Using the BigCommerce API to Get Customers in Javascript
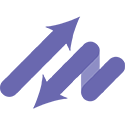
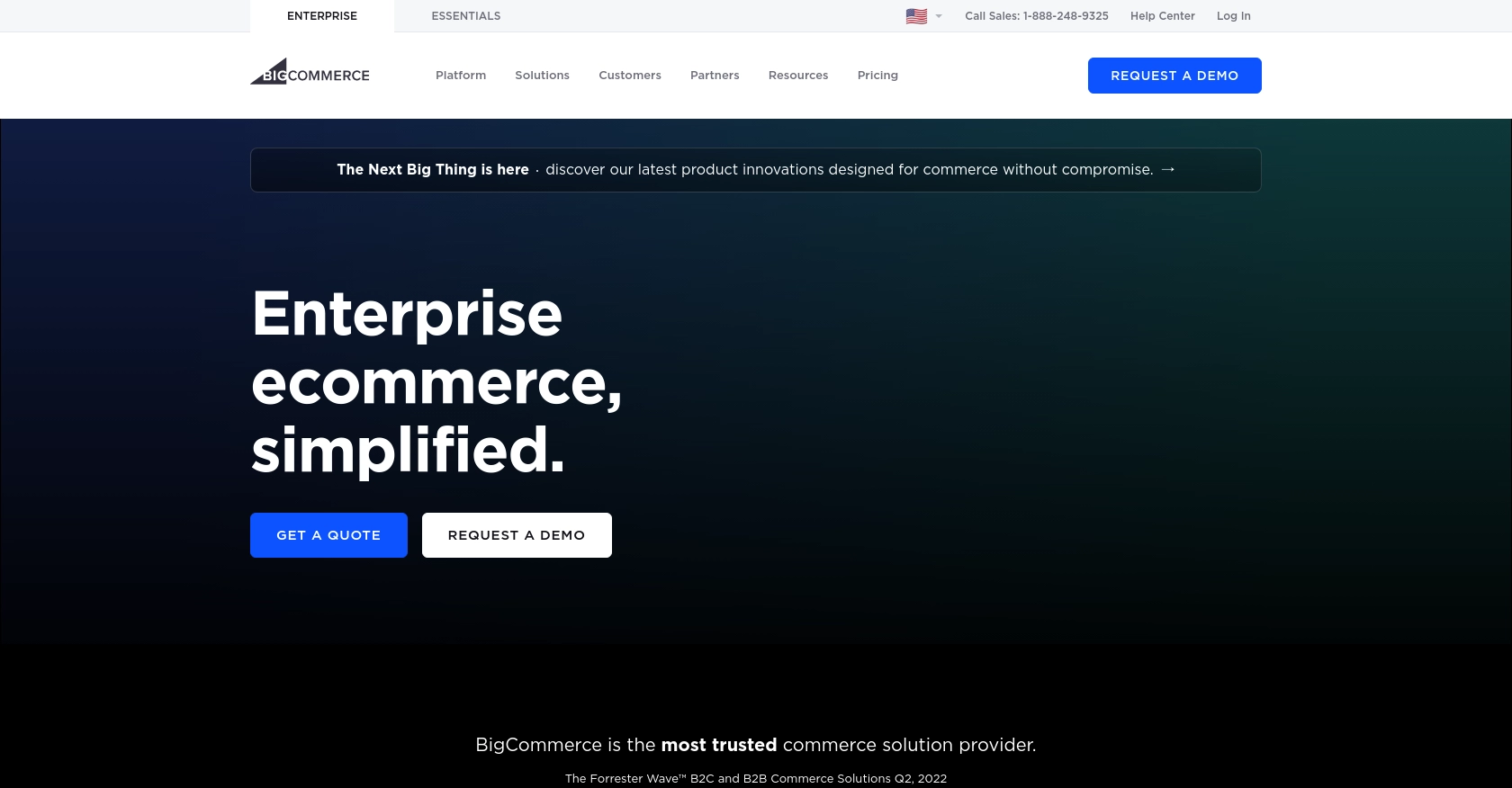
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide range of features, including product management, order processing, and customer engagement tools, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to access and manipulate store data programmatically. This can be particularly useful for automating tasks such as retrieving customer information, which can enhance customer relationship management and streamline marketing efforts. For example, a developer might use the BigCommerce API to fetch customer data and use it to personalize marketing campaigns or improve customer service interactions.
