Using the Salesforce API to Create or Update Records (with PHP examples)
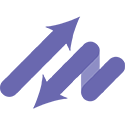
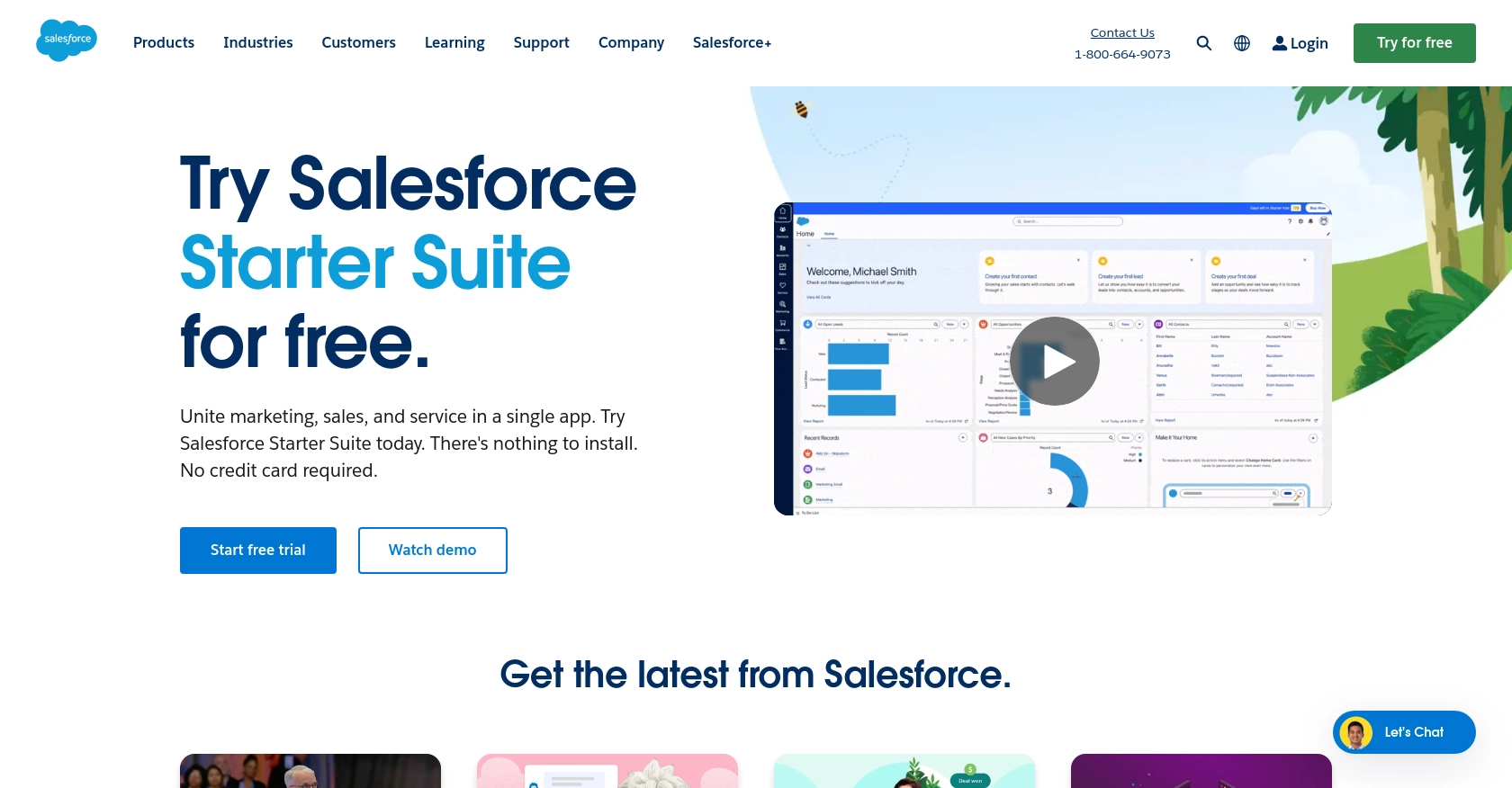
Introduction to Salesforce API Integration
Salesforce is a leading customer relationship management (CRM) platform that empowers businesses to manage their sales, customer service, and marketing efforts effectively. With its robust suite of tools, Salesforce enables organizations to streamline operations and enhance customer engagement.
Developers often seek to integrate with Salesforce's API to automate and optimize business processes, such as creating or updating records. For example, a developer might use the Salesforce API to automatically update customer information in the CRM whenever a new purchase is made, ensuring that sales teams have the most current data at their fingertips.
