How to Create Calendar Events with the Google Calendar API in Python
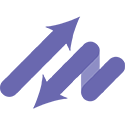
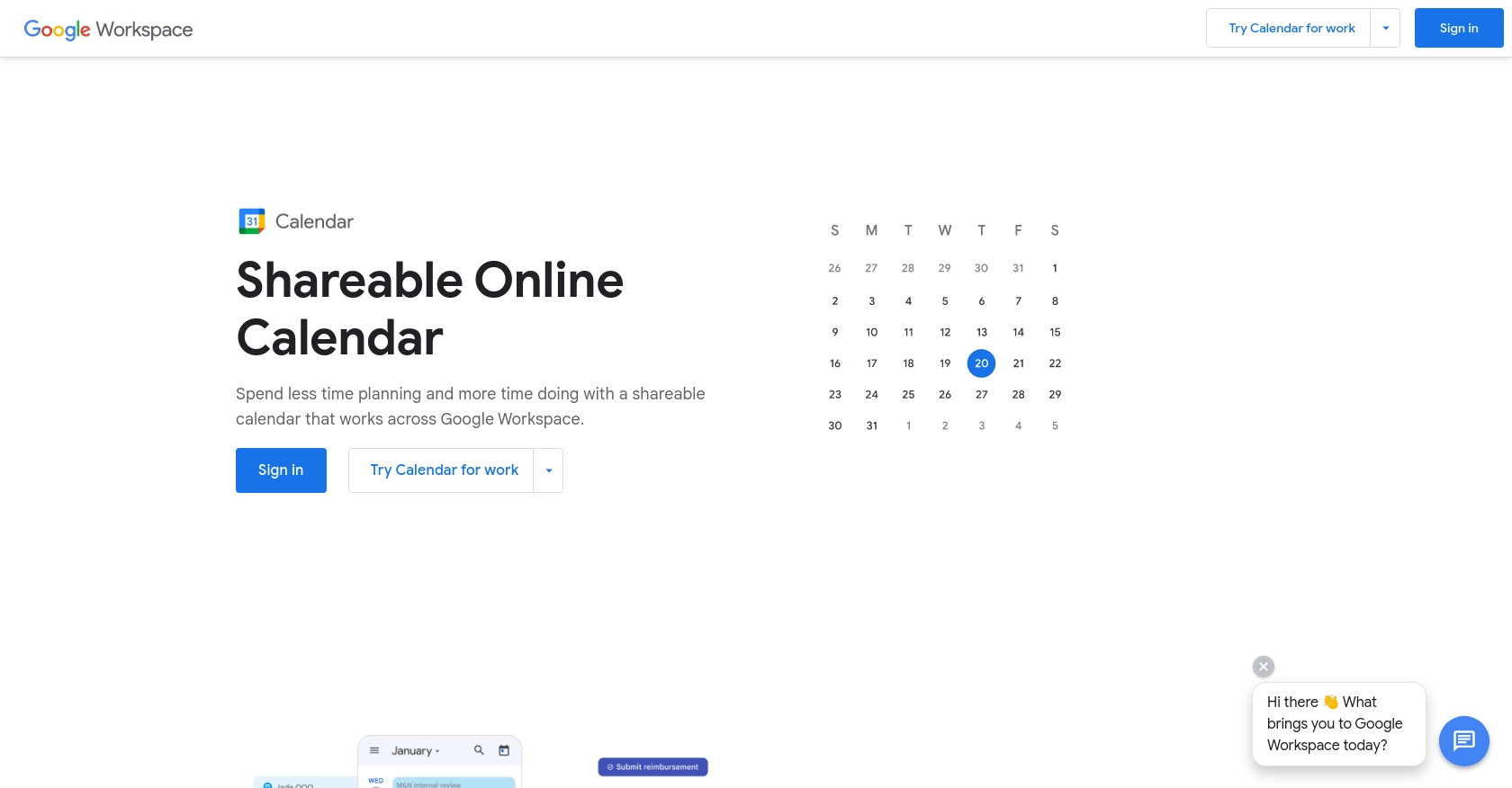
Introduction to Google Calendar API
Google Calendar is a widely used time-management and scheduling service that allows users to create and edit events. Its seamless integration with other Google services and user-friendly interface make it a popular choice for both personal and professional use.
Developers often integrate with the Google Calendar API to automate event management, streamline scheduling, and enhance productivity. For example, a developer might use the API to automatically schedule meetings based on availability, send reminders, or sync events across multiple platforms.
