Using the Hubspot API to Create Products (Professional/Enterprise Only) in Javascript
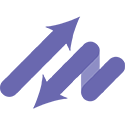
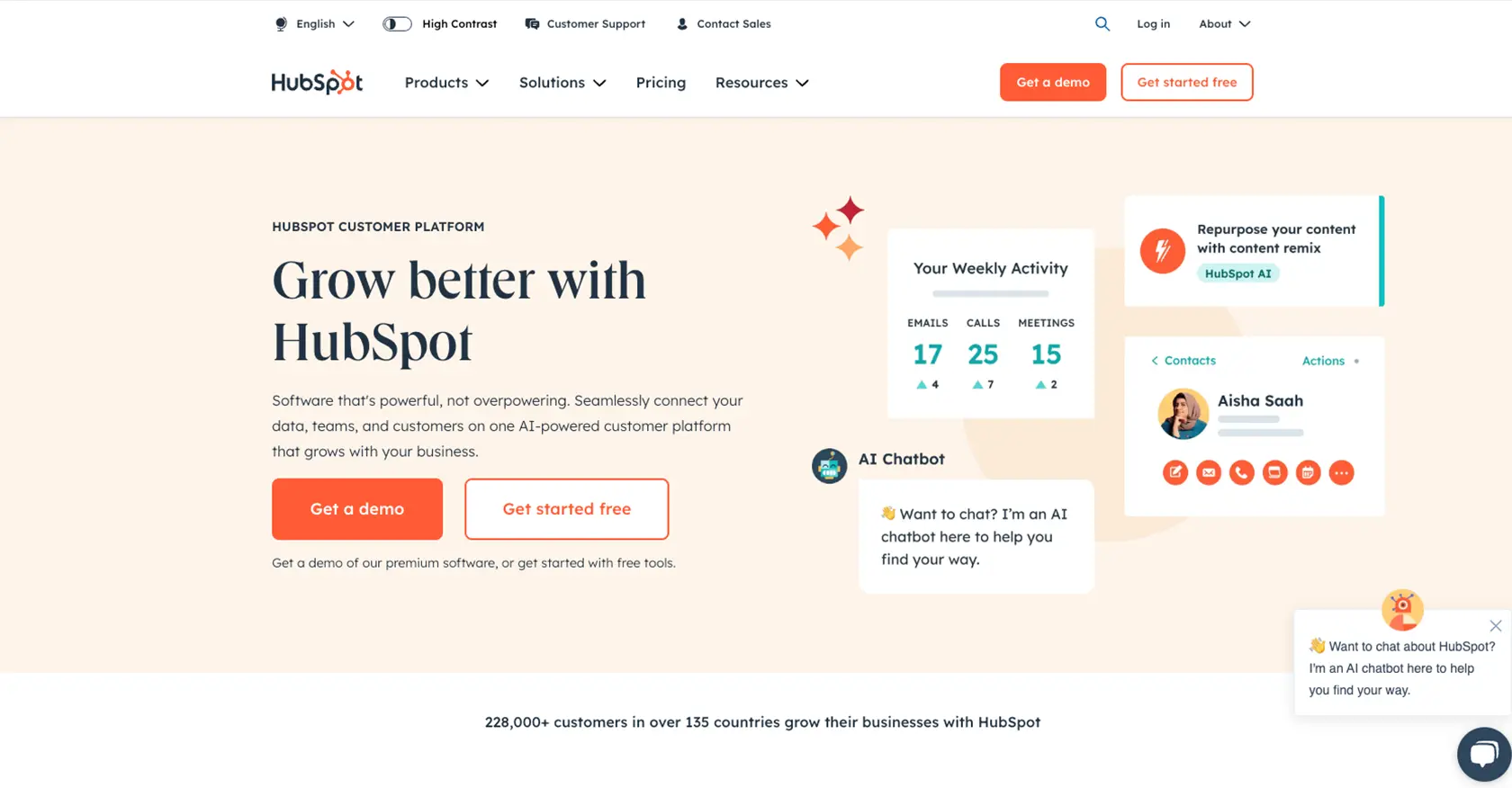
Introduction to HubSpot API for Product Management
HubSpot is a renowned CRM platform that offers a wide range of tools to enhance marketing, sales, and customer service efforts. Its robust API allows developers to integrate seamlessly with HubSpot's features, enabling businesses to automate and streamline their operations.
