How to Get Service Accounts with the Mixpanel API in PHP
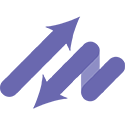
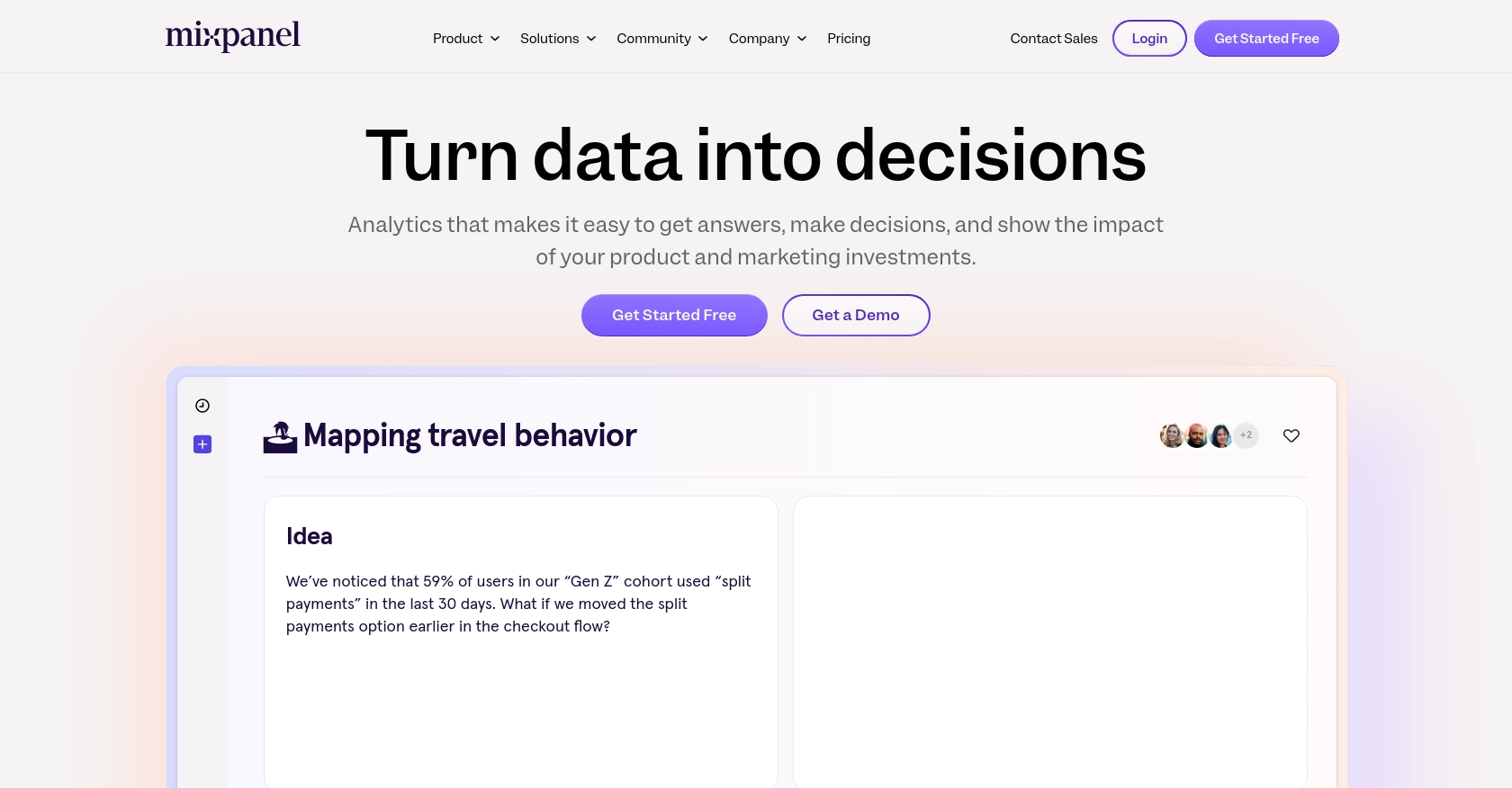
Introduction to Mixpanel API Integration
Mixpanel is a powerful analytics platform that helps businesses track user interactions with web and mobile applications. It provides deep insights into user behavior, enabling companies to make data-driven decisions to enhance user engagement and optimize product offerings.
