Using the Customer.io App API to Get Customers in Python
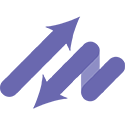
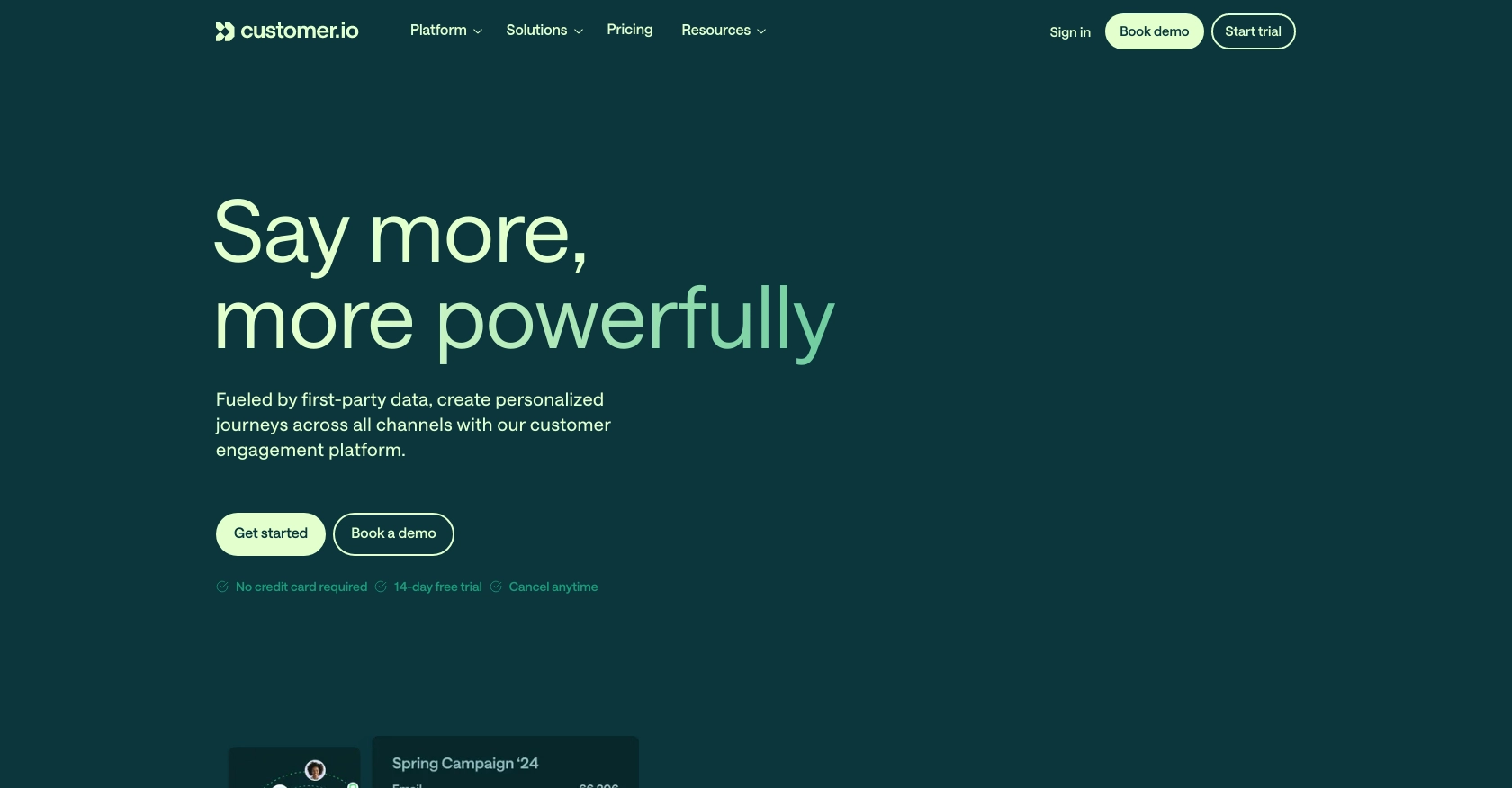
Introduction to Customer.io App API
Customer.io is a powerful platform designed to help businesses automate and personalize their customer interactions. It provides a suite of tools for sending targeted emails, SMS, and push notifications, allowing businesses to engage with their audience effectively.