This article will guide you through creating calendar events using the Google Calendar API in Python, providing step-by-step instructions to help you efficiently manage your scheduling needs.
Setting Up Your Google Calendar API Test Account
Before you can start creating calendar events with the Google Calendar API in Python, you'll need to set up a Google Cloud project and configure OAuth 2.0 for authentication. This process involves creating a project in the Google Cloud Console, enabling the Google Calendar API, and setting up the necessary credentials.
Create a Google Cloud Project
- Navigate to the Google Cloud Console.
- Click on the Menu icon, then select IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create. Your project will be created within a few minutes.
Enable Google Calendar API
- In the Google Cloud Console, go to APIs & Services > Library.
- Search for "Google Calendar API" and click on it.
- Click the Enable button to activate the API for your project.
Configure OAuth Consent Screen
- In the Google Cloud Console, go to APIs & Services > OAuth consent screen.
- Select the user type for your application and click Create.
- Fill out the required fields, such as application name and support email, then click Save and Continue.
Create OAuth 2.0 Credentials
- Go to APIs & Services > Credentials in the Google Cloud Console.
- Click Create Credentials and select OAuth client ID.
- Choose Desktop app as the application type and click Create.
- Note down the Client ID and Client Secret as you will need them for authentication.
With these steps completed, you have successfully set up your Google Calendar API test account. You are now ready to authenticate and make API calls to create calendar events using Python.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
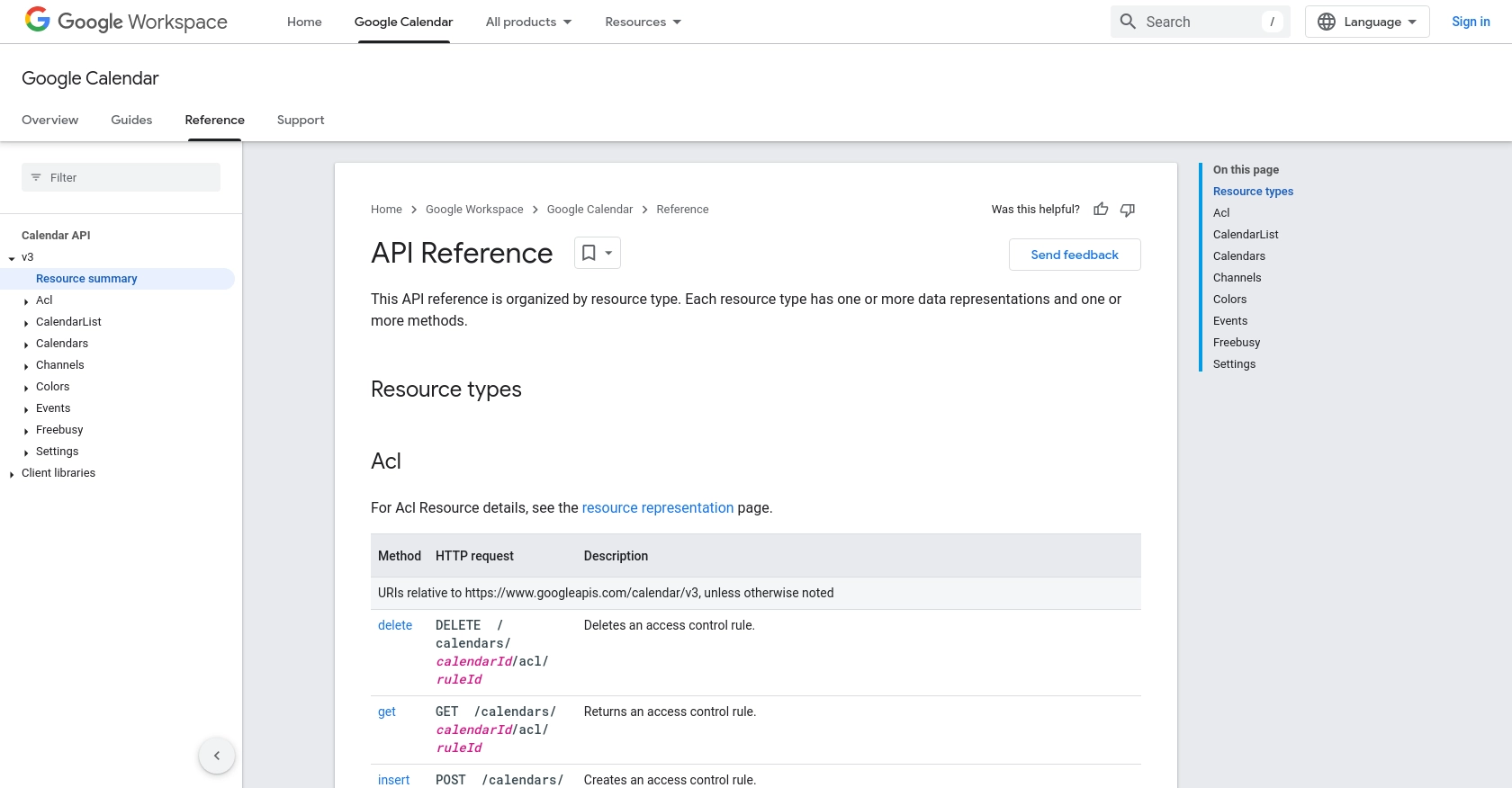
sbb-itb-96038d7
Making API Calls to Create Calendar Events with Google Calendar API in Python
To create calendar events using the Google Calendar API in Python, you'll need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up Python, installing dependencies, and writing the code to create events.
