How to Create Customers with the Endear API in Javascript
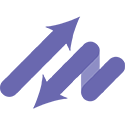
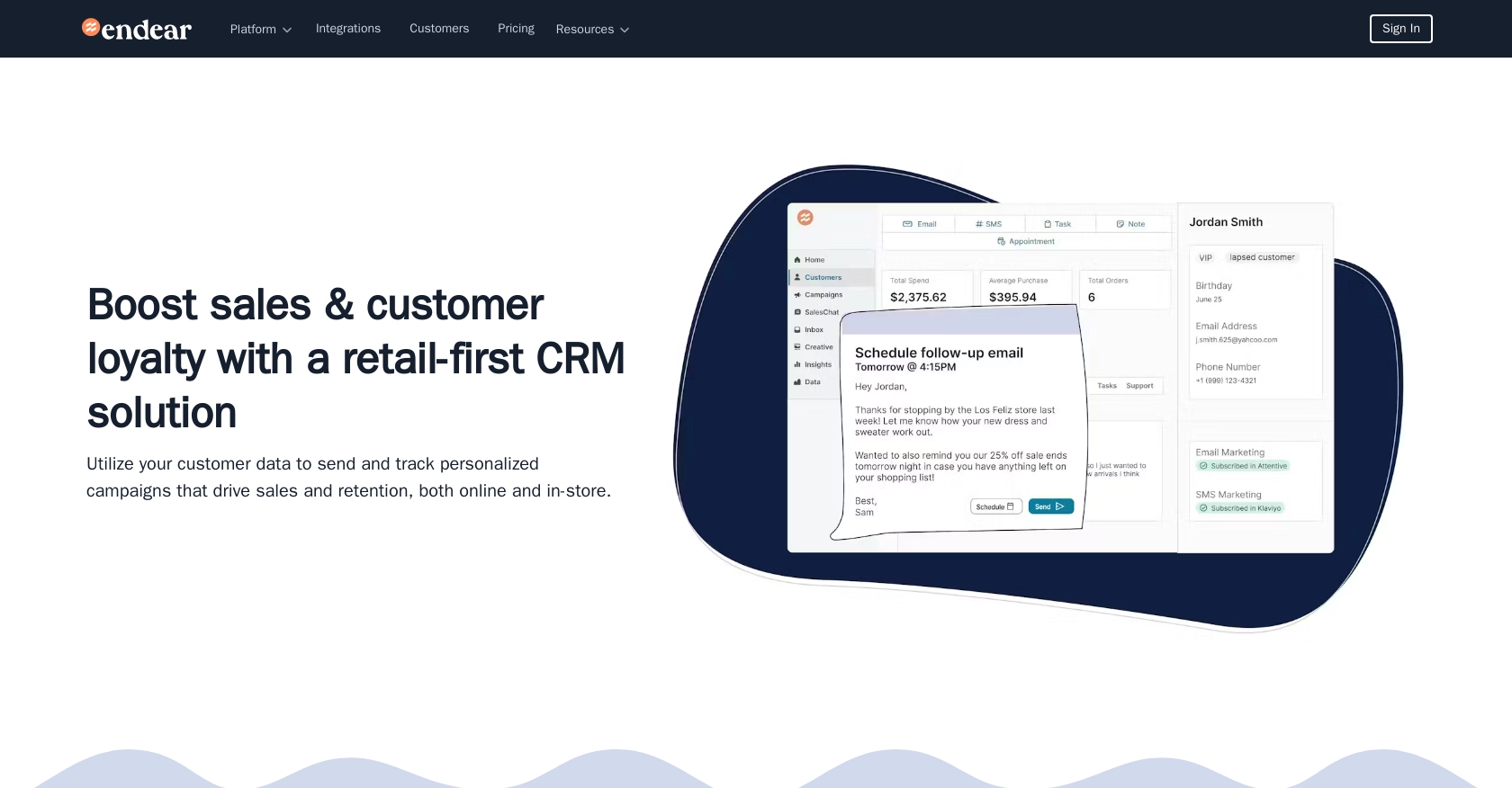
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationships by providing seamless integration capabilities for businesses. It offers a robust API that allows developers to interact with customer data efficiently, making it an ideal choice for businesses looking to streamline their customer management processes.
