How to Get Tax Rates with the Quickbooks API in Python
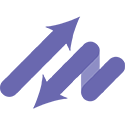
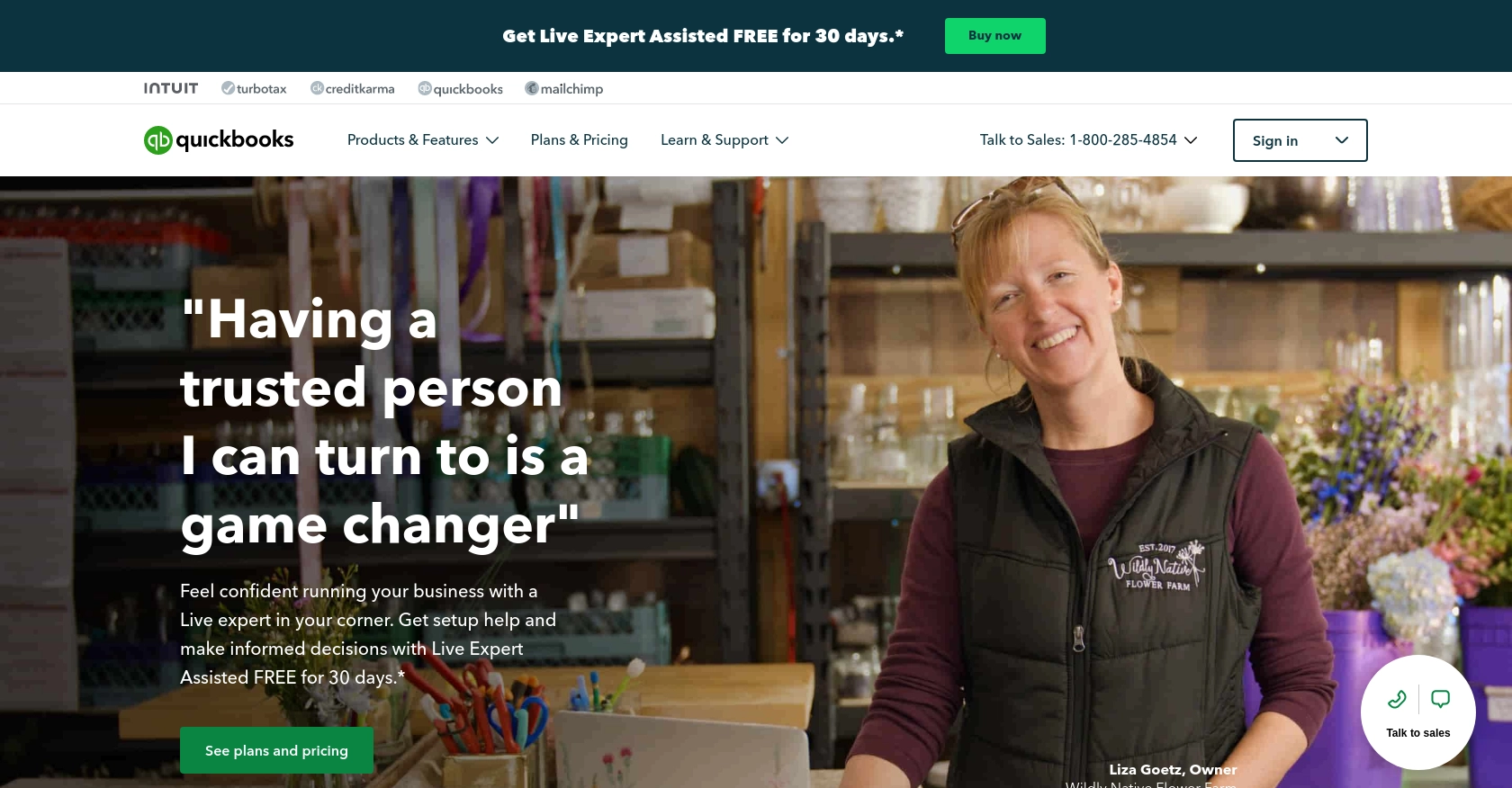
Introduction to QuickBooks API for Tax Rates
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a range of features, including invoicing, expense tracking, payroll, and tax management, making it a popular choice for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate financial processes, such as retrieving tax rates, which can be crucial for accurate invoicing and compliance. For example, a developer might use the QuickBooks API to fetch current tax rates and apply them to transactions within a custom application, ensuring that tax calculations are always up-to-date and accurate.
