Using the FreshDesk API to Create or Update Companies in Javascript
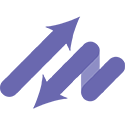
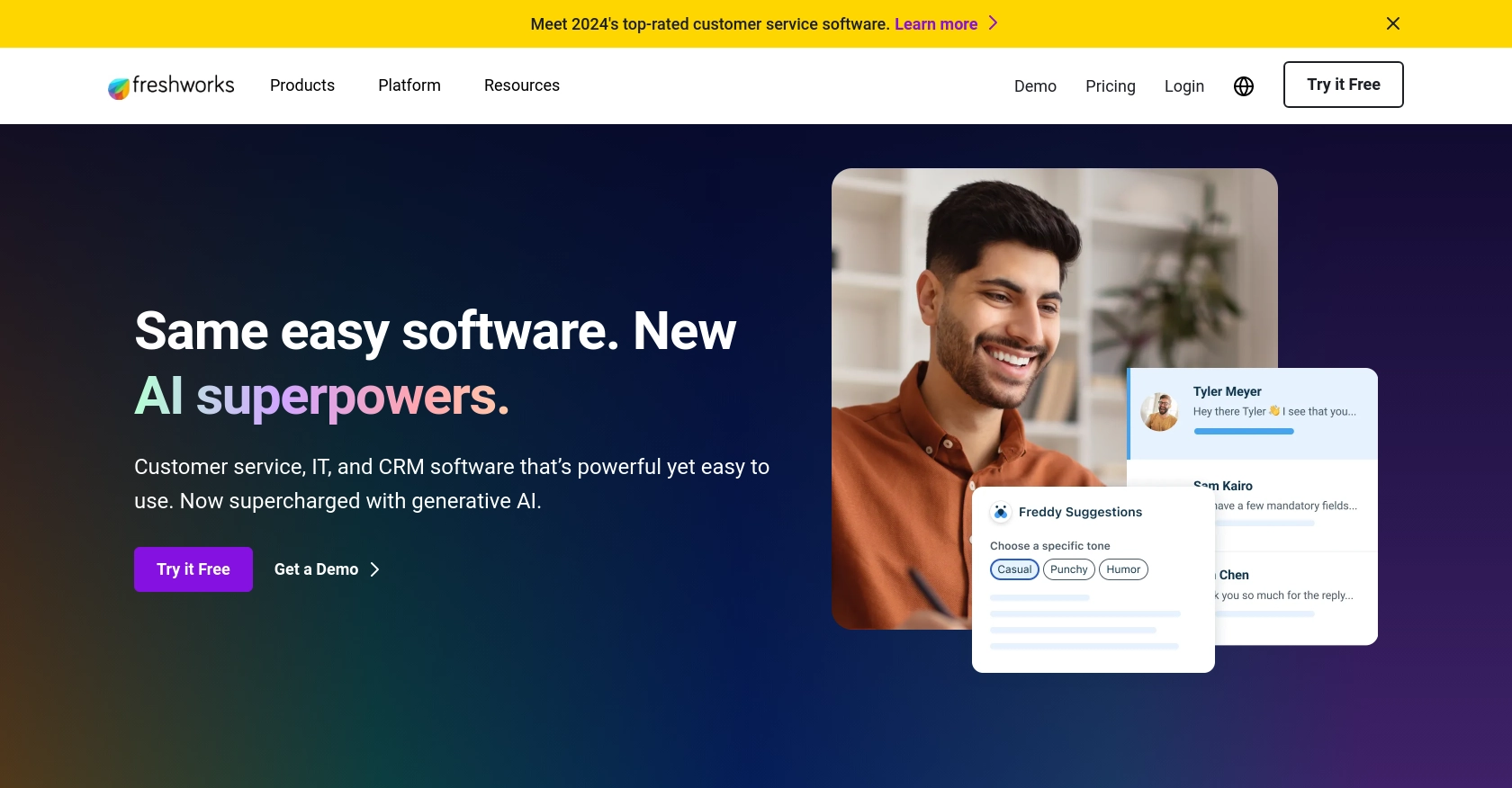
Introduction to FreshDesk API Integration
FreshDesk is a robust customer support software that empowers businesses to deliver exceptional customer service. With features like ticketing, automation, and reporting, FreshDesk streamlines support operations and enhances customer satisfaction.
