How to Get Vendors with the Sap Business One API in Javascript
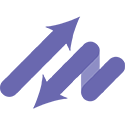
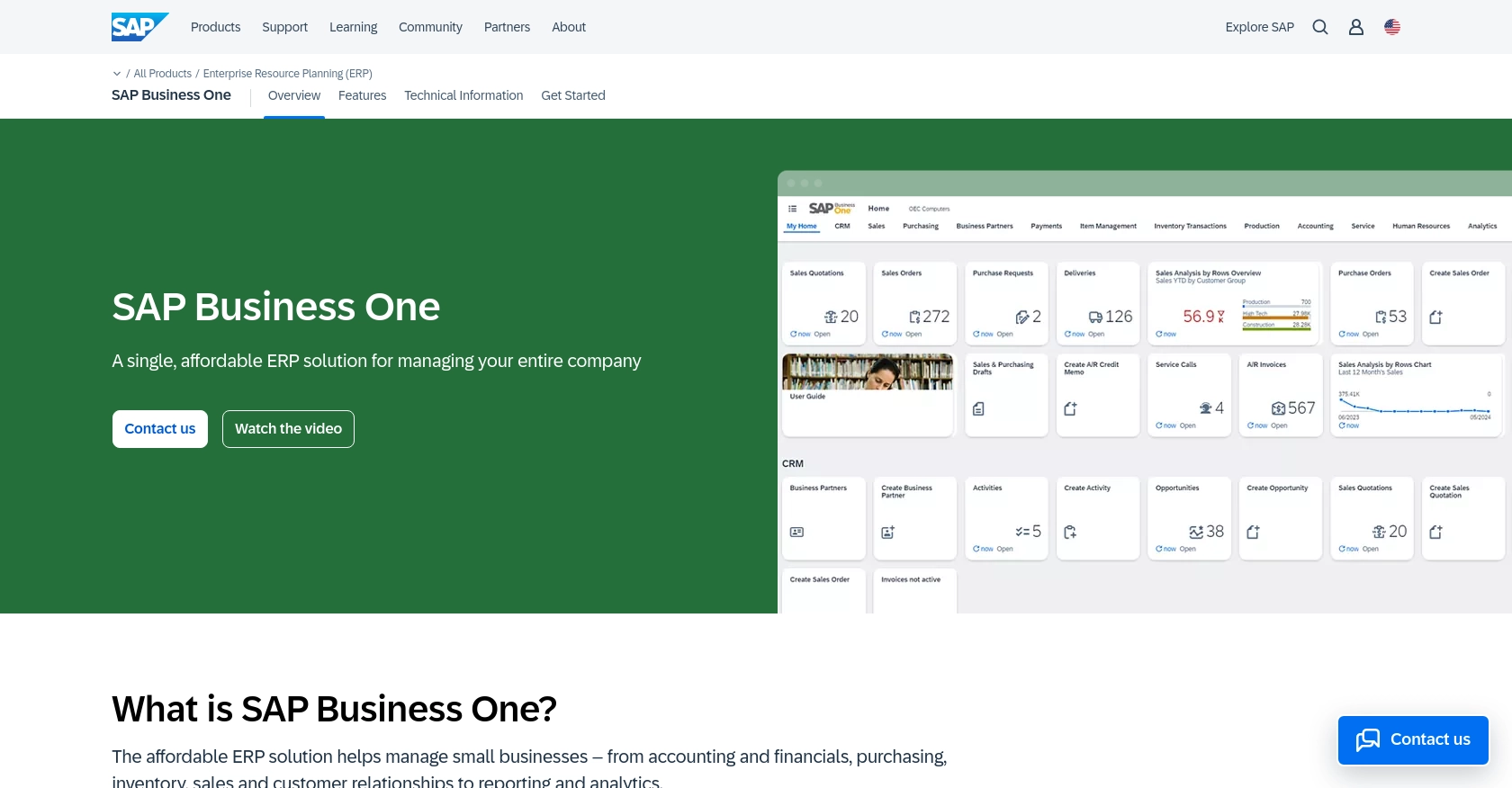
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small and medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain operations. By integrating with SAP Business One's API, developers can streamline business processes and enhance operational efficiency.