Integrating with the Customer.io App API enables developers to access and manage customer data seamlessly. For example, you can retrieve customer information to tailor marketing campaigns or automate customer segmentation based on specific attributes. This integration can significantly enhance the personalization of customer communications, leading to improved engagement and conversion rates.
Setting Up Your Customer.io App Test Account
Create a Customer.io Account
To begin integrating with the Customer.io App API, you'll need to set up an account. If you don't already have one, visit the Customer.io website and sign up for a free trial or demo account. Follow the on-screen instructions to complete the registration process.
Generate Your Customer.io App API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps to obtain your API key:
- Log in to your Customer.io account.
- Navigate to the Account Settings section.
- Select API Keys from the menu.
- Click on Create API Key and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it for API requests.
Configure API Key Permissions
Ensure your API key has the necessary permissions to access customer data. You can configure the scope of your API key by selecting the appropriate permissions during the key creation process. This will allow you to perform actions such as retrieving customer information.
Test Your Setup
Before proceeding with API calls, it's crucial to test your setup to ensure everything is configured correctly. You can do this by making a simple API request to verify that your API key is working as expected.
import requests
# Set the API endpoint and headers
endpoint = "https://api.customer.io/v1/customers"
headers = {
"Authorization": "Bearer YOUR_API_KEY"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check the response status
if response.status_code == 200:
print("Setup successful!")
else:
print("Setup failed. Please check your API key and permissions.")
Replace YOUR_API_KEY
with the API key you generated earlier. Run the code to ensure you receive a successful response, confirming that your setup is complete.
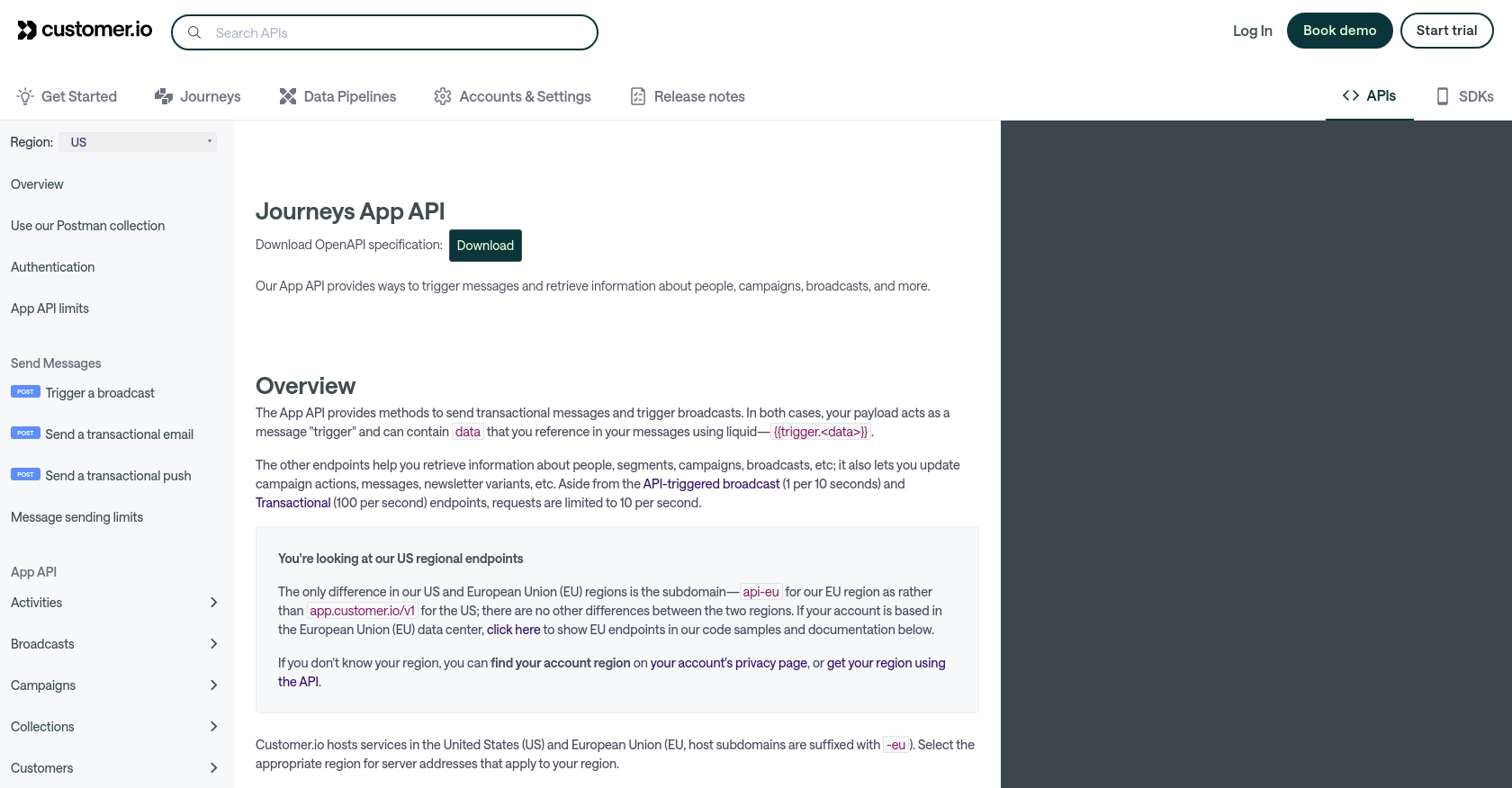
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data from Customer.io App
To interact with the Customer.io App API using Python, you'll need to ensure you have the correct environment set up. This includes having the necessary Python version and dependencies installed.
Python Environment Setup for Customer.io App API
