Using the Xero API to Get Tax Rates (with PHP examples)
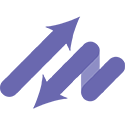
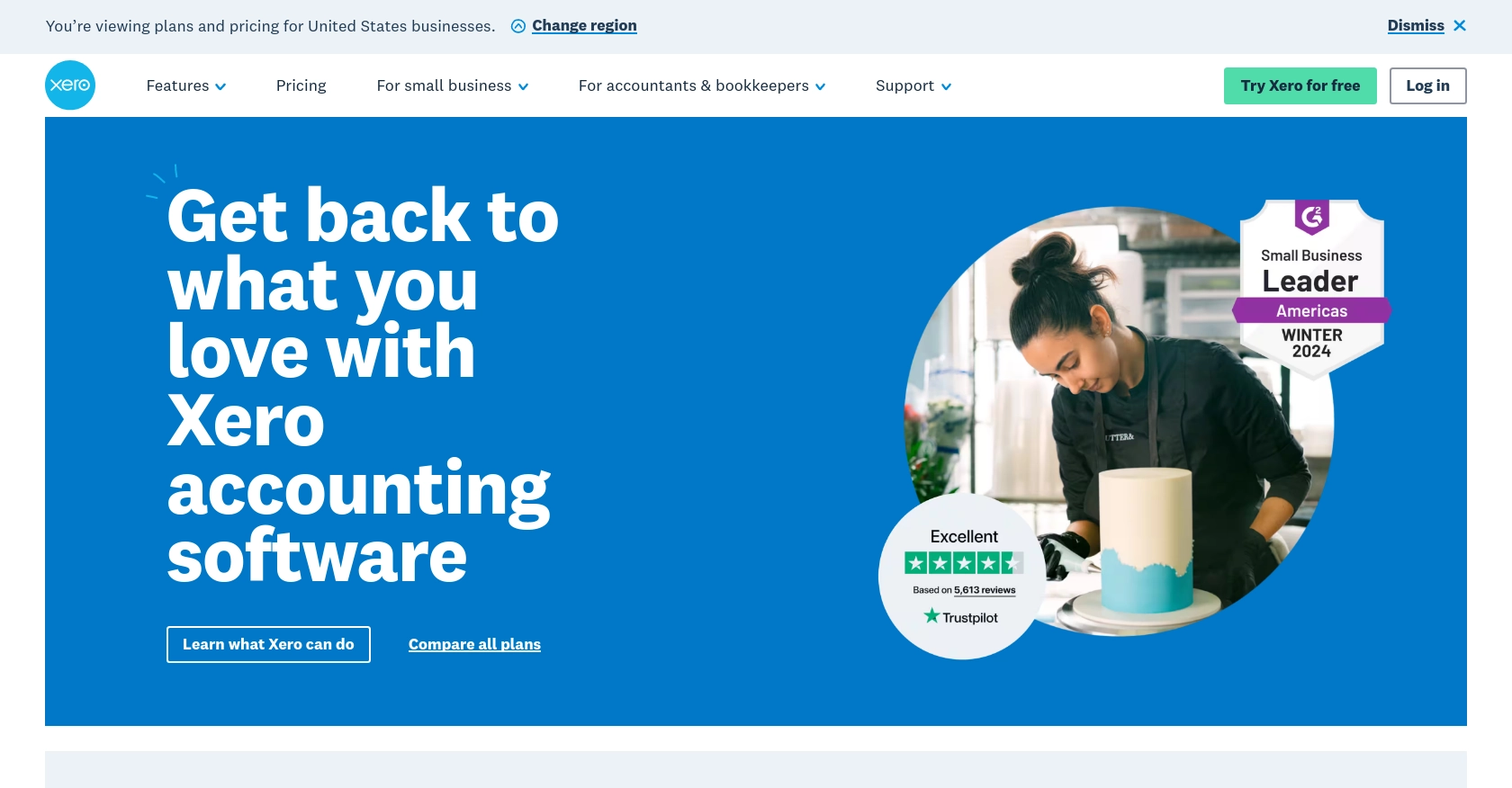
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software designed to help businesses manage their finances with ease. It offers a wide range of features, including invoicing, bank reconciliation, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Developers often integrate with Xero's API to streamline financial processes and enhance business operations. For example, accessing tax rates via the Xero API can automate tax calculations, ensuring accuracy and compliance in financial transactions.