Setting Up a BigCommerce Test or Sandbox Account
Before you can start interacting with the BigCommerce API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting a live store. Here's how to get started:
Create a BigCommerce Sandbox Account
To begin, you'll need to create a BigCommerce sandbox account. This account will provide you with a testing environment that mimics a live store.
- Visit the BigCommerce Developer Portal and sign up for a developer account if you haven't already.
- Once registered, navigate to the "Sandbox Stores" section and create a new sandbox store. Follow the prompts to set up your store with the necessary configurations.
Generate API Credentials for BigCommerce
After setting up your sandbox store, you'll need to generate API credentials to authenticate your requests.
- Log in to your BigCommerce control panel and go to the "Advanced Settings" section.
- Select "API Accounts" and click on "Create API Account."
- Choose the type of API account that suits your needs (e.g., "Store API Account").
- Configure the OAuth scopes required for accessing customer data. Make sure to include permissions for reading customer information.
- Once configured, save the account to generate your API credentials, including the Client ID, Client Secret, and Access Token.
Securely Store Your BigCommerce API Credentials
It's crucial to store your API credentials securely to prevent unauthorized access. Consider the following best practices:
- Use environment variables to store sensitive information like the Client ID and Access Token.
- Ensure that your code repository does not contain any hard-coded credentials.
- Regularly rotate your API keys and tokens to maintain security.
With your sandbox account and API credentials ready, you're now set to start making API calls to BigCommerce. In the next section, we'll explore how to use JavaScript to fetch customer data from your BigCommerce store.
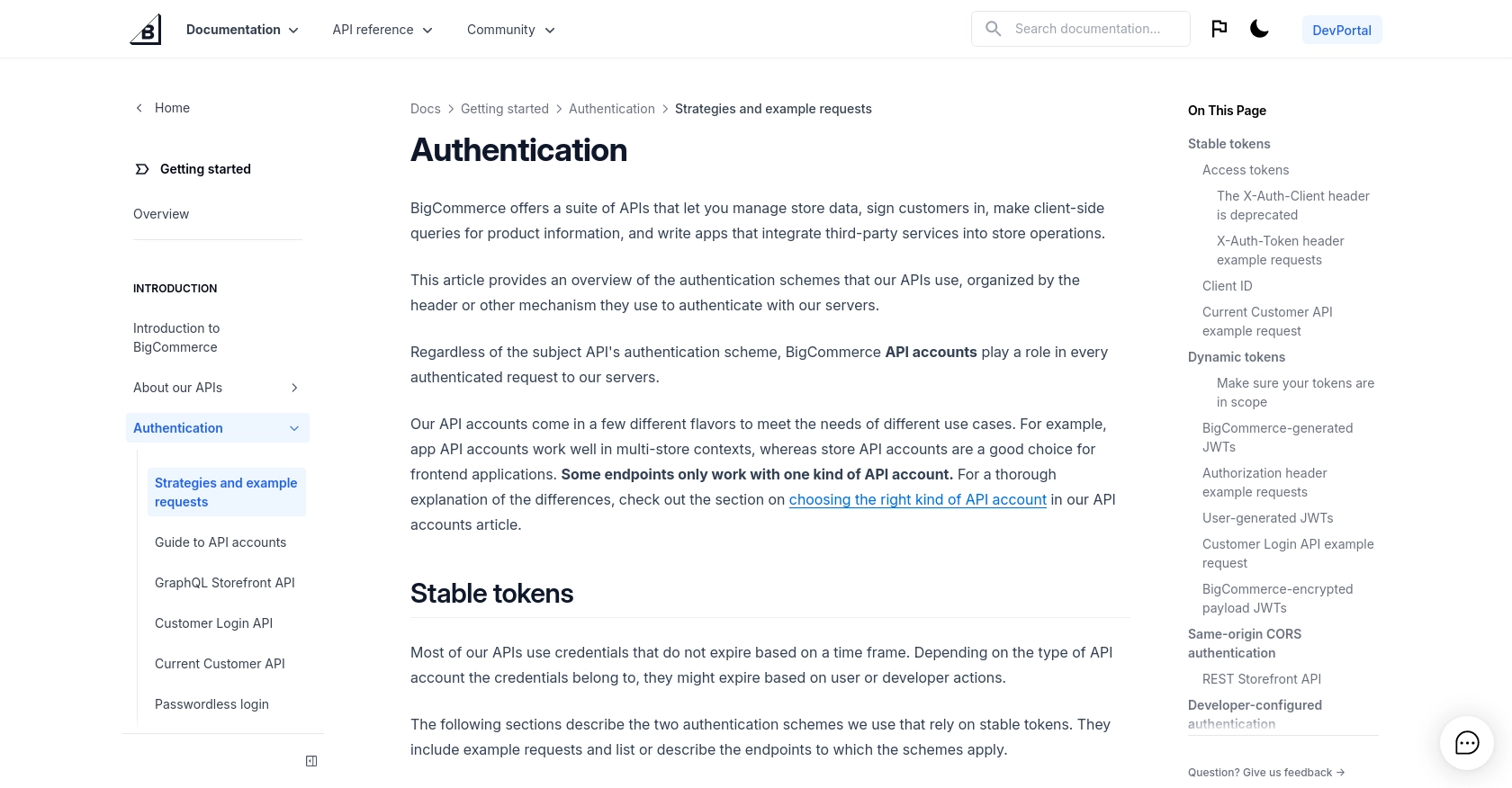
sbb-itb-96038d7
Making API Calls to Retrieve Customers from BigCommerce Using JavaScript
To interact with the BigCommerce API and retrieve customer data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for BigCommerce API
