How to Create or Update Companies with the Zoho CRM API in PHP
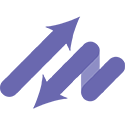
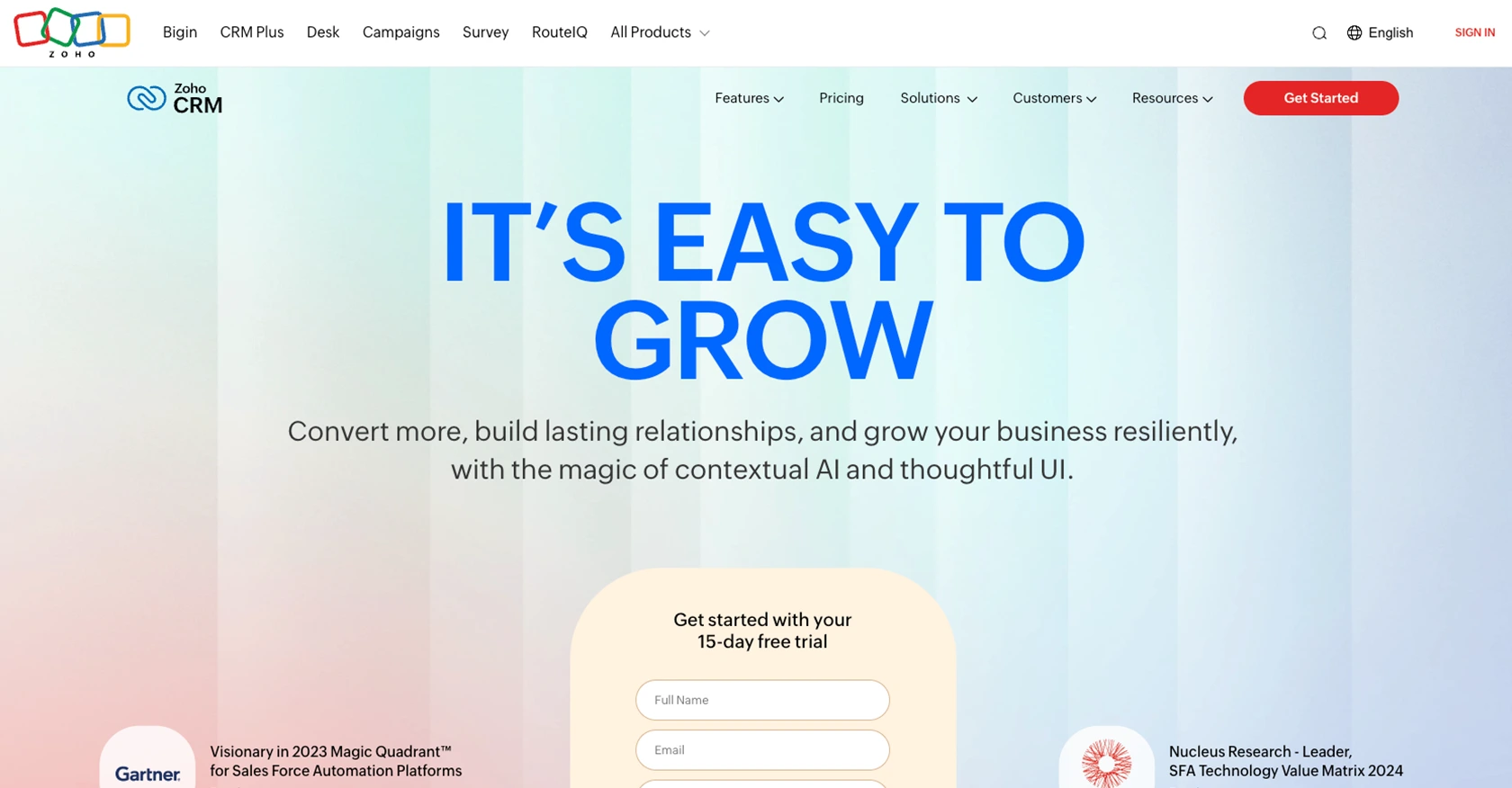
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in a unified system. Known for its flexibility and robust features, Zoho CRM is a popular choice for organizations looking to streamline their customer interactions and improve their sales processes.