For developers working with B2B SaaS products, integrating with HubSpot's API can significantly enhance product management capabilities. By leveraging the API, developers can create, update, and manage product listings directly within HubSpot, ensuring that product data is consistent and up-to-date across all platforms.
In this article, we will explore how to use JavaScript to create products in HubSpot, specifically for Professional and Enterprise accounts. This integration can be particularly useful for businesses looking to automate their product catalog management, ensuring that all product information is accurately reflected in their CRM system.
Setting Up Your HubSpot Developer Account for Product Integration
Before you can start creating products using the HubSpot API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your integration without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
To test your API integration, you'll need a sandbox account. Follow these steps to set it up:
- In your developer dashboard, navigate to the "Test Accounts" section.
- Click on "Create a test account" and select the Professional or Enterprise tier, as product creation is limited to these tiers.
- Once the sandbox account is created, you can use it to test API calls without impacting your live data.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for authentication, which requires setting up an app to obtain the necessary credentials:
- In your developer account, go to the "Apps" section and click on "Create an app."
- Fill in the app details and configure the necessary scopes for product management. Ensure you include scopes related to product creation and management.
- Once the app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are needed for OAuth authentication.
- Follow the OAuth 2.0 quickstart guide to complete the authentication setup.
With your developer account, sandbox environment, and OAuth configuration in place, you're ready to start integrating with the HubSpot API to create products using JavaScript.
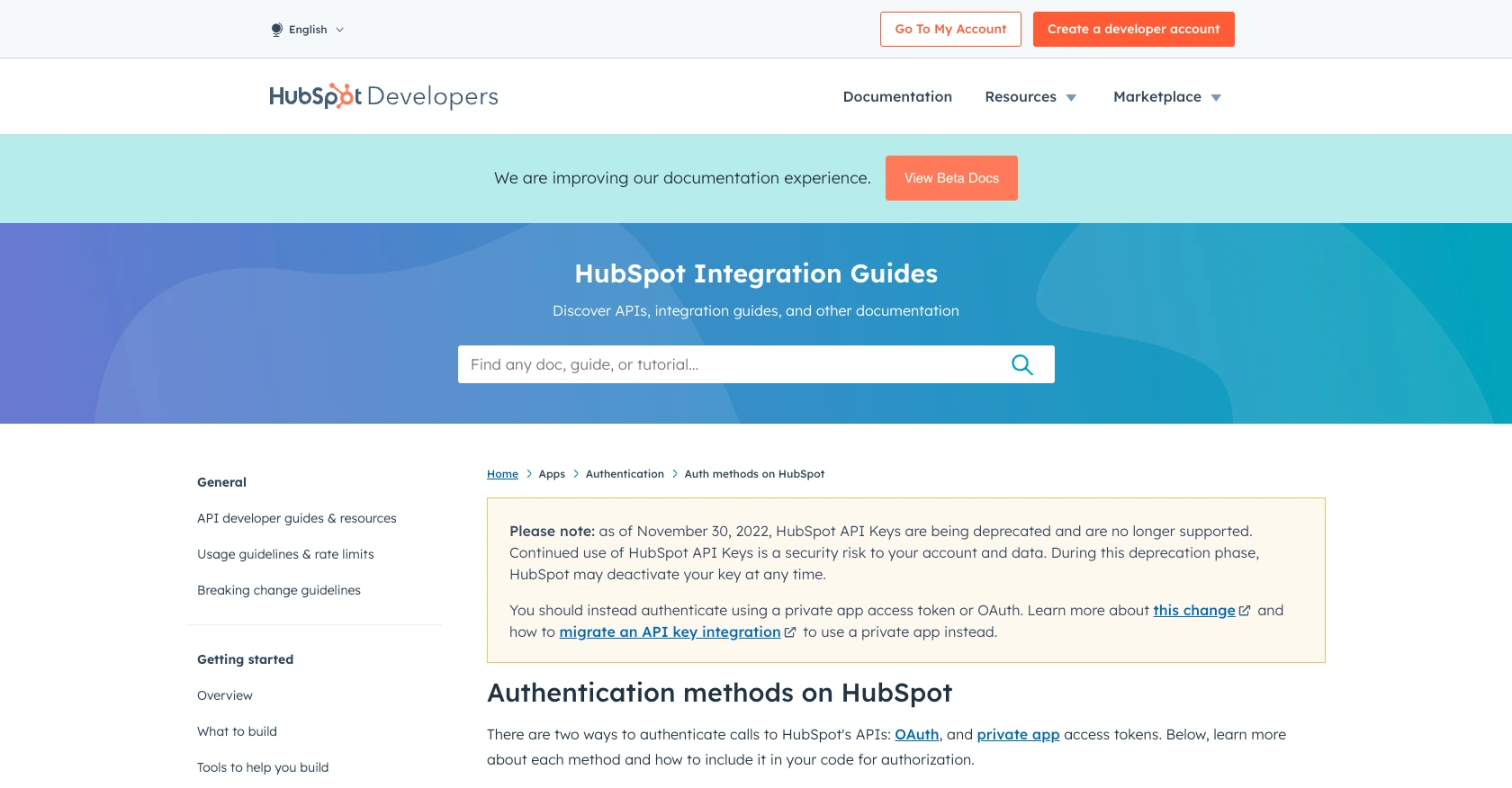
sbb-itb-96038d7
Making API Calls to Create Products in HubSpot Using JavaScript
To create products in HubSpot using JavaScript, you'll need to make API calls to the HubSpot CRM. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for HubSpot API Integration
Before making API calls, ensure you have Node.js installed on your machine. You will also need the @hubspot/api-client
package to interact with HubSpot's API.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the HubSpot API client by executing
npm install @hubspot/api-client
.
Writing JavaScript Code to Create Products in HubSpot
With your environment set up, you can now write the JavaScript code to create products in HubSpot. The following example demonstrates how to make a POST request to the HubSpot API to create a new product.
const hubspot = require('@hubspot/api-client');
// Initialize the HubSpot client
const hubspotClient = new hubspot.Client({ accessToken: 'YOUR_ACCESS_TOKEN' });
// Define the product data
const productData = {
properties: {
name: 'Sample Product',
