How to Get Customers with the Sap Business One API in Python
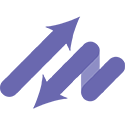
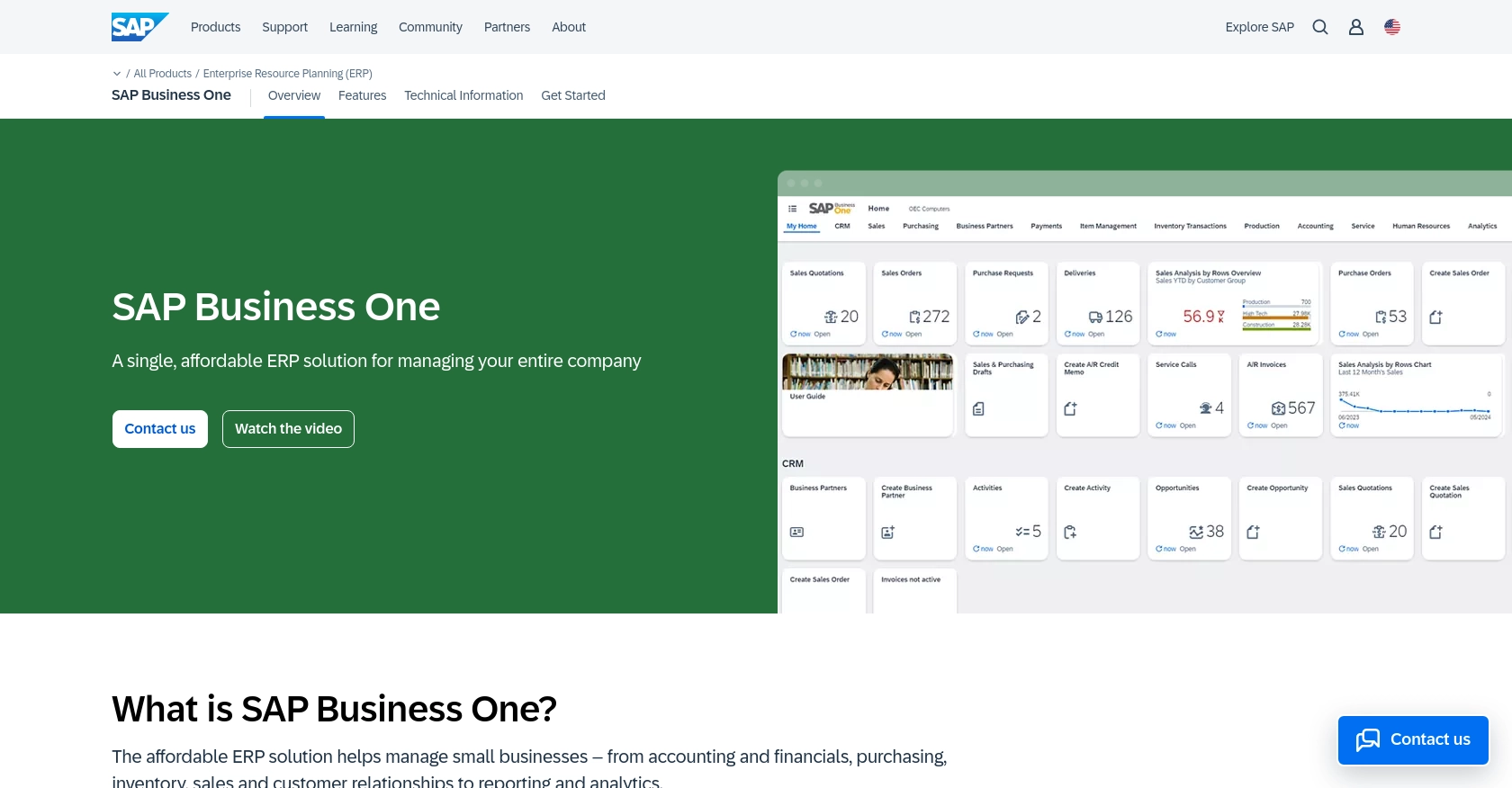
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed specifically for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain operations, all within a single platform.
For developers, integrating with the SAP Business One API can significantly enhance business processes by automating tasks and improving data accessibility. For example, you might want to retrieve customer data to synchronize with a CRM system, enabling better customer insights and personalized service offerings.
Setting Up Your SAP Business One Test or Sandbox Account
Before you can start integrating with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a test environment from your system administrator. If not, you may need to contact SAP directly to inquire about trial or sandbox options.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer section.
- Create a new application to generate your client ID and client secret.
- Ensure that you have the necessary permissions to access customer data.
Once you have your client ID and client secret, you can use them to authenticate your API requests.
Generating API Keys for SAP Business One
If your integration requires API key-based access, follow these steps to generate your API key:
- Go to the API Management section in your SAP Business One account.
- Select Create API Key and follow the prompts to generate a new key.
- Store the API key securely, as you will need it for making API calls.
With your sandbox account and authentication credentials set up, you're ready to start making API calls to retrieve customer data using Python.