This article will guide you through using the Salesforce API with PHP to create or update records, providing detailed steps and code examples to help you leverage Salesforce's powerful capabilities in your applications.
Setting Up Your Salesforce Developer Account and Sandbox Environment
Before you can start integrating with the Salesforce API, you'll need to set up a Salesforce Developer Account and configure a sandbox environment. This will allow you to test your API interactions without affecting live data.
Creating a Salesforce Developer Account
If you don't already have a Salesforce Developer Account, you can sign up for free. This account will give you access to a Salesforce Developer Edition, which includes a sandbox environment for testing.
- Visit the Salesforce Developer Signup Page.
- Fill out the registration form with your details and submit it.
- Check your email for a verification link from Salesforce and follow the instructions to activate your account.
Configuring a Salesforce Sandbox Environment
Once your Developer Account is set up, you can configure a sandbox environment to safely test your API interactions.
- Log in to your Salesforce Developer Account.
- Navigate to the Setup menu by clicking the gear icon in the top right corner.
- In the Quick Find box, type Sandboxes and select it from the menu.
- Click New Sandbox and follow the prompts to create a new sandbox environment.
Creating a Connected App for OAuth Authentication
Salesforce uses OAuth 2.0 for secure API authentication. You'll need to create a Connected App to obtain the necessary credentials.
- In the Setup menu, enter App Manager in the Quick Find box and select it.
- Click New Connected App and fill in the required fields, such as the app name and contact email.
- Under API (Enable OAuth Settings), check the box for Enable OAuth Settings.
- Enter a Callback URL. This is the URL Salesforce will redirect to after authentication (e.g.,
https://yourapp.com/callback
). - Select the OAuth scopes your app requires, such as Full access or Access and manage your data (api).
- Save the Connected App and note down the Consumer Key and Consumer Secret for use in your API calls.
For more detailed information on setting up OAuth and Connected Apps, refer to the Salesforce OAuth Documentation.
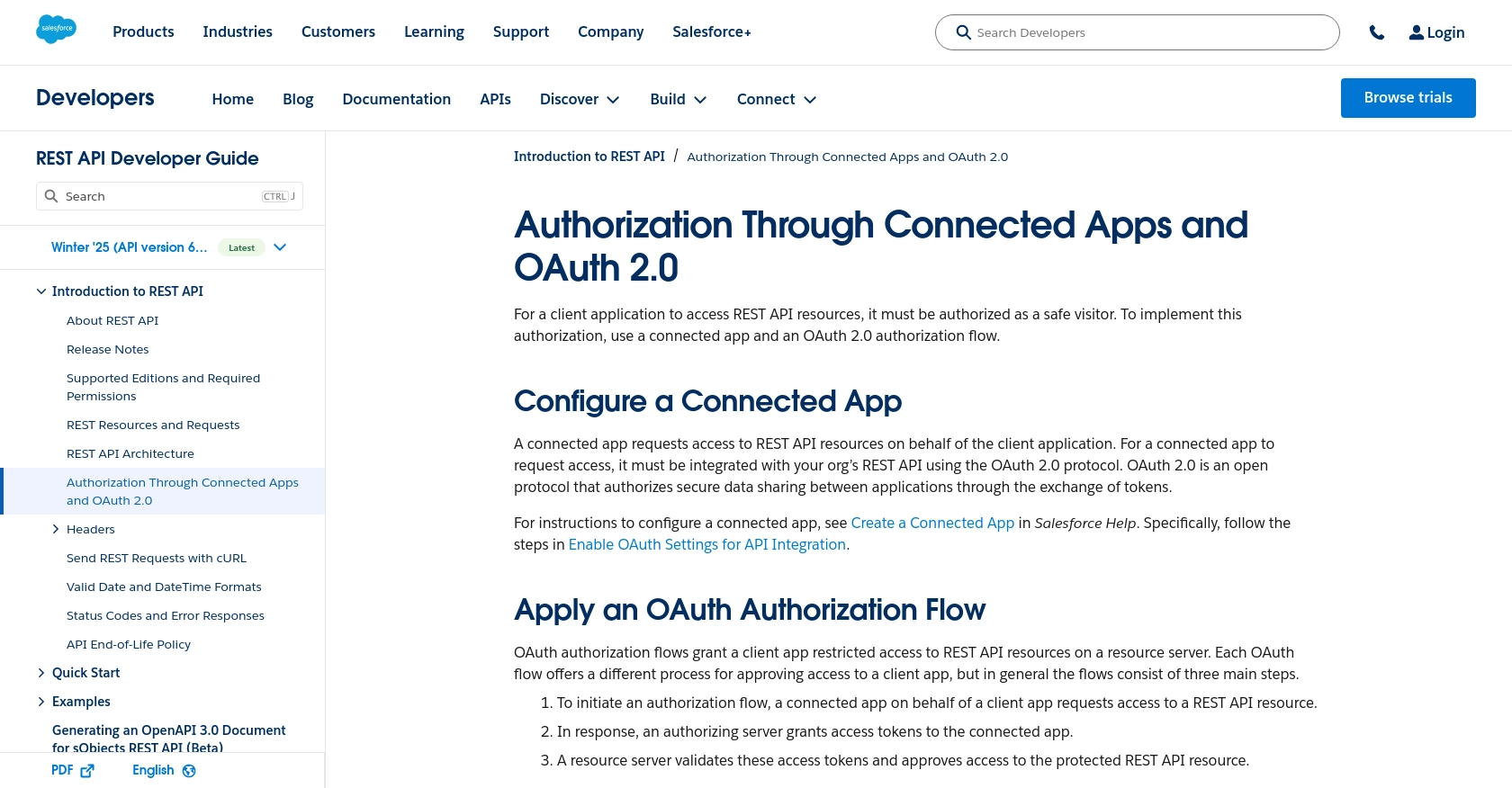
sbb-itb-96038d7
Making API Calls to Salesforce for Creating or Updating Records Using PHP
To interact with Salesforce's API using PHP, you'll need to set up your environment and write code to handle API requests. This section will guide you through the process of making API calls to create or update records in Salesforce.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer, the PHP package manager
You'll also need to install the guzzlehttp/guzzle
library to handle HTTP requests. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Creating or Updating Salesforce Records with PHP
Once your environment is set up, you can proceed to write the PHP code to interact with Salesforce's API. Below is an example of how to create or update a record:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
