Using the Nutshell API to Get Users (with Javascript examples)
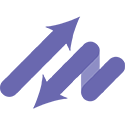
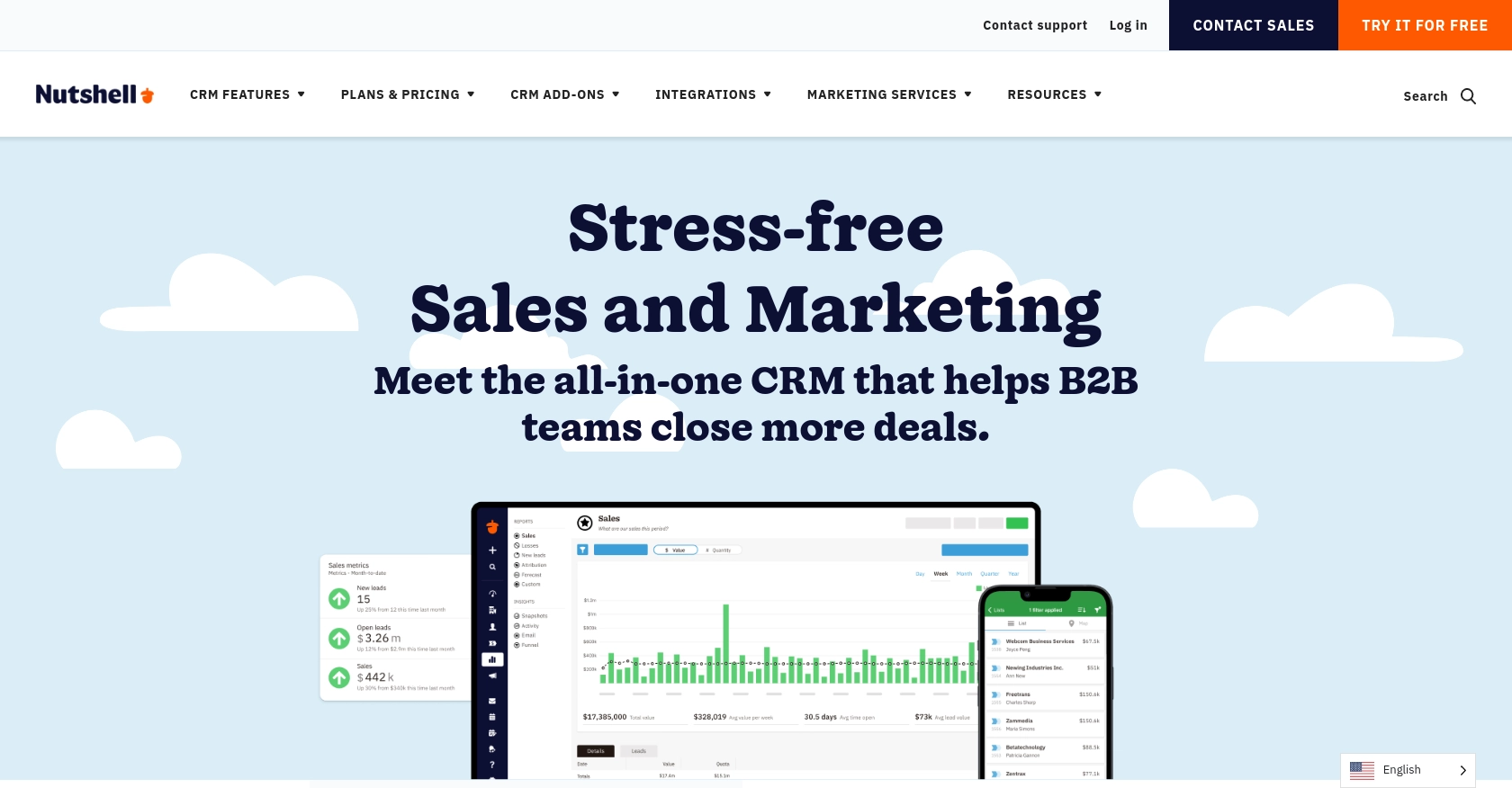
Introduction to Nutshell CRM API
Nutshell is a powerful CRM platform designed to help businesses manage their sales processes efficiently. With features like contact management, lead tracking, and sales automation, Nutshell is a popular choice for companies looking to streamline their customer relationship management.
