Using the Sugar Sell API to Get Records in Javascript
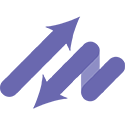
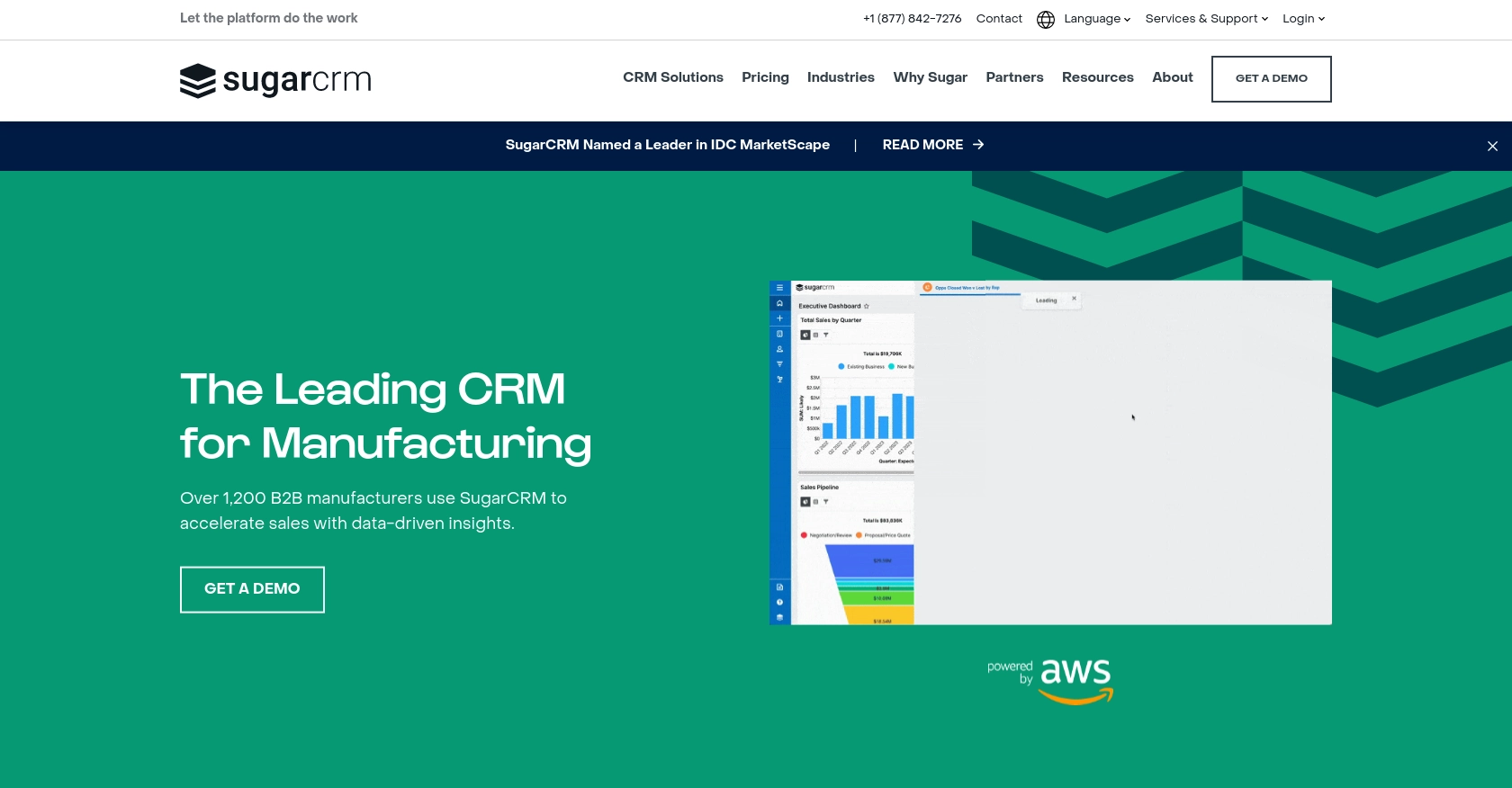
Introduction to Sugar Sell API
Sugar Sell is a robust customer relationship management (CRM) platform designed to enhance sales processes and customer interactions for businesses. It offers a comprehensive suite of tools that streamline sales activities, improve customer engagement, and drive revenue growth.
