Using the Pipedrive API to Get Leads in Python
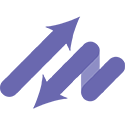
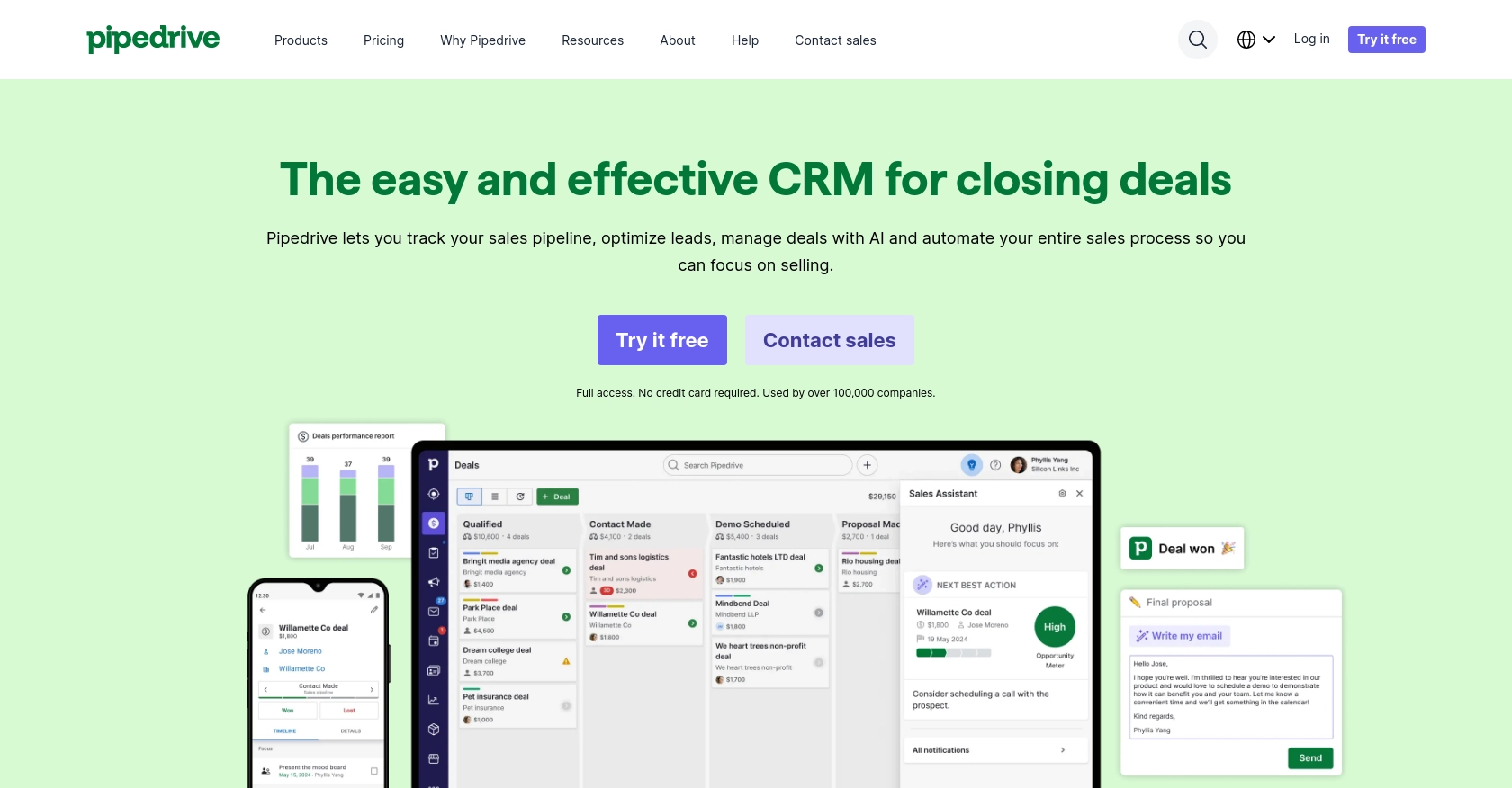
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and optimize their sales pipelines.