Developers might want to integrate with the Nutshell API to access and manage user data, enabling seamless synchronization between Nutshell and other business applications. For example, a developer could use the Nutshell API to retrieve user information and integrate it with an internal dashboard, providing real-time insights into team activities and performance.
Setting Up Your Nutshell API Test Account
Before diving into the integration with the Nutshell API, it's essential to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create a sandbox environment for your development needs.
Creating a Nutshell Account
If you don't already have a Nutshell account, you can sign up for a free trial on the Nutshell website. This trial will provide access to the necessary features to test API interactions.
- Visit the Nutshell website and click on "Start Free Trial."
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating an API Key for Authentication
Once your account is set up, you'll need to generate an API key to authenticate your API requests. Nutshell uses HTTP Basic authentication, where the username is your company's domain or a specific user's email address, and the password is the API key.
- Log in to your Nutshell account.
- Navigate to the "Setup" tab and select "API" from the menu.
- Click on "Create New API Key" and provide a name for your key.
- Ensure the key has the necessary permissions for your intended API interactions.
- Save the API key securely, as you'll need it for authentication in your API calls.
Understanding Nutshell API Authentication
The Nutshell API requires HTTPS for all requests to ensure the security of your API key and other sensitive information. Here's a basic example of how to authenticate using HTTP Basic authentication:
// Example of HTTP Basic authentication with the Nutshell API
const username = 'your-email@example.com';
const apiKey = 'your-api-key';
const authHeader = 'Basic ' + btoa(username + ':' + apiKey);
// Use the authHeader in your API requests
For more details on authentication, refer to the Nutshell API documentation.
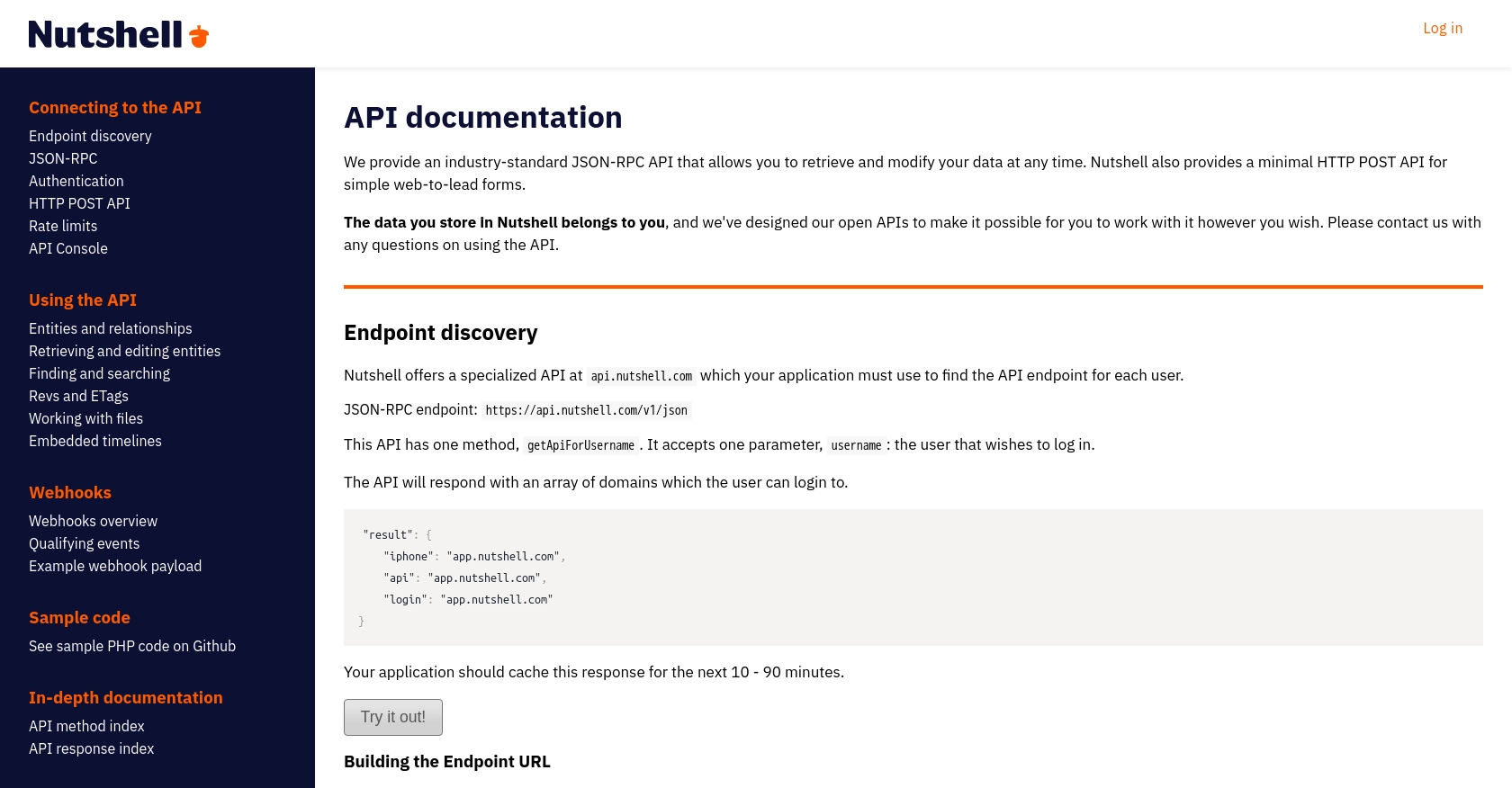
sbb-itb-96038d7
Making API Calls to Retrieve Users from Nutshell Using JavaScript
