Using the Pipedrive API to Create or Update Deals in Python
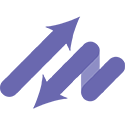
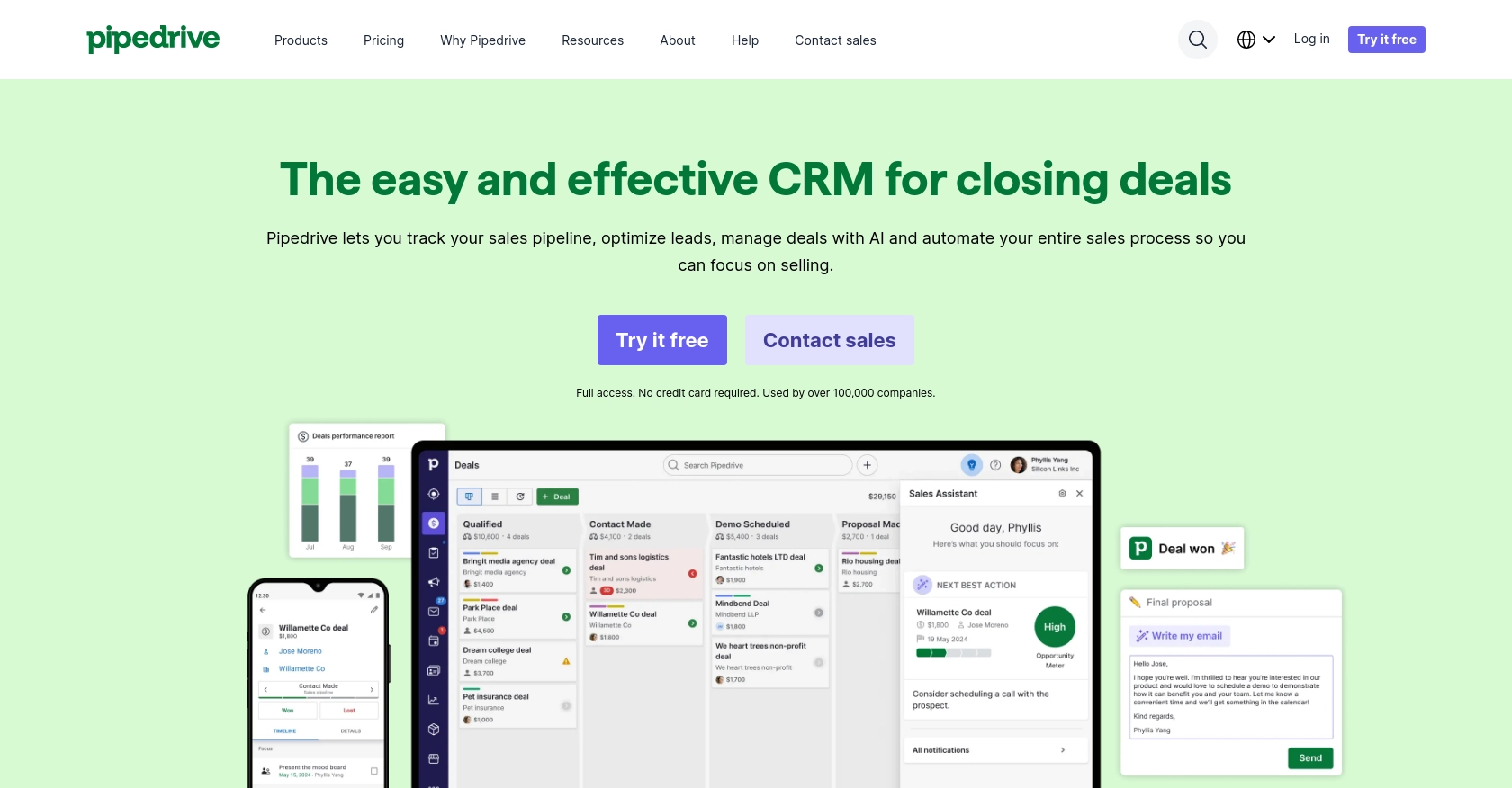
Introduction to Pipedrive CRM
Pipedrive is a robust sales CRM platform designed to help businesses manage their sales processes efficiently. Known for its intuitive interface and powerful features, Pipedrive enables sales teams to track deals, manage contacts, and automate workflows, making it a popular choice for organizations aiming to enhance their sales performance.