Integrating with Zoho CRM's API allows developers to automate and enhance various business operations. For example, you can create or update company records programmatically, ensuring that your CRM data remains accurate and up-to-date. This is particularly useful for businesses that need to synchronize data between Zoho CRM and other systems, such as ERP or marketing platforms.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you begin integrating with the Zoho CRM API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Create a Zoho CRM Developer Account
To get started, you'll need a Zoho CRM developer account. Follow these steps:
- Visit the Zoho Developer Console.
- Sign up for a free account if you don't already have one.
- Once registered, log in to access the developer console.
Register Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application. Here's how:
- In the Zoho Developer Console, select "Register Client".
- Choose "Web Based" as the client type.
- Enter the following details:
- Client Name: A name for your application.
- Homepage URL: The URL of your website.
- Authorized Redirect URIs: The URL where Zoho will redirect after authentication.
- Click "Create" to generate your Client ID and Client Secret.
Generate OAuth Tokens
With your application registered, you can now generate OAuth tokens:
- Direct users to the Zoho authorization URL with your Client ID and requested scopes.
- Upon user consent, Zoho will redirect to your specified URI with an authorization code.
- Exchange this code for access and refresh tokens by making a POST request to Zoho's token endpoint.
POST /oauth/v2/token
Host: accounts.zoho.com
Content-Type: application/x-www-form-urlencoded
client_id=Your_Client_ID&client_secret=Your_Client_Secret&grant_type=authorization_code&redirect_uri=Your_Redirect_URI&code=Authorization_Code
Store the access and refresh tokens securely, as they will be used to authenticate API requests.
Test Your Integration in the Sandbox
With your OAuth tokens ready, you can now test API calls in the sandbox environment:
- Use the access token in the Authorization header of your API requests.
