Using the Endear API to Get Tasks (with Javascript examples)
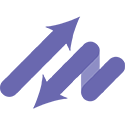
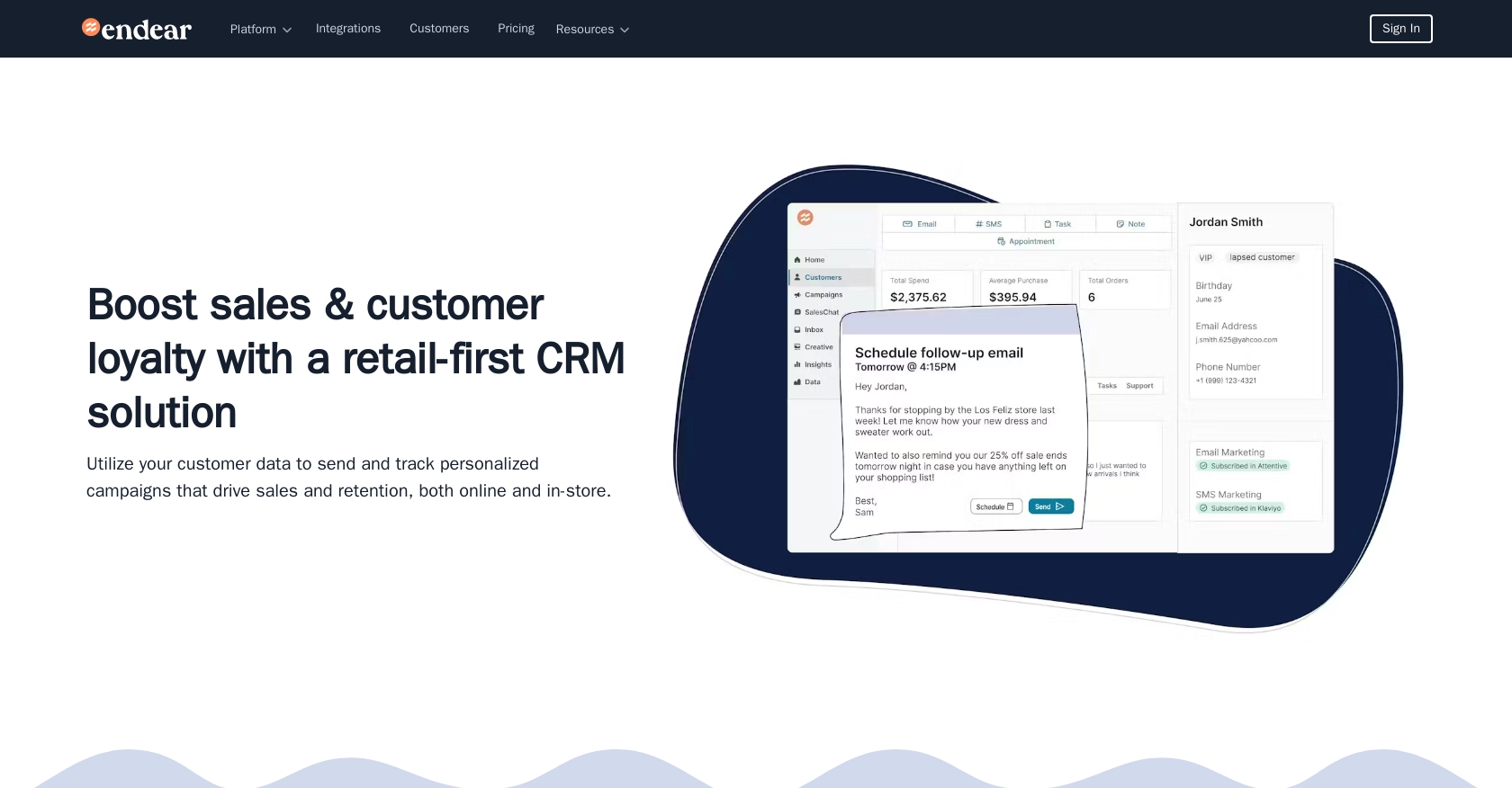
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationship management through seamless integration capabilities. It offers a robust API that allows developers to interact with various CRM functionalities, making it an ideal choice for businesses looking to streamline their customer engagement processes.
