Using the Nimble API to Get Companies in Javascript
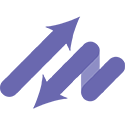
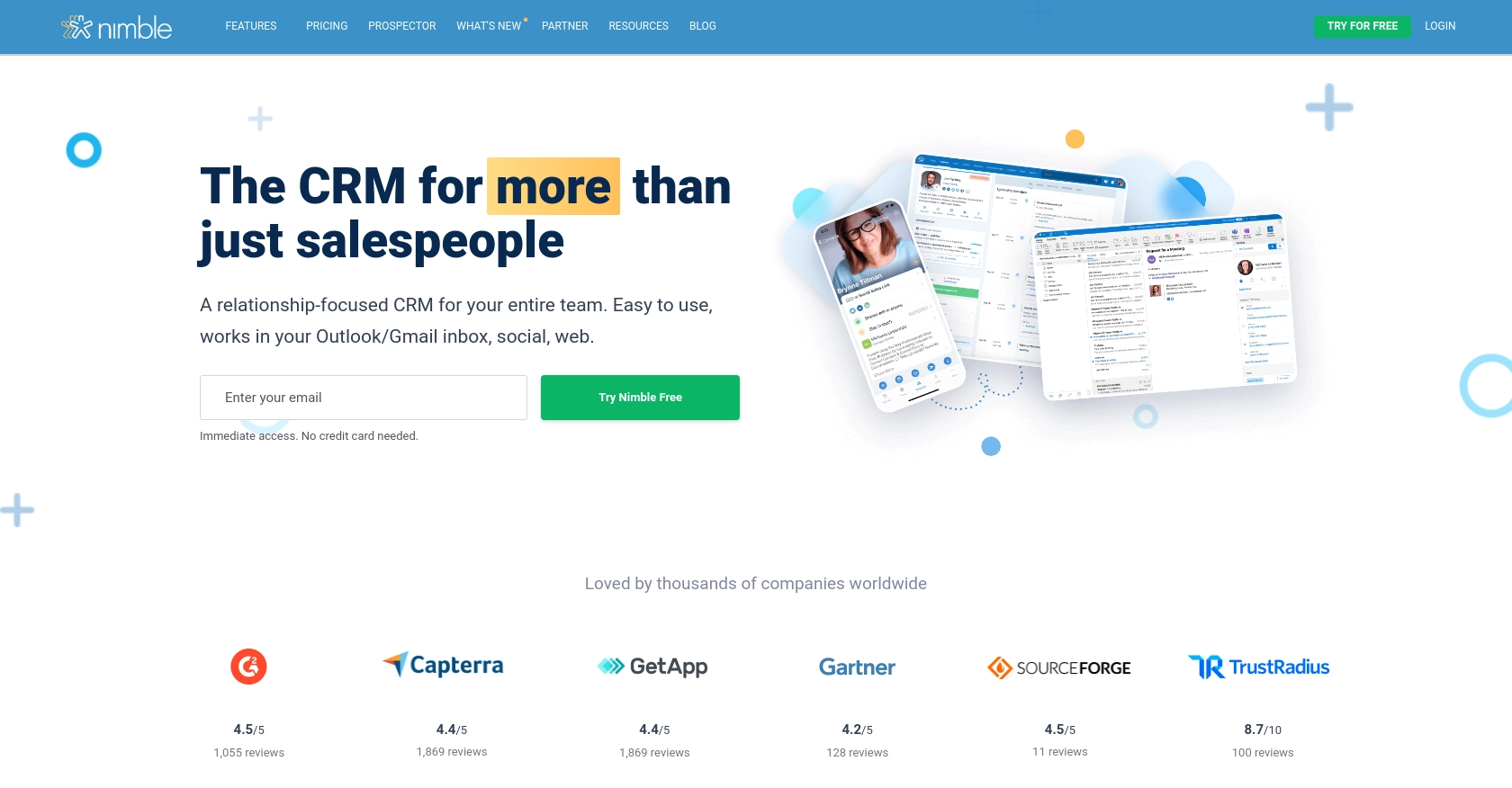
Introduction to Nimble CRM
Nimble is a relationship-focused CRM platform that integrates seamlessly with both Microsoft 365 and Google Workspace. It offers customizable contact records and intuitive workflows, making it easier for businesses to manage client information and nurture relationships.