This article will guide you through using PHP to interact with the Xero API, specifically focusing on retrieving tax rates. By the end of this tutorial, you'll be equipped to efficiently access and utilize tax rate data within your applications.
Setting Up Your Xero Developer Account and Sandbox Environment
Before you can start interacting with the Xero API to retrieve tax rates, you'll need to set up a Xero developer account and create a sandbox environment. This will allow you to test your API calls without affecting live data.
Creating a Xero Developer Account
To begin, you'll need to create a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal.
- Click on the "Sign Up" button and fill in the required information to create your account.
- Once registered, log in to your Xero developer account.
Setting Up a Xero Sandbox Company
Next, you'll need to set up a sandbox company to test your API interactions:
- Navigate to the "My Apps" section in your Xero developer dashboard.
- Click on "Create App" and provide the necessary details for your application.
- Once your app is created, you'll have access to a sandbox company where you can test API calls.
Configuring OAuth 2.0 Authentication for Xero API
The Xero API uses OAuth 2.0 for authentication. Follow these steps to configure it:
- In your Xero app settings, note down your client ID and client secret. These will be used for authentication.
- Set up the redirect URI as required by your application. This is where users will be redirected after authentication.
- Ensure you have the necessary scopes for accessing tax rates. Refer to the Xero OAuth 2.0 Scopes documentation for more details.
Generating Access Tokens
To interact with the Xero API, you'll need to generate access tokens:
- Use the standard OAuth 2.0 authorization code flow to obtain an access token. Detailed steps can be found in the Xero OAuth 2.0 Authorization Flow documentation.
- Store the access token securely, as it will be required for making API calls.
With your Xero developer account, sandbox company, and OAuth 2.0 authentication set up, you're now ready to start making API calls to retrieve tax rates using PHP.
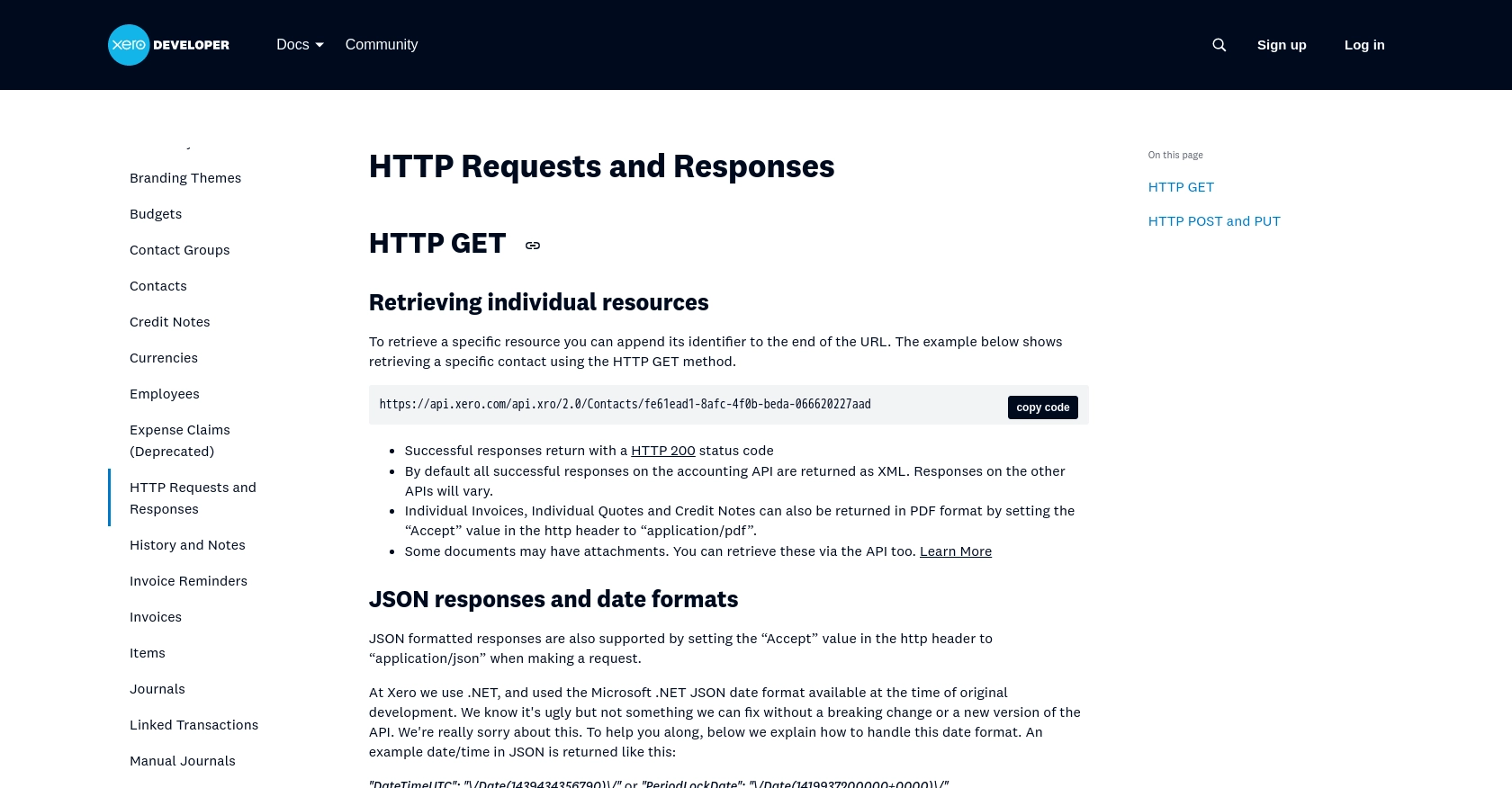