Developers often seek to integrate with Pipedrive's API to automate sales processes and enhance data management. For example, using the Pipedrive API, developers can retrieve leads and seamlessly integrate them into other business applications, streamlining workflows and improving sales efficiency.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you'll need to set up a developer sandbox account. This account provides a risk-free environment to test and develop your applications without affecting live data.
Creating a Pipedrive Developer Sandbox Account
To create a sandbox account, follow these steps:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account but is limited to five seats.
- Once your request is approved, you'll receive access to the Developer Hub, where you can manage your apps and perform testing.
Generating OAuth Credentials for Pipedrive API
Pipedrive uses OAuth 2.0 for authentication. To generate the necessary credentials, you'll need to create an app in the Developer Hub:
- Log in to your sandbox account and navigate to the Developer Hub.
- Register your app by providing the required details. This will generate a client ID and client secret.
- Ensure your app uses OAuth 2.0 for authentication and has proper installation flows. This involves adding server-side code to handle the OAuth flow.
With your sandbox account and OAuth credentials set up, you're ready to start making API calls to Pipedrive.
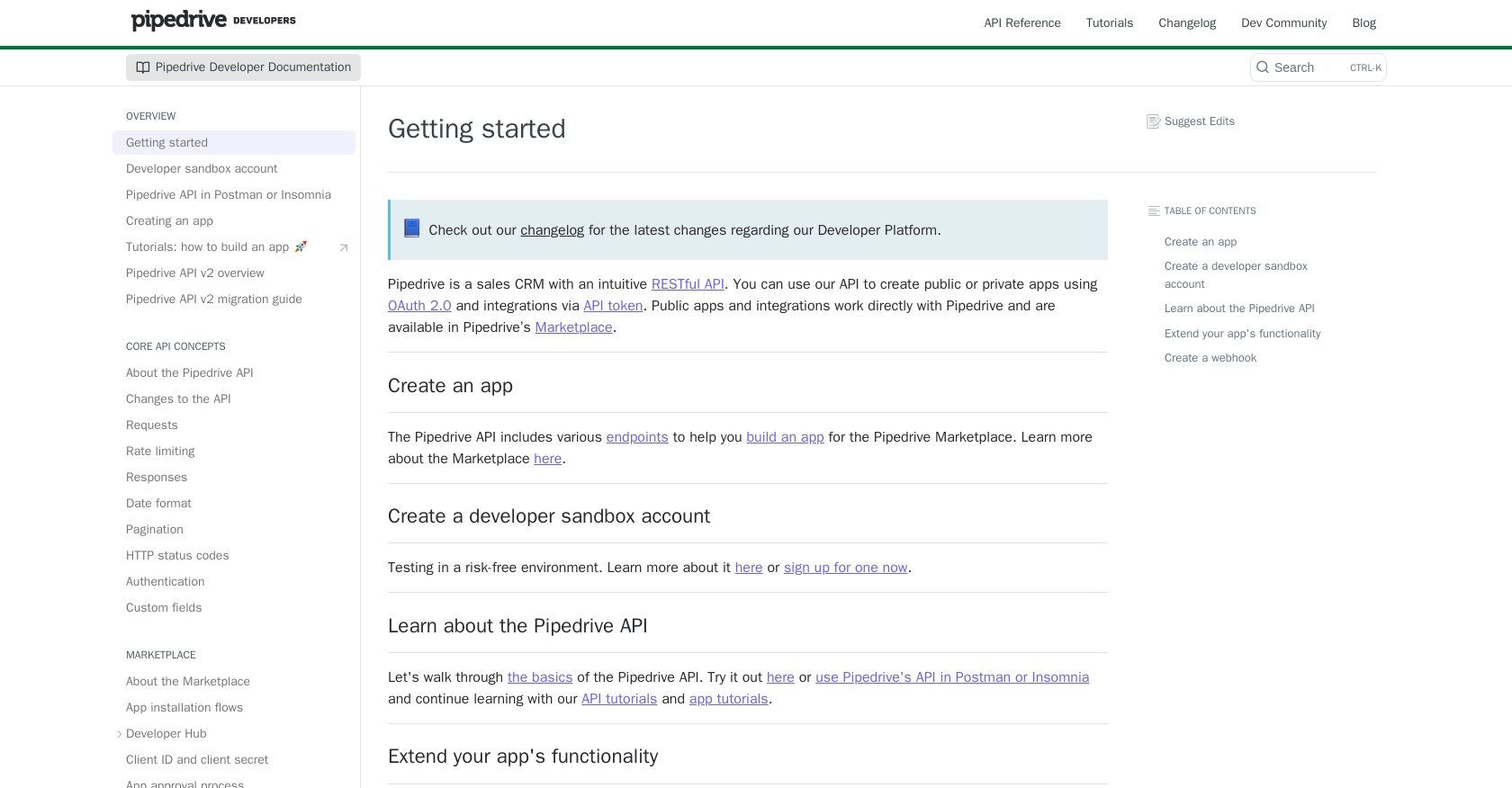
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Pipedrive Using Python
To interact with the Pipedrive API and retrieve leads, you'll need to use Python. This section will guide you through setting up your environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will help you make HTTP requests:
pip install requests
Writing Python Code to Retrieve Leads from Pipedrive
Now that your environment is set up, you can write a Python script to fetch leads from Pipedrive. Create a file named get_pipedrive_leads.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.pipedrive.com/v1/leads"
headers = {
"Authorization": "Bearer Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Parse the JSON data from the response
data = response.json()
# Loop through the leads and print their information
for lead in data.get("data", []):
print(lead)
Replace Your_Access_Token
with the access token obtained during the OAuth setup process.
Running the Python Script and Verifying the Output
Execute the script from your terminal or command line using the following command:
python get_pipedrive_leads.py
You should see the leads data printed in your terminal, confirming that the API call was successful.
Handling Errors and Understanding Pipedrive API Response Codes
When making API calls, it's crucial to handle potential errors. The Pipedrive API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid API token or access token.
- 429 Too Many Requests: Rate limit exceeded. Refer to the rate limiting documentation for more details.
Implement error handling in your script to manage these responses effectively:
if response.status_code == 200:
# Process the data
for lead in data.get("data", []):
print(lead)
else:
print(f"Error: {response.status_code} - {response.json().get('error')}")
By incorporating error handling, you can ensure that your application responds appropriately to different API responses.
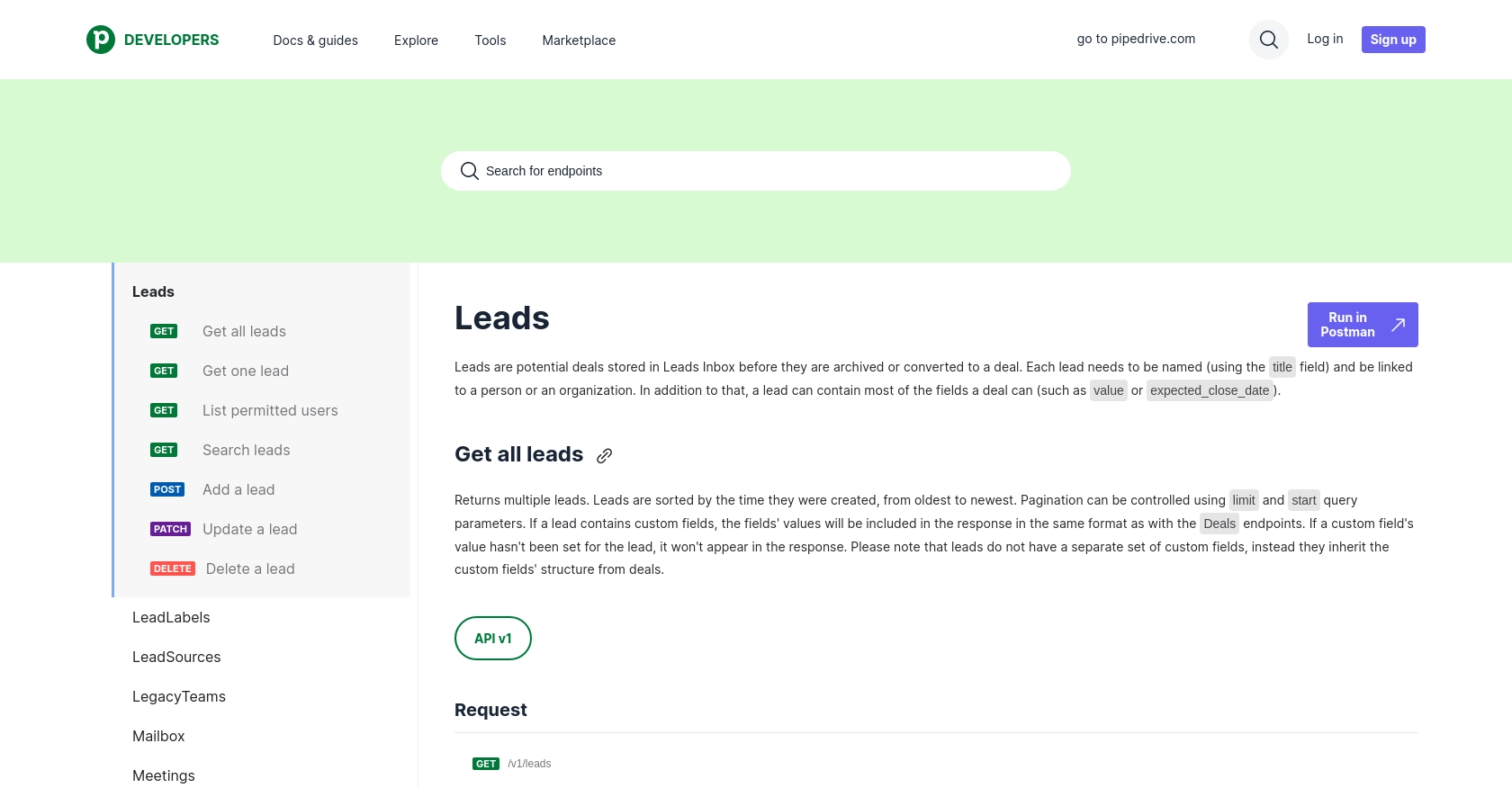
Conclusion and Best Practices for Using Pipedrive API with Python
Integrating with the Pipedrive API using Python can significantly enhance your sales processes by automating lead management and improving data integration across platforms. By following the steps outlined in this guide, you can efficiently retrieve leads and handle API responses effectively.
Best Practices for Storing Pipedrive API Credentials Securely
When working with API credentials, it's crucial to store them securely to prevent unauthorized access. Consider using environment variables or a secure vault to store your client ID
, client secret
, and access tokens. Avoid hardcoding these credentials directly in your scripts.
Managing Pipedrive API Rate Limits
Understanding and managing rate limits is essential to ensure smooth API interactions. Pipedrive's API allows up to 80 requests per 2 seconds per access token for the Essential plan, with higher limits available for advanced plans. Implement logic to handle 429 Too Many Requests
errors by introducing retries or exponential backoff strategies.
Transforming and Standardizing Data from Pipedrive API
When integrating data from Pipedrive into other systems, ensure that you transform and standardize the data fields to match your application's requirements. This might involve mapping Pipedrive's data structures to your internal data models or converting data formats as needed.
Enhancing Integration Efficiency with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with multiple platforms, including Pipedrive. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover how companies like yours have successfully scaled their integrations.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Leads
Ready to get started?