Integrating with Pipedrive's API allows developers to automate and streamline sales operations, such as creating or updating deals programmatically. For example, a developer might use the Pipedrive API to automatically update deal statuses based on customer interactions, ensuring that sales teams have up-to-date information at their fingertips.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with Pipedrive's API, you'll need to set up a developer sandbox account. This account provides a risk-free environment where you can test and develop your applications without affecting live data.
Steps to Create a Pipedrive Developer Sandbox Account
- Sign Up for a Sandbox Account: Visit the Pipedrive Developer Sandbox page and fill out the form to request access to a sandbox account. This account mimics a regular Pipedrive company account and is limited to 5 seats.
- Import Sample Data: To familiarize yourself with Pipedrive's interface, you can import sample data. Go to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app. You can use template spreadsheets available in English or Portuguese.
Creating a Pipedrive App for OAuth Authentication
Since Pipedrive uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials.
-
Register Your App: Navigate to the Developer Hub within your sandbox account. Register your app to obtain the
client_id
andclient_secret
needed for OAuth authentication. - Set Up OAuth Scopes: Ensure your app requests only the necessary scopes for your integration. This minimizes security risks and simplifies the user consent process.
Once your sandbox account and app are set up, you'll be ready to start making API calls to create or update deals in Pipedrive using Python.
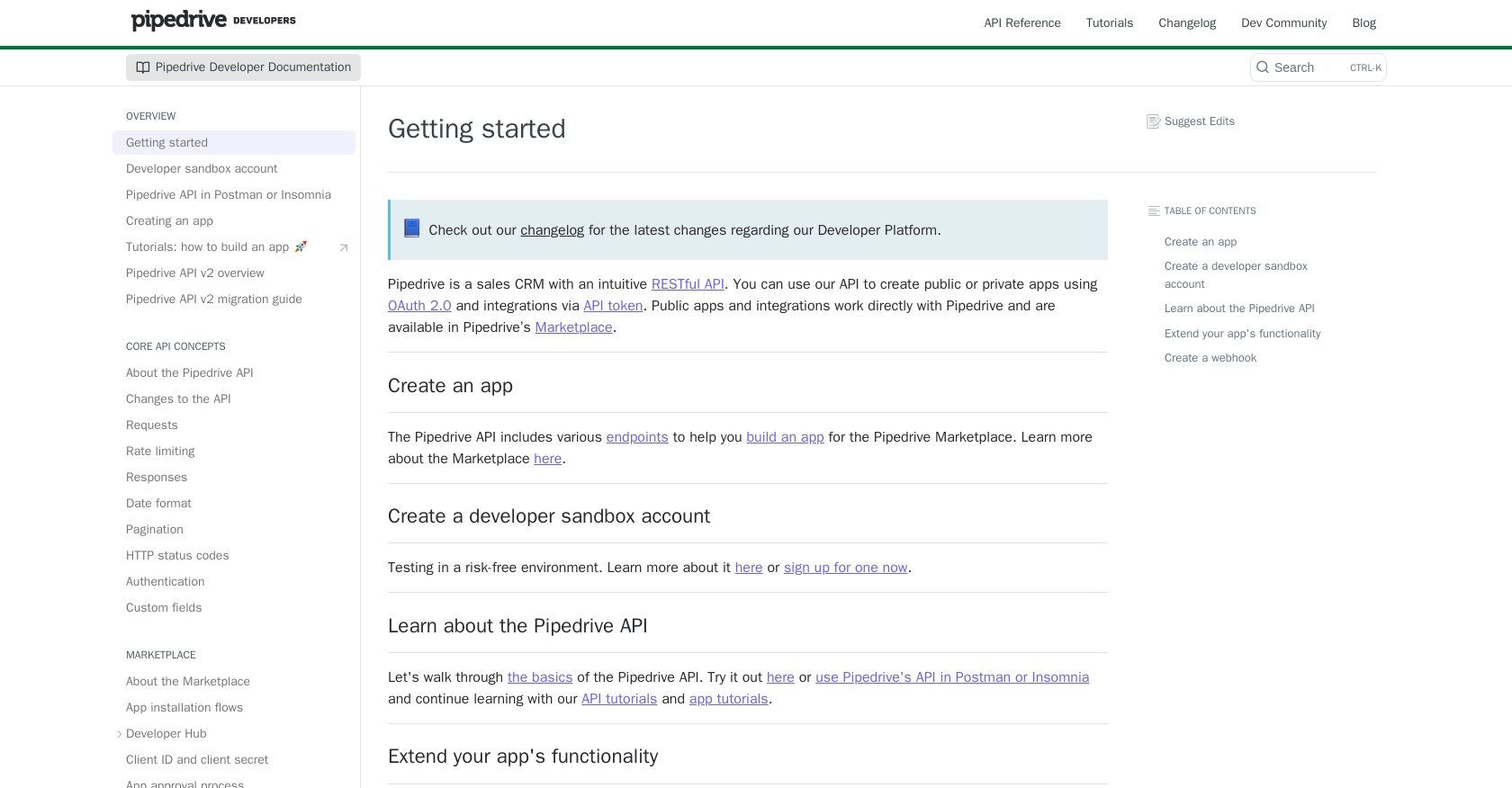
sbb-itb-96038d7
Making API Calls to Create or Update Deals in Pipedrive Using Python
To interact with Pipedrive's API for creating or updating deals, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses.
Setting Up Your Python Environment for Pipedrive API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating a New Deal in Pipedrive Using Python
To create a new deal in Pipedrive, you'll need to make a POST request to the Pipedrive API. Here's a step-by-step guide:
- Create a new Python file named
create_pipedrive_deal.py