Connecting with the SAP Business One API allows developers to access and manage various business data, such as vendor information. For example, a developer might want to retrieve vendor details to automate inventory management or streamline procurement processes. This article will guide you through using JavaScript to interact with the SAP Business One API to fetch vendor data efficiently.
Setting Up Your SAP Business One Test/Sandbox Account
Before you can start interacting with the SAP Business One API using JavaScript, you'll need to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting live data, ensuring a safe space for development and testing.
Creating a SAP Business One Sandbox Account
To begin, you'll need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, contact your system administrator to request access to a sandbox instance. If not, you may need to reach out to SAP or a certified partner to set up a trial or demo account.
Generating API Credentials for SAP Business One
SAP Business One uses a custom authentication method for API access. Follow these steps to generate the necessary credentials:
- Log in to your SAP Business One sandbox account.
- Navigate to the administration panel and locate the API management section.
- Create a new API user or application. This will provide you with a client ID and client secret, which are essential for authenticating your API requests.
- Ensure that the API user has the necessary permissions to access vendor data.
Configuring OAuth for SAP Business One API
Although SAP Business One uses a custom authentication method, it often involves OAuth-like steps. Here's how to configure it:
- Use the client ID and client secret obtained earlier to request an access token from the SAP Business One authentication server.
- Store the access token securely, as it will be used to authorize API requests.
- Note that access tokens may expire, so implement a mechanism to refresh them as needed.
With your sandbox account and API credentials set up, you're ready to start making API calls to retrieve vendor information using JavaScript. In the next section, we'll walk through the process of making these calls and handling the responses.
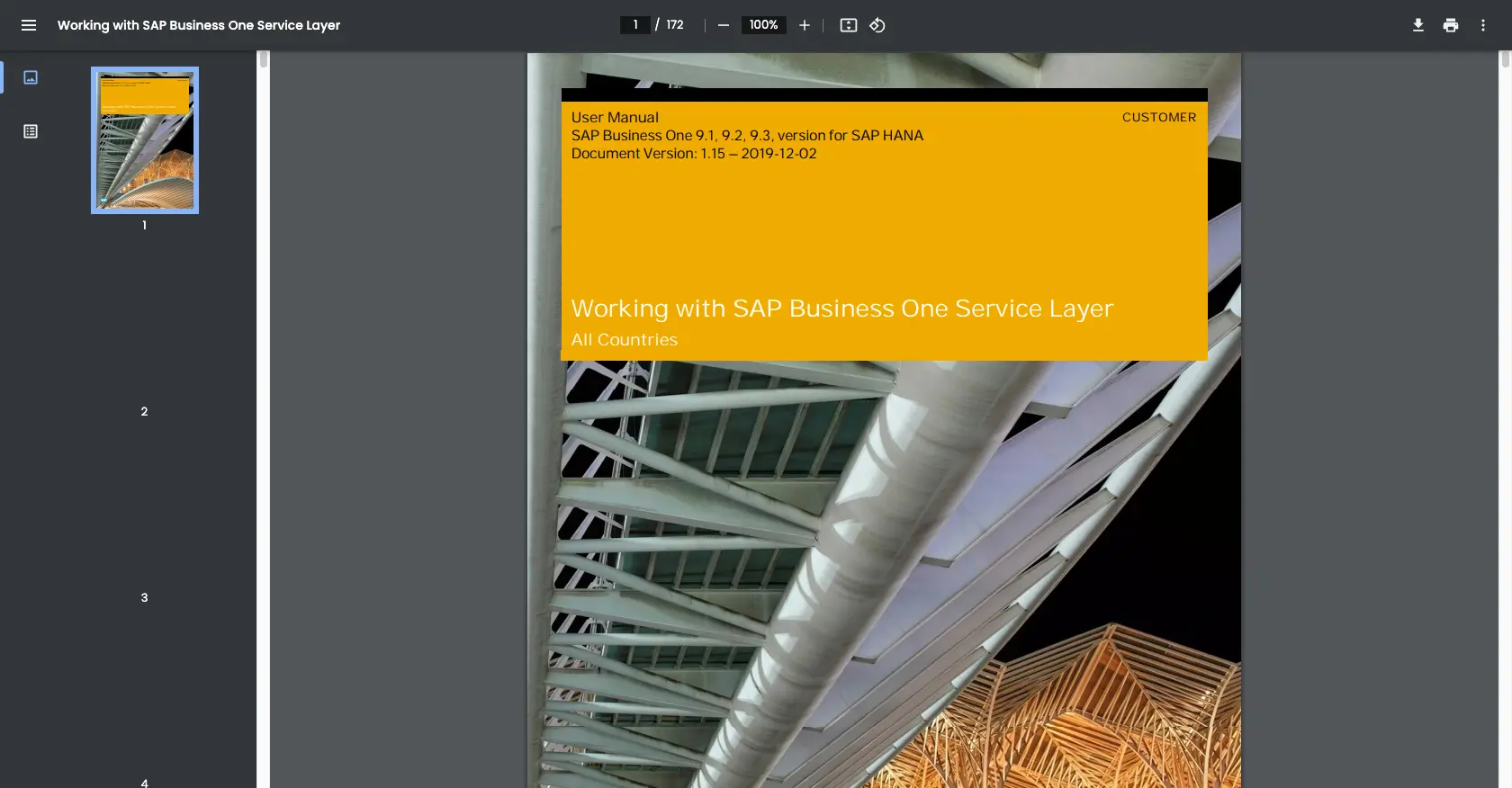
sbb-itb-96038d7
Making API Calls to Retrieve Vendor Information with SAP Business One in JavaScript
To interact with the SAP Business One API using JavaScript, you need to set up your development environment and write code to make API requests. This section will guide you through the process of retrieving vendor information efficiently.
Setting Up Your JavaScript Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor, such as Visual Studio Code, to write and manage your JavaScript code.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Vendor Data from SAP Business One API
With your environment set up, you can now write the JavaScript code to retrieve vendor information. Create a new file named getVendors.js
