How to Create or Update Contacts with the Teamwork CRM API in Javascript
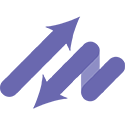
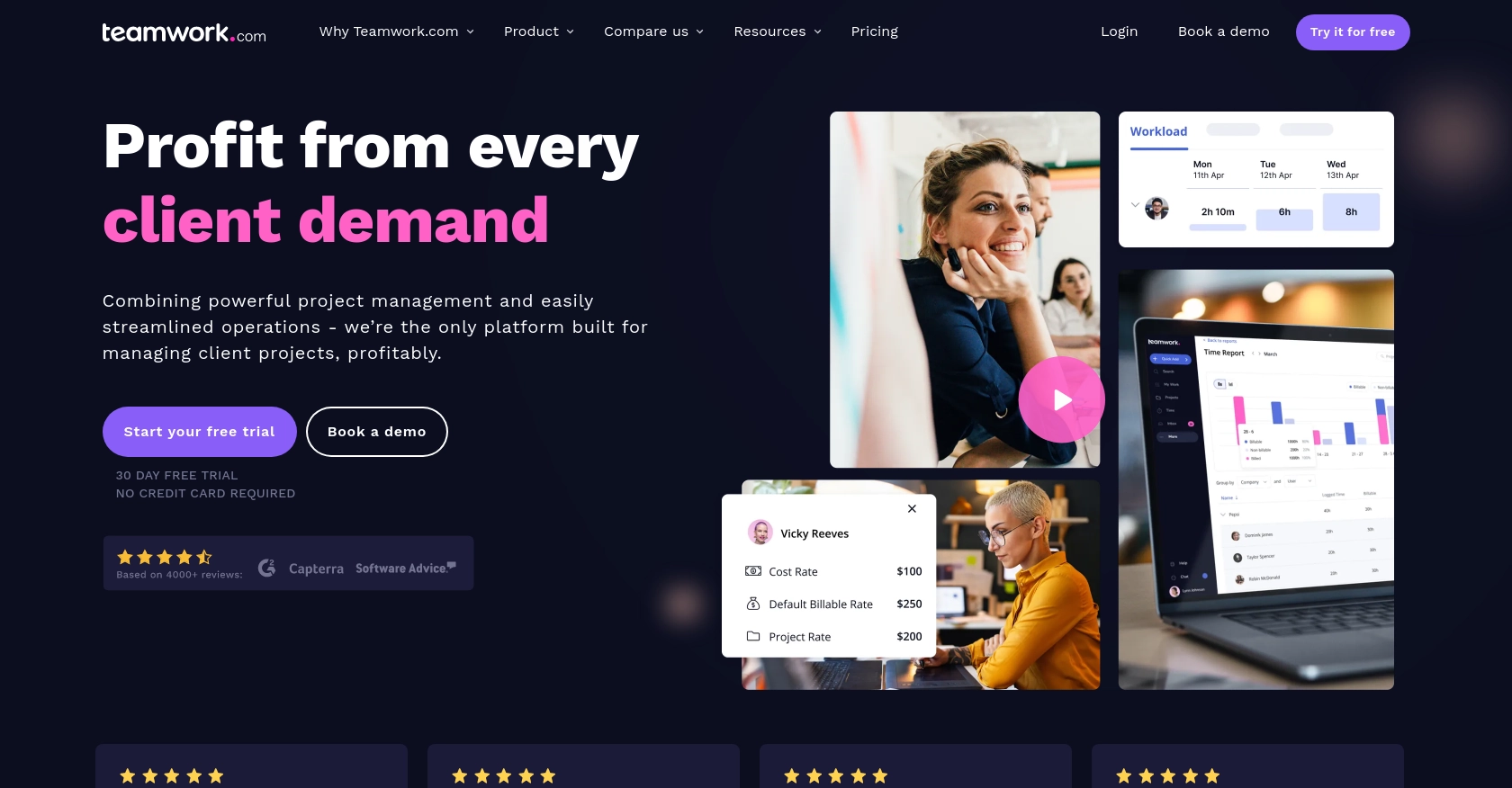
Introduction to Teamwork CRM
Teamwork CRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. It offers a comprehensive suite of tools for tracking leads, managing contacts, and automating sales workflows, making it an ideal choice for businesses looking to enhance their customer engagement strategies.
Integrating with the Teamwork CRM API allows developers to automate and streamline contact management tasks. For example, a developer might want to create or update contact information directly from a custom application, ensuring that the sales team always has access to the most current data without manual entry.