Integrating with Mixpanel's API allows developers to automate data collection and management processes. For example, you can use the Mixpanel API to retrieve service account details, which can be crucial for managing access and permissions across various projects and workspaces within an organization.
Setting Up Your Mixpanel Test Account
Before you can start interacting with the Mixpanel API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Mixpanel offers a sandbox environment that developers can use to test their integrations.
Creating a Mixpanel Account
If you don't already have a Mixpanel account, you can sign up for a free account on the Mixpanel website. Follow the instructions to create your account and log in. This will give you access to the Mixpanel dashboard where you can manage your projects and service accounts.
Generating Service Account Credentials
To authenticate your API requests, you'll need to create a service account in Mixpanel. Service accounts are designed for non-human entities like scripts or backend services and can be granted access to various projects within your organization.
- Navigate to the Organization Settings in your Mixpanel dashboard.
- Select the Service Accounts tab.
- Click on Create Service Account.
- Choose the role and projects you want the service account to have access to.
- Once created, immediately store the service account's secret in a secure location, as you won't be able to access it again.
For more details on managing service accounts, refer to the Mixpanel Service Accounts documentation.
Authenticating with Mixpanel API
Mixpanel uses HTTP Basic Auth for authenticating service accounts. You will need the service account's username and secret to make API calls.
// Example of authenticating with Mixpanel API using PHP
$serviceAccountUsername = 'your_service_account_username';
$serviceAccountSecret = 'your_service_account_secret';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://mixpanel.com/api/app/me');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_USERPWD, $serviceAccountUsername . ':' . $serviceAccountSecret);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Ensure you replace your_service_account_username
and your_service_account_secret
with your actual credentials. For more information on authentication, visit the Mixpanel Authentication documentation.
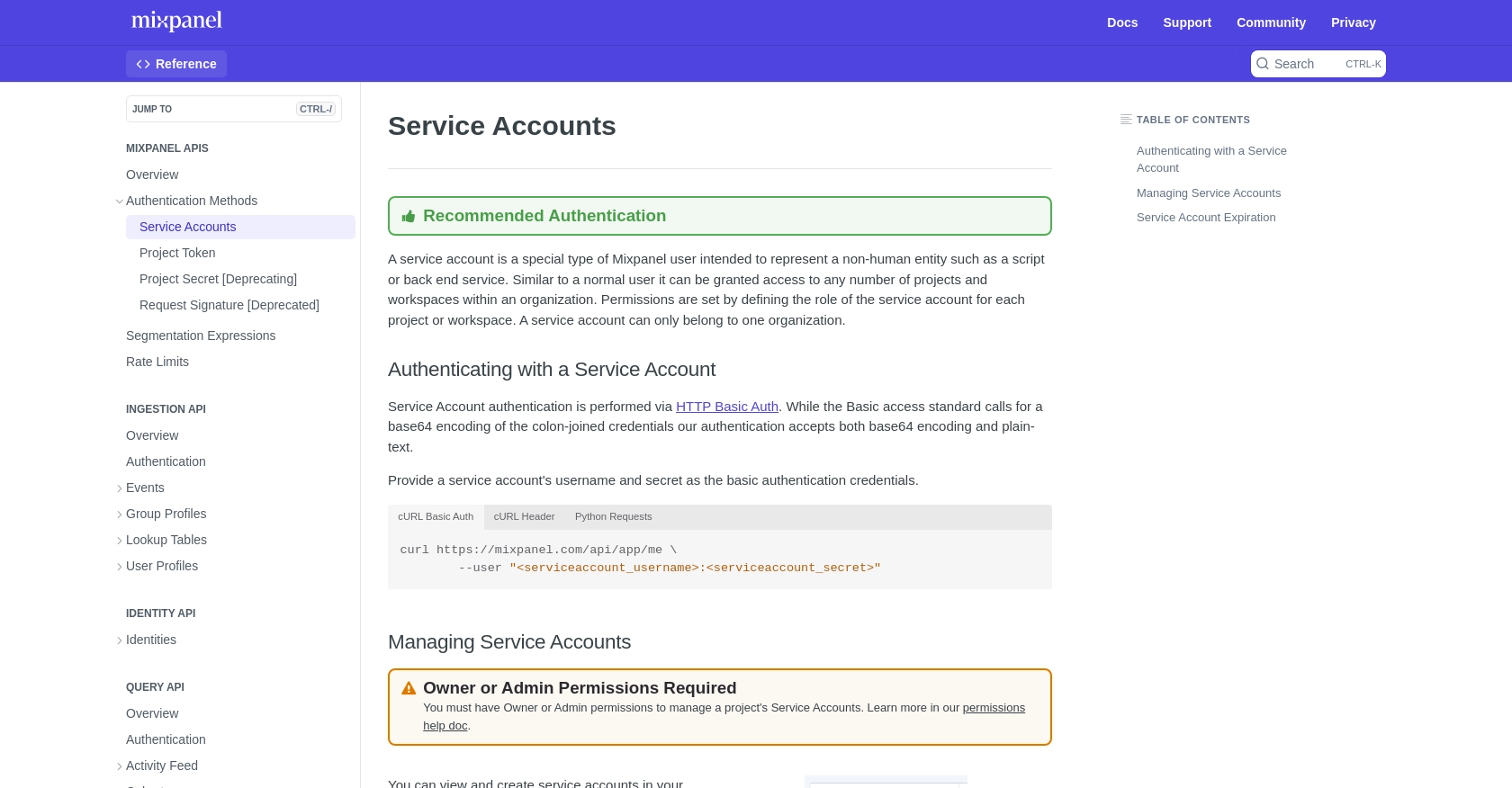
sbb-itb-96038d7
Making API Calls to Retrieve Mixpanel Service Accounts Using PHP
