Using the Moneybird API to Get Recurring Invoices (with Javascript examples)
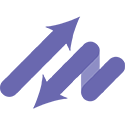
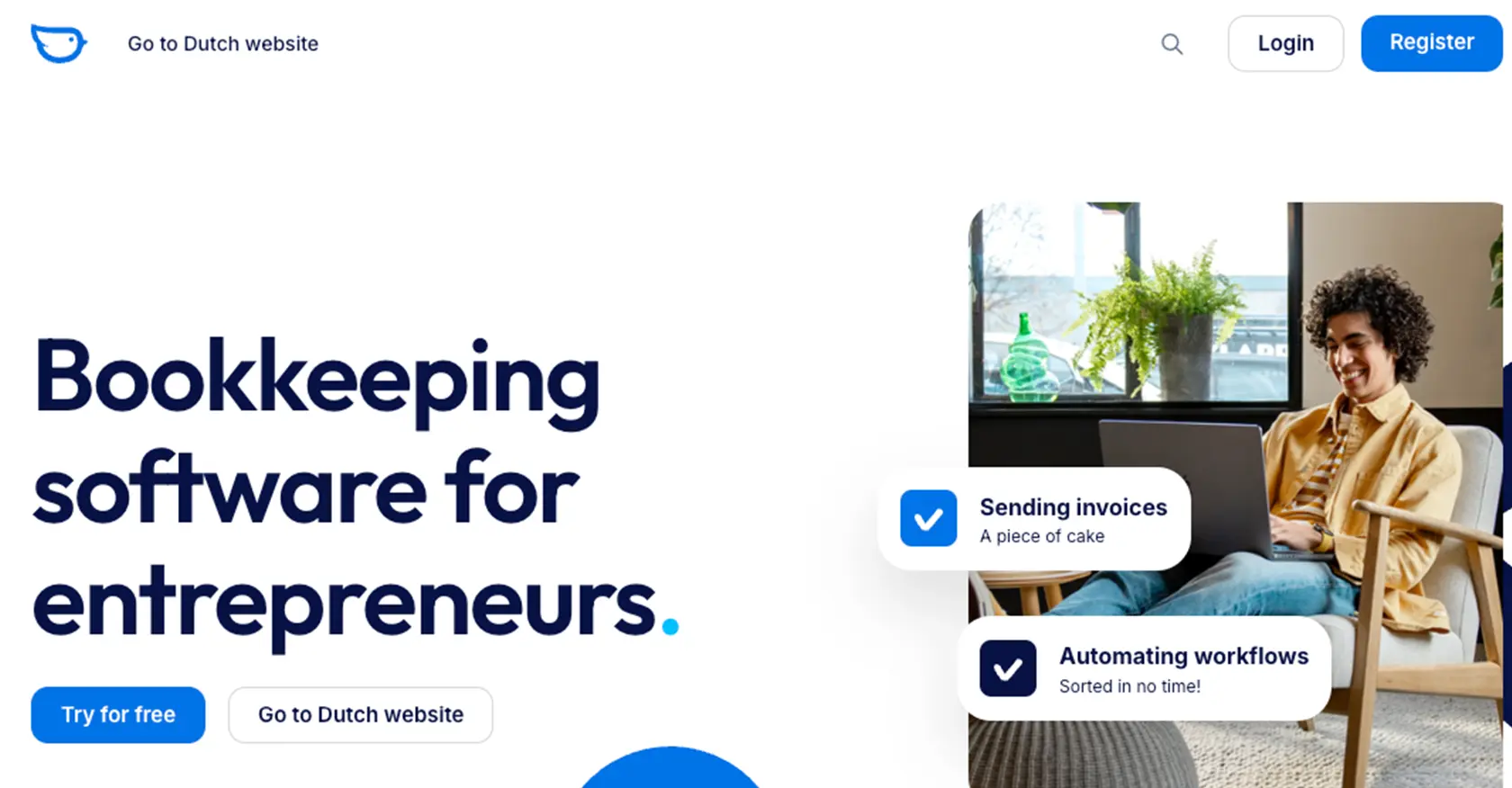
Introduction to Moneybird API for Recurring Invoices
Moneybird is a versatile online accounting software designed to simplify financial management for businesses. It offers a range of features including invoicing, expense tracking, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Developers might want to integrate with Moneybird's API to automate and streamline accounting processes. For example, accessing recurring invoices through the Moneybird API can help businesses manage their billing cycles efficiently, ensuring that invoices are sent out automatically at regular intervals without manual intervention.