and add the following code:
import requests
# Set the API endpoint
url = "https://api.pipedrive.com/v1/deals"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Set the deal information
deal_data = {
"title": "New Deal",
"value": 1000,
"currency": "USD"
}
# Send the request
response = requests.post(url, json=deal_data, headers=headers)
if response.status_code == 201:
print("Deal Created Successfully")
else:
print("Failed to Create Deal:", response.json())
Replace Your_Access_Token
with the access token obtained from your OAuth app setup.
Run the script using the command:
python create_pipedrive_deal.py
If successful, you'll see "Deal Created Successfully" in the output. Verify the creation by checking your Pipedrive sandbox account.
Updating an Existing Deal in Pipedrive Using Python
To update an existing deal, you'll need to make a PATCH request. Follow these steps:
- Create a new Python file named
update_pipedrive_deal.py
and add the following code:
import requests
# Set the API endpoint with the specific deal ID
deal_id = 12345
url = f"https://api.pipedrive.com/v1/deals/{deal_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Set the updated deal information
update_data = {
"title": "Updated Deal Title",
"value": 1500
}
# Send the request
response = requests.patch(url, json=update_data, headers=headers)
if response.status_code == 200:
print("Deal Updated Successfully")
else:
print("Failed to Update Deal:", response.json())
Replace Your_Access_Token
with your access token and deal_id
with the ID of the deal you wish to update.
Run the script using the command:
python update_pipedrive_deal.py
Upon success, "Deal Updated Successfully" will be displayed. Check your Pipedrive sandbox account to confirm the update.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. The Pipedrive API may return various HTTP status codes, such as:
- 200 OK: Request fulfilled successfully.
- 201 Created: New resource created.
- 400 Bad Request: Request not understood.
- 401 Unauthorized: Invalid API token.
- 429 Too Many Requests: Rate limit exceeded.
For more details on error codes, refer to the Pipedrive documentation.
To avoid exceeding rate limits, consider implementing retries with exponential backoff or upgrading your plan. For more information, see the rate limiting documentation.