Integrating with the FreshDesk API allows developers to automate and manage customer support data efficiently. For example, you can use the FreshDesk API to create or update company records, ensuring that your support team has the most up-to-date information about clients and their interactions.
This article will guide you through using JavaScript to interact with the FreshDesk API, focusing on creating or updating company records. By following this tutorial, you can enhance your application's ability to manage customer data seamlessly.
Setting Up Your FreshDesk Test or Sandbox Account
Before you can start integrating with the FreshDesk API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data.
Creating a FreshDesk Account
If you don't already have a FreshDesk account, you can sign up for a free trial on the FreshDesk website. This trial will give you access to the necessary features to test API integrations.
- Visit the FreshDesk signup page.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating API Key for FreshDesk Authentication
FreshDesk uses a custom authentication method that involves generating an API key. This key will be used to authenticate your API requests.
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile icon in the top right corner.
- Select Profile Settings from the dropdown menu.
- In the API Key section, click on Show API Key to reveal your key.
- Copy the API key and store it securely, as you will need it for making API requests.
Configuring Your FreshDesk App for API Access
To interact with the FreshDesk API, you may need to configure certain settings in your FreshDesk app.
- Ensure that your account has the necessary permissions to access and modify company records.
- Review the API documentation at FreshDesk API Documentation to understand the required scopes and permissions.
With your FreshDesk account set up and your API key ready, you're now prepared to start making API calls to create or update company records using JavaScript.
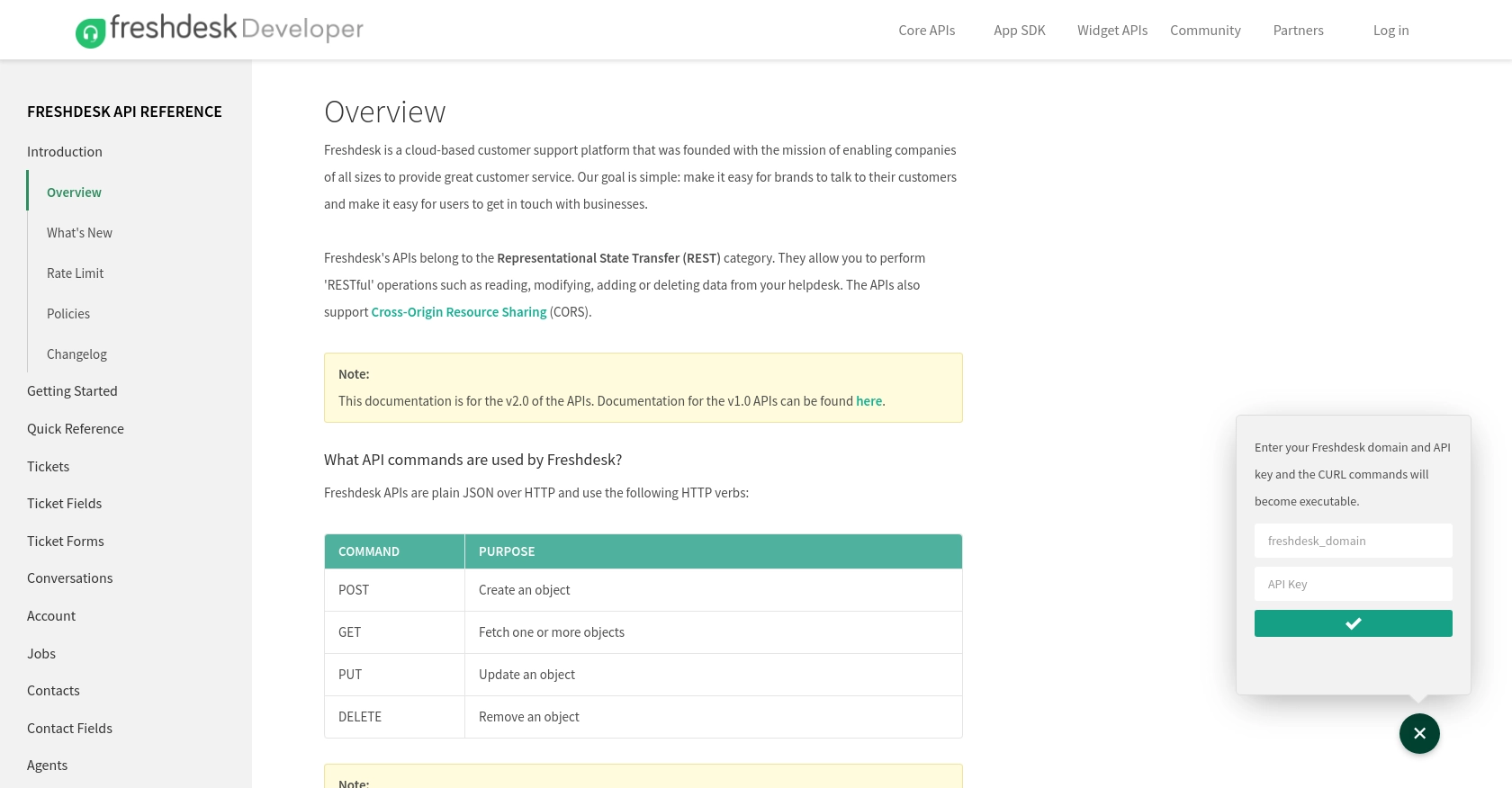
sbb-itb-96038d7
Making API Calls to FreshDesk Using JavaScript
To interact with the FreshDesk API for creating or updating company records, you'll need to use JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for FreshDesk API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A text editor or IDE, such as Visual Studio Code, to write your JavaScript code.
- Access to your FreshDesk API key, which you generated earlier.
Installing Required Dependencies for FreshDesk API Integration
You'll need the axios
library to make HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Create or Update Companies in FreshDesk
Create a new JavaScript file, freshdesk_companies.js
, and add the following code:
const axios = require('axios');
// Your FreshDesk API key
const apiKey = 'Your_API_Key';
// Base64 encode the API key for authentication
const auth = Buffer.from(apiKey + ':X').toString('base64');