Developers often integrate with the Sugar Sell API to access and manage CRM data programmatically. This integration enables automation of tasks such as retrieving customer records, updating sales information, and syncing data across platforms. For example, a developer might use the Sugar Sell API to automatically fetch and update customer records in a centralized database, ensuring that sales teams have access to the most current information.
Setting Up Your Sugar Sell Test or Sandbox Account
Before you can start integrating with the Sugar Sell API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Sugar Sell Sandbox Account
To begin, sign up for a Sugar Sell sandbox account. This can typically be done through the SugarCRM website, where you can access a free trial or request a sandbox environment specifically for development purposes.
- Visit the SugarCRM website and navigate to the trial or sandbox section.
- Follow the instructions to create your account, providing necessary details such as your email and company information.
- Once your account is set up, log in to access the Sugar Sell dashboard.
Configuring OAuth2 Authentication for Sugar Sell API
The Sugar Sell API uses OAuth2 for authentication, which requires setting up an application within your sandbox account. Follow these steps to configure OAuth2:
- Navigate to the Administration section in your Sugar Sell dashboard.
- Locate the OAuth2 settings and create a new application.
- Enter the required details, including the client_id and client_secret. These will be used to authenticate API requests.
- Ensure the platform is set to "custom" to avoid login conflicts, as recommended in the SugarCRM documentation.
Generating Access Tokens
With your OAuth2 application configured, you can now generate access tokens to authenticate your API calls:
// Example of a POST request to obtain an access token
const axios = require('axios');
const data = {
grant_type: 'password',
client_id: 'sugar',
client_secret: '',
username: '',
password: '',
platform: 'custom'
};
axios.post('https:///rest/v/oauth2/token', data)
.then(response => {
console.log('Access Token:', response.data.access_token);
})
.catch(error => {
console.error('Error fetching token:', error);
});
Replace <your_username>
, <your_password>
, and <site_url>
with your actual credentials and site URL. Store the access_token
securely for use in subsequent API requests.
By following these steps, you'll have a fully configured sandbox account and be ready to start making API calls to Sugar Sell. This setup ensures that you can test and develop integrations without impacting your production environment.
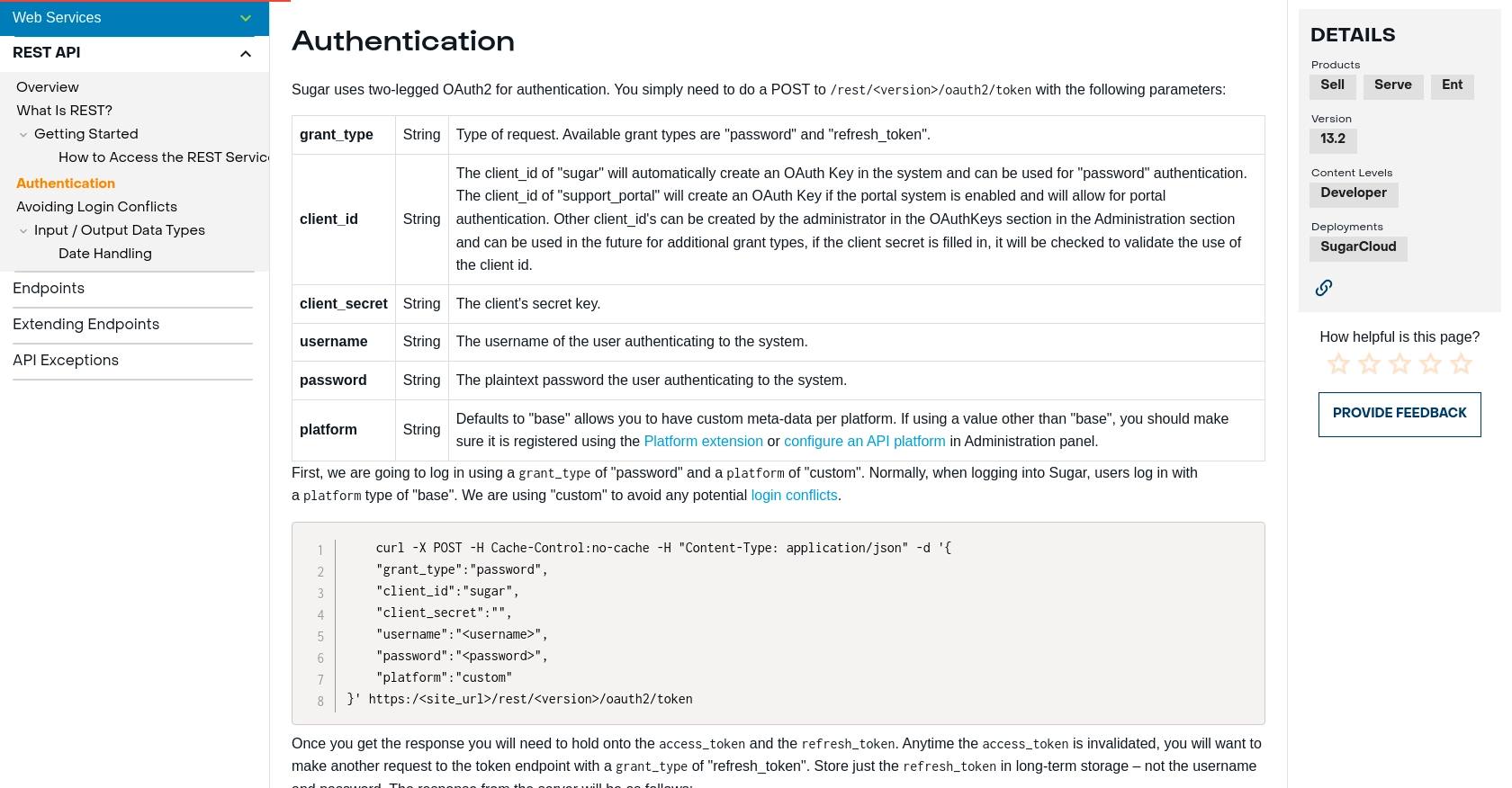
sbb-itb-96038d7
Making API Calls to Retrieve Records from Sugar Sell Using JavaScript
Once you have set up your Sugar Sell sandbox account and configured OAuth2 authentication, you can start making API calls to interact with the Sugar Sell platform. In this section, we'll guide you through the process of retrieving records using JavaScript.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Retrieving Records from Sugar Sell API