// Salesforce API endpoint for creating or updating records
$url = 'https://your-instance.salesforce.com/services/data/vXX.X/sobjects/Account/';
// OAuth token obtained from Salesforce
$accessToken = 'YOUR_ACCESS_TOKEN';
// Example data for creating or updating a record
$data = [
'Name' => 'New Account Name',
'Phone' => '123-456-7890'
];
$response = $client->request('POST', $url, [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $data
]);
if ($response->getStatusCode() == 201) {
echo "Record created or updated successfully.";
} else {
echo "Failed to create or update record.";
}
Replace YOUR_ACCESS_TOKEN
with the OAuth token you obtained from Salesforce. The $url
variable should point to the correct Salesforce instance and API version.
Verifying API Call Success in Salesforce Sandbox
After executing the PHP script, verify the success of your API call by checking the Salesforce sandbox environment. Navigate to the relevant object (e.g., Account) to see if the record has been created or updated as expected.
Handling Errors and Error Codes
When making API calls, it's crucial to handle potential errors. Salesforce provides error codes and messages that can help diagnose issues. Common error codes include:
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: The access token is missing or invalid.
- 403 Forbidden: The user does not have permission to perform the action.
- 404 Not Found: The requested resource does not exist.
For more detailed information on error handling, refer to the Salesforce API Documentation.
Conclusion and Best Practices for Salesforce API Integration with PHP
Integrating with the Salesforce API using PHP allows developers to automate and enhance business processes by creating or updating records efficiently. By following the steps outlined in this article, you can leverage Salesforce's powerful capabilities to ensure your applications are robust and responsive to business needs.
Best Practices for Secure and Efficient Salesforce API Integration
- Securely Store Credentials: Always store your OAuth tokens and credentials securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Salesforce imposes rate limits on API requests. Be sure to implement logic to handle
429 Too Many Requests
responses and retry after the specified wait time. For more details, refer to the Salesforce API Documentation. - Standardize Data Fields: Ensure that the data you send to Salesforce is consistent with the expected formats and field types to avoid errors and data inconsistencies.
- Implement Error Handling: Use the error codes provided by Salesforce to diagnose and handle issues effectively. This will improve the reliability and user experience of your application.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a bottleneck, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including Salesforce, allowing you to focus on your core product while outsourcing integration complexities.
By leveraging Endgrate, you can build once for each use case instead of multiple times for different integrations, saving time and resources. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/salesforce
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?