sbb-itb-96038d7
Making API Calls to Retrieve Xero Tax Rates Using PHP
With your Xero developer account and OAuth 2.0 authentication configured, you can now proceed to make API calls to retrieve tax rates. This section will guide you through setting up your PHP environment and executing the necessary API requests.
Setting Up Your PHP Environment for Xero API Integration
Before making API calls, ensure your PHP environment is correctly set up:
- Ensure you have PHP 7.4 or later installed on your machine.
- Install the required dependencies using Composer. You will need the
guzzlehttp/guzzle
package for making HTTP requests. Run the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Tax Rates from Xero API
Now, let's write the PHP code to interact with the Xero API and retrieve tax rates:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
$tenantId = 'Your_Tenant_ID'; // Replace with your actual tenant ID
$response = $client->request('GET', 'https://api.xero.com/api.xro/2.0/TaxRates', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Xero-tenant-id' => $tenantId,
'Accept' => 'application/json',
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['TaxRates'] as $taxRate) {
echo 'Name: ' . $taxRate['Name'] . '<br>';
echo 'Rate: ' . $taxRate['EffectiveRate'] . '%<br><br>';
}
?>
Replace Your_Access_Token
and Your_Tenant_ID
with your actual access token and tenant ID obtained during the OAuth 2.0 authentication setup.
Running the PHP Script and Verifying the Output
To execute the script, run the following command in your terminal:
php get_tax_rates.php
You should see a list of tax rates displayed, including their names and effective rates. This confirms that your API call was successful.
Handling Errors and Understanding Xero API Error Codes
When making API calls, it's crucial to handle potential errors. The Xero API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or access token is invalid.
- 403 Forbidden: The request is understood, but it has been refused.
- 429 Too Many Requests: Rate limit exceeded. Refer to the Xero API Rate Limits documentation for more details.
Implement error handling in your code to manage these responses effectively and ensure a robust integration.