- Verify the responses and ensure that your integration behaves as expected.
By following these steps, you can set up a secure and isolated environment to develop and test your Zoho CRM integrations.
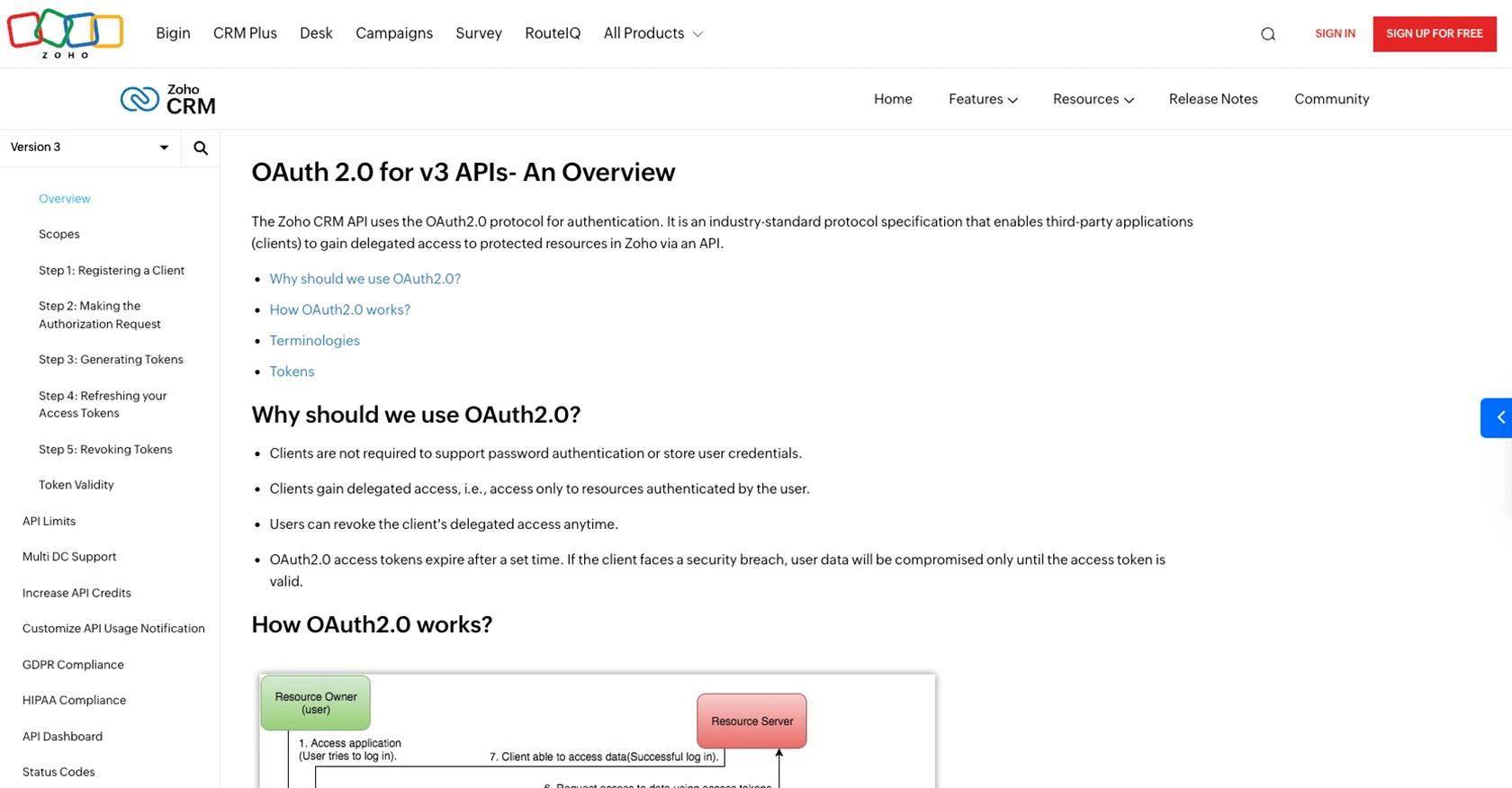
sbb-itb-96038d7
Making API Calls to Create or Update Companies in Zoho CRM Using PHP
To interact with the Zoho CRM API for creating or updating company records, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Zoho CRM API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP 7.4 or higher.
- Ensure the
cURL
extension is enabled in yourphp.ini
file. - Install Composer to manage dependencies.
Installing Required PHP Dependencies
Use Composer to install the guzzlehttp/guzzle
library, which simplifies HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Companies in Zoho CRM
Here's a sample PHP script to create or update a company record in Zoho CRM:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$apiUrl = 'https://www.zohoapis.com/crm/v3/Accounts';
$data = [
'data' => [
[
'Account_Name' => 'New Company',
'Website' => 'https://newcompany.com',
'Industry' => 'Technology'
]
]
];
$response = $client->request('POST', $apiUrl, [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $data
]);
echo $response->getBody();
Handling API Responses and Errors
After making the API call, you'll receive a response that indicates whether the operation was successful:
- Check the HTTP status code. A 200 status indicates success.
- Parse the JSON response to verify the operation's result.
- Handle errors by checking for specific error codes and messages. Refer to the Zoho CRM status codes documentation for more details.
Verifying Changes in Zoho CRM Sandbox
To ensure your API calls are successful, verify the changes in your Zoho CRM sandbox:
- Log in to your Zoho CRM sandbox account.
- Navigate to the "Accounts" module to see the newly created or updated company records.
By following these steps, you can efficiently create or update company records in Zoho CRM using PHP, ensuring your CRM data is always current and accurate.
