Using the Hubspot API to Create Deals in PHP
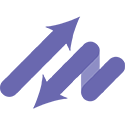
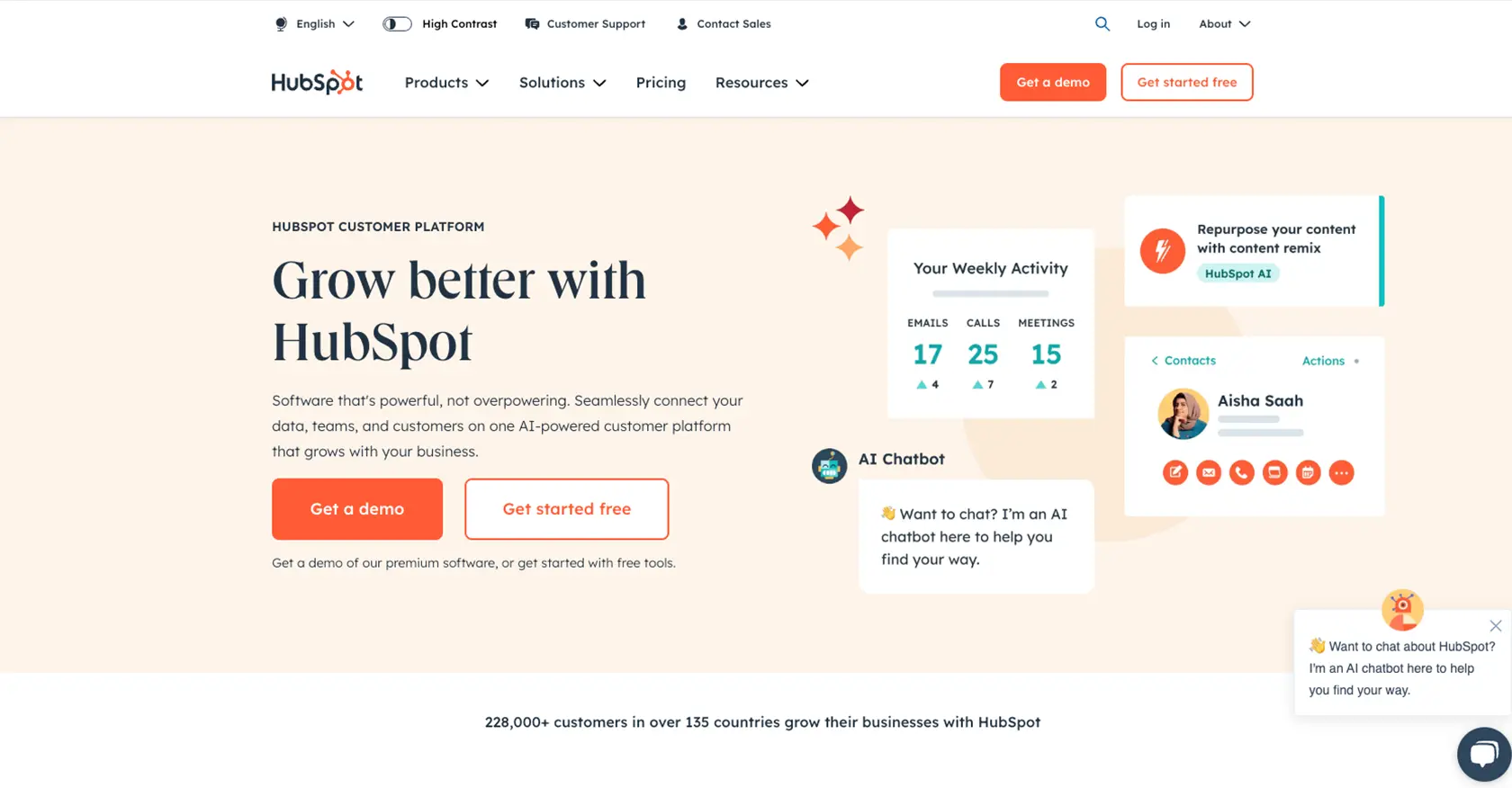
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for companies looking to streamline their operations and enhance customer engagement.
Integrating with HubSpot's API allows developers to automate and manage various CRM tasks, such as creating deals, which are essential for tracking sales opportunities. By using the HubSpot API, developers can efficiently manage deal records, ensuring that sales teams have up-to-date information to close deals effectively.