Integrating with Endear's API can empower developers to automate task management, such as retrieving and organizing tasks within the CRM. For example, a developer might use the Endear API to fetch tasks and display them in a custom dashboard, enabling teams to manage their workflows more efficiently.
Setting Up Your Endear Test Account and API Key
Before you can start interacting with the Endear API, you'll need to set up a test account and generate an API key. This key will allow you to authenticate your requests and access the API's functionalities.
Creating an Endear Integration and Generating an API Key
Follow these steps to create an integration and obtain your API key:
- Log in to your Endear account. If you don't have one, you can sign up for a free trial on the Endear website.
- Navigate to the Settings section in your Endear dashboard.
- Click on Integrations and then select Add Integration.
- Choose API from the options provided.
- Fill in the required details for your integration and submit the form.
- Once your integration is created, you will receive an API key. Make sure to store this key securely, as it will be used to authenticate your API requests.
Authenticating API Requests with Your API Key
To authenticate your requests to the Endear API, include your API key in the request headers. Here's how you can do it using a POST request:
// Example of authenticating a request to the Endear API
const endpoint = 'https://api.endearhq.com/graphql';
const headers = {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'Your_API_Key'
};
// Example POST request
fetch(endpoint, {
method: 'POST',
headers: headers,
body: JSON.stringify({
query: `query { currentIntegration { id } }`
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace Your_API_Key
with the API key you generated earlier. This setup will allow you to authenticate and interact with the Endear API seamlessly.
For more detailed information on authentication, refer to the Endear Authentication Documentation.
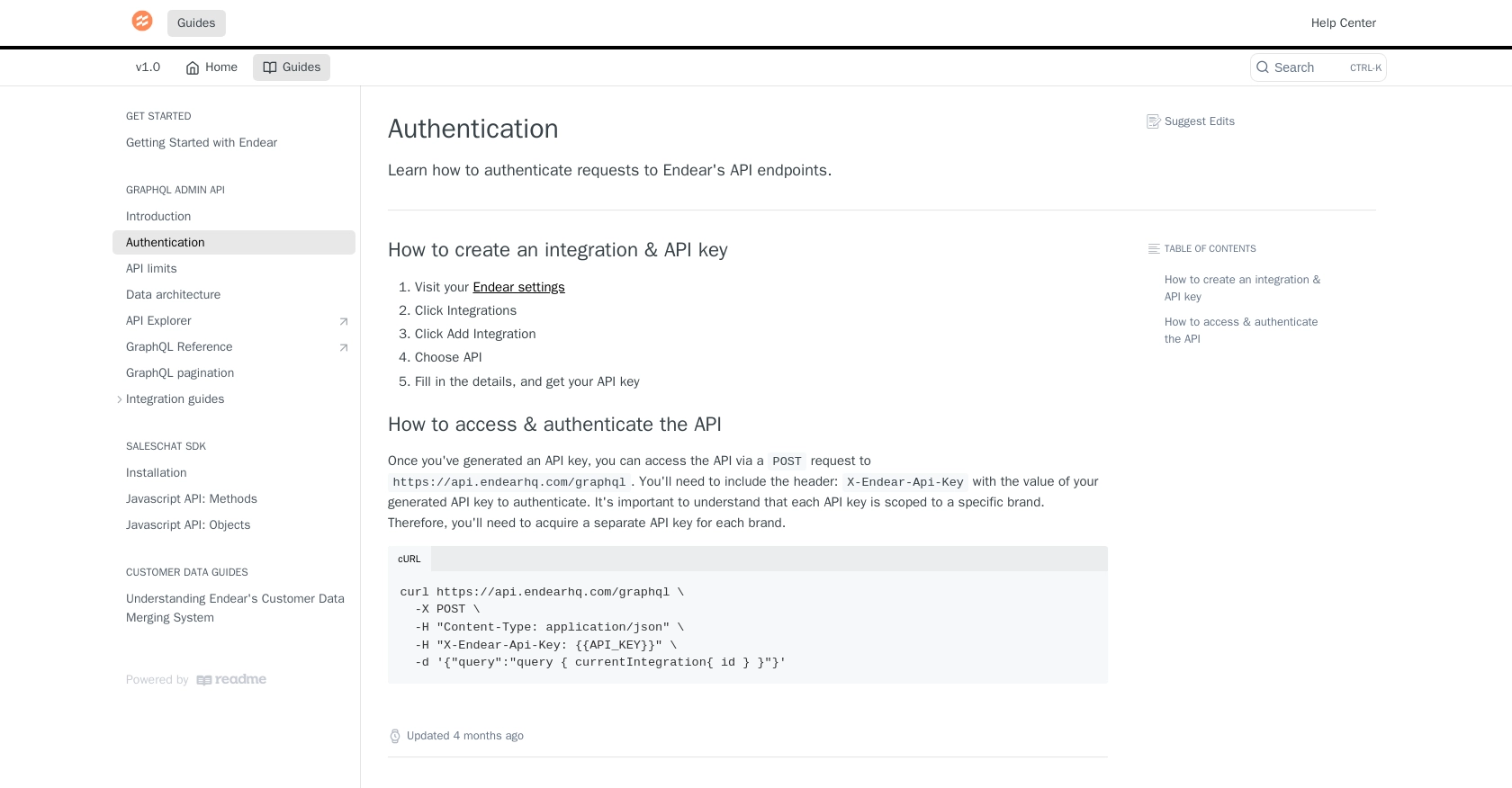
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Endear Using JavaScript
