Using the Chargebee API to Get Subscriptions in PHP
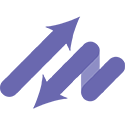
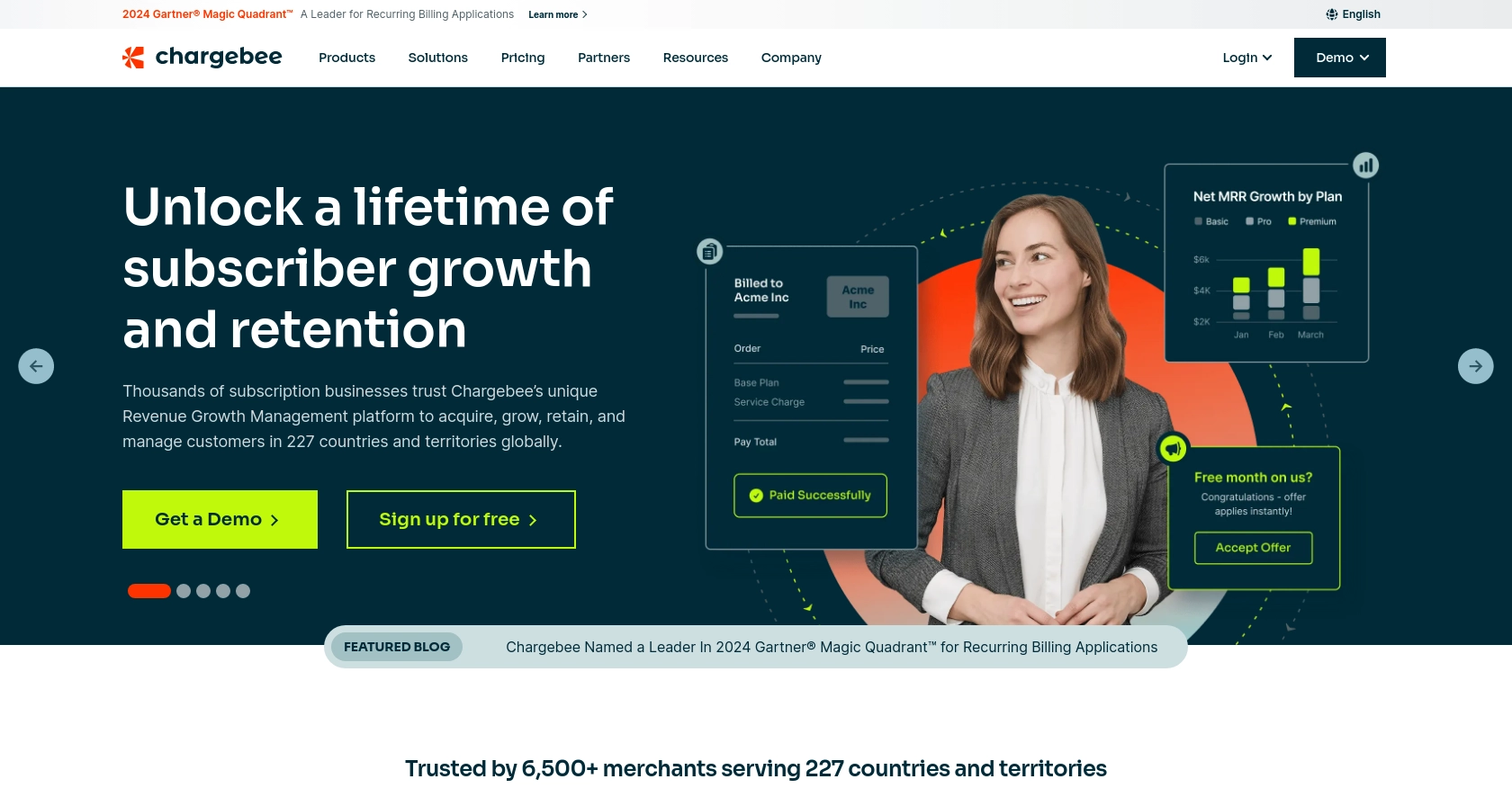
Introduction to Chargebee and Its Subscription Management API
Chargebee is a robust subscription management platform that simplifies billing and revenue operations for businesses of all sizes. It offers a comprehensive suite of tools to manage subscriptions, invoicing, and payments, making it an ideal choice for SaaS companies looking to streamline their billing processes.
Developers may want to integrate with Chargebee's API to automate subscription management tasks, such as retrieving subscription details for analytics or customer service purposes. For example, using the Chargebee API, a developer can fetch a list of active subscriptions to analyze customer engagement and billing cycles.