Integrating with Endear's API can empower developers to automate customer data handling, such as creating new customer profiles directly from external sources. For example, a developer might use the Endear API to automatically add new customers from an e-commerce platform, ensuring that customer data is always up-to-date and accessible.
Setting Up Your Endear Test Account for API Integration
Before you can start creating customers with the Endear API using JavaScript, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting live data. Follow these steps to get started:
Create an Endear Account and Access the Integration Settings
- Visit the Endear website and sign up for a free account if you haven't already. This will give you access to the platform's features and integration capabilities.
- Once logged in, navigate to the Settings section of your Endear dashboard.
- Click on Integrations to manage your API integrations.
Generate an API Key for Endear API Access
- In the Integrations section, click on Add Integration and select API from the options.
- Fill in the required details to create your integration. This will generate an API key specific to your brand.
- Make sure to copy and securely store your API key, as you will need it to authenticate your API requests.
For more information on authentication, refer to the Endear authentication documentation.
Understanding API Key Authentication with Endear
Endear uses API key-based authentication to secure its endpoints. When making API requests, include the header X-Endear-Api-Key
with the value of your generated API key. This ensures that your requests are authenticated and authorized to access the API.
It's important to note that each API key is scoped to a specific brand, so you'll need a separate key for each brand you wish to integrate with.
Verify Your Setup in the Endear Sandbox Environment
Once your API key is set up, you can test your integration in the Endear sandbox environment. This allows you to verify that your API calls are functioning correctly without impacting live data.
To ensure your setup is correct, you can make a simple API call to check the connection. Use the following example code to test your setup:
// Example JavaScript code to test Endear API connection
fetch('https://api.endearhq.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'YOUR_API_KEY'
},
body: JSON.stringify({
query: 'query { currentIntegration { id } }'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace YOUR_API_KEY
with your actual API key. If the setup is correct, you should receive a response confirming the integration ID.
For further details on API limits and error handling, refer to the Endear rate limits and error documentation.
