How to Get Document Texts with the Google Docs API in Python
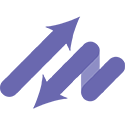
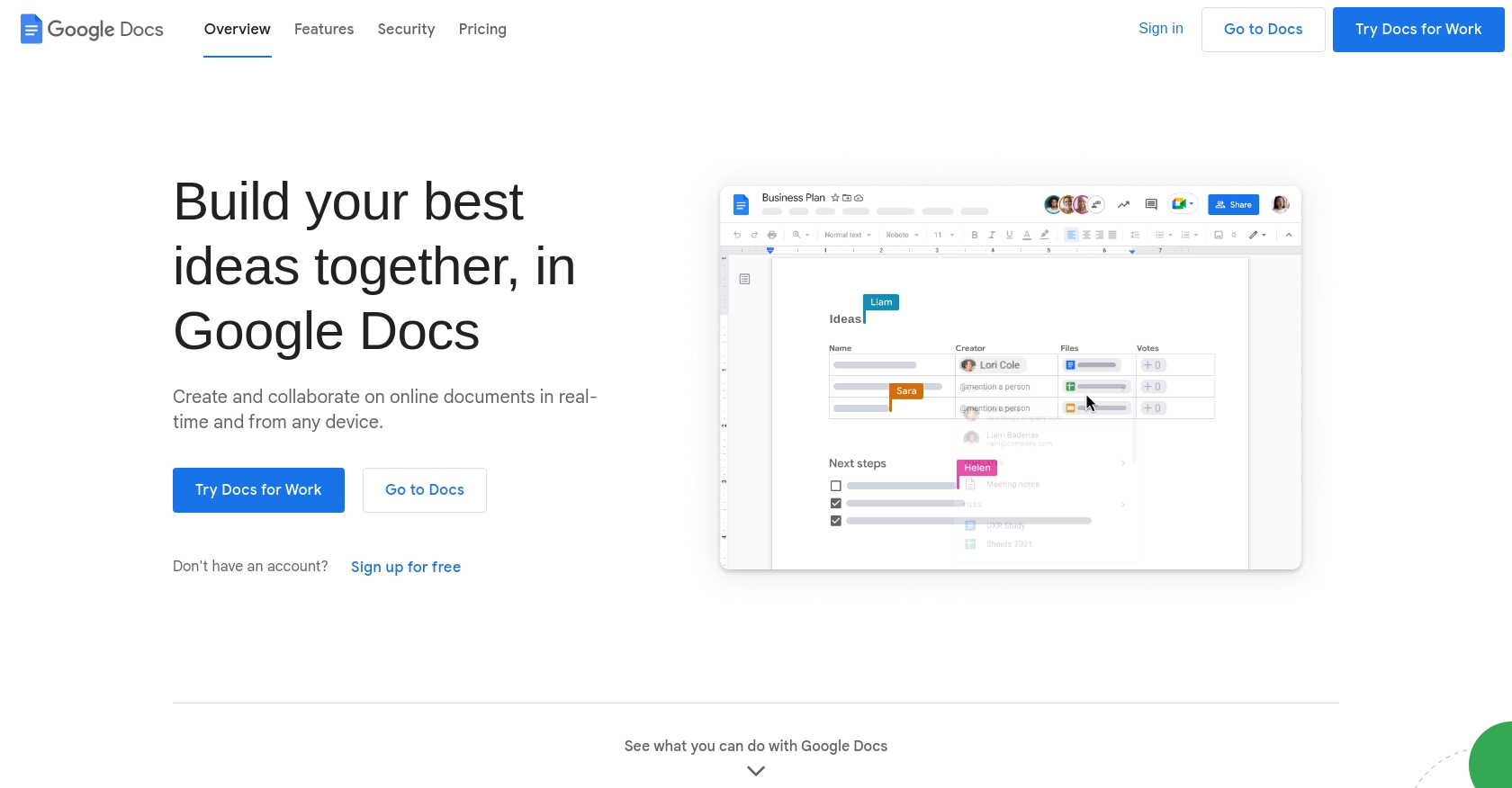
Introduction to Google Docs API
Google Docs is a widely used cloud-based word processing tool that allows users to create, edit, and collaborate on documents in real-time. Its seamless integration with other Google Workspace applications makes it a preferred choice for businesses and individuals alike.
For developers, integrating with the Google Docs API offers the ability to programmatically access and manipulate documents. This can be particularly useful for automating document management tasks, such as extracting text for data analysis or generating reports.
For example, a developer might use the Google Docs API to retrieve the text content of a document and analyze it for specific keywords or phrases, streamlining workflows that involve large volumes of documents.
Setting Up Your Google Docs API Test Environment
Before you can start interacting with the Google Docs API, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access the API and manage your documents programmatically.
Create a Google Cloud Project for Google Docs API
To begin, you'll need a Google Cloud project. Follow these steps to create one:
- Go to the Google Cloud Console.
