Using the Clickup API to Create Task (with PHP examples)
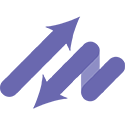
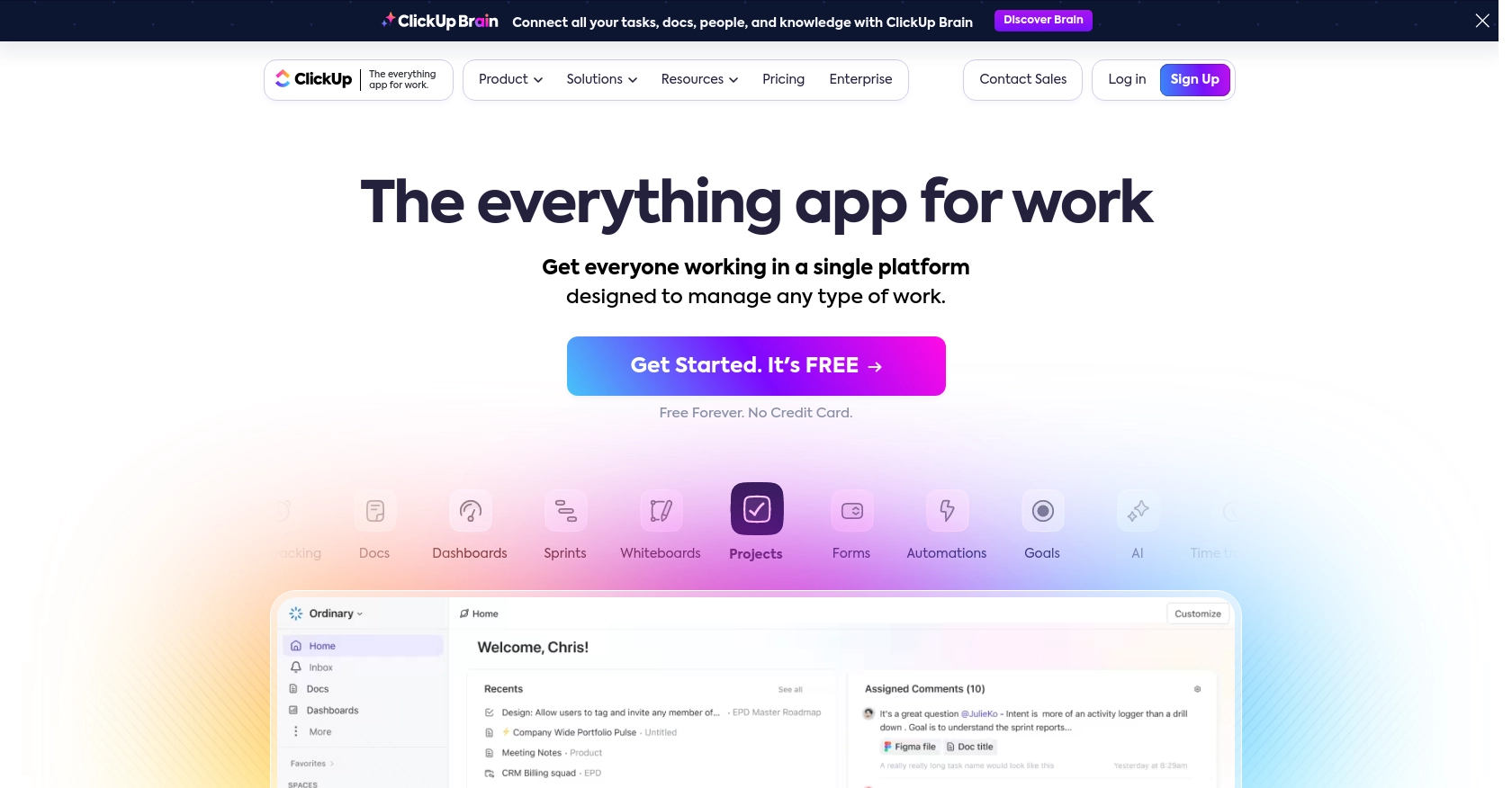
Introduction to ClickUp API Integration
ClickUp is a versatile project management tool that offers a wide range of features to help teams collaborate and manage tasks efficiently. With its customizable interface, ClickUp allows users to tailor their workspace to fit their specific needs, making it a popular choice for businesses of all sizes.