This article will guide you through using PHP to interact with Chargebee's API, specifically focusing on retrieving subscription data. By the end of this tutorial, you will be equipped to efficiently access and manage subscription information within the Chargebee platform using PHP.
Setting Up Your Chargebee Test Account
Before you can start using the Chargebee API, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting any live data. Chargebee provides a sandbox environment specifically for this purpose.
Creating a Chargebee Sandbox Account
To begin, you'll need to create a Chargebee sandbox account. Follow these steps:
- Visit the Chargebee signup page and register for a free sandbox account.
- Fill in the required details, such as your name, email, and company information.
- Once registered, you'll receive a confirmation email. Follow the instructions in the email to activate your account.
- Log in to your Chargebee dashboard using the credentials you set up during registration.
Generating API Keys for Chargebee
After setting up your sandbox account, you'll need to generate API keys to authenticate your requests. Chargebee uses HTTP Basic authentication, where the username is your API key and the password is left blank.
- Navigate to the Chargebee dashboard and click on your profile icon in the top right corner.
- Select API & Webhooks from the dropdown menu.
- Under the API Keys section, click on Create a Key.
- Provide a name for your API key and select the appropriate permissions.
- Click Create to generate the API key. Make sure to copy and store it securely, as you will need it to authenticate your API requests.
Configuring OAuth for Chargebee API Access
Chargebee supports OAuth for secure API access. To configure OAuth, follow these steps:
- In the Chargebee dashboard, go to Settings and select API & Webhooks.
- Click on OAuth Apps and then Create an OAuth App.
- Fill in the necessary details, such as the app name, redirect URL, and scopes required for your application.
- Once configured, you'll receive a client ID and client secret. Keep these credentials secure, as they are essential for OAuth authentication.
With your sandbox account set up and API keys generated, you're now ready to start integrating with the Chargebee API using PHP. In the next section, we'll cover how to make API calls to retrieve subscription data.
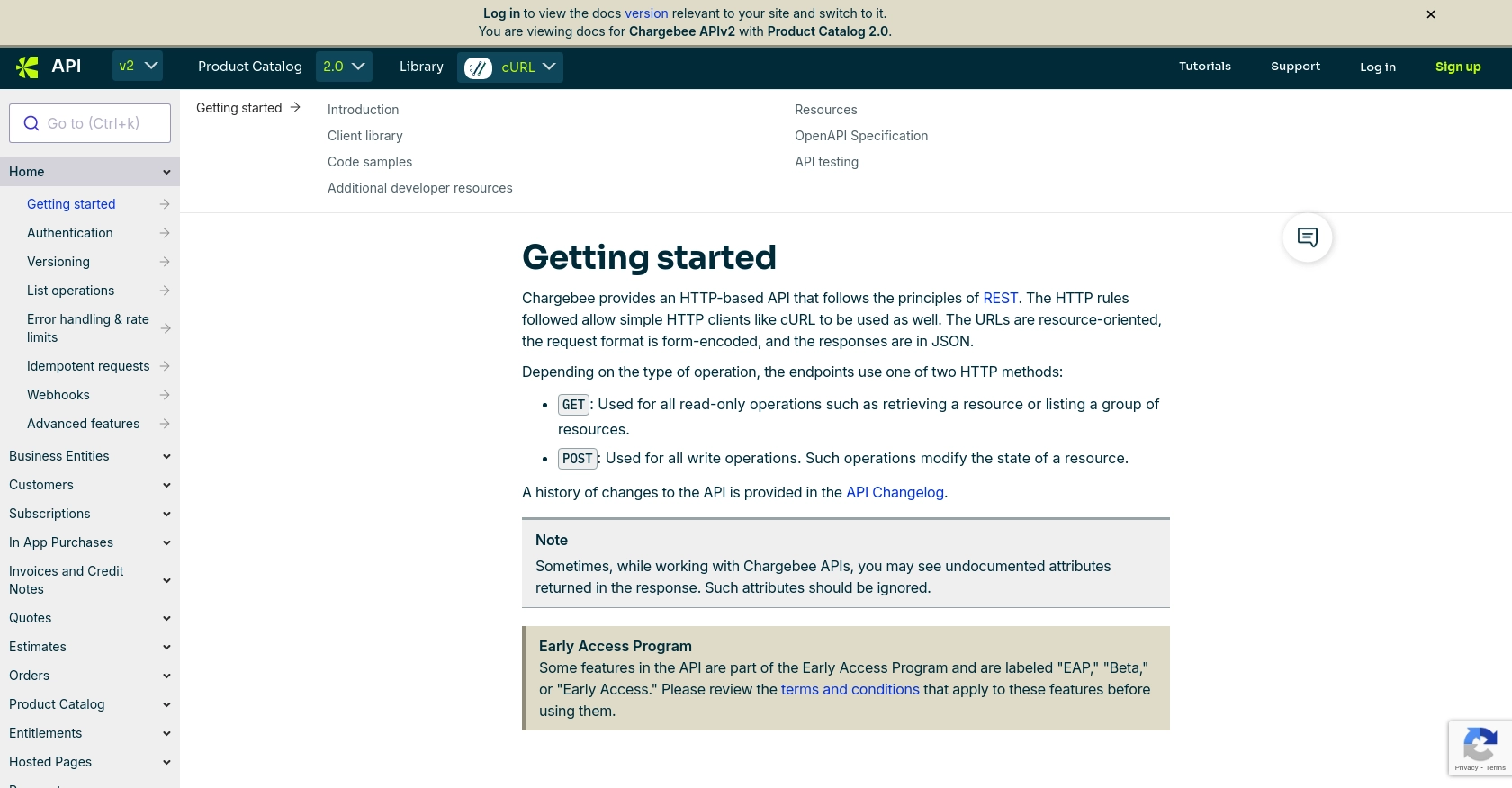
sbb-itb-96038d7
Making API Calls to Retrieve Chargebee Subscriptions Using PHP
To interact with Chargebee's API and retrieve subscription data, you'll need to use PHP to send HTTP requests. This section will guide you through setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for Chargebee API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled. Follow these steps to set up your environment:
- Verify your PHP version by running
php -v
in your terminal. Ensure it's 7.4 or higher. - Check if the cURL extension is enabled by running
php -m
and looking for "curl" in the list of modules. - If cURL is not enabled, modify your
php.ini
file to include the lineextension=curl
and restart your web server.
Installing Required PHP Dependencies for Chargebee API
To simplify HTTP requests, install the Guzzle HTTP client, a popular PHP library. Use Composer, the PHP package manager, to install it:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Subscriptions from Chargebee
With your environment set up, you can now write PHP code to interact with Chargebee's API. Below is a sample script to retrieve a list of subscriptions:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle HTTP client
$client = new Client();
// Define the Chargebee API endpoint and your site-specific URL
$endpoint = 'https://{your-site}.chargebee.com/api/v2/subscriptions';
// Set up the API key for authentication
$apiKey = 'your_api_key_here';
// Make a GET request to the Chargebee API
$response = $client->request('GET', $endpoint, [
'auth' => [$apiKey, ''],
'query' => ['limit' => 10] // Limit the number of subscriptions retrieved
]);
// Parse the JSON response
$data = json_decode($response->getBody(), true);
// Display the subscription data
foreach ($data['list'] as $subscription) {
echo "Subscription ID: " . $subscription['subscription']['id'] . "\n";
echo "Status: " . $subscription['subscription']['status'] . "\n";
echo "Customer ID: " . $subscription['subscription']['customer_id'] . "\n\n";
}
Handling API Responses and Errors in Chargebee
After making an API call, it's crucial to handle responses and potential errors. Chargebee uses standard HTTP status codes to indicate success or failure:
- 2XX: Success. The API call was successful.
- 4XX: Client error. Check your request parameters or authentication.
- 5XX: Server error. Retry the request later.
In the sample code, the response is parsed using json_decode()
, and the subscription details are printed. Ensure you handle exceptions and errors gracefully to improve user experience.
Verifying Chargebee API Requests in the Sandbox Environment
To verify that your API requests are successful, log in to your Chargebee sandbox account and navigate to the Subscriptions section. You should see the subscriptions retrieved by your PHP script. If there are discrepancies, check your API key and endpoint URL.
By following these steps, you can efficiently retrieve subscription data from Chargebee using PHP, enabling you to automate and streamline your subscription management processes.