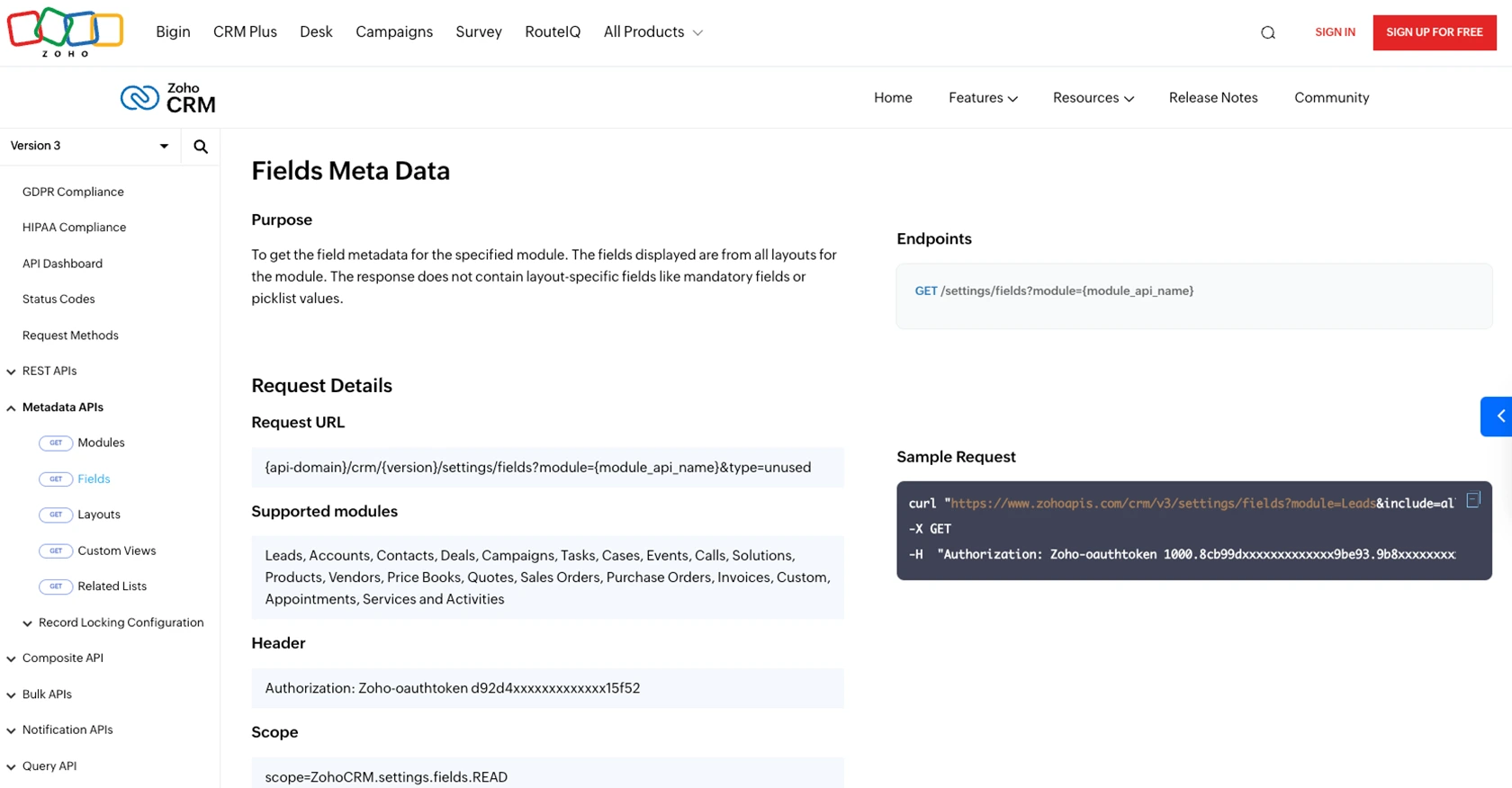
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using PHP provides a powerful way to automate and streamline your business processes. By creating or updating company records programmatically, you ensure that your CRM data is always accurate and up-to-date, which is crucial for effective customer relationship management.
Best Practices for Secure and Efficient Zoho CRM API Integration
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid exposing them in public repositories or client-side code to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Zoho CRM's API rate limits. Implement logic to handle rate limiting gracefully to avoid disruptions in your integration. Refer to the Zoho CRM API limits documentation for more details.
- Standardize Data Fields: Ensure that data fields are standardized and consistent across your systems to prevent data discrepancies and improve data quality.
- Error Handling: Implement robust error handling to manage API errors effectively. Use the Zoho CRM status codes to identify and resolve issues promptly.
Streamlining Integrations with Endgrate
For businesses looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Zoho CRM. By using Endgrate, you can save time and resources, allowing your team to focus on core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?