To interact with the Nutshell API and retrieve user data, you'll need to set up your development environment with the necessary tools and libraries. This section will guide you through the process of making API calls using JavaScript, ensuring you can efficiently access user information from Nutshell.
Setting Up Your JavaScript Environment for Nutshell API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a web browser. Additionally, you'll need the axios
library to handle HTTP requests.
- Download and install Node.js if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library by executingnpm install axios
.
Example Code for Retrieving Users from Nutshell API
With your environment set up, you can now write a JavaScript script to retrieve users from the Nutshell API. The following example demonstrates how to make a GET request to the API endpoint using HTTP Basic authentication.
// Import the axios library
const axios = require('axios');
// Set up authentication details
const username = 'your-email@example.com';
const apiKey = 'your-api-key';
const authHeader = 'Basic ' + Buffer.from(username + ':' + apiKey).toString('base64');
// Define the API endpoint for retrieving users
const endpoint = 'https://app.nutshell.com/api/v1/json';
// Function to get users from Nutshell
async function getUsers() {
try {
const response = await axios.post(endpoint, {
method: 'findUsers',
params: {},
id: '1'
}, {
headers: {
'Authorization': authHeader,
'Content-Type': 'application/json'
}
});
// Log the user data
console.log(response.data.result);
} catch (error) {
console.error('Error retrieving users:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getUsers();
In this script, we use the axios
library to send a POST request to the Nutshell API. The request includes the findUsers
method, which retrieves user data. Ensure you replace your-email@example.com
and your-api-key
with your actual credentials.
Verifying Successful API Requests and Handling Errors
After executing the script, you should see a list of users printed in the console. To verify the request's success, check the response data for user details. If the request fails, the error message will be logged, helping you diagnose issues such as incorrect authentication details or network problems.
For more information on handling errors and understanding response codes, refer to the Nutshell API documentation.