This article will guide you through using JavaScript to interact with the Moneybird API, specifically focusing on retrieving recurring invoices. By the end of this tutorial, you'll be able to seamlessly integrate Moneybird's invoicing capabilities into your own applications.
Setting Up Your Moneybird Sandbox Account for API Integration
Before you begin interacting with the Moneybird API, it's essential to set up a sandbox account. This allows you to test your integration without affecting live data. Moneybird provides a sandbox environment that mimics the production environment, enabling you to experiment with API calls safely.
Creating a Moneybird Sandbox Account
- Visit the Moneybird website and sign up for a user account if you don't already have one.
- Once logged in, navigate to the sandbox creation page. This can be found under your account settings or directly via Moneybird's API documentation.
- Follow the instructions to create a sandbox administration. This will provide you with full access to Moneybird's features in a test environment.
Registering Your Application for OAuth Authentication
To access the Moneybird API, you'll need to authenticate using OAuth. Follow these steps to register your application:
- Log in to your Moneybird account and visit the application registration page.
- Fill in the required details to register your application. You will receive a Client ID and Client Secret, which are necessary for OAuth authentication.
- Ensure you store these credentials securely, as they are crucial for accessing the API.
Obtaining OAuth Access Tokens
With your application registered, you can now obtain access tokens to interact with the API:
- Initiate an authorization request to get a request token. Use the following URL format, replacing
client_id
andredirect_uri
with your application's details: - Redirect the user to the authorization URL provided in the response. Once authorized, Moneybird will redirect to your specified URI with an authorization code.
- Exchange the authorization code for an access token using the following command:
- Store the access token securely, as it will be used in the Authorization header for API requests.
curl -X GET "https://moneybird.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code"
curl -X POST "https://moneybird.com/oauth/token" \
-d "client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code"
For more detailed information on authentication, refer to the Moneybird Authentication Documentation.
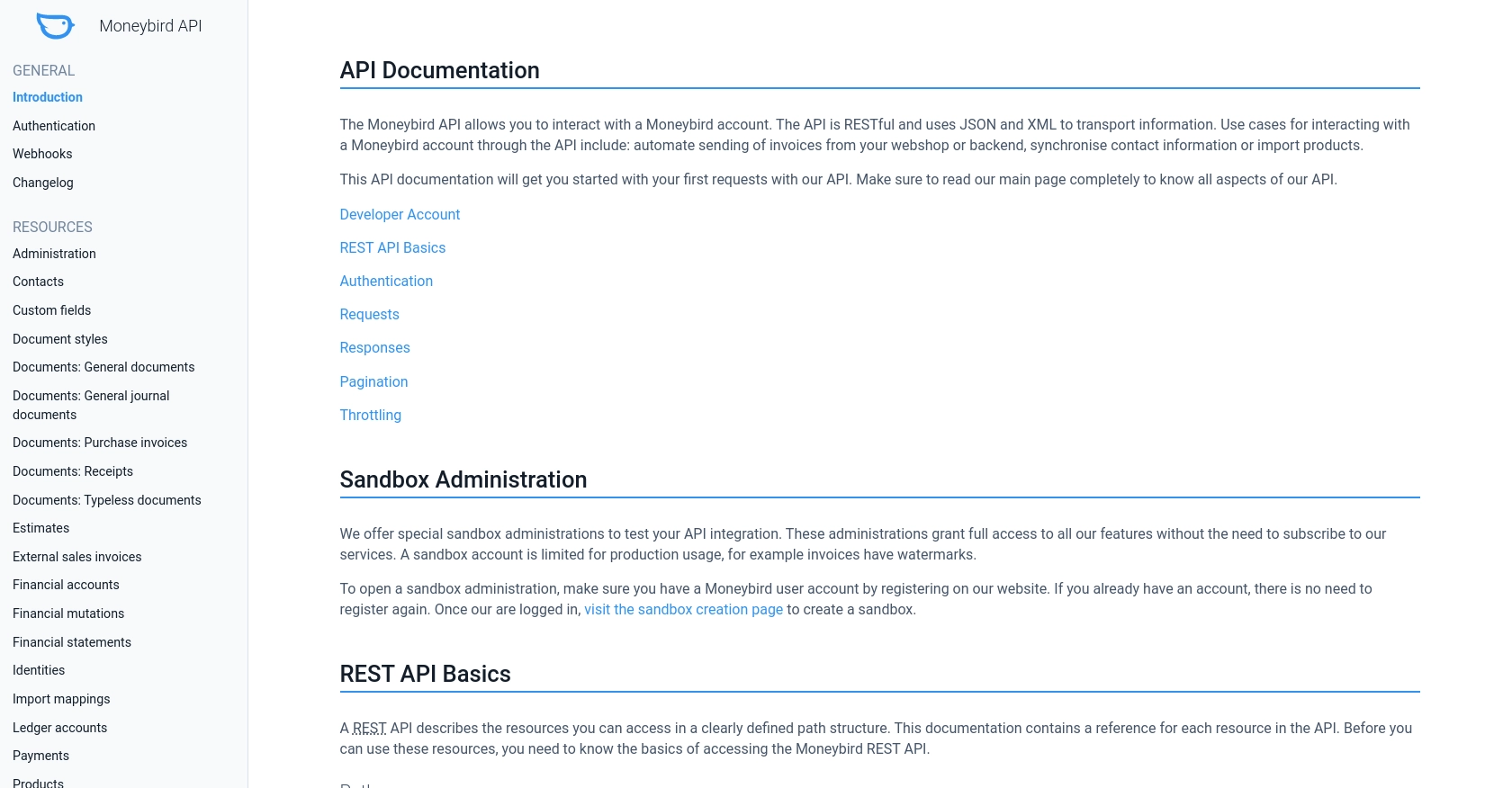
sbb-itb-96038d7