Before making API calls, ensure you have Python 3.11.1 installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Example Code to Retrieve Customers from Customer.io App
Once your environment is ready, you can proceed to make API calls to retrieve customer data. Below is a sample Python script to get customers from the Customer.io App API:
import requests
# Set the API endpoint and headers
endpoint = "https://api.customer.io/v1/customers"
headers = {
"Authorization": "Bearer YOUR_API_KEY"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Parse the JSON data from the response
if response.status_code == 200:
data = response.json()
for customer in data["results"]:
print(customer)
else:
print(f"Failed to retrieve customers. Status code: {response.status_code}")
Replace YOUR_API_KEY
with your actual API key. This script sends a GET request to the Customer.io App API to fetch customer data. If successful, it prints the customer details.
Handling API Response and Errors
It's important to handle API responses and potential errors gracefully. The above script checks the response status code to ensure the request was successful. If the status code is not 200, it prints an error message.
For more detailed error handling, refer to the Customer.io API documentation to understand the possible error codes and their meanings.
Verifying API Call Success in Customer.io App
After running the script, verify the retrieved data by checking your Customer.io App account. Ensure that the data matches the expected output from the API call.
By following these steps, you can efficiently interact with the Customer.io App API to manage customer data, enhancing your application's capabilities.
Conclusion and Best Practices for Using Customer.io App API
Integrating with the Customer.io App API can significantly enhance your ability to manage and personalize customer interactions. By following the steps outlined in this guide, you can efficiently retrieve and utilize customer data to improve engagement and conversion rates.
Best Practices for Secure API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of the API rate limits, which are set at 10 requests per second. Implement retry logic to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
Enhancing Integration with Endgrate
For developers looking to streamline their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including Customer.io App.
Explore the benefits of using Endgrate for a seamless and intuitive integration experience. Visit Endgrate to learn more about how it can support your integration needs.
Read More
Ready to get started?