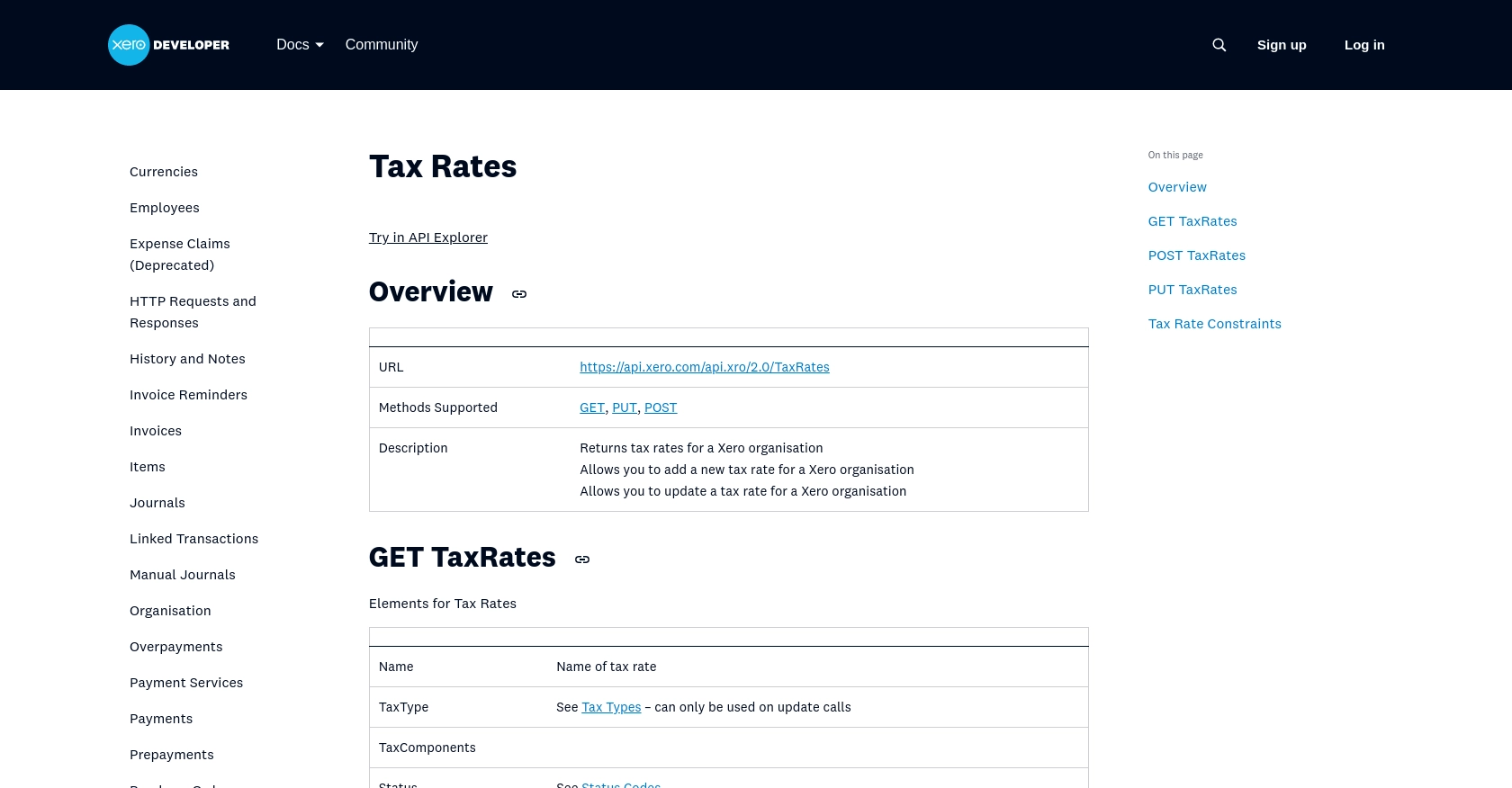
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API to retrieve tax rates using PHP can significantly enhance your application's financial management capabilities. By automating tax calculations, you ensure accuracy and compliance, streamlining your business operations.
Here are some best practices to consider when working with the Xero API:
- Secure Storage of Credentials: Always store your access tokens and client secrets securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of Xero's API rate limits to avoid disruptions. Implement retry logic with exponential backoff to manage requests efficiently. Refer to the Xero API Rate Limits documentation for more details.
- Data Transformation and Standardization: Ensure that the tax rate data retrieved from Xero is transformed and standardized to fit your application's data structures and requirements.
- Error Handling: Implement robust error handling to manage API responses effectively. Log errors and provide meaningful feedback to users when issues arise.
By following these best practices, you can create a reliable and efficient integration with the Xero API, enhancing your application's functionality and user experience.
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/taxrates
Ready to get started?