Using the Pipedrive API to Get People in Python
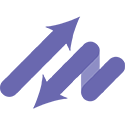
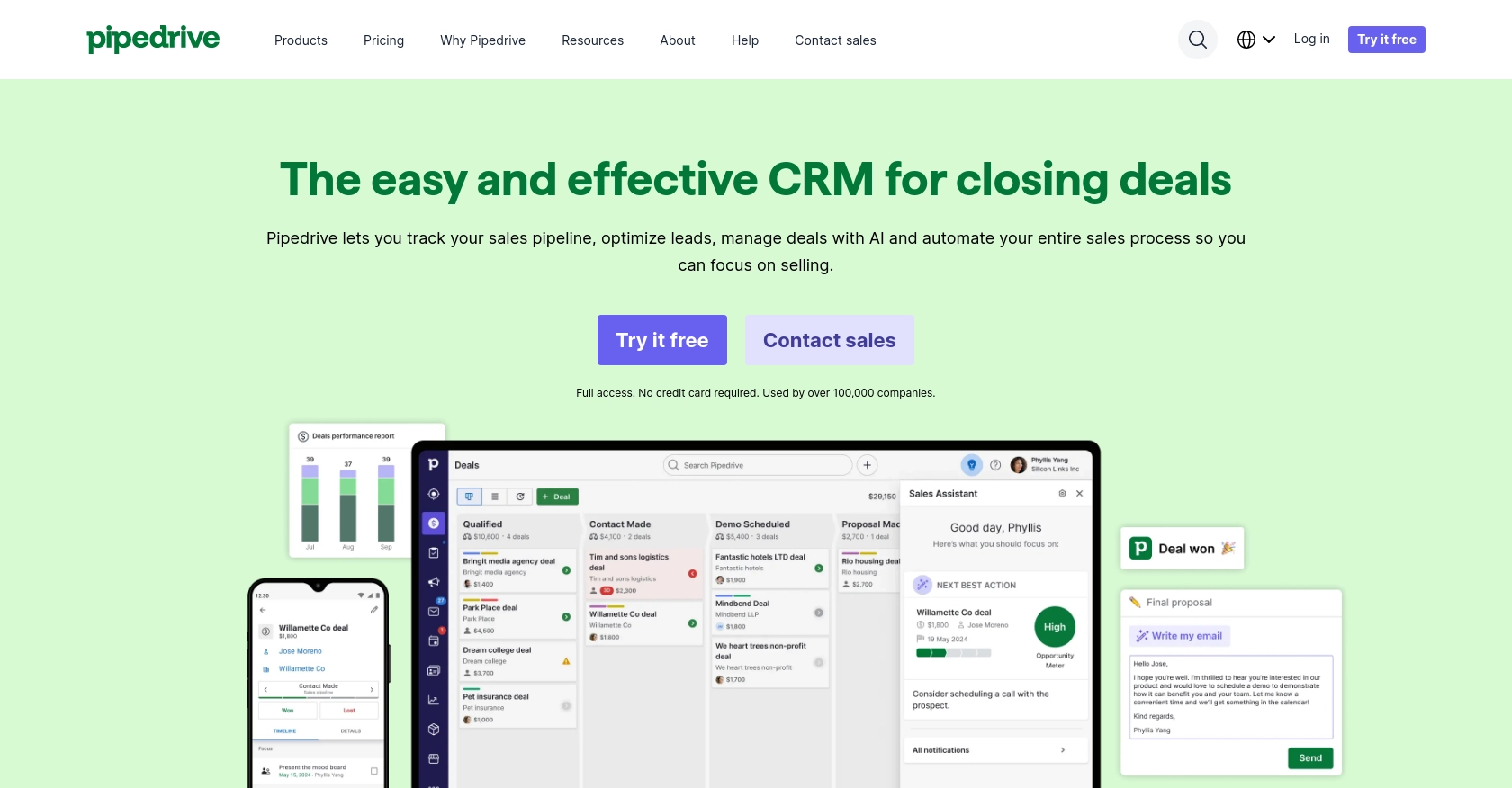
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes efficiently. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage customer relationships, and close deals more effectively.
