Using the Linear API to Get Issues (with Javascript examples)
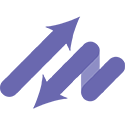
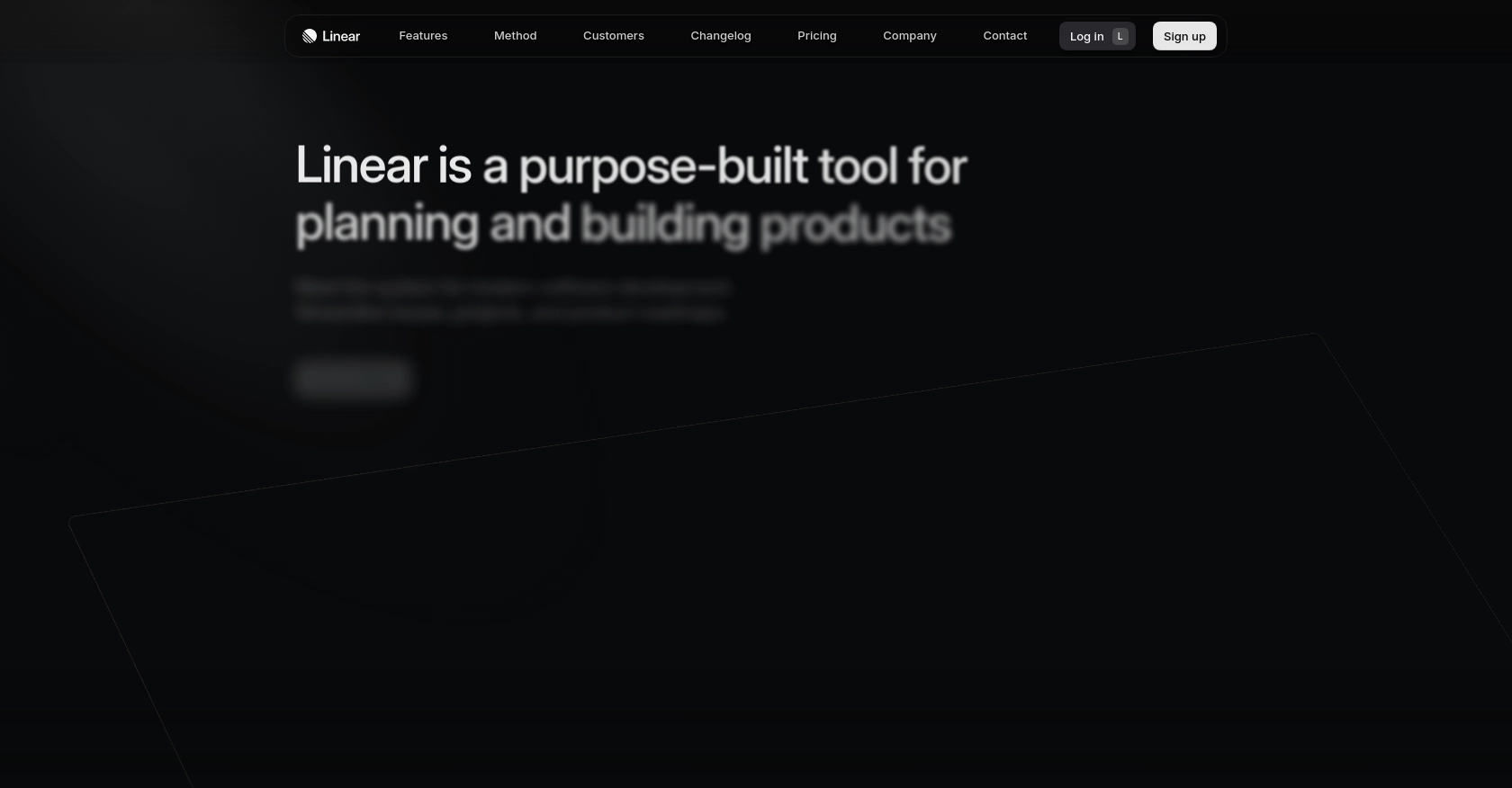
Introduction to Linear API Integration
Linear is a modern issue tracking tool designed to streamline project management and enhance team productivity. It offers a sleek interface and powerful features that help software development teams manage tasks, track progress, and collaborate effectively.