For example, a developer might use the HubSpot API to automatically create deals in the CRM whenever a potential customer fills out a contact form on the company's website. This automation ensures that no sales opportunity is missed and that the sales team can follow up promptly.
Setting Up Your HubSpot Test Account for API Integration
Before you can start creating deals using the HubSpot API in PHP, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. HubSpot provides a developer-friendly environment to facilitate this process.
Create a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the necessary details.
- Once your account is set up, log in to access the developer dashboard.
Generate a HubSpot Sandbox Account
With your developer account ready, you can now create a sandbox account:
- In the developer dashboard, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- This sandbox will mimic a real HubSpot account, allowing you to test API interactions.
Set Up OAuth Authentication for HubSpot API
HubSpot uses OAuth for secure API authentication. Follow these steps to set up OAuth:
- In your developer account, go to the "Apps" section and click "Create an app."
- Fill in the app details, including name and description.
- Under "Auth," select "OAuth 2.0" as the authentication method.
- Specify the necessary scopes for your app, such as
crm.objects.deals.write
for deal creation. - Save your app settings to generate a client ID and client secret.
For more detailed information on OAuth setup, refer to the HubSpot Authentication Documentation.
Obtain OAuth Access Token
To make API calls, you'll need an access token:
- Direct users to the OAuth authorization URL provided by HubSpot.
- Once authorized, HubSpot will redirect to your specified callback URL with an authorization code.
- Exchange this authorization code for an access token using the token endpoint.
Ensure you securely store the access token and refresh it as needed, as it will expire after a set period.
With your test account and OAuth setup complete, you're ready to start integrating with the HubSpot API to create deals in PHP.
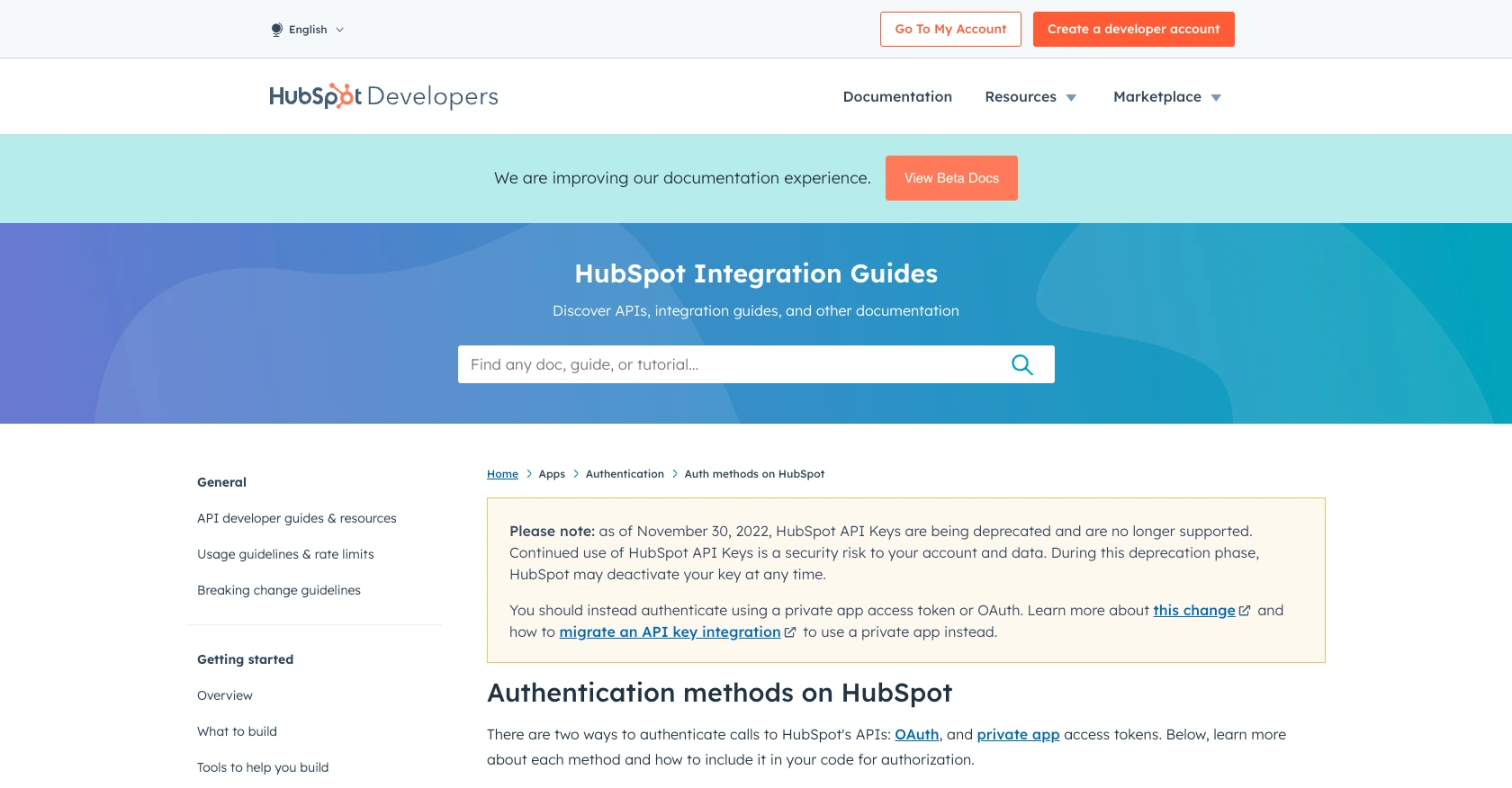
sbb-itb-96038d7
Making API Calls to Create Deals in HubSpot Using PHP
To create deals in HubSpot using PHP, you'll need to interact with the HubSpot API. This section will guide you through the process of setting up your PHP environment, making the necessary API calls, and handling responses effectively.