Integrating with Pipedrive's API allows developers to automate and enhance sales operations by accessing and managing customer data programmatically. For example, you can use the Pipedrive API to retrieve a list of people (contacts) and integrate this data into other systems, such as marketing automation tools, to streamline communication and improve customer engagement.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you'll need to set up a developer sandbox account. This account provides a risk-free environment to test and develop your applications without affecting real data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account but is limited to five seats by default.
- Once your account is set up, you can import sample data to familiarize yourself with Pipedrive's features. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets.
Creating a Pipedrive App for OAuth Authentication
Since the Pipedrive API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- Log in to your sandbox account and go to the Developer Hub.
- Register your app by providing the required information, such as the app name and description.
- Once registered, you'll receive a client ID and client secret. These credentials are essential for implementing the OAuth flow.
- Ensure your app has the proper scopes and permissions to access the data you need. Refer to the Pipedrive documentation for more details on scopes.
With your sandbox account and app set up, you're ready to start making API calls to Pipedrive. In the next section, we'll explore how to use Python to interact with the Pipedrive API and retrieve a list of people.
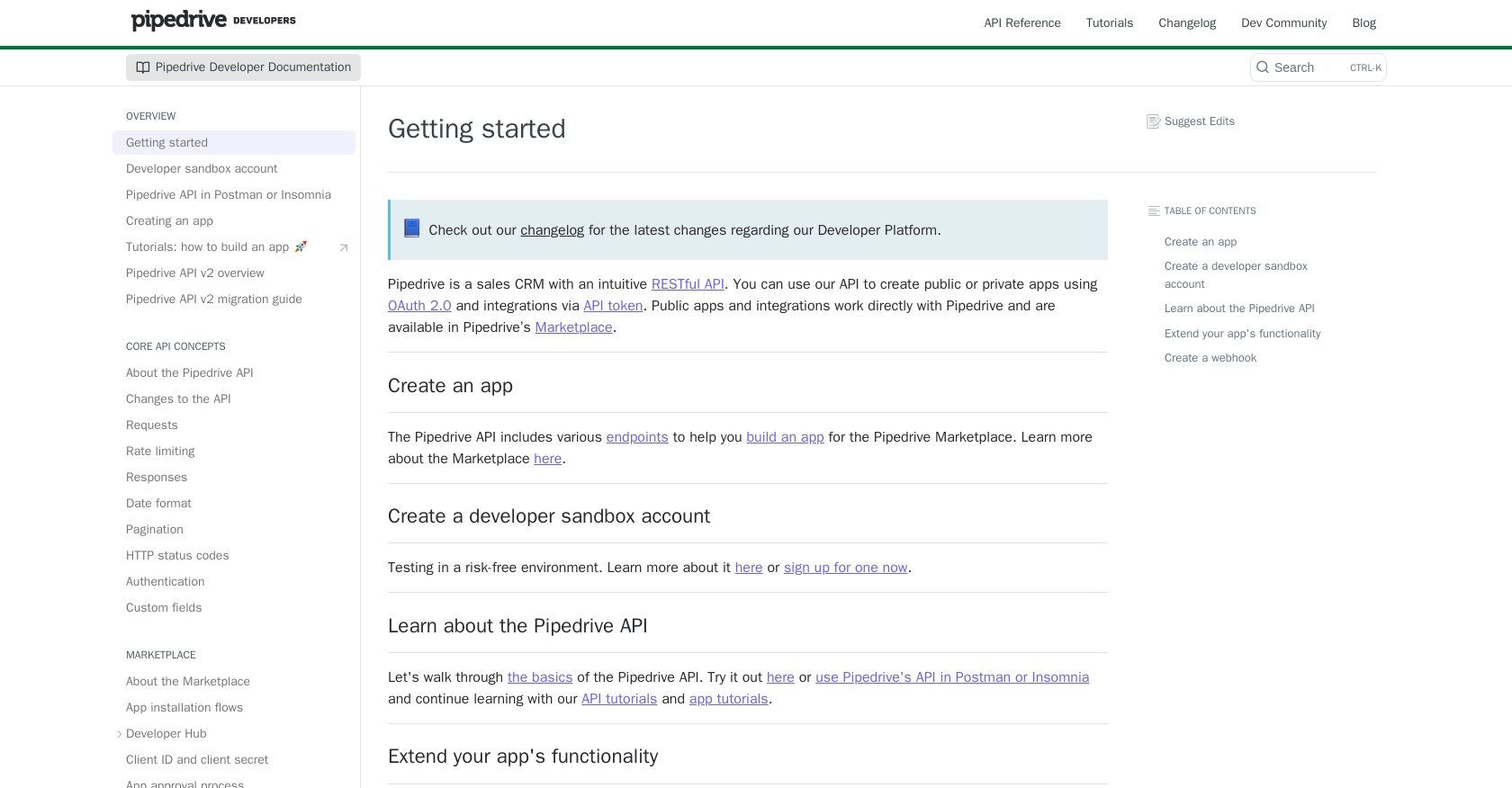
sbb-itb-96038d7
Making API Calls to Retrieve People from Pipedrive Using Python