Before you begin coding, ensure you have the necessary tools and libraries:
- Ensure you have a modern web browser with JavaScript support.
- Use a code editor like Visual Studio Code or Sublime Text for writing your JavaScript code.
- Familiarize yourself with the Fetch API or a library like Axios for making HTTP requests.
Writing JavaScript Code to Fetch Customers from BigCommerce
With your environment ready, you can now write the JavaScript code to fetch customer data from BigCommerce. Follow these steps:
// Define the API endpoint and your store's access token
const endpoint = 'https://api.bigcommerce.com/stores/{{STORE_HASH}}/v3/customers';
const accessToken = 'Your_Access_Token';
// Set up the request headers
const headers = {
'X-Auth-Token': accessToken,
'Accept': 'application/json',
'Content-Type': 'application/json'
};
// Function to fetch customers
async function fetchCustomers() {
try {
const response = await fetch(endpoint, { headers });
if (response.ok) {
const data = await response.json();
console.log('Customer Data:', data);
} else {
console.error('Error fetching customers:', response.status, response.statusText);
}
} catch (error) {
console.error('Network error:', error);
}
}
// Call the function to fetch customers
fetchCustomers();
Replace {{STORE_HASH}}
and Your_Access_Token
with your actual store hash and access token obtained from your BigCommerce API credentials.
Understanding the Response and Handling Errors
After executing the code, you should see the customer data logged in the console. Here's how to verify and handle responses:
- Check the response status code to ensure the request was successful (status code 200).
- Handle errors gracefully by logging them and providing meaningful messages.
- Use the returned data to perform further operations, such as displaying customer information on your website.
Verifying API Call Success in BigCommerce Sandbox
To confirm that your API call is successful, log in to your BigCommerce sandbox account and verify the customer data matches the response. This ensures your integration is working as expected.
By following these steps, you can effectively use JavaScript to interact with the BigCommerce API and retrieve customer data, enhancing your e-commerce operations.
