Using the Teamleader API to Create Or Update Contacts in Python
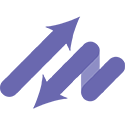
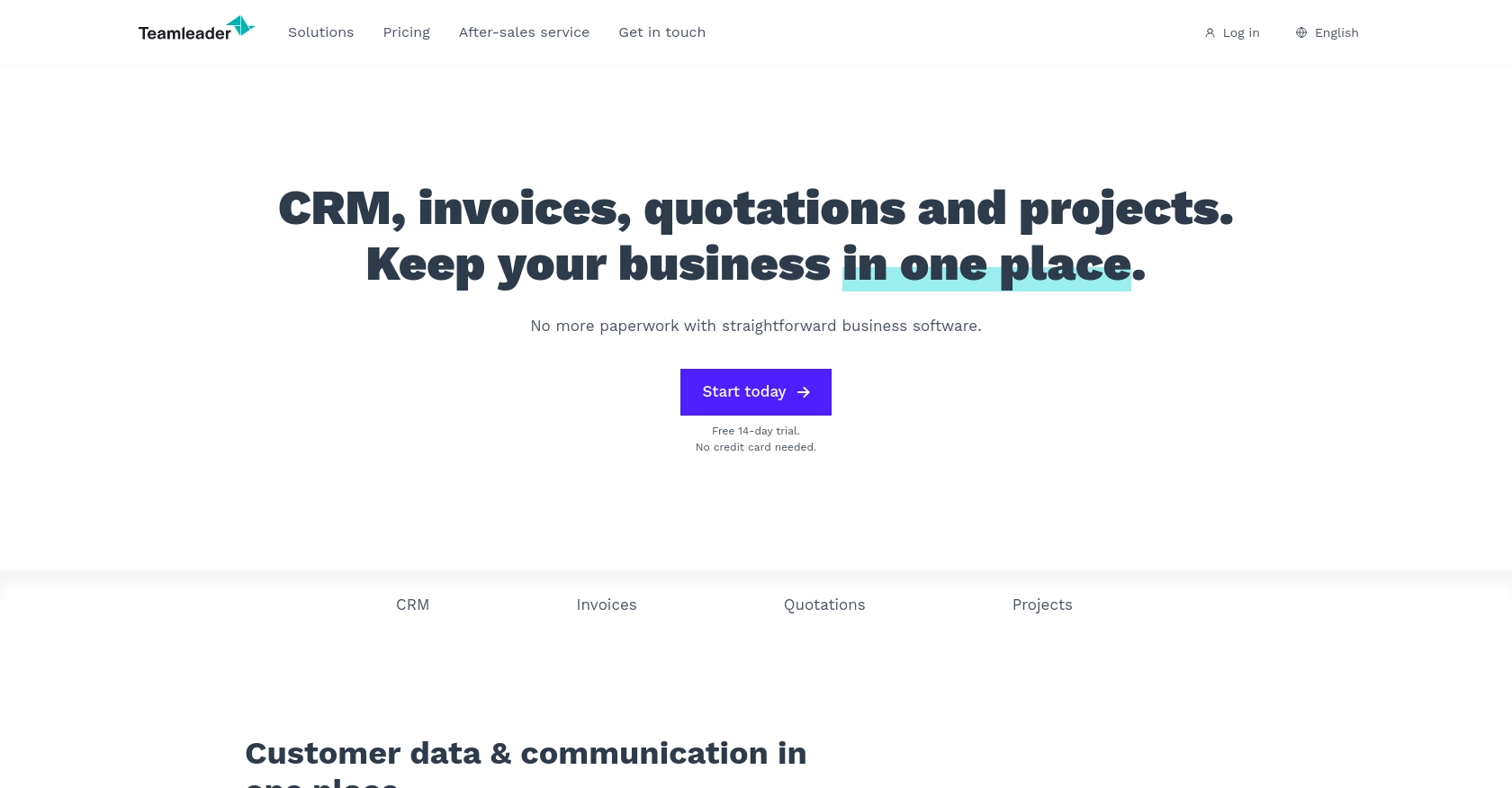
Introduction to Teamleader API Integration
Teamleader is a versatile business management software that helps companies streamline their operations by combining CRM, project management, and invoicing into a single platform. It is designed to enhance productivity and efficiency for businesses of all sizes, offering a comprehensive suite of tools to manage customer relationships, track projects, and handle billing seamlessly.