Setting Up Your QuickBooks Test/Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API to retrieve tax rates, you need to set up a test or sandbox account. This environment allows you to safely develop and test your integration without affecting live data.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Sign up for a free developer account or log in if you already have one.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can create a sandbox company:
- Navigate to the "Dashboard" on the QuickBooks Developer Portal.
- Select "Sandbox" from the menu and click "Add Sandbox Company."
- Follow the prompts to set up your sandbox company, which will mimic a real QuickBooks environment.
Creating a QuickBooks App for OAuth Authentication
To interact with the QuickBooks API, you need to create an app to obtain OAuth credentials:
- Go to the "My Apps" section in your QuickBooks Developer account.
- Click "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required details, such as app name and description.
Obtaining Client ID and Client Secret
After creating your app, you'll need the client ID and client secret for authentication:
- Navigate to the "Keys & OAuth" section of your app settings.
- Copy the client ID and client secret. These will be used to authenticate API requests.
For more detailed instructions, refer to the QuickBooks OAuth Documentation.
Configuring OAuth 2.0 for API Access
QuickBooks uses OAuth 2.0 for secure API access. Follow these steps to configure it:
- In your app settings, go to "Redirect URIs" and add the URL where users will be redirected after authentication.
- Ensure you have the necessary scopes selected for accessing tax rates.