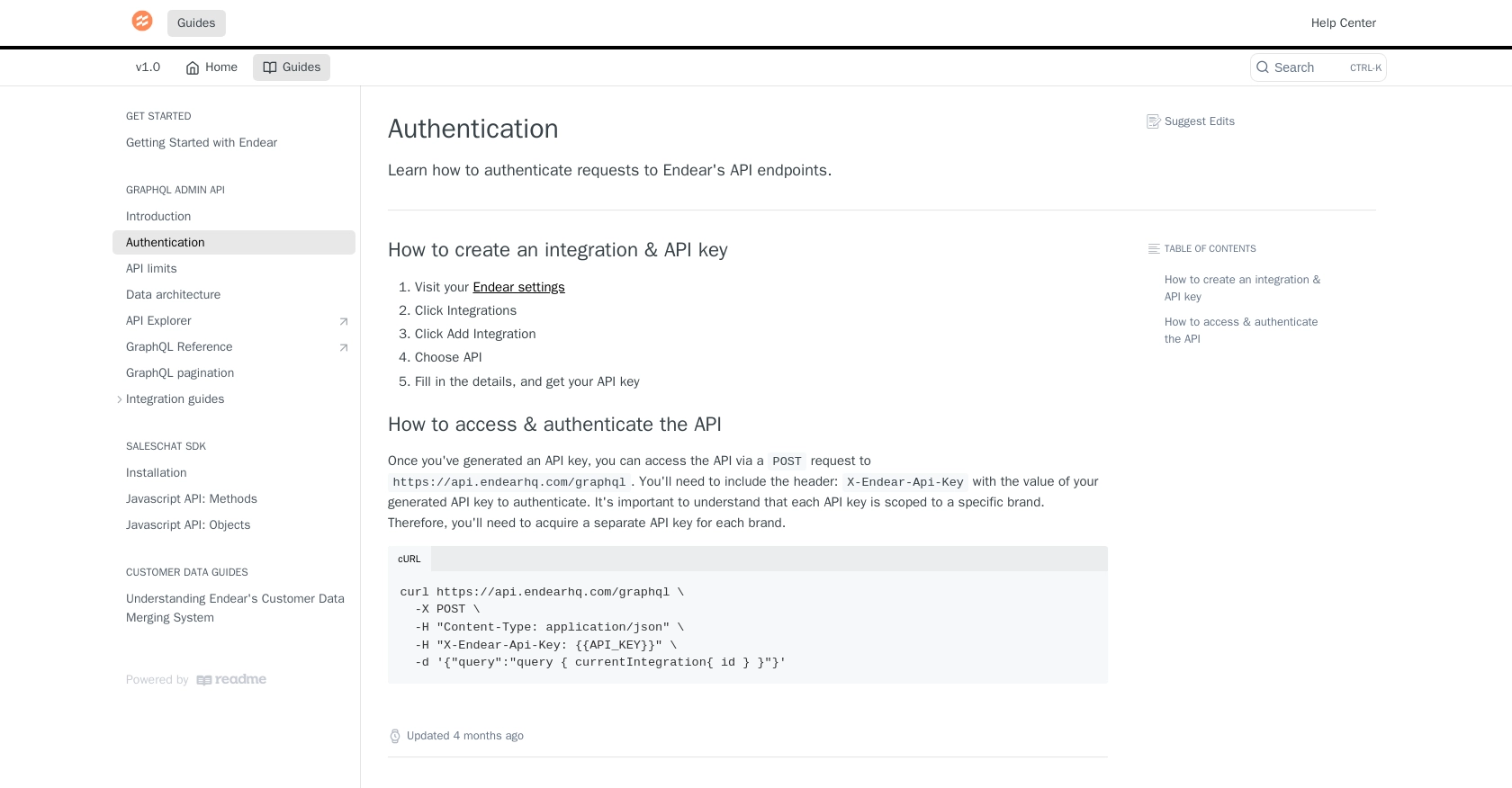
sbb-itb-96038d7
Making API Calls to Create Customers with Endear API in JavaScript
To create customers using the Endear API with JavaScript, you'll need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process, ensuring you have everything you need to successfully create customer profiles.
Setting Up Your JavaScript Environment for Endear API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Basic understanding of JavaScript and API interactions.
Installing Required Dependencies for Endear API
To make HTTP requests in JavaScript, you can use the node-fetch
library. Install it by running the following command in your terminal:
npm install node-fetch
Writing JavaScript Code to Create Customers with Endear API
Now, let's write the JavaScript code to create a new customer using the Endear API. Follow these steps:
const fetch = require('node-fetch');
// Define the API endpoint and headers
const endpoint = 'https://api.endearhq.com/graphql';
const headers = {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'YOUR_API_KEY'
};
// Define the GraphQL mutation for creating a customer
const createCustomerMutation = `
mutation {
createCustomer(input: {
email: "newcustomer@example.com",
firstName: "John",
lastName: "Doe"
}) {
customer {
id
email
firstName
lastName
}
}
}
`;
// Make the API call
fetch(endpoint, {
method: 'POST',
headers: headers,
body: JSON.stringify({ query: createCustomerMutation })
})
.then(response => response.json())
.then(data => {
if (data.errors) {
console.error('Error creating customer:', data.errors);
} else {
console.log('Customer created successfully:', data.data.createCustomer.customer);
}
})
.catch(error => console.error('Fetch error:', error));
Replace YOUR_API_KEY
with your actual API key. This code sends a GraphQL mutation to create a new customer with specified details. If successful, it logs the newly created customer's information.
Verifying Successful Customer Creation in Endear Sandbox
After running the code, verify that the customer was created by checking the response data. You should see the customer's details logged in the console. Additionally, you can log into your Endear sandbox account to confirm the new customer profile appears in the system.
Handling Errors and Understanding Endear API Rate Limits
While making API calls, it's crucial to handle potential errors. The Endear API may return errors if the request is malformed or if rate limits are exceeded. Refer to the Endear rate limits and error documentation for more details.
Endear's API allows up to 120 requests per minute. If you exceed this limit, you'll receive an HTTP 429 error. Implement retry logic or backoff strategies to handle rate limiting gracefully.