Developers may want to integrate with Nimble's API to access and manage company data efficiently. For example, you can use the Nimble API to retrieve a list of companies and synchronize this data with other business applications, enhancing your organization's data management capabilities.
Setting Up Your Nimble Test Account for API Access
Before you can start using the Nimble API to retrieve company data, you'll need to set up a test account. This process involves generating an API key, which will allow you to authenticate your requests to the Nimble API.
Creating a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial on the Nimble website. This will give you access to the platform's features and allow you to explore its capabilities.
Generating an API Key in Nimble
Once your account is set up, follow these steps to generate an API key:
- Log in to your Nimble account.
- Navigate to the Settings menu.
- Select API Token from the options available.
- Click on Generate New Token.
- Provide a description for your token to help identify its purpose.
- Click Generate to create your API key.
After generating the API key, make sure to copy and store it securely. You will need this key to authenticate your API requests.
Granting API Access in Nimble
Ensure that API access is granted by the admin of your Nimble account. Admins can do this by navigating to the Settings and then to the Users page, where they can manage permissions.
For more detailed instructions, you can refer to the Nimble API documentation: Generate an API Key to Access the Nimble API.
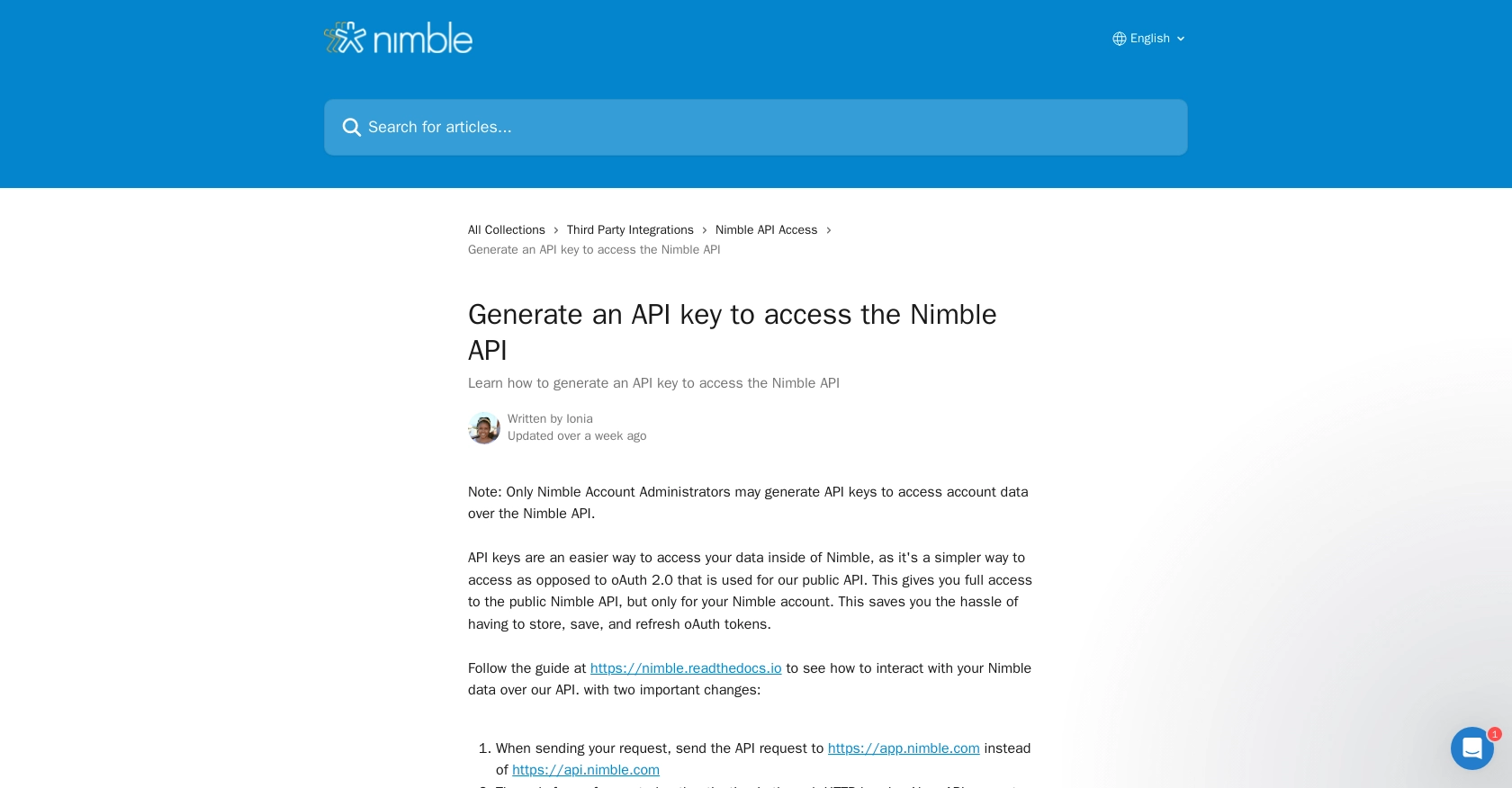
sbb-itb-96038d7
Making API Calls to Retrieve Companies Using Nimble API in JavaScript
To interact with the Nimble API and retrieve company data using JavaScript, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process, including setting up JavaScript, installing dependencies, and writing the code to fetch company data.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can create a new project directory and initialize it:
mkdir nimble-api-integration
cd nimble-api-integration
npm init -y
Installing Required Dependencies
For making HTTP requests in JavaScript, you can use the popular axios
library. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Companies from Nimble API
Create a new file named getCompanies.js
and add the following code to it:
const axios = require('axios');
// Replace 'YOUR_API_KEY' with your actual Nimble API key
const apiKey = 'YOUR_API_KEY';
// Set the API endpoint for retrieving companies
const endpoint = 'https://app.nimble.com/api/v1/companies';
// Configure the request headers
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
};
// Function to fetch companies
async function fetchCompanies() {
try {
const response = await axios.get(endpoint, { headers });
const companies = response.data.resources;