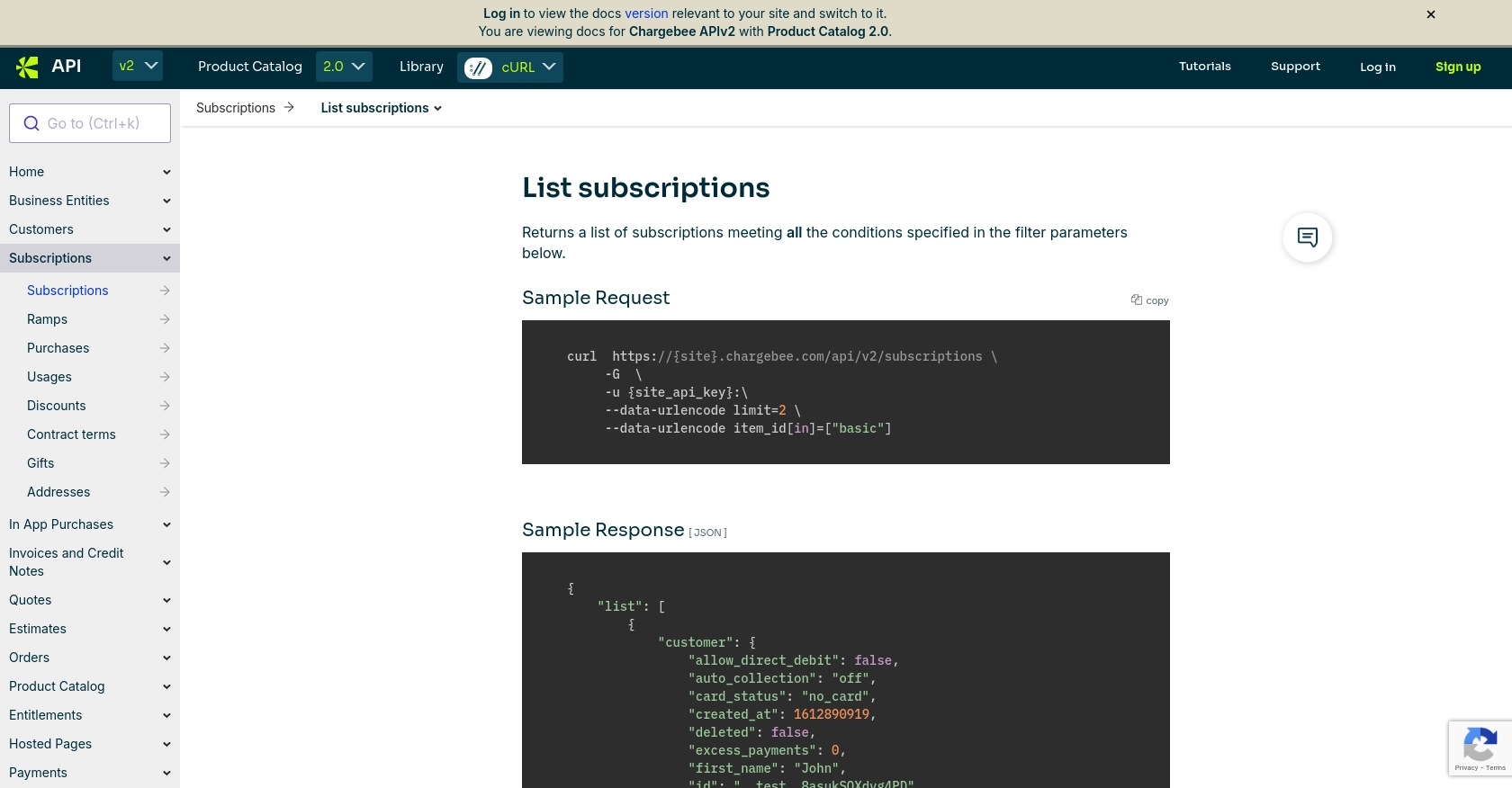
Best Practices for Chargebee API Integration in PHP
When integrating with Chargebee's API using PHP, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Chargebee imposes rate limits on API requests. Ensure your application respects these limits to avoid disruptions. Implement exponential backoff strategies for retries.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application. This will help in maintaining data integrity and ease of integration with other systems.
- Error Handling: Implement robust error handling to manage API response errors gracefully. Use Chargebee's error codes to provide meaningful feedback to users and log errors for debugging.
Streamlining Subscription Management with Endgrate
While integrating with Chargebee's API can significantly enhance your subscription management capabilities, it can also be time-consuming and complex. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API that simplifies the integration process, allowing you to connect with multiple platforms, including Chargebee, through a single endpoint. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop integration logic once and apply it across various platforms, reducing redundancy and maintenance efforts.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience, boosting satisfaction and retention.
To learn more about how Endgrate can streamline your integration processes, visit Endgrate's website.
Conclusion: Mastering Chargebee API Integration with PHP
Integrating with Chargebee's API using PHP empowers developers to automate and optimize subscription management tasks, enhancing business operations and customer engagement. By following the steps outlined in this guide, you can efficiently retrieve and manage subscription data, ensuring a smooth and effective integration process.
Remember to adhere to best practices for security and efficiency, and consider leveraging tools like Endgrate to further simplify and enhance your integration efforts. With the right approach, you can unlock the full potential of Chargebee's robust subscription management capabilities.
Read More
Ready to get started?