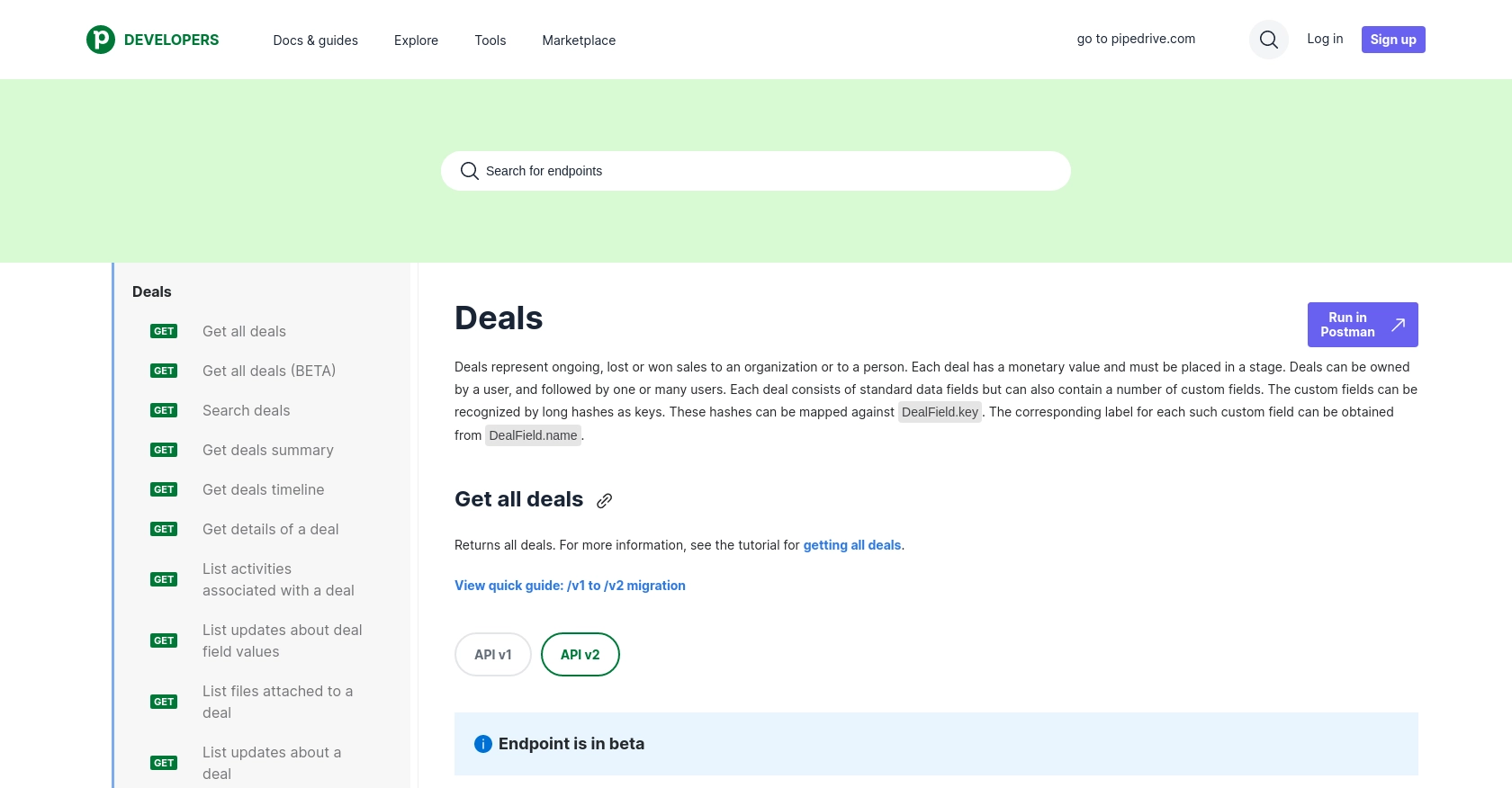
Best Practices for Pipedrive API Integration
When integrating with the Pipedrive API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Pipedrive's API has rate limits based on your plan. Implement retries with exponential backoff to handle HTTP 429 errors gracefully. For detailed rate limit information, refer to the rate limiting documentation.
- Data Standardization: Ensure that data fields are standardized across your application to maintain consistency and avoid errors when interacting with the API.
Leveraging Endgrate for Seamless Pipedrive Integrations
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Pipedrive.
With Endgrate, you can:
- Focus on Core Product Development: Outsource integration management to save time and resources, allowing your team to concentrate on core product features.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms, reducing redundancy and effort.
- Enhance Customer Experience: Offer an intuitive and seamless integration experience for your users, improving satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Deals
- https://developers.pipedrive.com/docs/api/v1/DealFields
Ready to get started?