Integrating with ClickUp's API can empower developers to automate task management processes, such as creating tasks programmatically. For example, a developer might want to use the ClickUp API to automatically generate tasks from a form submission on a website, streamlining the workflow and ensuring that no task is overlooked.
Setting Up Your ClickUp Test or Sandbox Account
Before diving into the ClickUp API integration, it's essential to set up a test or sandbox account. This will allow you to experiment with API calls without affecting your live data. ClickUp provides a straightforward process to create a sandbox environment using either a personal API token or OAuth for broader applications.
Creating a ClickUp Account
If you don't already have a ClickUp account, you can sign up for a free account on the ClickUp website. Follow the instructions to complete the registration process. Once your account is created, you'll be able to access the ClickUp dashboard.
Generating a Personal API Token for ClickUp
For personal use or testing, you can generate a personal API token. Here's how:
- Log into your ClickUp account.
- In ClickUp 3.0, click your avatar in the upper-right corner and select Settings.
- Scroll down to the Apps section in the sidebar and click on it.
- Under API Token, click Generate.
- Copy and save your personal API token, which will begin with
pk_
.
This token will be used in the Authorization header of your API requests.
Setting Up OAuth for ClickUp API Integration
If you're developing an application for others to use, you'll need to set up OAuth authentication. Follow these steps:
- Log into your ClickUp account.
- Click on your avatar in the lower-left corner and select Integrations.
- Click on ClickUp API and then Create an App.
- Provide a name for your app and a redirect URL.
- Once created, you'll receive a
client_id
andsecret
.
These credentials will be used to authenticate users and access their ClickUp resources.
For more detailed information on authentication, refer to the ClickUp Authentication Documentation.
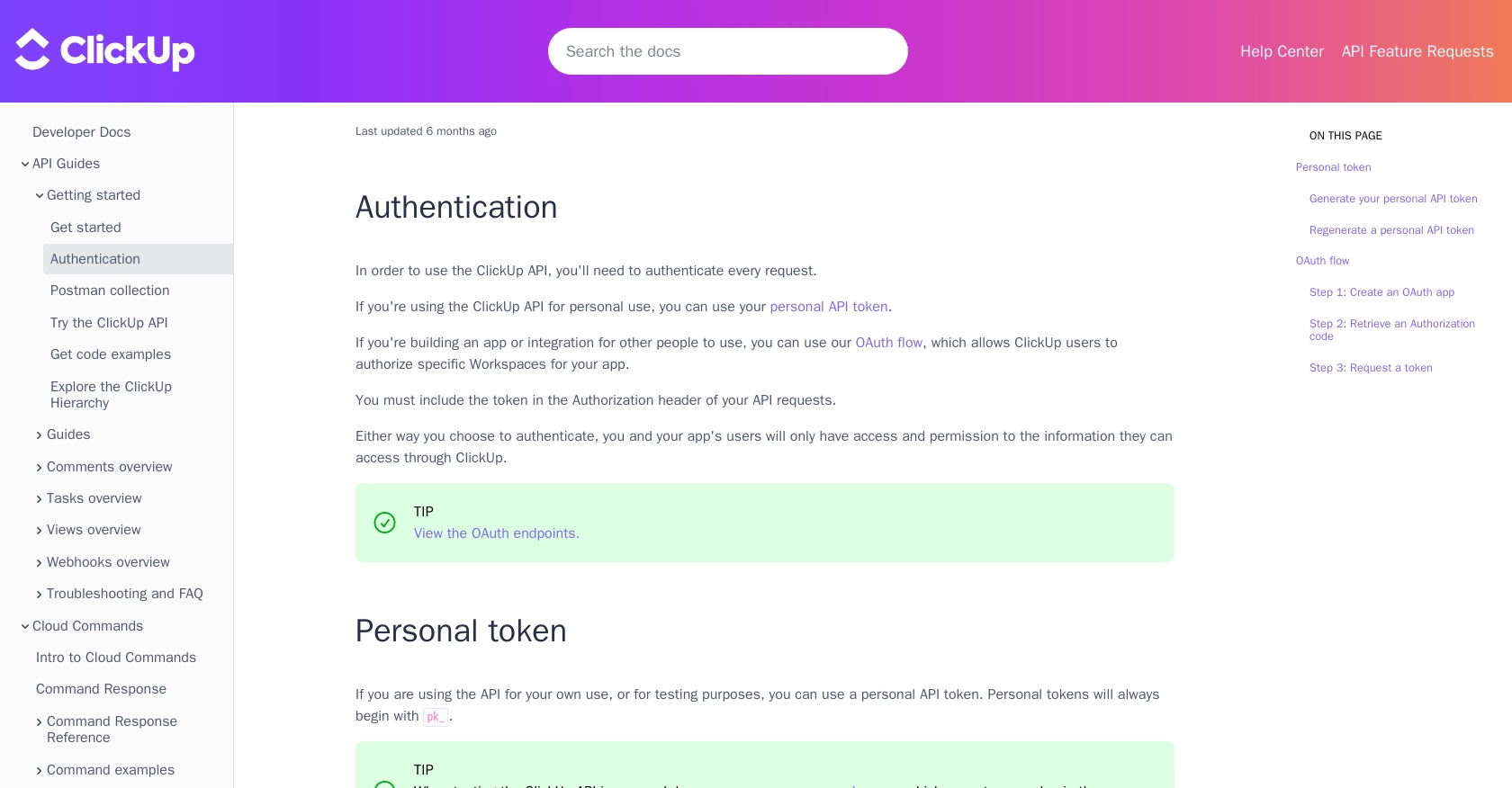
sbb-itb-96038d7
How to Make an API Call to Create a Task in ClickUp Using PHP
To interact with the ClickUp API and create tasks programmatically, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your PHP Environment for ClickUp API Integration