To interact with the Endear API and retrieve tasks, you'll need to use JavaScript to send a POST request to the API endpoint. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Endear API Integration
Before making API calls, ensure you have a modern JavaScript environment. You can use Node.js or any browser-based environment that supports the Fetch API. No additional dependencies are required for this example.
Writing JavaScript Code to Fetch Tasks from Endear API
Below is an example of how to write a JavaScript function to fetch tasks from the Endear API:
// Define the API endpoint and headers
const endpoint = 'https://api.endearhq.com/graphql';
const headers = {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'Your_API_Key'
};
// Define the GraphQL query to fetch tasks
const query = `
query Edges {
searchTasks {
edges {
node {
recurring
recurrence_rule
note {
title
description
}
deadline
timezone
id
}
}
pageInfo {
endCursor
hasNextPage
}
}
}`;
// Function to fetch tasks
function fetchTasks() {
fetch(endpoint, {
method: 'POST',
headers: headers,
body: JSON.stringify({ query: query })
})
.then(response => response.json())
.then(data => {
console.log('Tasks:', data);
// Handle the task data here
})
.catch(error => console.error('Error fetching tasks:', error));
}
// Call the function to fetch tasks
fetchTasks();
Replace Your_API_Key
with the API key you obtained from your Endear account. This code sends a POST request to the Endear API to retrieve tasks, then logs the response data to the console.
Verifying Successful API Requests and Handling Errors
After running the code, check the console for the output. You should see the tasks data returned from the API. If the request fails, the error will be logged to the console.
Endear's API employs rate limits of 120 requests per minute. If you exceed this limit, you'll receive an HTTP 429 error. To handle such errors, consider implementing a retry mechanism with exponential backoff.
For more information on error codes and rate limits, refer to the Endear Rate Limits and Errors Documentation.