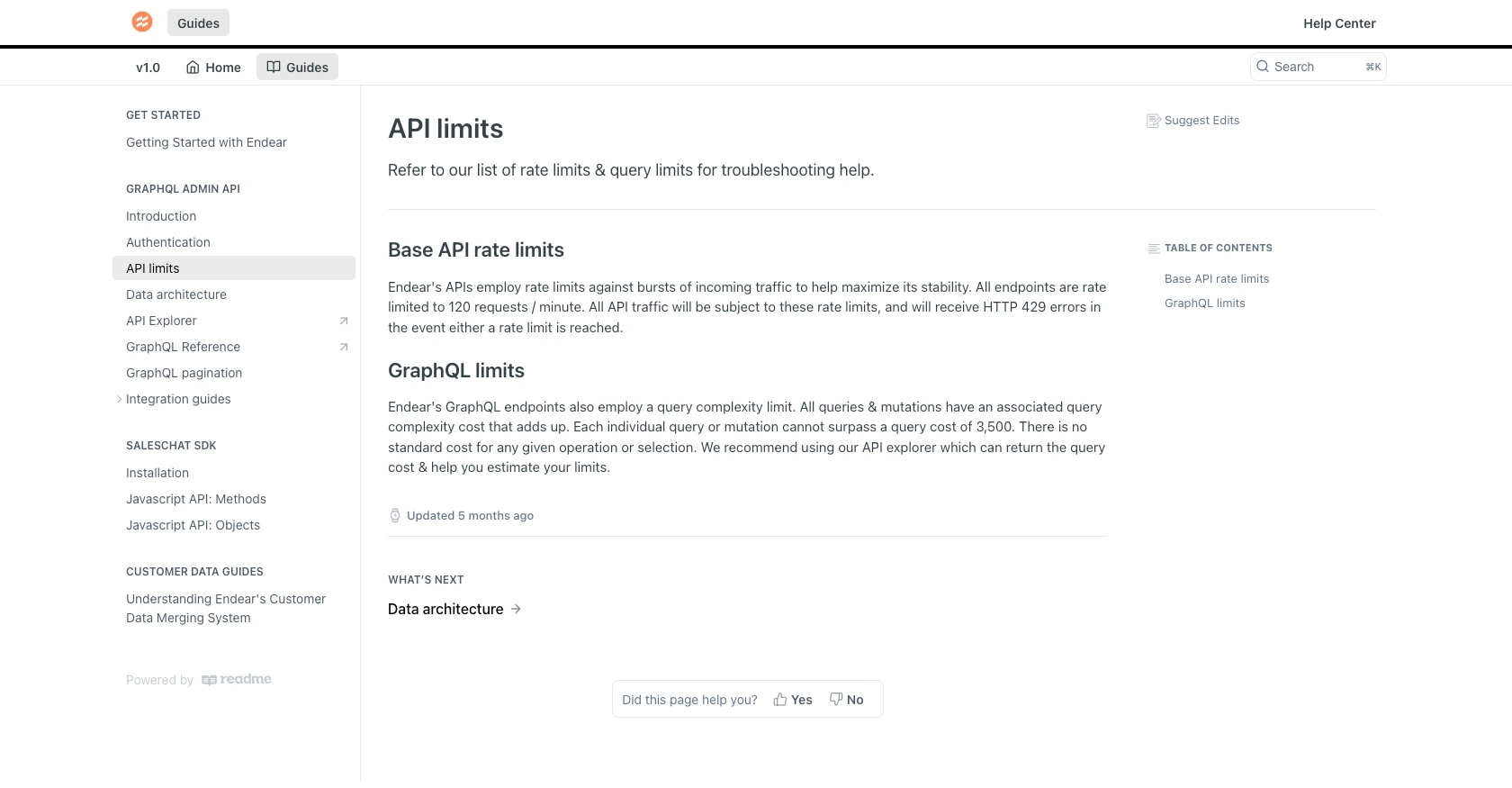
Conclusion and Best Practices for Using Endear API in JavaScript
Integrating with the Endear API using JavaScript provides a powerful way to manage customer data efficiently. By following the steps outlined in this guide, you can seamlessly create customer profiles and ensure your data is up-to-date and accessible.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits Gracefully: Endear's API enforces a rate limit of 120 requests per minute. Implement retry logic or exponential backoff strategies to handle HTTP 429 errors effectively.
- Data Standardization: Ensure consistent data formats when interacting with the API. This helps in maintaining data integrity and simplifies integration with other systems.
Enhance Your Integration Strategy with Endgrate
While building integrations with Endear API is straightforward, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Endear.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a unified API approach.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Customer
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?