Integrating with the Teamleader API allows developers to automate and optimize various business processes. For example, by using the Teamleader API, developers can create or update contact information directly from their applications, ensuring that the CRM data remains accurate and up-to-date. This can be particularly useful for businesses looking to synchronize customer data across multiple platforms or automate the onboarding of new clients.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start integrating with the Teamleader API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Teamleader provides a sandbox environment for developers to test their applications and integrations.
Creating a Teamleader Sandbox Account
To begin, you'll need to sign up for a Teamleader account if you haven't already. Visit the Teamleader Developer Portal and follow the instructions to create a new account. Once your account is set up, you can access the sandbox environment.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth for authentication, which requires you to create an application in your sandbox account. Follow these steps to set up OAuth authentication:
- Log in to your Teamleader sandbox account.
- Navigate to the "Integrations" section and select "Create a new app."
- Fill in the required details for your application, such as the app name and description.
- Specify the redirect URI, which is the URL where users will be redirected after they authorize your app.
- Once your app is created, note down the client ID and client secret. These credentials will be used to obtain an access token.
Obtaining an Access Token
With your client ID and client secret, you can now obtain an access token to authenticate API requests. Here's how you can do it:
- Direct users to the Teamleader authorization URL, where they can log in and authorize your app.
- After authorization, users will be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Teamleader token endpoint. Include your client ID, client secret, and authorization code in the request.
import requests
# Set the token endpoint and payload
token_url = "https://app.teamleader.eu/oauth2/access_token"
payload = {
'client_id': 'Your_Client_ID',
'client_secret': 'Your_Client_Secret',
'redirect_uri': 'Your_Redirect_URI',
'grant_type': 'authorization_code',
'code': 'Authorization_Code'
}
# Make the POST request to obtain the access token
response = requests.post(token_url, data=payload)
access_token = response.json().get('access_token')
print("Access Token:", access_token)
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Code
with your actual credentials and authorization code.
With the access token, you can now authenticate your API requests and start interacting with the Teamleader API in your sandbox environment.
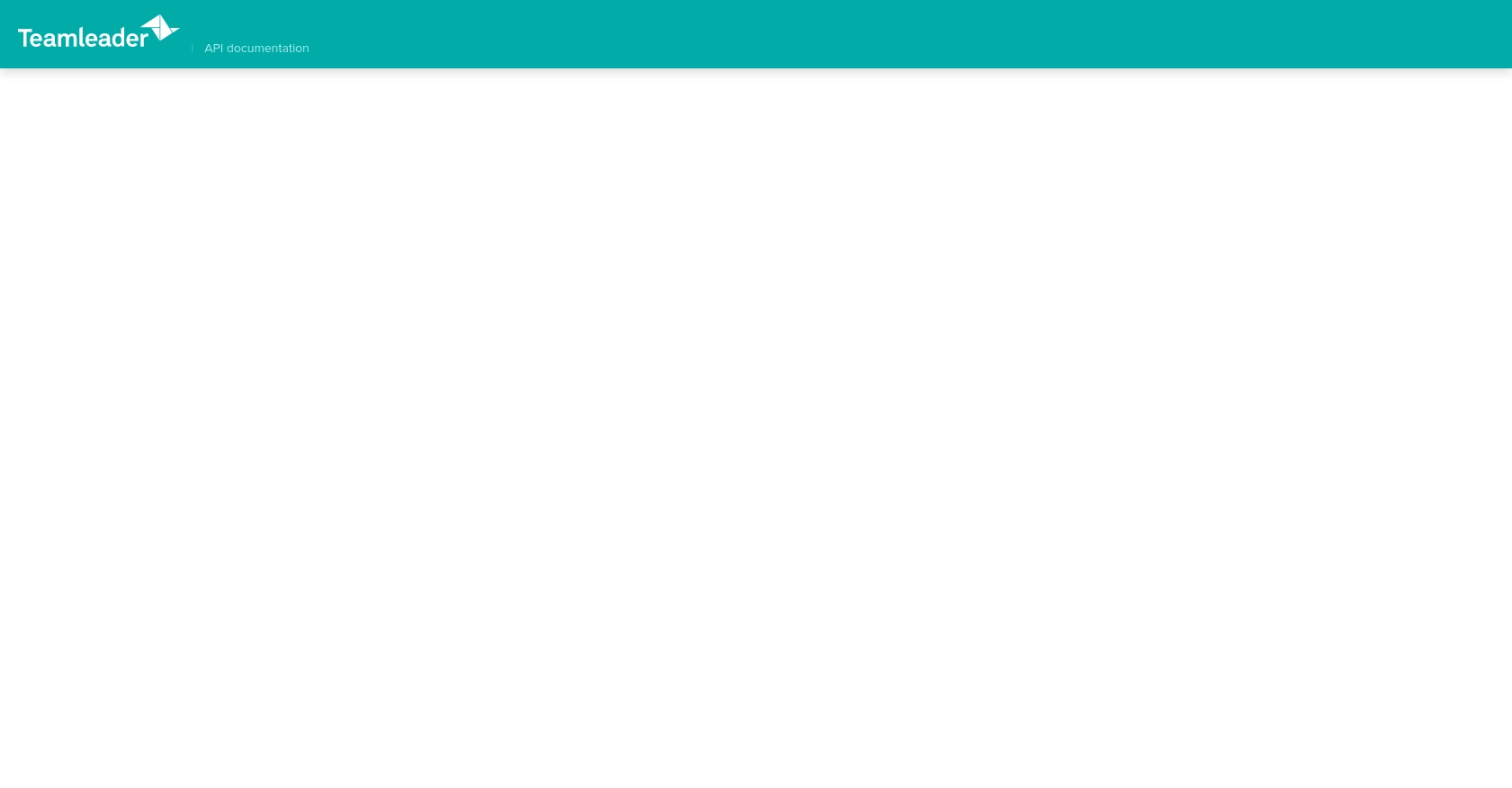
sbb-itb-96038d7
Making API Calls to Teamleader for Contact Management Using Python
With your Teamleader sandbox account set up and OAuth authentication configured, you are ready to make API calls to create or update contacts. This section will guide you through the process of interacting with the Teamleader API using Python, ensuring you can efficiently manage contact data.
Prerequisites for Using Python with Teamleader API
