How to Get Users with the Copper API in Python
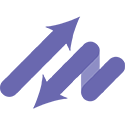
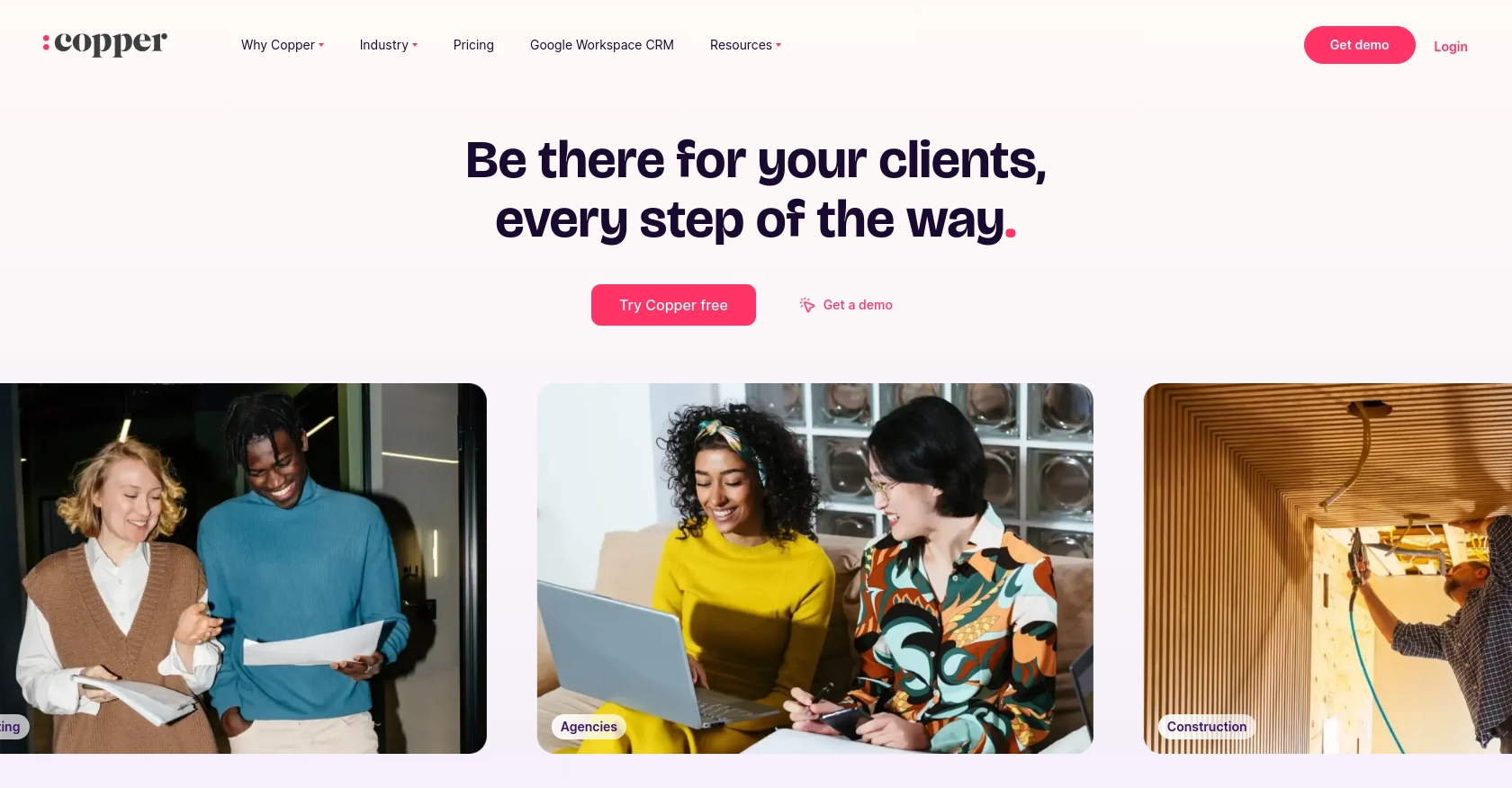
Introduction to Copper API
Copper is a robust platform designed to streamline cryptocurrency management for businesses. It offers a comprehensive suite of tools for managing digital assets, including trading, staking, and settlement services. Copper's API provides developers with the ability to integrate these functionalities into their own applications, enabling seamless interaction with the platform.