To interact with the Mixpanel API and retrieve service account details, you'll need to make HTTP requests using PHP. This section will guide you through setting up your PHP environment and executing the necessary API calls.
Setting Up Your PHP Environment for Mixpanel API Integration
Before making API calls, ensure you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need the cURL extension enabled, which is typically included by default in most PHP installations.
Installing Required PHP Dependencies
To make HTTP requests, you'll use PHP's cURL functions. Ensure that the cURL extension is enabled in your php.ini
file. You can verify this by running the following command:
php -m | grep curl
If cURL is not listed, enable it by uncommenting the line ;extension=curl
in your php.ini
file and restarting your web server.
Executing the Mixpanel API Call to List Service Accounts
With your environment set up, you can now make an API call to retrieve the list of service accounts associated with your Mixpanel organization. Below is a sample PHP script to achieve this:
// Define your Mixpanel service account credentials
$serviceAccountUsername = 'your_service_account_username';
$serviceAccountSecret = 'your_service_account_secret';
// Initialize cURL session
$ch = curl_init();
// Set the Mixpanel API endpoint for listing service accounts
curl_setopt($ch, CURLOPT_URL, 'https://mixpanel.com/api/app/organizations/{organizationId}/service-accounts');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// Set HTTP Basic Authentication credentials
curl_setopt($ch, CURLOPT_USERPWD, $serviceAccountUsername . ':' . $serviceAccountSecret);
// Execute the API call
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display the response
$serviceAccounts = json_decode($response, true);
foreach ($serviceAccounts as $account) {
echo 'Service Account ID: ' . $account['id'] . "\n";
echo 'Service Account Name: ' . $account['name'] . "\n";
}
}
// Close the cURL session
curl_close($ch);
Replace your_service_account_username
and your_service_account_secret
with your actual Mixpanel service account credentials. Ensure you also replace {organizationId}
with your organization's ID.
Verifying Successful API Requests and Handling Errors
After executing the script, you should see a list of service accounts printed to your console. If the request fails, the script will output an error message. Common error codes include:
- 401 Unauthorized: Check your credentials and ensure they are correct.
- 429 Too Many Requests: You have hit the rate limit. Refer to the Mixpanel Rate Limits documentation for more details.
For more information on the API call, visit the Mixpanel API documentation.
