How to Create or Update Records with the Salesforce Sandbox API in Python
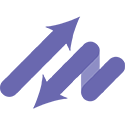
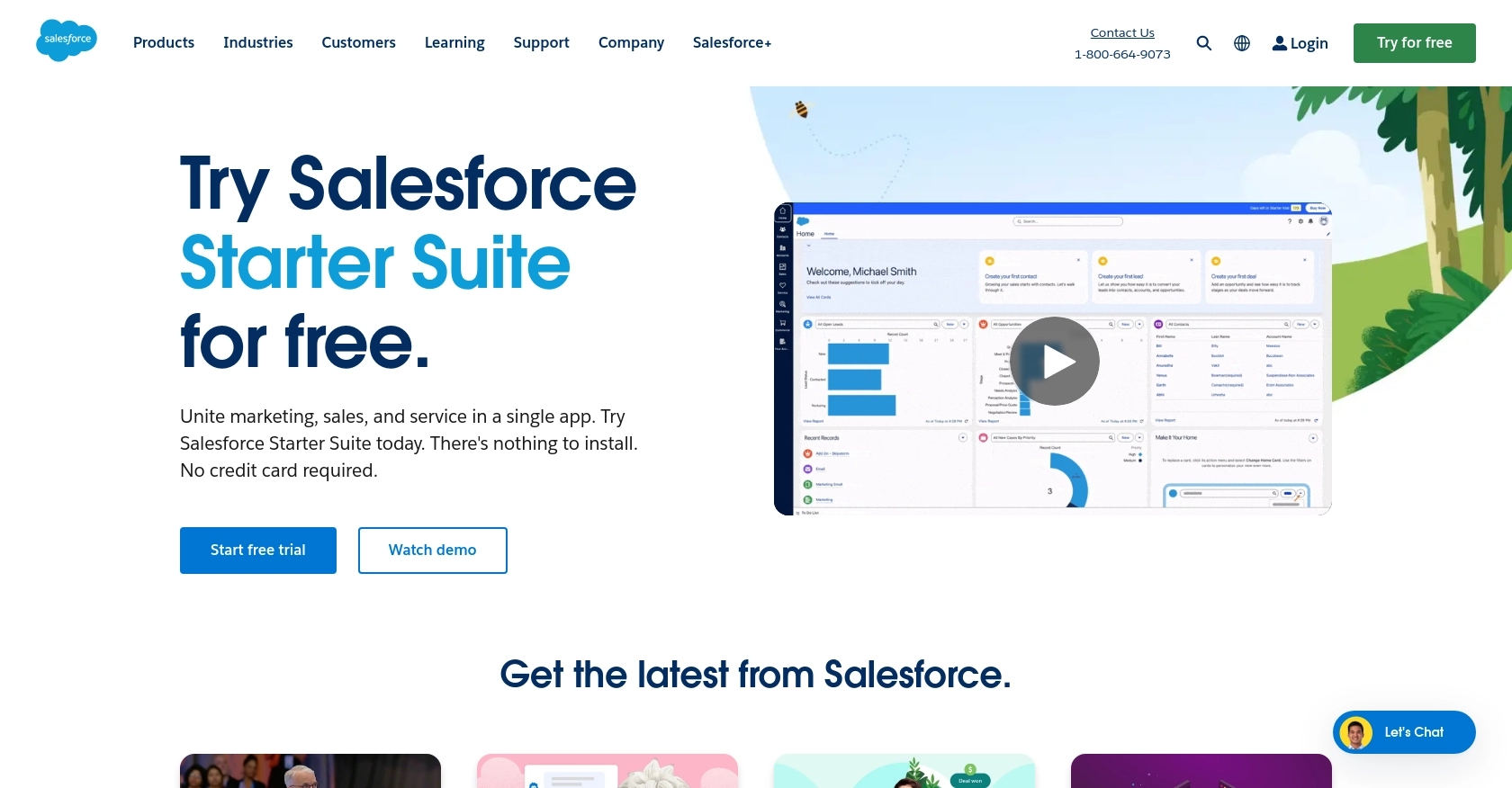
Introduction to Salesforce Sandbox API
Salesforce Sandbox is a powerful tool that allows developers to create and test applications in a secure environment that mirrors their production instance. It provides a safe space to experiment with new features, develop integrations, and ensure that changes won't disrupt live operations.
Integrating with the Salesforce Sandbox API can significantly enhance a developer's ability to manage and manipulate data within Salesforce. For example, you might want to create or update records programmatically to automate data entry processes or synchronize data between Salesforce and other systems.
This article will guide you through using Python to interact with the Salesforce Sandbox API, focusing on creating and updating records efficiently. By leveraging this integration, developers can streamline workflows and improve data accuracy across platforms.