Integrating with Copper's API allows developers to automate various processes, such as retrieving user data, managing portfolios, and executing trades. For example, a developer might use the Copper API to fetch user information and display it in a custom dashboard, providing real-time insights into user activities and asset holdings.
In this article, we will explore how to interact with the Copper API using Python, focusing on retrieving user data. This guide will walk you through the necessary steps to set up your environment, authenticate with the API, and execute API calls to get user information efficiently.
Setting Up Your Copper API Test or Sandbox Account
Before you can start interacting with the Copper API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Copper Account
If you don't already have a Copper account, visit the Copper website and sign up for a free trial or demo account. This will give you access to the platform's features and the ability to create API keys for testing purposes.
Generate Copper API Keys
Once your account is set up, you'll need to generate API keys to authenticate your requests. Follow these steps:
- Log in to your Copper account.
- Navigate to the API section in your account settings.
- Click on "Create API Key" and provide a name for your key.
- Copy the API key and API secret. Store them securely as you'll need them for authentication.
Understanding Copper's Custom Authentication
Copper uses a custom authentication method that requires you to sign your requests. Here's how you can set it up in Python:
import hashlib
import hmac
import time
# Replace with your Copper API key and secret
api_key = 'your_api_key'
api_secret = 'your_api_secret'
# Generate a timestamp
timestamp = str(round(time.time() * 1000))
# Define the HTTP method and endpoint path
method = "GET"
path = "/platform/accounts"
# Create the signature
signature = hmac.new(
key=bytes(api_secret, 'utf-8'),
msg=bytes(timestamp + method + path, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
# Headers for the API request
headers = {
'Authorization': f'ApiKey {api_key}',
'X-Signature': signature,
'X-Timestamp': timestamp
}
With these headers, you can now authenticate your requests to the Copper API. This setup ensures that your API interactions are secure and authorized.
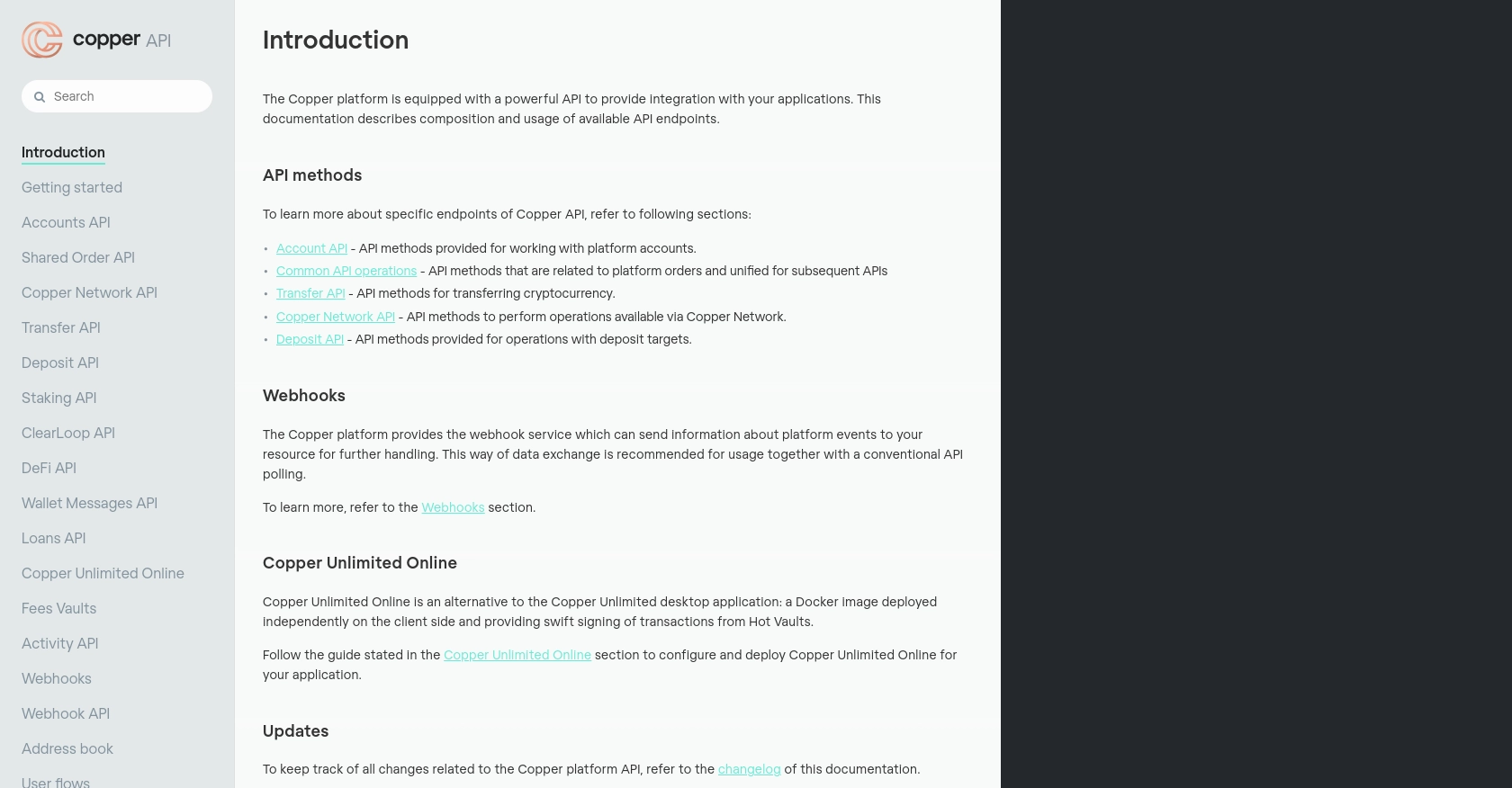
sbb-itb-96038d7
Executing Copper API Calls Using Python
To interact with the Copper API and retrieve user data, you'll need to set up your Python environment and execute API calls. This section will guide you through the process, ensuring you have the necessary tools and code to make successful requests.
Python Environment Setup for Copper API Integration
Before making API calls, ensure your Python environment is ready. You'll need Python 3.7 or later and the requests
library to handle HTTP requests. Install the library using pip:
pip install requests
Sample Code to Retrieve User Data from Copper API
With your environment set up, you can now write a Python script to fetch user data from Copper. Here's a sample code snippet:
import requests
import hashlib
import hmac
import time