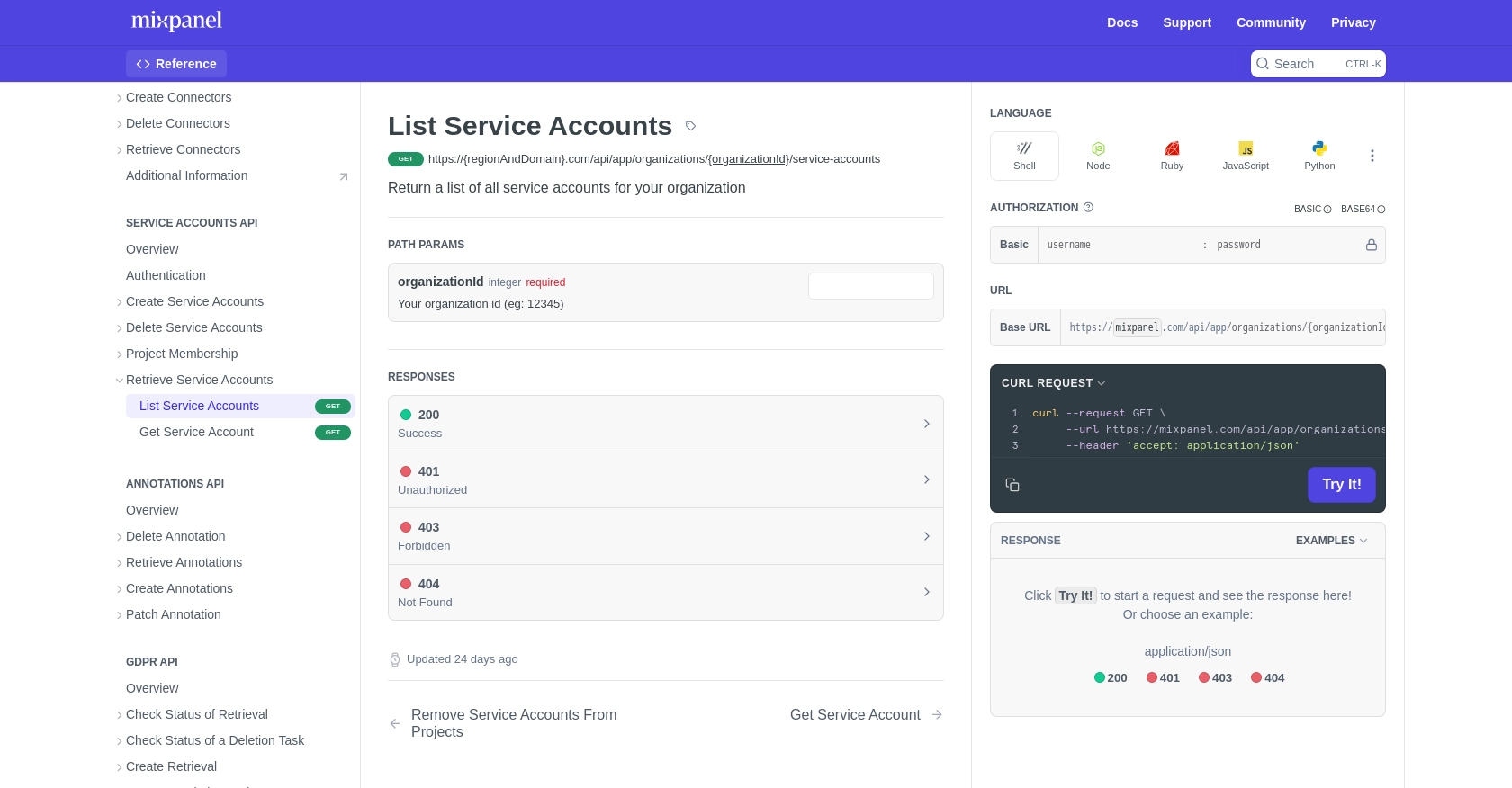
Conclusion and Best Practices for Mixpanel API Integration
Integrating with the Mixpanel API using PHP allows developers to efficiently manage service accounts and automate data processes. By following the steps outlined in this guide, you can successfully authenticate and retrieve service account details, enhancing your ability to manage access and permissions within your organization.
Best Practices for Secure Mixpanel API Integration
- Secure Storage of Credentials: Always store your service account credentials in a secure location. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mixpanel's rate limits. Implement logic to handle
429 Too Many Requests
errors by spreading out API calls or consolidating queries. For more details, refer to the Mixpanel Rate Limits documentation. - Data Standardization: Ensure that data retrieved from Mixpanel is standardized and transformed as needed to fit your application's requirements.
Enhance Your Integration Strategy with Endgrate
While integrating with Mixpanel's API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?