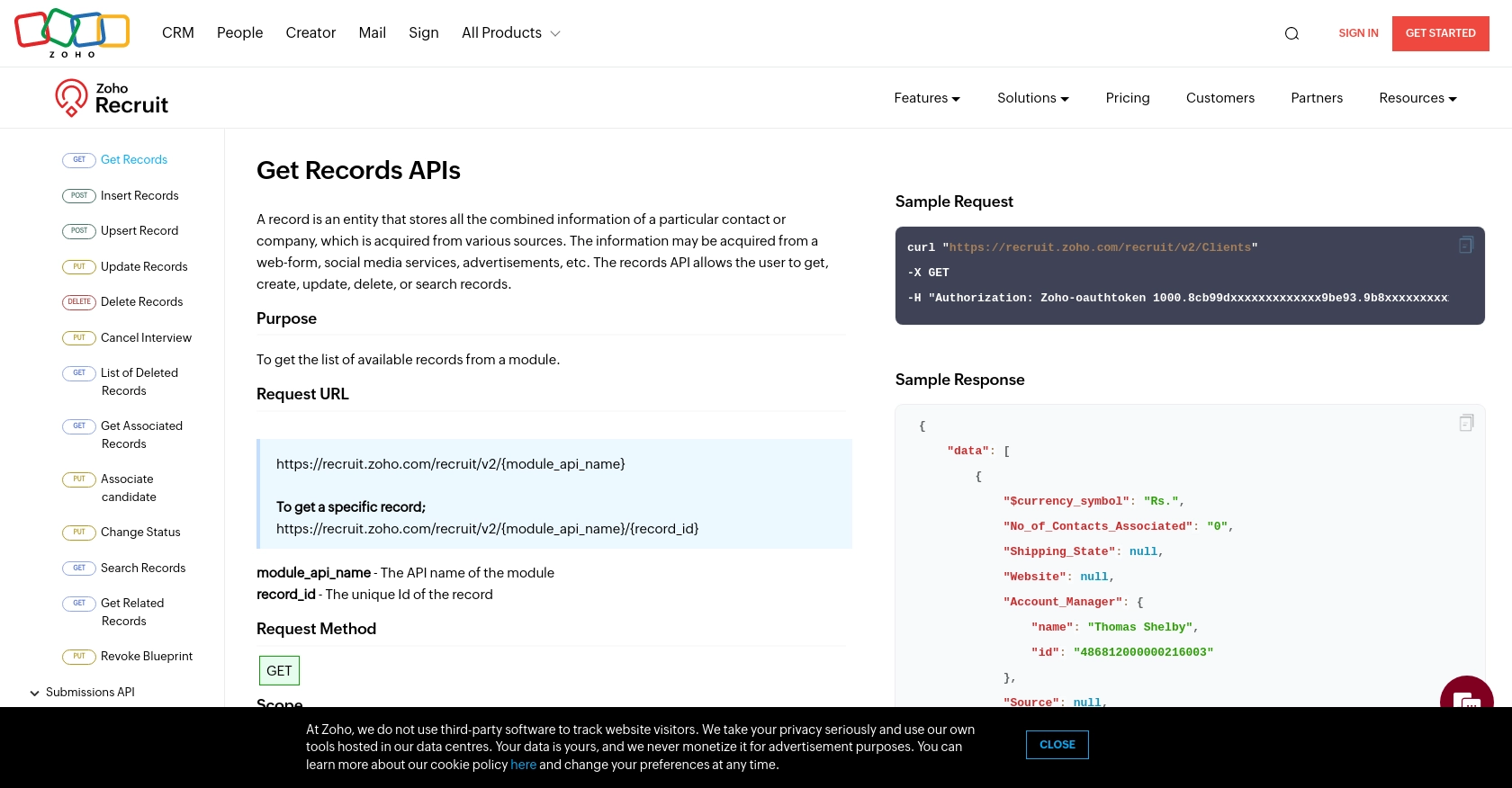
Conclusion and Best Practices for Zoho Recruit API Integration
Integrating with the Zoho Recruit API using Python provides a powerful way to automate and enhance your recruitment processes. By retrieving candidate data programmatically, you can streamline workflows, reduce manual data entry, and improve overall efficiency.
Best Practices for Secure and Efficient Zoho Recruit API Use
- Securely Store Credentials: Always keep your OAuth tokens, client ID, and client secret secure. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limits: Be mindful of Zoho Recruit's API rate limits. Implement logic to handle the
429 TOO MANY REQUESTS
error by retrying requests after a delay. For more details, refer to the Zoho Recruit API Limits Documentation. - Manage Data Consistency: Regularly update and synchronize data between your systems and Zoho Recruit to ensure consistency and accuracy.
- Use Scopes Wisely: Define and use scopes that provide only the necessary permissions for your application, enhancing security.
Enhancing Your Integration Strategy with Endgrate
While integrating with Zoho Recruit is a significant step, managing multiple integrations can be complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Zoho Recruit. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohorecruit
- https://www.zoho.com/recruit/developer-guide/apiv2/oauth-overview.html
- https://www.zoho.com/recruit/developer-guide/apiv2/register-client.html
- https://www.zoho.com/recruit/developer-guide/apiv2/limits.html
- https://www.zoho.com/recruit/developer-guide/apiv2/status-codes.html
- https://www.zoho.com/recruit/developer-guide/apiv2/get-records.html
Ready to get started?