Setting Up Your Teamwork CRM Test Account
Before you can start integrating with the Teamwork CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork CRM website. Follow the instructions to create your account. Once your account is set up, you'll have access to the CRM dashboard.
Generate API Credentials for Teamwork CRM
To interact with the Teamwork CRM API, you'll need to generate API credentials. Follow these steps to obtain your API key:
- Log in to your Teamwork CRM account.
- Navigate to the Settings section in the top navigation bar.
- Select API & Webhooks from the dropdown menu.
- Click on Create API Key and follow the prompts to generate your key.
- Copy the API key and store it securely, as you'll need it for authentication in your API requests.
Understanding Teamwork CRM API Authentication
The Teamwork CRM API supports both Basic Authentication and OAuth 2.0. For this tutorial, we'll use Basic Authentication. Here's how to set it up:
- Use the API key you generated as the username.
- Leave the password field empty.
- Encode the username:password combination in base64 format.
- Include the encoded string in the
Authorization
header of your API requests, prefixed withBasic
.
For more details on authentication, refer to the Teamwork CRM Authentication Documentation.
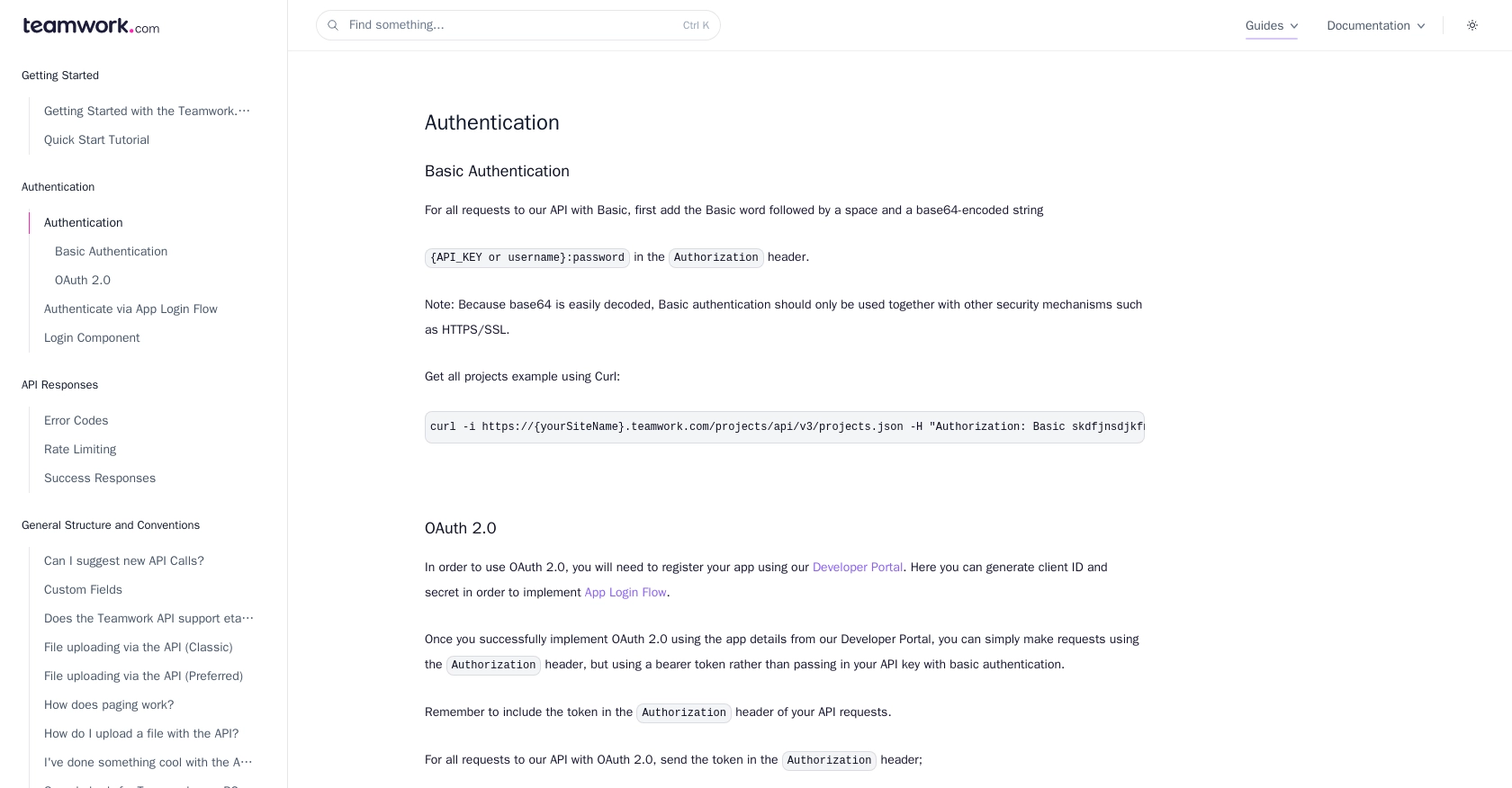
sbb-itb-96038d7
Making API Calls to Create or Update Contacts in Teamwork CRM Using JavaScript
Setting Up Your JavaScript Environment for Teamwork CRM API Integration
To interact with the Teamwork CRM API using JavaScript, you'll need to ensure your environment is properly configured. This includes having Node.js installed, which allows you to run JavaScript outside of a browser. Additionally, you'll need a package manager like npm to install necessary dependencies.