description: 'This is a sample product description.',
price: '100.00',
currency: 'USD'
}
};
// Function to create a product
async function createProduct() {
try {
const response = await hubspotClient.crm.products.basicApi.create(productData);
console.log('Product created successfully:', response.body);
} catch (error) {
console.error('Error creating product:', error.response ? error.response.body : error);
}
}
// Call the function to create the product
createProduct();
Replace YOUR_ACCESS_TOKEN
with the OAuth access token obtained during the authentication setup. The productData
object contains the properties of the product you wish to create.
Handling API Responses and Errors
After making the API call, it's crucial to handle the response correctly. If the product is created successfully, the response will contain the product details. In case of an error, the catch block will log the error message.
Check the HubSpot sandbox account to verify that the product has been created. If the API call fails, review the error message for details and ensure your access token and product data are correct.
Understanding HubSpot API Rate Limits and Best Practices
HubSpot imposes rate limits on API calls to ensure fair usage. For Professional and Enterprise accounts, the limit is 150 requests per 10 seconds per private app. Exceeding this limit will result in a 429 error response. For more details, refer to the HubSpot API usage guidelines.
To optimize your integration, consider implementing caching strategies and batching requests where possible. This will help reduce the number of API calls and improve performance.
.webp)
Conclusion and Best Practices for Using HubSpot API to Create Products in JavaScript
Integrating with the HubSpot API to manage products can greatly enhance your business operations by ensuring that product information is consistently updated across platforms. By following the steps outlined in this guide, you can efficiently create products using JavaScript, leveraging HubSpot's robust CRM capabilities.
Best Practices for Secure and Efficient HubSpot API Integration
- Secure Storage of Credentials: Always store your OAuth access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of HubSpot's API rate limits. Implement retry logic with exponential backoff to handle 429 errors gracefully. Refer to the HubSpot API usage guidelines for more information.
- Data Standardization: Ensure that your product data is standardized before making API calls. This helps maintain data consistency across your CRM and other integrated platforms.
- Error Handling: Implement comprehensive error handling to capture and log errors. This will aid in troubleshooting and maintaining a smooth integration experience.
Leveraging Endgrate for Streamlined Integrations
While integrating with HubSpot's API can be powerful, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and seamlessly connect with multiple platforms, including HubSpot.
Explore how Endgrate can save you time and resources by visiting Endgrate's website and discover an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/products
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?