Setting Up Your PHP Environment for HubSpot API Integration
Before you start coding, ensure your PHP environment is properly configured:
- Install PHP 7.4 or higher on your server or local machine.
- Use Composer to manage dependencies. If you haven't installed Composer, follow the instructions on the Composer website.
- Install the HubSpot API client for PHP using Composer:
composer require hubspot/api-client
Creating a Deal in HubSpot with PHP
Once your environment is set up, you can proceed to create a deal in HubSpot. Here's a step-by-step guide:
require 'vendor/autoload.php';
use HubSpot\Factory;
use HubSpot\Client\Crm\Deals\ApiException;
// Initialize the HubSpot client
$client = Factory::createWithAccessToken('YOUR_ACCESS_TOKEN');
// Define the deal properties
$dealProperties = [
'properties' => [
'dealname' => 'New Deal',
'amount' => '1500.00',
'pipeline' => 'default',
'dealstage' => 'contractsent',
'closedate' => '2023-12-31T23:59:59Z'
]
];
try {
// Create the deal
$response = $client->crm()->deals()->basicApi()->create($dealProperties);
echo "Deal created successfully. Deal ID: " . $response->getId();
} catch (ApiException $e) {
echo "Exception when creating deal: ", $e->getMessage(), PHP_EOL;
}
Replace YOUR_ACCESS_TOKEN
with the OAuth access token you obtained earlier. This code initializes the HubSpot client, sets the deal properties, and makes a POST request to create the deal.
Verifying the API Call and Handling Responses
After executing the API call, you should verify the response to ensure the deal was created successfully:
- Check the response status code. A successful creation will return a 201 status code.
- Log the response or display a success message with the deal ID.
- Handle any exceptions using try-catch blocks to manage errors gracefully.
For more detailed information on error handling and response codes, refer to the HubSpot Deals API Documentation.
Testing and Debugging Your HubSpot API Integration
To ensure your integration works as expected, perform the following tests:
- Create test deals in your sandbox account and verify their appearance in the HubSpot dashboard.
- Check for any error messages or exceptions in your PHP logs.
- Use HubSpot's API monitoring tools to track API usage and performance.
By following these steps, you can effectively integrate with the HubSpot API to create deals using PHP, enhancing your CRM capabilities and automating sales processes.