Making API Calls to Retrieve Recurring Invoices from Moneybird Using JavaScript
To interact with the Moneybird API and retrieve recurring invoices, you'll need to set up your development environment with JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Moneybird API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
- Familiarity with JavaScript and asynchronous programming.
To manage HTTP requests, you can use the popular axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Recurring Invoices from Moneybird
With your environment set up, you can now write the JavaScript code to interact with the Moneybird API. The following example demonstrates how to retrieve recurring invoices:
const axios = require('axios');
// Replace with your actual administration ID and access token
const administrationId = 'YOUR_ADMINISTRATION_ID';
const accessToken = 'YOUR_ACCESS_TOKEN';
// Define the API endpoint
const endpoint = `https://moneybird.com/api/v2/${administrationId}/recurring_sales_invoices.json`;
// Set up the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Bearer ${accessToken}`
};
// Function to get recurring invoices
async function getRecurringInvoices() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Recurring Invoices:', response.data);
} catch (error) {
console.error('Error fetching recurring invoices:', error.response ? error.response.data : error.message);
}
}
// Call the function
getRecurringInvoices();
In this code, you define the API endpoint using your administration ID and set the authorization header with your access token. The axios.get
method is used to make the GET request, and the response is logged to the console.
Handling Responses and Errors from Moneybird API
After making the API call, it's crucial to handle responses and errors appropriately:
- Successful responses will have a status code of
200
and contain the recurring invoices data. - Common error codes include
401
for unauthorized access and429
for too many requests. Refer to the Moneybird API documentation for a complete list of status codes and their meanings.
Ensure you implement error handling to manage these scenarios effectively, as shown in the example code.
Verifying API Call Success in Moneybird Sandbox
To verify the success of your API calls, check the Moneybird sandbox account. The retrieved recurring invoices should match the data in your sandbox environment. This ensures that your integration is functioning correctly.
For further details on the API endpoints and response structures, visit the Moneybird API documentation.