With your sandbox account and app set up, you're ready to start making API calls to retrieve tax rates using Python.
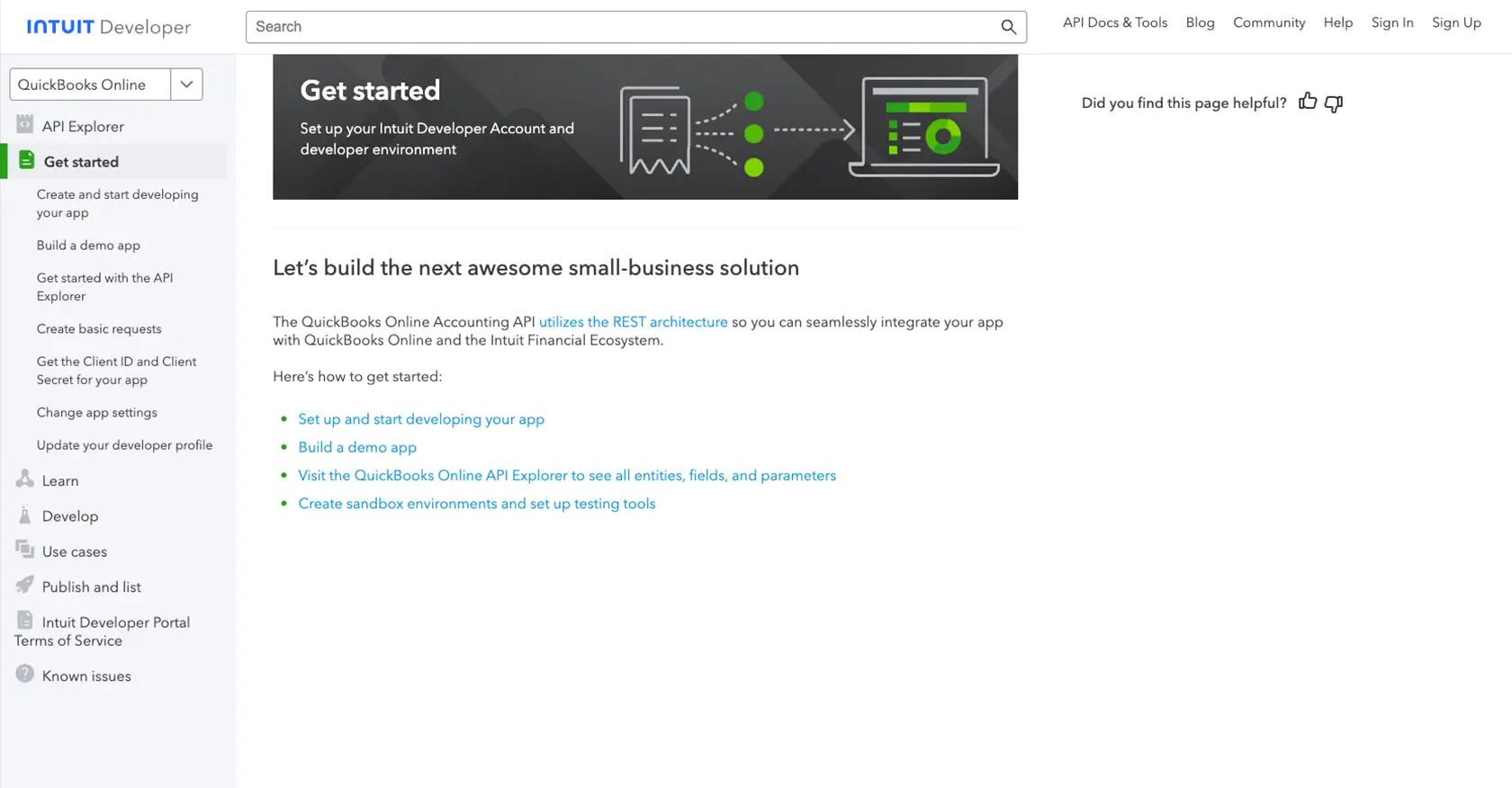
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates with QuickBooks API in Python
To interact with the QuickBooks API and retrieve tax rates, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your Python Environment for QuickBooks API Integration
Before you begin, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests to the QuickBooks API:
pip install requests
Writing Python Code to Fetch Tax Rates from QuickBooks API
Create a new Python file named get_quickbooks_tax_rates.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/query?query=SELECT * FROM TaxRate"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the tax rates and print their information
for tax_rate in data.get("QueryResponse", {}).get("TaxRate", []):
print(tax_rate)
else:
print(f"Failed to retrieve tax rates: {response.status_code} - {response.text}")
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token.
Running the Python Script and Verifying the Output
Run the script from your terminal or command line using the following command:
python get_quickbooks_tax_rates.py
If successful, you should see a list of tax rates printed to the console. Verify these rates against your QuickBooks sandbox account to ensure accuracy.
Handling Errors and Understanding QuickBooks API Response Codes
It's essential to handle potential errors when making API calls. The QuickBooks API may return various HTTP status codes, such as:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server side.
Refer to the QuickBooks API Documentation for more detailed information on error codes and handling strategies.