Integrating with Linear's API allows developers to automate workflows and access critical project data. For example, you might want to retrieve a list of issues assigned to a specific team member to generate a custom report or dashboard. This can help teams stay organized and prioritize tasks efficiently.
Setting Up a Linear Test/Sandbox Account for API Integration
Before you can start interacting with the Linear API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your production data.
Creating a Linear Account
If you don't already have a Linear account, you can sign up for a free trial on the Linear website. This will give you access to all the features you need to test the API integration.
- Visit the Linear website and click on "Sign Up".
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Linear dashboard.
Setting Up OAuth 2.0 Authentication for Linear API
Linear supports OAuth 2.0 authentication, which is recommended for building applications that integrate with Linear. Follow these steps to create an OAuth 2.0 application:
- Navigate to the "Settings" section in your Linear dashboard.
- Under "Integrations", select "OAuth Applications" and click "Create New Application".
- Fill in the required details, including the redirect callback URLs for your application.
- Save the application to generate your Client ID and Client Secret.
Authorizing Users with OAuth 2.0
To authorize users, redirect them to Linear's authorization URL with the appropriate parameters:
GET https://linear.app/oauth/authorize?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URL&scope=read
After the user approves your application, they will be redirected back to your specified URL with an authorization code.
Exchanging Authorization Code for Access Token
Once you have the authorization code, exchange it for an access token by making a POST request:
POST https://api.linear.app/oauth/token
Content-Type: application/x-www-form-urlencoded
Parameters:
- code: Authorization code from the previous step
- redirect_uri: Same redirect URI used previously
- client_id: Your application's client ID
- client_secret: Your application's client secret
- grant_type: authorization_code
Upon successful request, you will receive an access token, which you can use to authenticate API calls.
For more detailed information on OAuth 2.0 authentication with Linear, refer to the official Linear OAuth documentation.
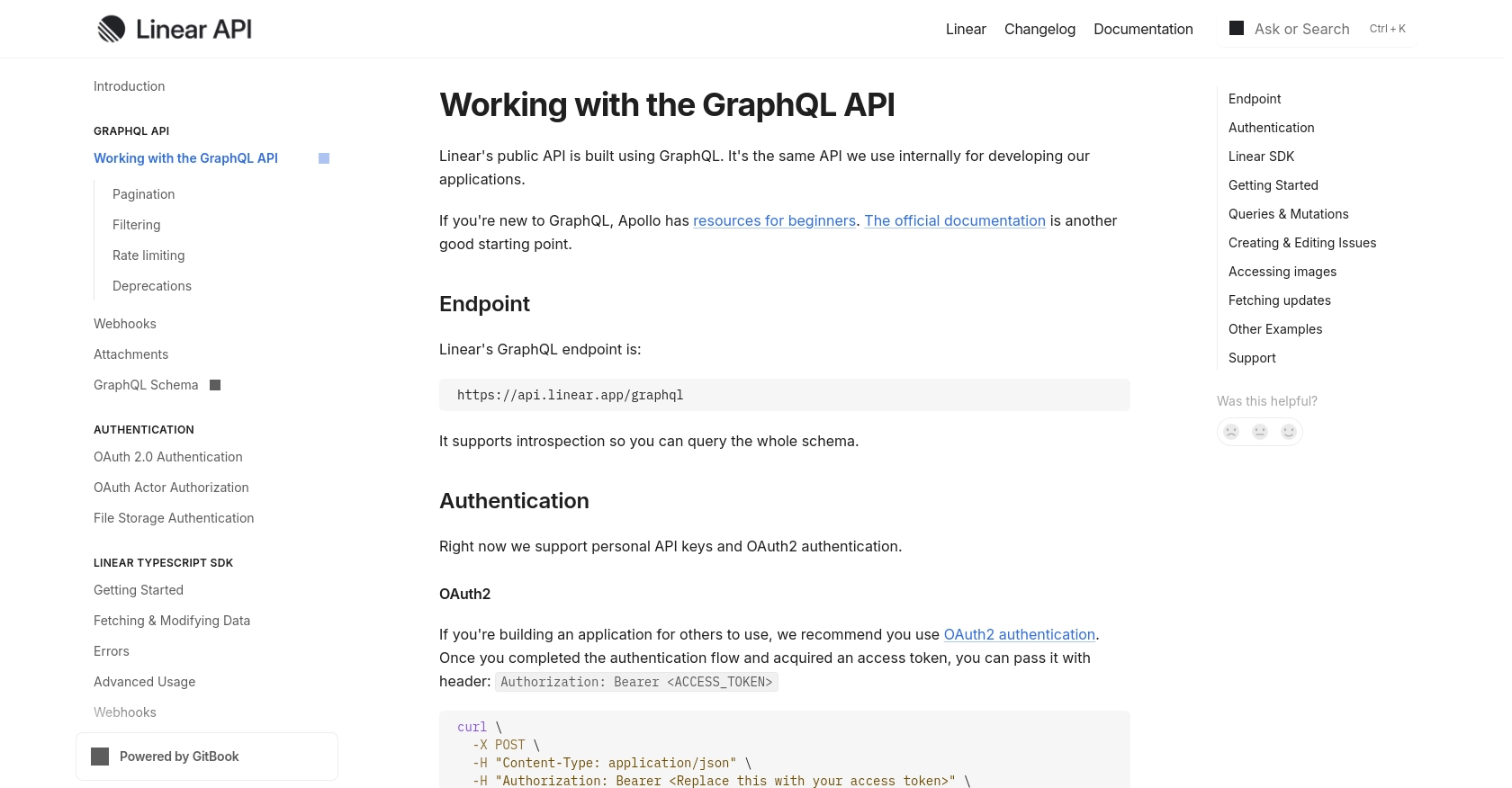
sbb-itb-96038d7
Making API Calls to Retrieve Issues from Linear Using JavaScript
To interact with the Linear API and retrieve issues, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment and writing the code to fetch issues.
Setting Up Your JavaScript Environment for Linear API Integration
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can set up a new project and install the required dependencies. Open your terminal and run the following commands:
mkdir linear-api-integration
cd linear-api-integration
npm init -y
npm install axios
The axios
library will be used to make HTTP requests to the Linear API.
Writing JavaScript Code to Fetch Issues from Linear API
Create a new file named getLinearIssues.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.linear.app/graphql';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Define the GraphQL query to fetch issues
const query = `
query {
issues {
nodes {
id
title
description
createdAt
}
}
}
`;
// Make a POST request to the Linear API
axios.post(endpoint, { query }, { headers })
.then(response => {
const issues = response.data.data.issues.nodes;
issues.forEach(issue => {
console.log(`ID: ${issue.id}, Title: ${issue.title}, Description: ${issue.description}`);
});
})
.catch(error => {
console.error('Error fetching issues:', error);
});
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 authentication process.
Running the JavaScript Code to Retrieve Linear Issues
To execute the code and fetch issues from Linear, run the following command in your terminal:
node getLinearIssues.js
Upon successful execution, you should see a list of issues printed in the console, including their IDs, titles, and descriptions.
Handling Errors and Validating API Requests
When making API calls, it's essential to handle potential errors gracefully. The code above includes a catch
block to log any errors that occur during the request. Additionally, verify the success of your API calls by checking the response data and ensuring it matches the expected output in your Linear test account.
For more information on handling errors, refer to the Linear API documentation.
Conclusion and Best Practices for Using Linear API with JavaScript
Integrating with the Linear API using JavaScript provides a powerful way to automate workflows and access project data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve issues and enhance your team's productivity.
Best Practices for Secure and Efficient Linear API Integration
- Securely Store Credentials: Always store your OAuth 2.0 credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Linear's API rate limits to avoid disruptions. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Linear is standardized and transformed as needed to fit your application's requirements. This will help maintain consistency across different systems.
Leverage Endgrate for Streamlined Integration Management
While building integrations with Linear can be rewarding, it can also be time-consuming. Endgrate offers a unified API solution that simplifies integration management across multiple platforms, including Linear. By using Endgrate, you can save time and resources, allowing your team to focus on core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website. Take advantage of their intuitive platform to build once and deploy across various integrations effortlessly.
Read More
Ready to get started?