Setting Up Your Salesforce Sandbox Account for API Integration
Before diving into the integration process, it's essential to set up a Salesforce Sandbox account. This environment allows you to safely test and develop without affecting your live data. Follow these steps to get started:
Step 1: Sign Up for Salesforce Developer Edition
If you don't already have a Salesforce Developer Edition account, you'll need to create one. This account provides access to a Salesforce Sandbox environment where you can test your integrations.
- Visit the Salesforce Developer Edition signup page.
- Fill in the required details and submit the form.
- Check your email for a confirmation message and follow the instructions to verify your account.
Step 2: Create a Salesforce Sandbox
Once you have your Developer Edition account, you can create a Sandbox environment:
- Log in to your Salesforce account.
- Navigate to Setup by clicking the gear icon in the top right corner.
- In the Quick Find box, type Sandboxes and select it from the menu.
- Click New Sandbox and follow the prompts to create your Sandbox environment.
Step 3: Set Up OAuth Authentication
Salesforce uses OAuth 2.0 for secure API authentication. You'll need to create a connected app to obtain the necessary credentials:
- In Salesforce Setup, enter App Manager in the Quick Find box and select it.
- Click New Connected App.
- Fill in the required fields, such as Connected App Name and API Name.
- Under API (Enable OAuth Settings), check Enable OAuth Settings.
- Set the Callback URL to a valid URL (e.g.,
https://localhost/callback
). - Select the necessary OAuth scopes, such as Access and manage your data (api).
- Save the app and note down the Consumer Key and Consumer Secret.
For more detailed information, refer to the Salesforce OAuth documentation.
Step 4: Obtain Access Tokens
With your connected app set up, you can now obtain access tokens to authenticate API requests:
import requests
# Set your credentials
client_id = 'Your_Consumer_Key'
client_secret = 'Your_Consumer_Secret'
username = 'Your_Salesforce_Username'
password = 'Your_Salesforce_Password'
security_token = 'Your_Security_Token'
# Define the token endpoint
token_url = 'https://login.salesforce.com/services/oauth2/token'
# Prepare the payload
payload = {
'grant_type': 'password',
'client_id': client_id,
'client_secret': client_secret,
'username': username,
'password': password + security_token
}
# Make the request
response = requests.post(token_url, data=payload)
access_token = response.json().get('access_token')
print(f"Access Token: {access_token}")
Replace the placeholders with your actual credentials. This script will return an access token, which you will use in subsequent API calls.
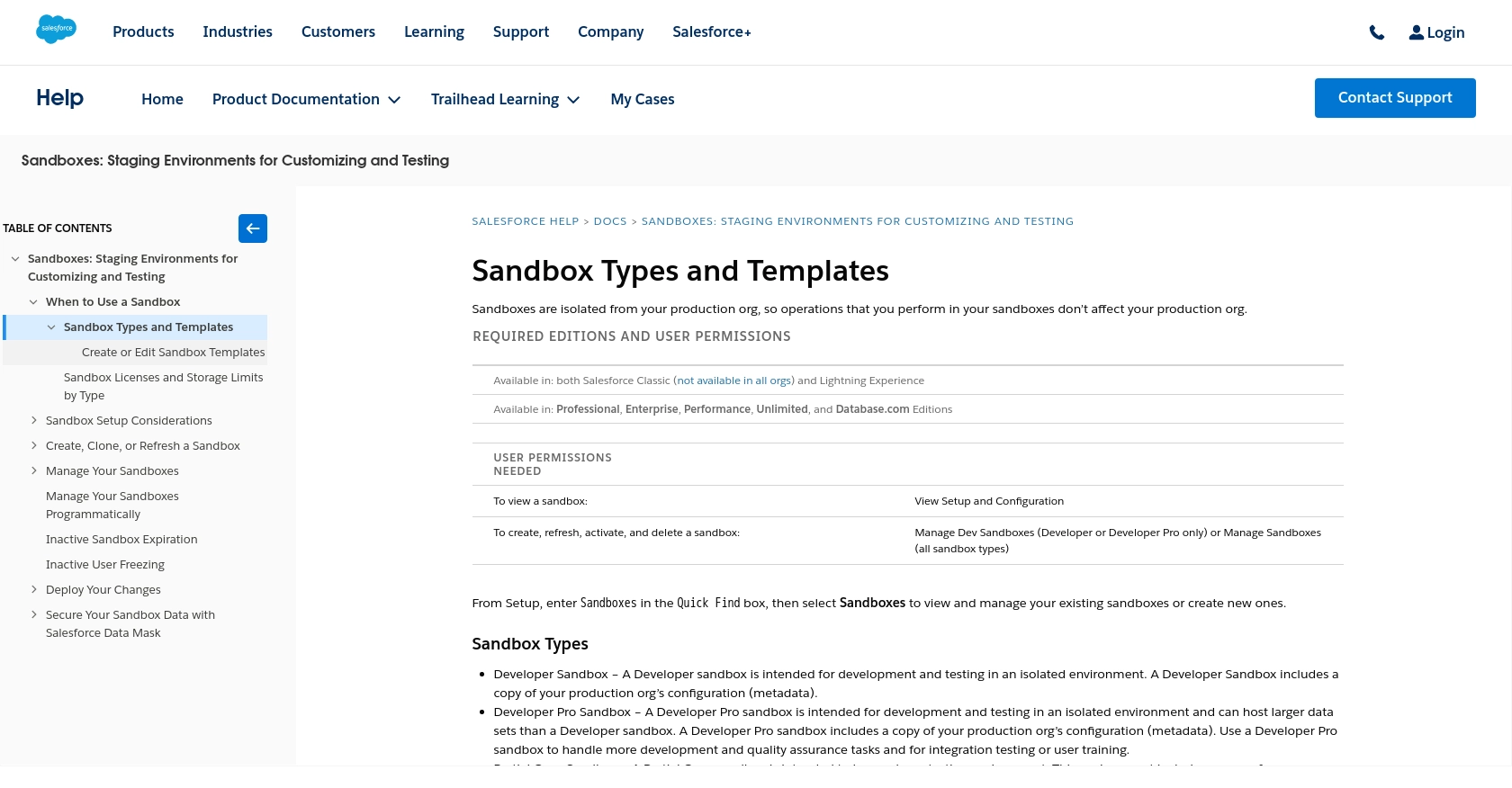
sbb-itb-96038d7
Making API Calls to Create or Update Records in Salesforce Sandbox Using Python
With your Salesforce Sandbox environment and OAuth authentication set up, you can now proceed to make API calls to create or update records. This section will guide you through the process using Python, ensuring you have the necessary tools and knowledge to interact with the Salesforce Sandbox API effectively.
Prerequisites for Salesforce Sandbox API Integration with Python
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
You'll also need to install the requests
library to handle HTTP requests. You can do this by running the following command in your terminal:
pip install requests
Creating a Record in Salesforce Sandbox
To create a record in Salesforce Sandbox, you'll use the POST
method to send data to the Salesforce API. Here's a step-by-step guide:
import requests
import json