Installing Required Packages for Teamwork CRM API Calls
For making HTTP requests, we'll use the popular axios
library. Install it by running the following command in your terminal:
npm install axios
Creating and Updating Contacts with Teamwork CRM API
Below is a step-by-step guide to creating or updating contacts using the Teamwork CRM API with JavaScript.
Example Code for Creating a Contact in Teamwork CRM
Create a new JavaScript file named createContact.js
and add the following code:
const axios = require('axios');
// Set the API endpoint
const url = 'https://{yourSiteName}.teamwork.com/crm/v2/contacts';
// Set the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Basic ' + Buffer.from('Your_API_Key:').toString('base64')
};
// Define the contact data
const contactData = {
"contact": {
"firstName": "John",
"lastName": "Doe",
"email": "john.doe@example.com"
}
};
// Make the POST request to create a contact
axios.post(url, contactData, { headers })
.then(response => {
console.log('Contact Created:', response.data);
})
.catch(error => {
console.error('Error Creating Contact:', error.response.data);
});
Replace Your_API_Key
with your actual API key and {yourSiteName}
with your Teamwork site name.
Example Code for Updating a Contact in Teamwork CRM
To update an existing contact, create a file named updateContact.js
and use the following code:
const axios = require('axios');
// Set the API endpoint with the contact ID
const url = 'https://{yourSiteName}.teamwork.com/crm/v2/contacts/{contactId}';
// Set the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Basic ' + Buffer.from('Your_API_Key:').toString('base64')
};
// Define the updated contact data
const updatedContactData = {
"contact": {
"firstName": "Jane",
"lastName": "Doe",
"email": "jane.doe@example.com"
}
};
// Make the PUT request to update the contact
axios.put(url, updatedContactData, { headers })
.then(response => {
console.log('Contact Updated:', response.data);
})
.catch(error => {
console.error('Error Updating Contact:', error.response.data);
});
Replace {contactId}
with the ID of the contact you wish to update.
Verifying API Call Success in Teamwork CRM
After running the scripts, you should verify the success of your API calls by checking the Teamwork CRM dashboard. Newly created or updated contacts should appear in your contact list.
Handling Errors and Understanding Teamwork CRM API Error Codes
When making API calls, you might encounter errors. Common error codes include:
- 401 Unauthorized: Check your API key and ensure it's correctly set up.
- 422 Unprocessable Entity: Ensure the JSON structure is correct.
- 429 Too Many Requests: You've exceeded the rate limit of 150 requests per minute. Wait a while before retrying.
For more details on error codes, refer to the Teamwork CRM Error Codes Documentation.
Conclusion and Best Practices for Integrating with Teamwork CRM API
Integrating with the Teamwork CRM API using JavaScript can significantly enhance your ability to manage contacts efficiently. By automating the creation and updating of contact information, you ensure that your sales team always has access to the most current data, reducing manual entry errors and saving valuable time.
Best Practices for Secure and Efficient API Integration with Teamwork CRM
- Secure Storage of API Credentials: Always store your API keys securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of the rate limit of 150 requests per minute. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data fields are consistently formatted before sending them to the API. This helps in maintaining data integrity across systems.
Leveraging Endgrate for Streamlined Integration Processes
While integrating with individual APIs like Teamwork CRM can be beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help. By providing a unified API endpoint, Endgrate allows you to connect with multiple platforms seamlessly, saving you time and resources.
With Endgrate, you can focus on your core product development while outsourcing the complexities of integration management. This not only accelerates your development process but also enhances the integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate today.
Read More
Ready to get started?