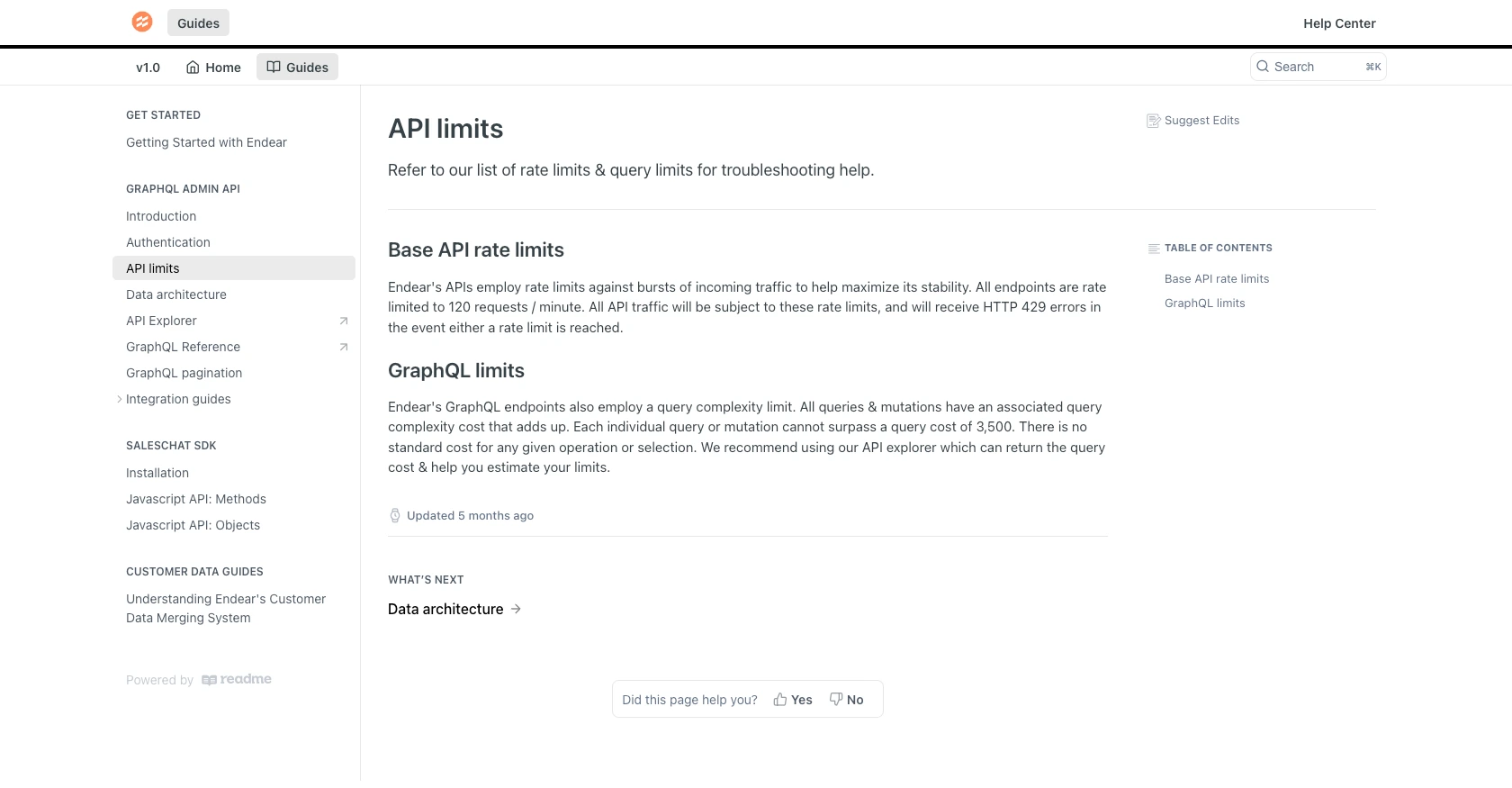
Best Practices for Using Endear API in JavaScript
When integrating with the Endear API, it's crucial to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Endear's API has a rate limit of 120 requests per minute. Implement a retry mechanism with exponential backoff to handle HTTP 429 errors gracefully.
- Optimize Data Handling: When fetching tasks, consider paginating results to manage large datasets efficiently. Use the
pageInfo
object in the response to navigate through pages. - Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application. This can help in maintaining a unified data structure.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Endear.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of integration.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing redundancy and maintenance efforts.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Task
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?