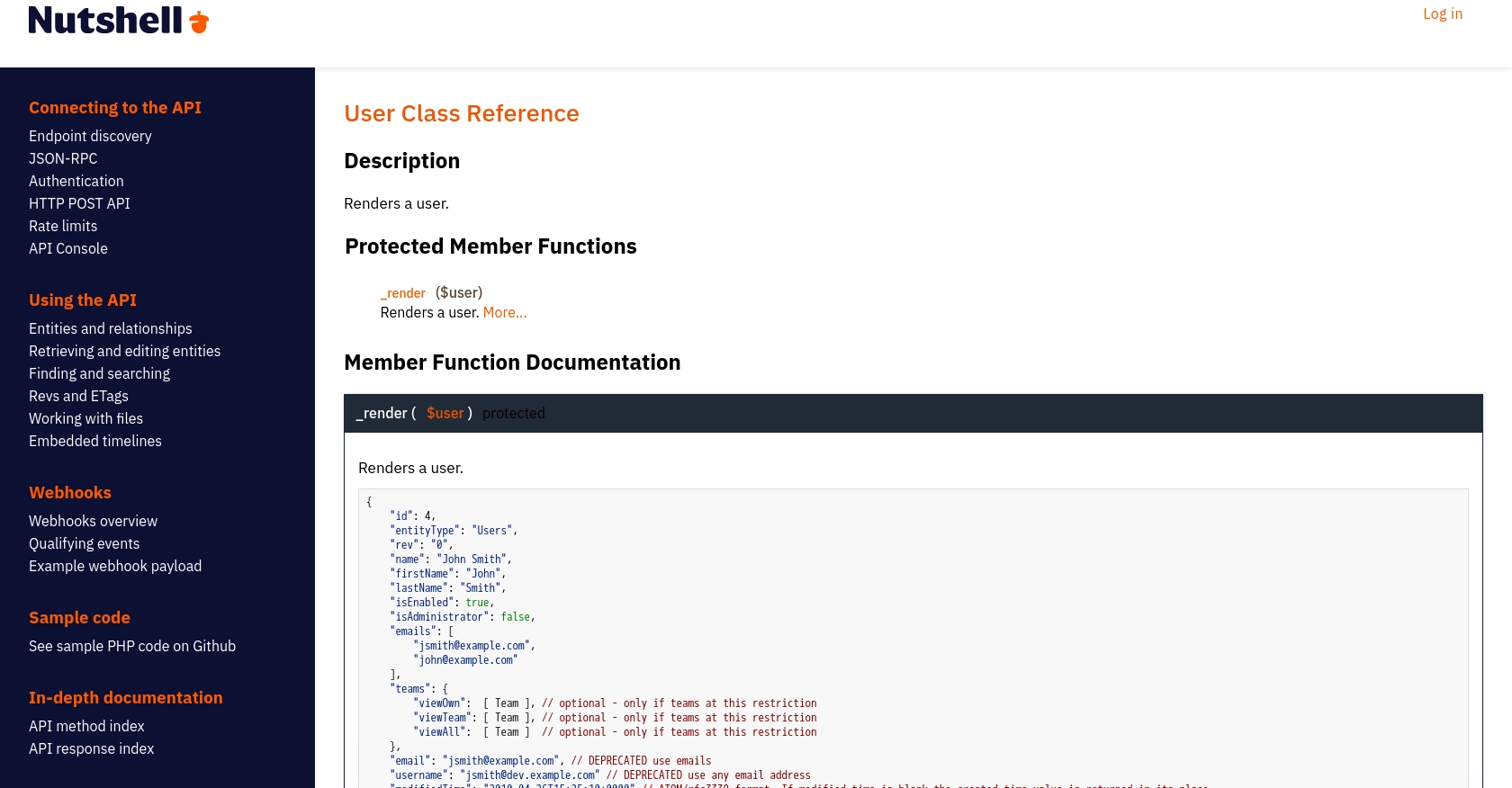
Best Practices for Using the Nutshell API
When integrating with the Nutshell API, it's crucial to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store Credentials: Always store your API keys and user credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the Nutshell API documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application. This will help maintain data integrity across different systems.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and provide user-friendly messages where applicable.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including Nutshell. This allows you to focus on your core product while Endgrate handles the integration complexities.
By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?