Before you start coding, ensure that your development environment is ready. You'll need PHP installed on your machine, along with the cURL
extension, which is commonly used for making HTTP requests in PHP.
To check if cURL
is installed, run the following command in your terminal:
php -m | grep curl
If cURL
is not listed, you can install it by following the instructions for your specific operating system.
Writing PHP Code to Create a Task in ClickUp
Now that your environment is set up, you can write the PHP code to create a task in ClickUp. The following example demonstrates how to make a POST request to the ClickUp API to create a new task.
<?php
// Set the API endpoint and your personal API token
$endpoint = 'https://api.clickup.com/api/v2/list/{list_id}/task';
$apiToken = 'pk_your_personal_api_token';
// Prepare the task data
$data = [
'name' => 'New Task Name',
'description' => 'New Task Description',
'assignees' => [183], // Replace with actual assignee IDs
'status' => 'Open',
'priority' => 3
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: ' . $apiToken,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display the response
$responseData = json_decode($response, true);
echo 'Task Created Successfully: ' . $responseData['id'];
}
// Close cURL session
curl_close($ch);
?>
Replace {list_id}
with the actual list ID where you want to create the task, and pk_your_personal_api_token
with your personal API token.
Verifying the API Request and Handling Errors
After running the PHP script, you should see a success message with the task ID if the task was created successfully. You can verify the creation by checking your ClickUp workspace.
In case of errors, the script will output the error message. Common issues might include incorrect API tokens, invalid list IDs, or network issues. Ensure that your API token is valid and that you have the necessary permissions to create tasks in the specified list.
Handling ClickUp API Rate Limits and Best Practices
While making API calls, be mindful of ClickUp's rate limits to avoid being throttled. Implement error handling to manage rate limit responses and retry requests if necessary. Always store sensitive information like API tokens securely and avoid hardcoding them in your scripts.
For more details on creating tasks, refer to the ClickUp Create Task Documentation.