# Define the Salesforce API endpoint for creating records
url = 'https://your_instance.salesforce.com/services/data/vXX.X/sobjects/Account/'
# Set the headers, including the access token
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json'
}
# Define the data for the new record
data = {
'Name': 'New Account Name',
'Industry': 'Technology'
}
# Make the POST request to create the record
response = requests.post(url, headers=headers, data=json.dumps(data))
# Check if the request was successful
if response.status_code == 201:
print("Record created successfully:", response.json())
else:
print("Failed to create record:", response.json())
Replace your_instance
with your Salesforce instance URL and vXX.X
with the appropriate API version. This script will create a new account record in Salesforce Sandbox.
Updating a Record in Salesforce Sandbox
To update an existing record, you'll use the PATCH
method. Here's how you can do it:
# Define the Salesforce API endpoint for updating records
record_id = 'Your_Record_ID'
url = f'https://your_instance.salesforce.com/services/data/vXX.X/sobjects/Account/{record_id}'
# Define the data to update the record
update_data = {
'Industry': 'Updated Industry'
}
# Make the PATCH request to update the record
response = requests.patch(url, headers=headers, data=json.dumps(update_data))
# Check if the request was successful
if response.status_code == 204:
print("Record updated successfully")
else:
print("Failed to update record:", response.json())
Replace Your_Record_ID
with the ID of the record you wish to update. This script will update the specified fields of the account record in Salesforce Sandbox.
Verifying API Requests in Salesforce Sandbox
After making API calls, you can verify the changes in your Salesforce Sandbox environment:
- Log in to your Salesforce Sandbox account.
- Navigate to the relevant object (e.g., Accounts) to see the newly created or updated records.
If the records appear as expected, your API calls were successful. If not, review the error messages returned by the API to troubleshoot any issues.
Handling Errors and Common Error Codes
When interacting with the Salesforce API, you may encounter various error codes. Here are some common ones:
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: The access token is missing or invalid.
- 403 Forbidden: The authenticated user does not have permission to perform the operation.
- 404 Not Found: The requested resource does not exist.
Always check the response from the API and handle errors gracefully in your code to ensure a robust integration.
Best Practices for Salesforce Sandbox API Integration
Successfully integrating with the Salesforce Sandbox API requires adherence to best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the client ID and client secret, securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Salesforce imposes rate limits on API requests. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Salesforce documentation.
- Standardize Data Fields: Ensure that data fields are standardized across systems to maintain consistency. This can help prevent errors and improve data integrity.
- Implement Error Handling: Always check API responses for errors and handle them appropriately. Log errors for debugging and provide meaningful feedback to users.
Streamline Your Integrations with Endgrate
Building and maintaining integrations with platforms like Salesforce can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Salesforce Sandbox.
With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/salesforce-sandbox
- https://help.salesforce.com/s/articleView?id=sf.create_test_instance.htm&type=5
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?