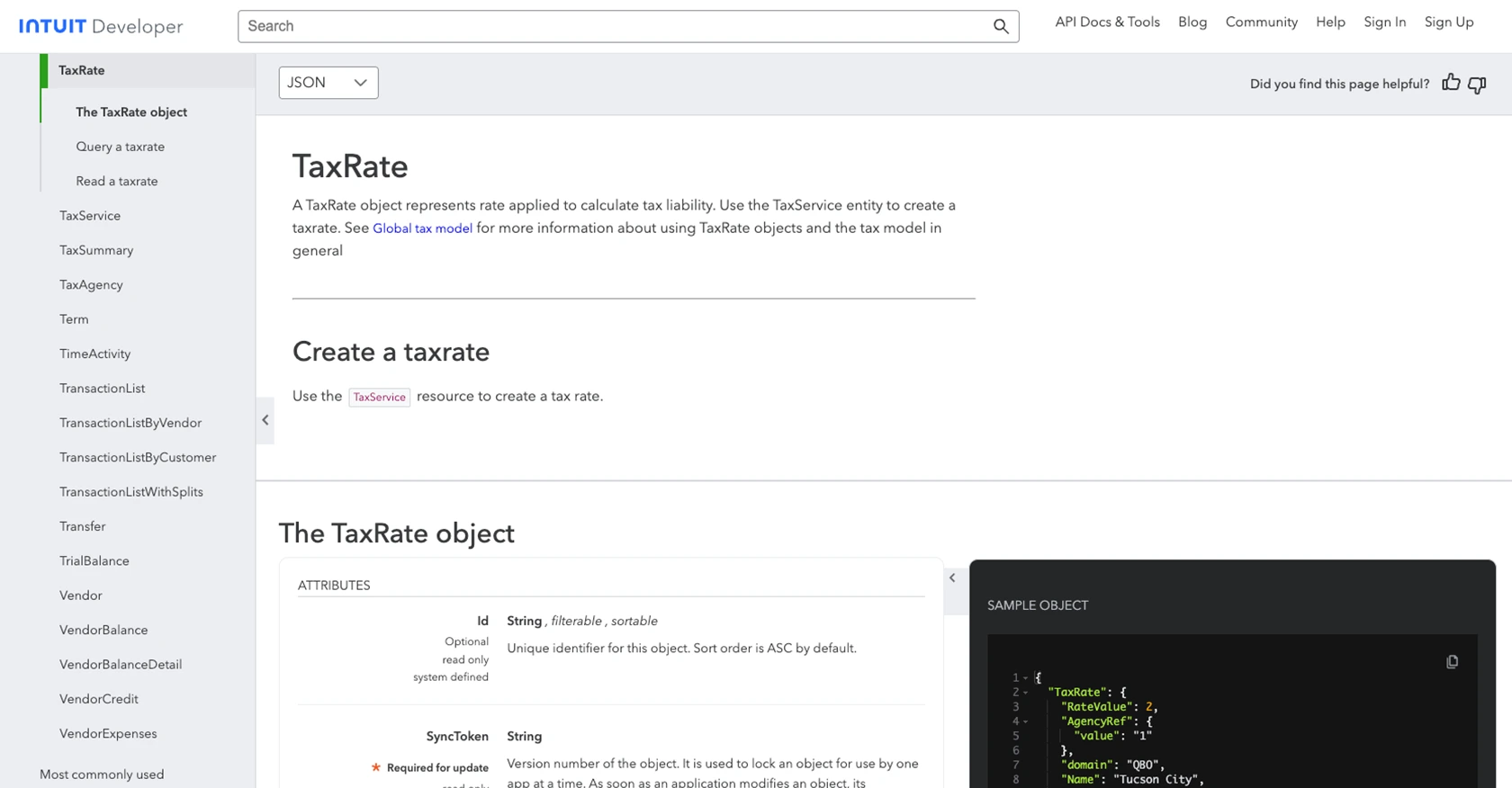
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to retrieve tax rates can significantly streamline financial processes and ensure compliance with current tax regulations. By automating these tasks, developers can enhance the accuracy and efficiency of their applications, providing real-time tax data for invoicing and financial reporting.
Best Practices for Storing QuickBooks API Credentials
- Secure Storage: Store your client ID, client secret, and access tokens securely, using environment variables or a secure vault service.
- Regular Rotation: Regularly rotate your access tokens to minimize security risks.
Handling QuickBooks API Rate Limits
QuickBooks API imposes rate limits to ensure fair use. To handle these limits effectively:
- Monitor Usage: Keep track of your API usage to avoid hitting rate limits.
- Implement Backoff Strategies: Use exponential backoff strategies to retry requests when rate limits are exceeded.
Data Transformation and Standardization with QuickBooks API
When working with tax rates and other financial data, it's crucial to standardize and transform data fields to fit your application's requirements. Consider using data transformation libraries or custom scripts to ensure consistency across your application.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including QuickBooks, allowing you to focus on your core product while outsourcing integration complexities.
By leveraging Endgrate, you can build once for each use case and enjoy an intuitive integration experience, saving time and resources. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/taxrate
Ready to get started?