// Function to create or update a company
async function createOrUpdateCompany(companyData) {
try {
const response = await axios({
method: 'post', // Use 'put' for updating
url: 'https://yourdomain.freshdesk.com/api/v2/companies',
headers: {
'Authorization': `Basic ${auth}`,
'Content-Type': 'application/json'
},
data: companyData
});
console.log('Company created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating company:', error.response ? error.response.data : error.message);
}
}
// Example company data
const companyData = {
name: 'Example Company',
description: 'A sample company for demonstration purposes'
};
// Call the function
createOrUpdateCompany(companyData);
Replace Your_API_Key
with your actual FreshDesk API key and yourdomain
with your FreshDesk domain.
Running the JavaScript Code and Verifying FreshDesk API Call Success
Run the script using Node.js:
node freshdesk_companies.js
If successful, you should see a confirmation message in the console. Verify the creation or update by checking the company records in your FreshDesk account.
Handling Errors and Troubleshooting FreshDesk API Calls
When making API calls, you may encounter errors. Common issues include:
- Authentication Errors: Ensure your API key is correct and properly encoded.
- Rate Limiting: FreshDesk may limit the number of requests. Refer to the FreshDesk API Documentation for rate limit details.
- Validation Errors: Check the structure of your request data to ensure it meets FreshDesk's requirements.
By following these steps, you can efficiently create or update company records in FreshDesk using JavaScript.
Conclusion and Best Practices for FreshDesk API Integration Using JavaScript
Integrating with the FreshDesk API to create or update company records using JavaScript can significantly enhance your application's ability to manage customer support data. By following the steps outlined in this guide, you can ensure a smooth and efficient integration process.
Best Practices for Secure and Efficient FreshDesk API Usage
- Secure API Key Storage: Always store your FreshDesk API key securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of FreshDesk's rate limits to avoid service disruptions. Implement retry logic and exponential backoff strategies to manage rate limits effectively.
- Data Validation and Error Handling: Validate your request data to ensure it meets FreshDesk's requirements. Implement robust error handling to manage potential API errors gracefully.
- Regularly Review API Documentation: Stay updated with FreshDesk's API documentation to leverage new features and understand any changes in API behavior.
Enhance Your Integration Strategy with Endgrate
While integrating with FreshDesk is a powerful way to manage customer support data, handling multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including FreshDesk.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?