and add the following code:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Vendors';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Function to fetch vendor data
async function getVendors() {
try {
const response = await axios.get(endpoint, { headers });
const vendors = response.data.value;
// Log vendor information
vendors.forEach(vendor => {
console.log(`Vendor Name: ${vendor.VendorName}, Vendor Code: ${vendor.VendorCode}`);
});
} catch (error) {
console.error('Error fetching vendor data:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getVendors();
Replace Your_Access_Token
with the access token you obtained during the authentication setup.
Running the JavaScript Code and Verifying API Response
To execute the code, open your terminal and run the following command:
node getVendors.js
If the request is successful, you should see a list of vendors printed in the console. Verify the data by cross-checking with the vendor information in your SAP Business One sandbox account.
Handling Errors and Troubleshooting API Requests
When making API calls, it's essential to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Common issues may include:
- Invalid access token: Ensure your token is correct and not expired.
- Network issues: Check your internet connection and endpoint URL.
- Insufficient permissions: Verify that your API user has the necessary permissions to access vendor data.
For more detailed error information, refer to the SAP Business One API documentation: SAP Business One Service Layer Documentation.
Conclusion and Best Practices for Using SAP Business One API in JavaScript
Integrating with the SAP Business One API using JavaScript provides developers with the ability to automate and streamline business processes, enhancing operational efficiency. By following the steps outlined in this article, you can successfully retrieve vendor information and leverage it for various business applications.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials, such as access tokens, securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of any rate limits imposed by the SAP Business One API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized according to your application's requirements. This will help maintain data consistency across systems.
- Regular Token Refresh: Implement mechanisms to refresh access tokens regularly to avoid authentication failures due to expired tokens.
Streamlining Integrations with Endgrate
While integrating with SAP Business One API can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including SAP Business One.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Whether you need to build once for each use case or provide an intuitive integration experience for your customers, Endgrate can help streamline your integration efforts.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
Ready to get started?