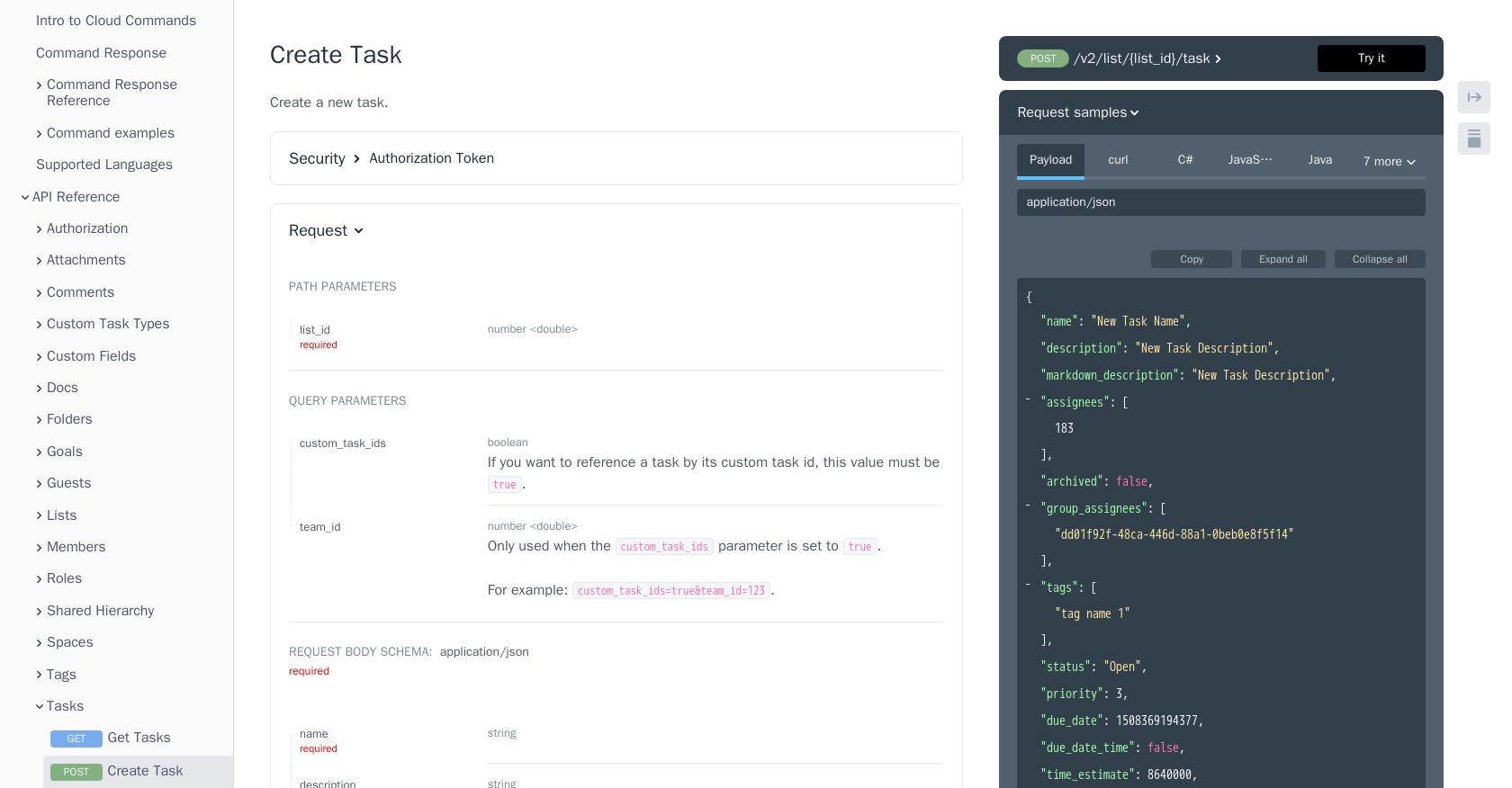
Best Practices for Secure and Efficient ClickUp API Integration
When integrating with the ClickUp API, it's crucial to follow best practices to ensure security and efficiency. Here are some key recommendations:
- Secure Storage of API Tokens: Store API tokens securely using environment variables or secure vaults. Avoid hardcoding them in your scripts to prevent unauthorized access.
- Handle Rate Limits Gracefully: Be aware of ClickUp's rate limits and implement error handling to manage rate limit responses. Consider implementing exponential backoff strategies to retry requests when necessary.
- Data Validation and Error Handling: Validate data before sending it to the API to reduce errors. Implement comprehensive error handling to manage API responses effectively and provide meaningful feedback to users.
- Optimize API Calls: Minimize the number of API calls by batching requests or using webhooks to receive updates in real-time, reducing the load on both your application and ClickUp's servers.
Streamlining Task Management with Endgrate's Integration Solutions
Integrating with ClickUp's API can significantly enhance your task management processes, but managing multiple integrations can be complex and time-consuming. This is where Endgrate can help.
Endgrate provides a unified API endpoint that connects to multiple platforms, including ClickUp, allowing you to build once for each use case instead of multiple times for different integrations. This saves you time and resources, enabling you to focus on your core product.
By leveraging Endgrate's intuitive integration experience, you can offer your customers a seamless and efficient way to manage their tasks across various platforms. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?