To interact with the Pipedrive API and retrieve a list of people, we'll use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your Python Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Retrieve People from Pipedrive
Create a file named get_pipedrive_people.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.pipedrive.com/v1/persons"
headers = {
"Authorization": "Bearer Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the people and print their information
for person in data["data"]:
print(person)
else:
print(f"Failed to retrieve people: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth flow.
Understanding the Sample Output and Verifying Success
When you run the script using the command below, you should see a list of people from your Pipedrive sandbox account:
python get_pipedrive_people.py
The output will display the details of each person, confirming that the API call was successful. You can verify the retrieved data by checking the Pipedrive sandbox account for consistency.
Handling Errors and Understanding Pipedrive API Error Codes
It's crucial to handle potential errors when making API calls. The Pipedrive API may return various HTTP status codes, such as:
- 200 OK: Request fulfilled successfully.
- 401 Unauthorized: Invalid API token.
- 429 Too Many Requests: Rate limit exceeded.
For a complete list of status codes, refer to the Pipedrive documentation.
Managing Pipedrive API Rate Limits
The Pipedrive API enforces rate limits to ensure fair usage. For OAuth apps, the rate limit is 80 requests per 2 seconds per access token. If you exceed this limit, you'll receive a 429 error. To avoid this, consider implementing request throttling or upgrading your plan. More details can be found in the Pipedrive rate limiting documentation.