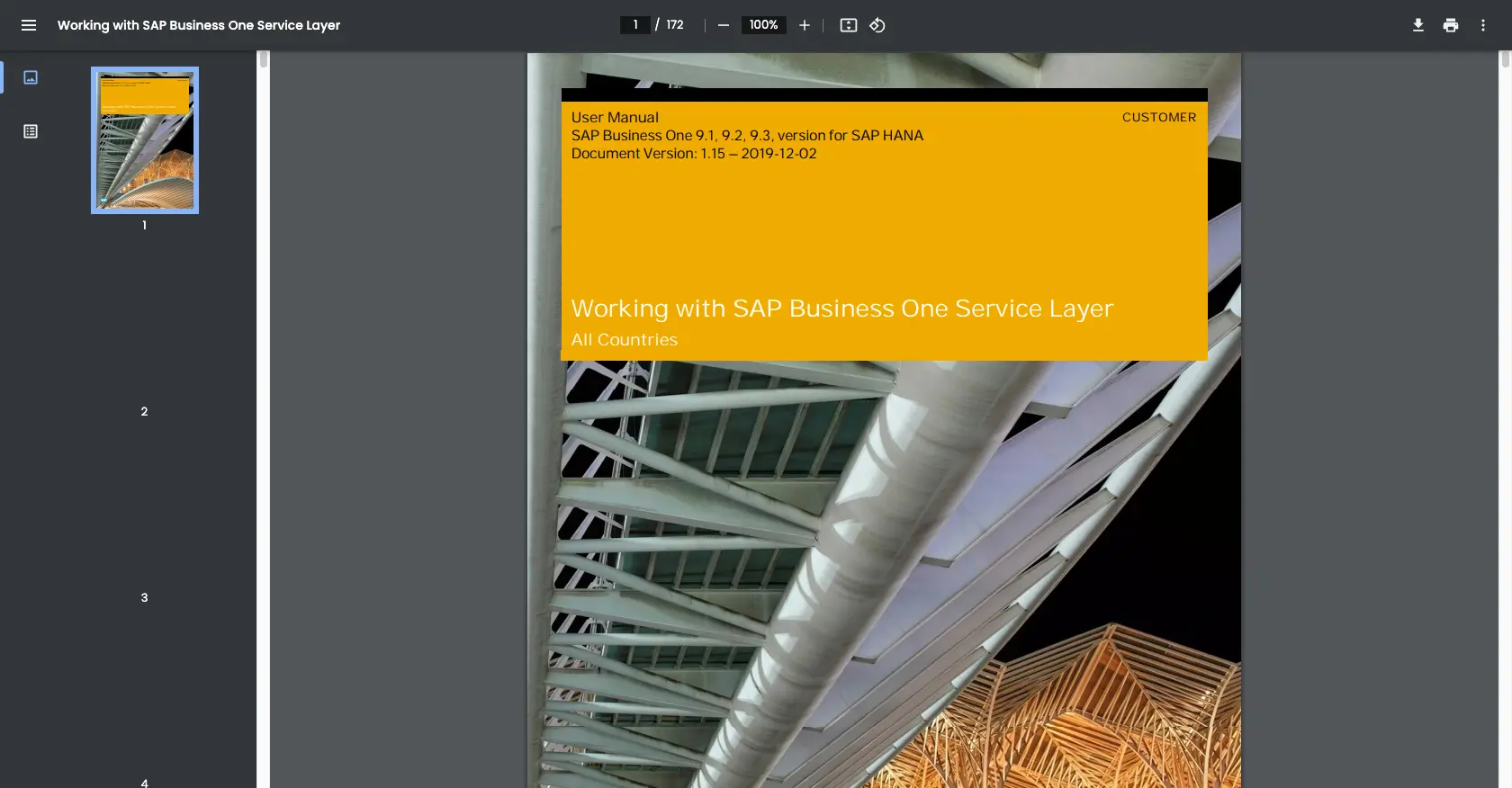
sbb-itb-96038d7
Making API Calls to Retrieve Customers from SAP Business One Using Python
To interact with the SAP Business One API and retrieve customer data, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Retrieve Customer Data from SAP Business One
Create a new Python file named get_sap_customers.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://your-sap-business-one-url.com/b1s/v1/BusinessPartners"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
customers = response.json()
for customer in customers['value']:
print(f"Customer Code: {customer['CardCode']}, Customer Name: {customer['CardName']}")
else:
print(f"Failed to retrieve customers: {response.status_code} - {response.text}")
Replace Your_Token
with the token obtained from your SAP Business One account.
Verifying API Call Success and Handling Errors
Run the script using the following command:
python get_sap_customers.py
If successful, the script will output a list of customer codes and names. If the request fails, the script will print an error message with the status code and response text.
Common error codes include:
- 401 Unauthorized: Check your authentication token.
- 404 Not Found: Verify the API endpoint URL.
- 500 Internal Server Error: Contact SAP support if the issue persists.
For more details, refer to the SAP Business One Service Layer documentation.
Best Practices for Using SAP Business One API in Python
When working with the SAP Business One API, it's essential to follow best practices to ensure secure and efficient integration. Here are some key recommendations:
- Securely Store Credentials: Always store your API keys and tokens securely. Avoid hardcoding them in your scripts. Consider using environment variables or a secure vault service.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from SAP Business One is transformed and standardized to match your application's data model. This will help maintain consistency across systems.
- Error Handling: Implement robust error handling to manage different types of errors, such as network issues or API-specific errors. Log errors for troubleshooting and monitoring purposes.
Streamlining Integrations with Endgrate
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including SAP Business One.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Deploy Anywhere: Create a single integration for each use case and deploy it across multiple platforms.
- Enhance Customer Experience: Offer your customers an intuitive and seamless integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?