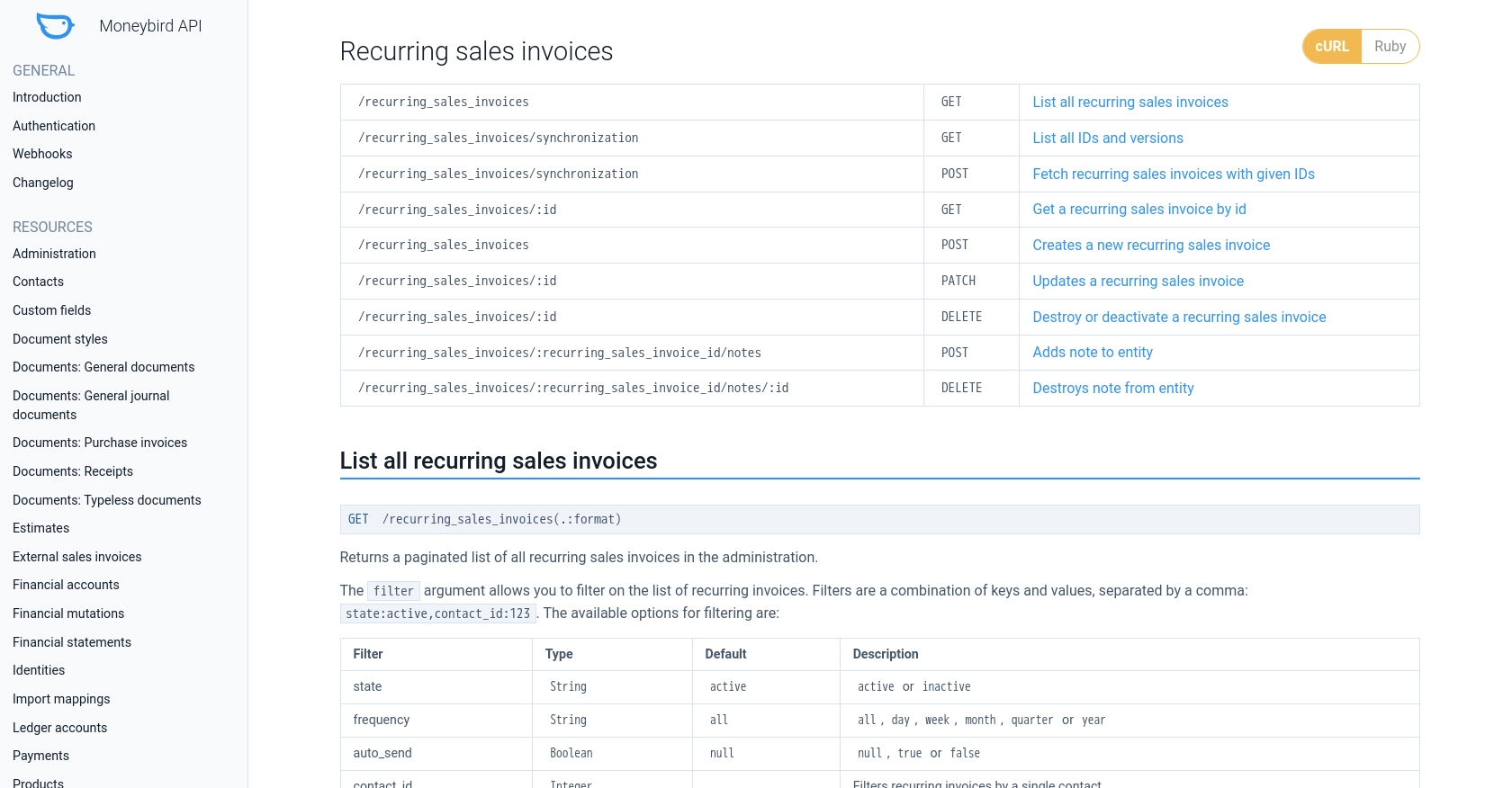
Conclusion and Best Practices for Using Moneybird API with JavaScript
Integrating with the Moneybird API to retrieve recurring invoices using JavaScript can significantly streamline your accounting processes. By automating invoice retrieval, businesses can ensure timely billing and maintain accurate financial records without manual intervention.
Best Practices for Secure and Efficient Moneybird API Integration
- Secure Storage of Credentials: Always store your OAuth credentials, such as Client ID, Client Secret, and access tokens, securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: The Moneybird API allows 150 requests every 5 minutes. Implement logic to handle rate limiting by checking the
429 Too Many Requests
status code and using theRetry-After
header to pause requests until the limit resets. - Data Standardization: Ensure that the data retrieved from Moneybird is standardized to match your application's requirements. This might include transforming date formats, currency symbols, or other fields.
- Error Handling: Implement robust error handling to manage various HTTP status codes. Log errors for debugging and provide user-friendly messages for known issues like authentication failures or invalid requests.
Enhancing Your Integration Experience with Endgrate
While integrating with Moneybird's API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various services, including Moneybird.
By using Endgrate, you can:
- Save time and resources by outsourcing integration management and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy and maintenance efforts.
- Provide an intuitive integration experience for your customers, enhancing their interaction with your application.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?