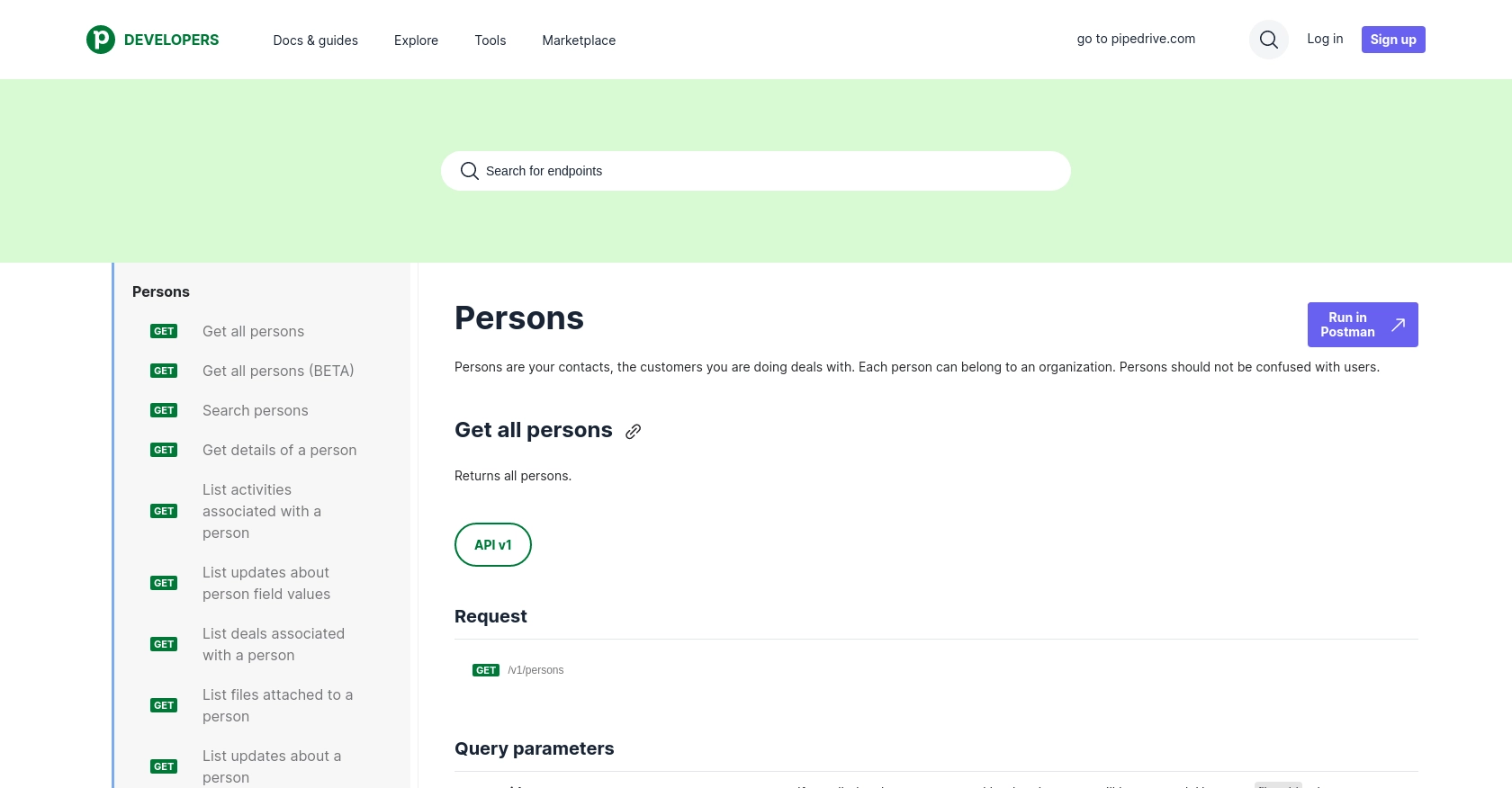
Conclusion and Best Practices for Using Pipedrive API in Python
Integrating with the Pipedrive API using Python offers a powerful way to automate and enhance your sales operations. By following the steps outlined in this guide, you can efficiently retrieve and manage contact data, enabling seamless integration with other systems and improving overall workflow efficiency.
Best Practices for Secure and Efficient Pipedrive API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Pipedrive's rate limits. Implement request throttling and monitor your API usage to avoid exceeding limits. For more details, refer to the Pipedrive rate limiting documentation.
- Implement Error Handling: Ensure robust error handling in your code to manage different HTTP status codes and unexpected responses. This will improve the reliability of your integration.
- Optimize Data Handling: Transform and standardize data fields as needed to ensure compatibility with other systems. This will help maintain data integrity across platforms.
Streamline Your Integrations with Endgrate
While integrating with Pipedrive is a valuable endeavor, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Pipedrive. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Persons
- https://developers.pipedrive.com/docs/api/v1/PersonFields
Ready to get started?