console.log('Companies:', companies);
} catch (error) {
console.error('Error fetching companies:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchCompanies();
In this code, you import the axios
library and set up the API endpoint and headers. The fetchCompanies
function makes a GET request to the Nimble API to retrieve a list of companies. If successful, it logs the companies to the console. If an error occurs, it logs the error message.
Running Your JavaScript Code
To execute your code and fetch companies from the Nimble API, run the following command in your terminal:
node getCompanies.js
You should see a list of companies printed in your terminal. If there are any errors, ensure your API key is correct and that you have the necessary permissions set in your Nimble account.
Handling Errors and Verifying API Requests
When making API requests, it's crucial to handle potential errors gracefully. The Nimble API may return various HTTP status codes indicating different errors:
- 401 Unauthorized: Check if your API key is correct.
- 403 Forbidden: Ensure your account has the necessary permissions.
- 500 Internal Server Error: Retry the request or contact Nimble support.
For more information on error codes, refer to the Nimble API documentation.
Conclusion and Best Practices for Using Nimble API in JavaScript
Integrating with the Nimble API using JavaScript can significantly enhance your ability to manage and synchronize company data across various platforms. By following the steps outlined in this guide, you can efficiently retrieve company information and incorporate it into your business applications.
Best Practices for Secure and Efficient Nimble API Integration
- Secure API Key Storage: Always store your API keys securely and avoid hardcoding them in your source files. Consider using environment variables or a secure vault service.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to manage rate limits effectively.
- Data Transformation and Standardization: Ensure that the data retrieved from the Nimble API is transformed and standardized to fit your application's data model, enhancing data consistency and usability.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging purposes.
By adhering to these best practices, you can create a reliable and secure integration with Nimble, ensuring that your applications can leverage the full potential of Nimble's CRM capabilities.
Streamline Your Integration Process with Endgrate
If you're looking to simplify your integration process and focus more on your core product, consider using Endgrate. Endgrate allows you to build once for each use case and manage multiple integrations effortlessly. With Endgrate, you can provide an intuitive integration experience for your customers, saving time and resources.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?