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Additionally, install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating or Updating Contacts with Teamleader API
To create or update contacts in Teamleader, you will need to make a POST request to the appropriate API endpoint. Below is an example of how to achieve this using Python:
import requests
# Set the API endpoint for creating or updating contacts
url = "https://api.teamleader.eu/contacts.createOrUpdate"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the contact data
contact_data = {
"first_name": "John",
"last_name": "Doe",
"emails": [{"type": "primary", "email": "john.doe@example.com"}]
}
# Make the POST request to create or update the contact
response = requests.post(url, json=contact_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Contact created or updated successfully:", response.json())
else:
print("Failed to create or update contact:", response.status_code, response.text)
Replace Your_Access_Token
with the access token obtained during the OAuth authentication process.
Verifying API Call Success in Teamleader Sandbox
After executing the above code, you can verify the success of the API call by checking the contact list in your Teamleader sandbox account. If the contact was successfully created or updated, it should appear in the list with the specified details.
Handling Errors and Error Codes
When making API calls, it is crucial to handle potential errors gracefully. The Teamleader API may return various error codes, such as:
- 400 Bad Request: The request was malformed or contained invalid data.
- 401 Unauthorized: The access token is missing or invalid.
- 404 Not Found: The specified resource could not be found.
- 500 Internal Server Error: An error occurred on the server side.
Ensure your application can handle these errors by implementing appropriate error-handling logic, such as retrying the request or logging the error for further investigation.
Best Practices for Using Teamleader API in Python
When working with the Teamleader API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault service.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Teamleader API. Implement logic to handle rate limit responses, such as exponential backoff, to avoid overwhelming the API.
- Data Standardization: Ensure that the data you send to and receive from the API is standardized. This includes consistent formatting of contact details, such as names and email addresses.
- Error Handling: Implement robust error handling to manage different error codes returned by the API. Log errors for debugging and consider retry mechanisms for transient errors.
Streamlining Integrations with Endgrate
While integrating with the Teamleader API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can simplify your integration process.
Endgrate provides a unified API endpoint that connects to various platforms, including Teamleader. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integrations to Endgrate, reducing development time and costs.
- Build Once, Use Everywhere: Develop a single integration for each use case and leverage it across multiple platforms, eliminating redundant work.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with minimal effort.
To learn more about how Endgrate can help you streamline your integrations, visit Endgrate's website and explore the possibilities.
Read More
Ready to get started?