Setting Up Your Python Environment
Before making API calls, ensure you have Python 3.11.1 installed on your machine. You will also need the Python package installer, pip
, to manage your dependencies.
- Verify your Python installation by running the following command in your terminal or command prompt:
python3 --version
- If Python is not installed, download and install it from the official Python website.
- Install the Google client library using
pip
:
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
Writing Python Code to Create Google Calendar Events
With your environment set up, you can now write the Python code to create events in Google Calendar. Follow these steps:
- Create a new Python file named
create_calendar_event.py
. - Import the necessary libraries and set up authentication:
from __future__ import print_function import datetime import os.path from google.oauth2.credentials import Credentials from google_auth_oauthlib.flow import InstalledAppFlow from google.auth.transport.requests import Request from googleapiclient.discovery import build # If modifying these SCOPES, delete the file token.json. SCOPES = ['https://www.googleapis.com/auth/calendar'] def main(): creds = None # The file token.json stores the user's access and refresh tokens, and is # created automatically when the authorization flow completes for the first # time. if os.path.exists('token.json'): creds = Credentials.from_authorized_user_file('token.json', SCOPES) # If there are no (valid) credentials available, let the user log in. if not creds or not creds.valid: if creds and creds.expired and creds.refresh_token: creds.refresh(Request()) else: flow = InstalledAppFlow.from_client_secrets_file( 'credentials.json', SCOPES) creds = flow.run_local_server(port=0) # Save the credentials for the next run with open('token.json', 'w') as token: token.write(creds.to_json()) service = build('calendar', 'v3', credentials=creds) # Call the Calendar API event = { 'summary': 'Google I/O 2023', 'location': '800 Howard St., San Francisco, CA 94103', 'description': 'A chance to hear more about Google\'s developer products.', 'start': { 'dateTime': '2023-05-28T09:00:00-07:00', 'timeZone': 'America/Los_Angeles', }, 'end': { 'dateTime': '2023-05-28T17:00:00-07:00', 'timeZone': 'America/Los_Angeles', }, 'recurrence': [ 'RRULE:FREQ=DAILY;COUNT=2' ], 'attendees': [ {'email': 'lpage@example.com'}, {'email': 'sbrin@example.com'}, ], 'reminders': { 'useDefault': False, 'overrides': [ {'method': 'email', 'minutes': 24 * 60}, {'method': 'popup', 'minutes': 10}, ], }, } event = service.events().insert(calendarId='primary', body=event).execute() print('Event created: %s' % (event.get('htmlLink'))) if __name__ == '__main__': main()
Running Your Python Script
Execute your script to create a calendar event:
python3 create_calendar_event.py
Upon successful execution, you should see a message with a link to the newly created event in your Google Calendar.
Handling Errors and Verifying Success
To ensure your request was successful, check the response from the API call. If the event is created, the response will include a link to the event. Handle potential errors by checking for exceptions and reviewing error messages. Refer to the Google Calendar API documentation for more details on error handling and response codes.