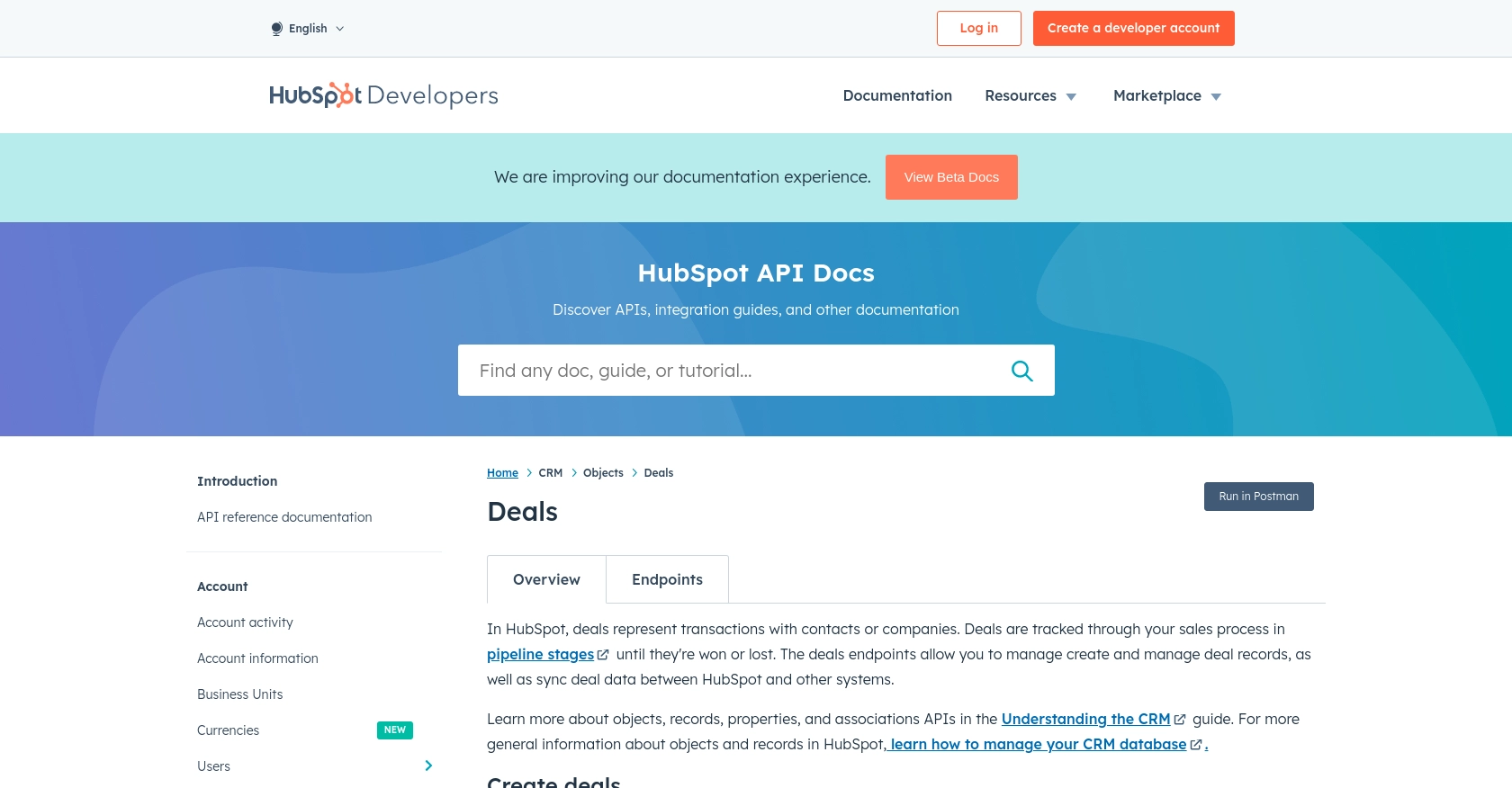
Conclusion and Best Practices for HubSpot API Integration Using PHP
Integrating with the HubSpot API to create deals using PHP can significantly enhance your CRM capabilities by automating sales processes and ensuring that your sales team has access to the most up-to-date information. By following the steps outlined in this guide, you can efficiently set up your PHP environment, authenticate using OAuth, and make API calls to manage deals in HubSpot.
Best Practices for Secure and Efficient HubSpot API Integration
- Securely Store Credentials: Always store your OAuth access tokens securely and refresh them as needed to maintain access without compromising security.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which allow 100 requests every 10 seconds for OAuth apps. Implement throttling to avoid hitting these limits and receiving 429 error responses. For more details, refer to the HubSpot API Usage Guidelines.
- Standardize Data Fields: Ensure that data fields are standardized and consistent across your application to maintain data integrity and facilitate seamless integration.
- Error Handling: Implement robust error handling to gracefully manage API exceptions and ensure that your application can recover from unexpected issues.
Enhance Your Integration Strategy with Endgrate
While integrating with HubSpot's API can be a powerful way to automate CRM tasks, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product while outsourcing the complexities of integration management.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including HubSpot. This streamlined approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/deals
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?