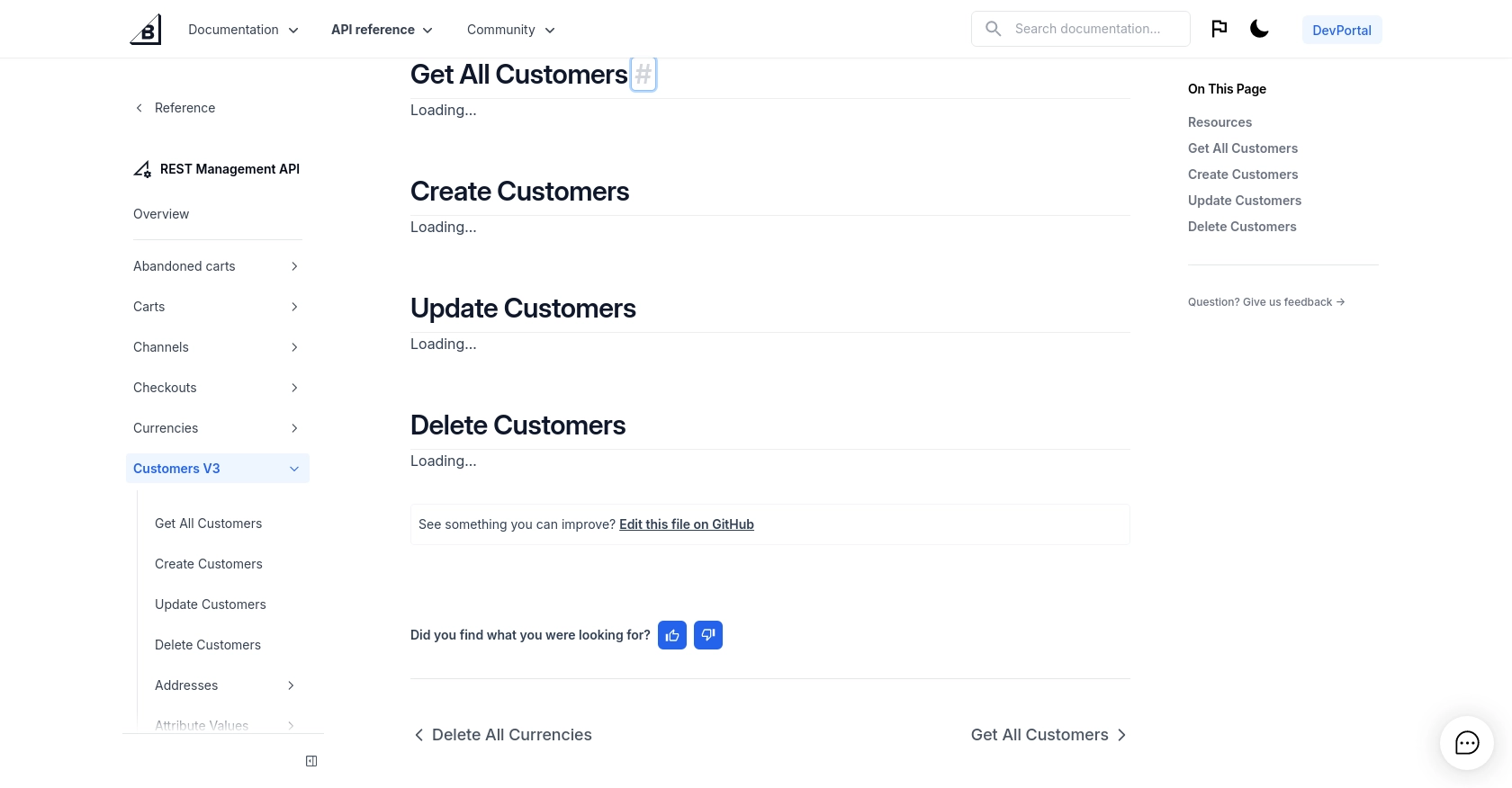
Conclusion and Best Practices for Using BigCommerce API with JavaScript
Integrating with the BigCommerce API using JavaScript provides a powerful way to automate and enhance your e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve customer data and use it to improve customer engagement and streamline your business processes.
Best Practices for Storing BigCommerce API Credentials
- Always store your API credentials securely using environment variables or secure vaults.
- Avoid hard-coding sensitive information directly in your source code.
- Regularly rotate your API keys and tokens to maintain security.
Handling BigCommerce API Rate Limits
BigCommerce imposes rate limits on API requests to ensure fair usage. It's important to handle these limits gracefully:
- Implement exponential backoff strategies to retry requests when rate limits are reached.
- Monitor your API usage to stay within the allowed limits and avoid disruptions.
Transforming and Standardizing Customer Data from BigCommerce
When working with customer data, consider transforming and standardizing the data fields to match your application's requirements. This can include normalizing names, formatting addresses, and ensuring consistent data types.
Call to Action: Simplify Integrations with Endgrate
While integrating with BigCommerce API can be rewarding, it can also be complex and time-consuming. Endgrate offers a solution to simplify your integration process. By using Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?