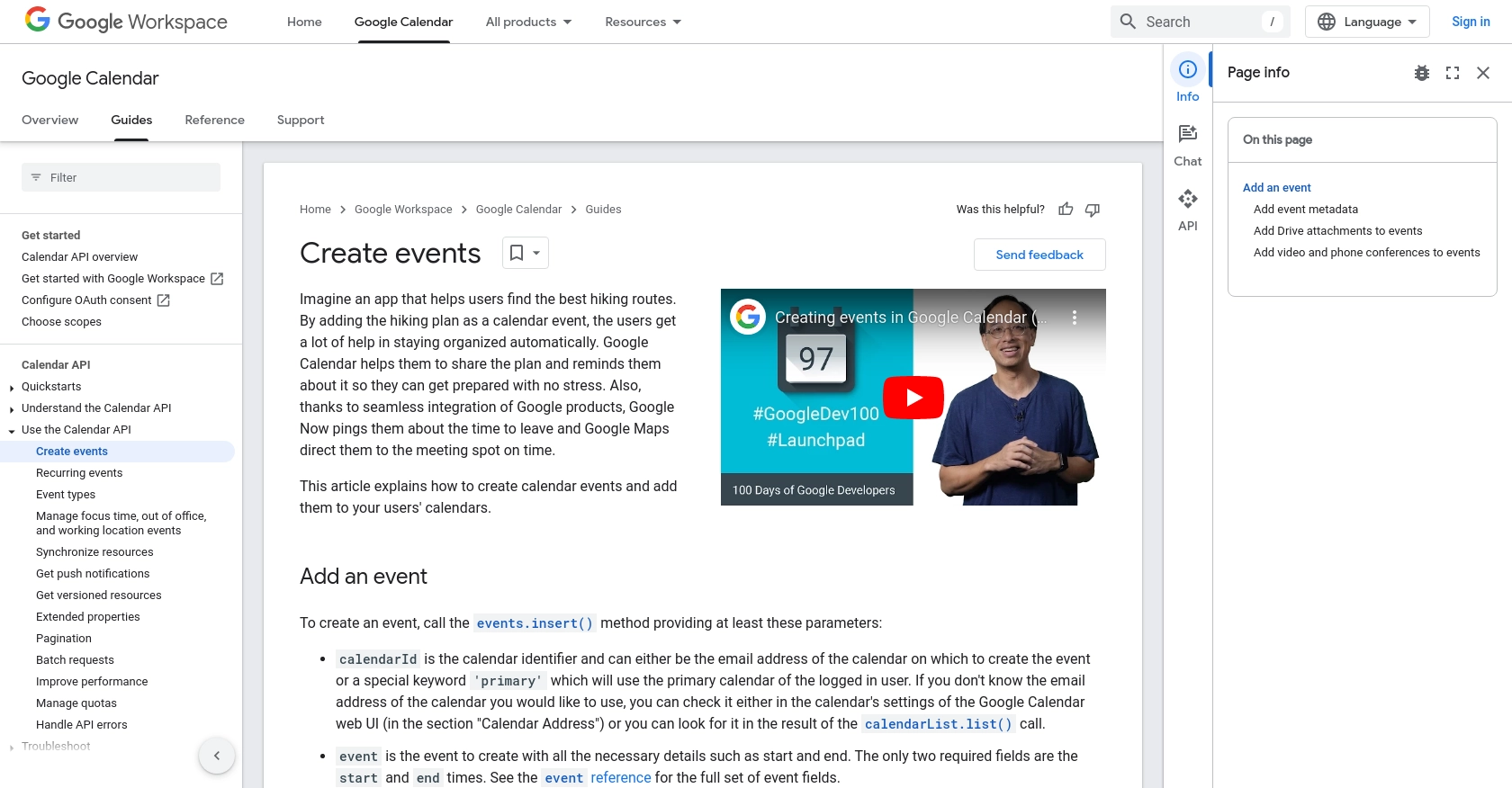
Conclusion and Best Practices for Using Google Calendar API in Python
Integrating with the Google Calendar API using Python allows developers to automate and enhance scheduling functionalities within their applications. By following the steps outlined in this guide, you can efficiently create calendar events and manage them programmatically.
Best Practices for Storing User Credentials Securely
- Always store OAuth tokens securely, using environment variables or secure vaults to prevent unauthorized access.
- Regularly rotate credentials and tokens to minimize security risks.
Handling Google Calendar API Rate Limits
The Google Calendar API enforces rate limits to ensure fair usage. To handle these limits:
- Implement exponential backoff strategies to retry requests after receiving rate limit errors.
- Monitor your application's API usage and adjust request patterns to stay within limits.
Transforming and Standardizing Data Fields
When working with calendar events, ensure that data fields such as dates, times, and time zones are consistently formatted. This helps maintain data integrity and prevents errors during API interactions.
Call to Action: Simplify Integrations with Endgrate
While integrating with Google Calendar API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product. Save time and resources by leveraging Endgrate's intuitive integration platform for seamless connectivity across various services.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/googlecalendar
- https://developers.google.com/calendar/api/v3/reference
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/calendar/api/guides/create-events
Ready to get started?