# Replace with your Copper API key and secret
api_key = 'your_api_key'
api_secret = 'your_api_secret'
# Generate a timestamp
timestamp = str(round(time.time() * 1000))
# Define the HTTP method and endpoint path
method = "GET"
path = "/platform/accounts"
# Create the signature
signature = hmac.new(
key=bytes(api_secret, 'utf-8'),
msg=bytes(timestamp + method + path, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
# Headers for the API request
headers = {
'Authorization': f'ApiKey {api_key}',
'X-Signature': signature,
'X-Timestamp': timestamp
}
# Make the API request
response = requests.get(f'https://api.copper.co{path}', headers=headers)
# Check if the request was successful
if response.status_code == 200:
user_data = response.json()
print("User Data:", user_data)
else:
print("Failed to retrieve user data:", response.status_code, response.text)
Replace your_api_key
and your_api_secret
with your actual Copper API credentials. This script generates the necessary headers and makes a GET request to the Copper API to retrieve user data.
Verifying Successful API Requests in Copper Sandbox
After executing the script, verify the success of your API call by checking the response. A status code of 200 indicates success, and the user data should be printed in the console. If the request fails, the script will output the error code and message, helping you troubleshoot any issues.
Error Handling and Common Copper API Error Codes
When interacting with the Copper API, it's essential to handle potential errors gracefully. Here are some common error codes you might encounter:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
- 429 Too Many Requests: Rate limit exceeded. Copper API allows 30,000 requests per 5 minutes.
For more detailed information on error handling, refer to the Copper API documentation.
Best Practices for Secure and Efficient Copper API Integration
When integrating with the Copper API, it's crucial to follow best practices to ensure security and efficiency. Here are some recommendations:
- Secure Storage of API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Copper API allows up to 30,000 requests per 5 minutes. Implement rate limiting in your application to avoid exceeding this limit and encountering a 429 error.
- Data Standardization: Ensure that the data retrieved from the Copper API is standardized and transformed as needed for your application. This will help maintain consistency and improve data handling.
- Error Handling: Implement robust error handling to manage API errors gracefully. Use the error codes provided by Copper to troubleshoot and resolve issues effectively.
Leverage Endgrate for Streamlined Copper API Integrations
Integrating multiple APIs can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration that works across multiple platforms, reducing redundancy and maintenance efforts.
- Enhance Customer Experience: Provide an intuitive and seamless integration experience for your users, improving satisfaction and engagement.
Explore how Endgrate can help you streamline your Copper API integrations by visiting Endgrate's website.
Read More
Ready to get started?