To fetch records from Sugar Sell, you'll need to make a GET request to the appropriate API endpoint. Below is an example of how to retrieve records using JavaScript:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https:///rest/v/module/Accounts';
const headers = {
'Authorization': 'Bearer ',
'Content-Type': 'application/json'
};
// Make a GET request to the API
axios.get(endpoint, { headers: headers })
.then(response => {
console.log('Records:', response.data);
})
.catch(error => {
console.error('Error fetching records:', error);
});
Replace <site_url>
, <version>
, and <your_access_token>
with your actual site URL, API version, and access token. This code will fetch records from the "Accounts" module and log them to the console.
Verifying API Call Success
After executing the API call, verify the success of the request by checking the response data. The retrieved records should match the data available in your Sugar Sell sandbox account. If the request is successful, you will see the records printed in the console.
Handling Errors and Error Codes
It's crucial to handle potential errors when making API calls. The catch
block in the example code captures any errors that occur during the request. You can further enhance error handling by checking specific error codes and implementing retry logic if necessary.
For more detailed information on error codes, refer to the SugarCRM API documentation.
Conclusion and Best Practices for Using Sugar Sell API with JavaScript
Integrating with the Sugar Sell API using JavaScript can significantly enhance your CRM capabilities by automating data retrieval and management. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth2, and make API calls to retrieve records.
Best Practices for Secure and Efficient Sugar Sell API Integration
- Securely Store Credentials: Always store your access tokens securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic and exponential backoff strategies to manage requests efficiently.
- Standardize Data Formats: Ensure consistent data formats when integrating with other systems. This can help in maintaining data integrity and ease of processing.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with Sugar Sell API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sugar Sell. By using Endgrate, you can focus on your core product while outsourcing the complexities of integration management.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover how you can build once for each use case, providing an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/sugarsell
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/#Authentication
- https://support.sugarcrm.com/documentation/sugar_developer/sugar_developer_guide_13.2/integration/web_services/rest_api/endpoints/
Ready to get started?