- Click on the Menu icon, then navigate to IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Docs API in Your Project
Next, you need to enable the Google Docs API for your project:
- In the Google Cloud Console, go to APIs & Services > Library.
- Search for Google Docs API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Docs API Access
Setting up the OAuth consent screen is crucial for managing how users authorize your application:
- In the Google Cloud Console, navigate to APIs & Services > OAuth consent screen.
- Select the user type and click Create.
- Fill out the required fields and click Save and Continue.
Create OAuth 2.0 Credentials for Google Docs API
To access the API, you need to create OAuth 2.0 credentials:
- Go to APIs & Services > Credentials in the Google Cloud Console.
- Click Create Credentials and select OAuth client ID.
- Choose the application type that suits your needs, such as Desktop app, and click Create.
- Note down the Client ID and Client Secret for later use.
With your Google Cloud project and OAuth credentials set up, you're now ready to start making API calls to Google Docs. This setup ensures secure and authorized access to manage your documents programmatically.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Credentials.
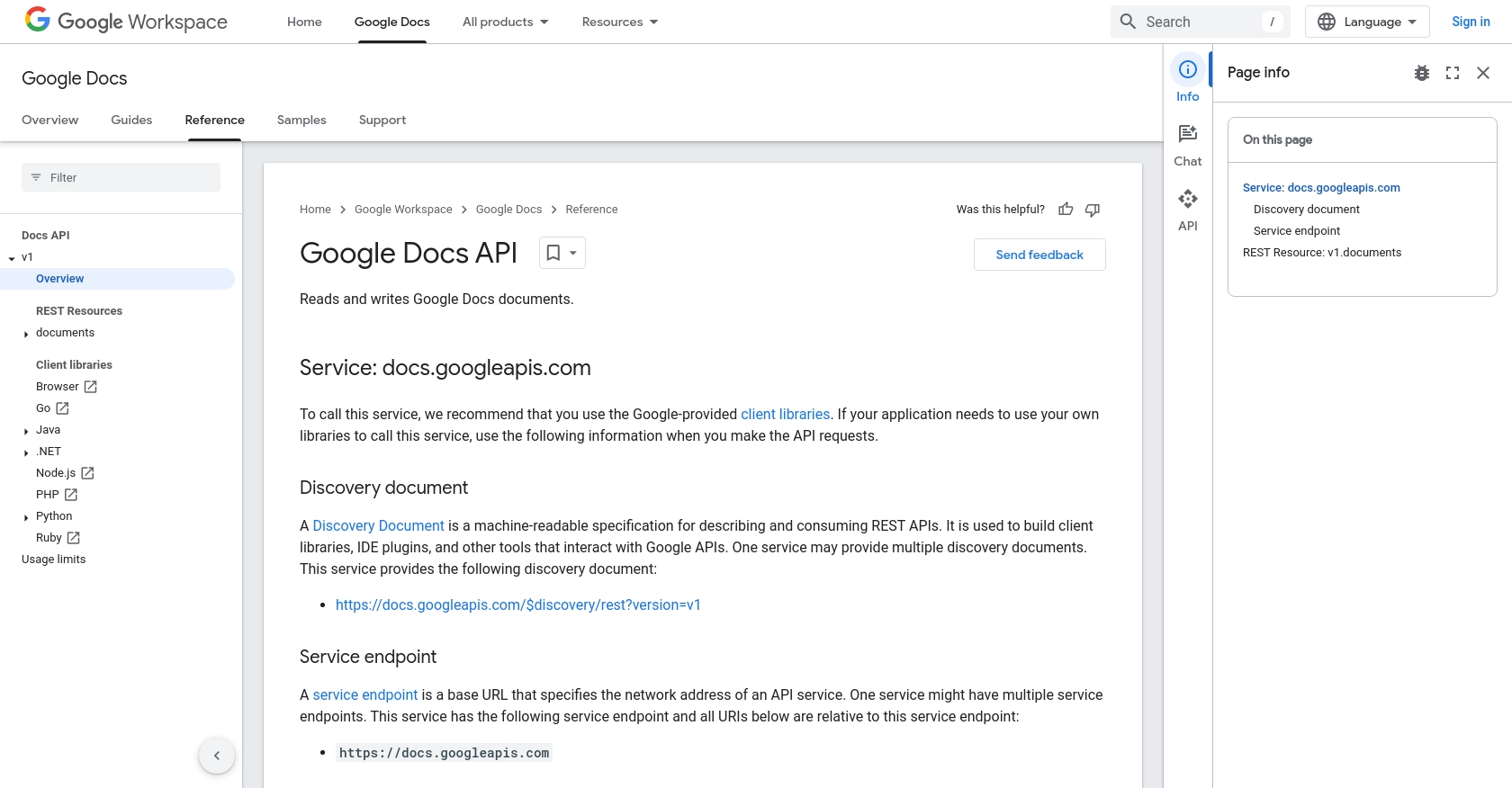
sbb-itb-96038d7
Making API Calls to Retrieve Document Texts with Google Docs API in Python
To interact with the Google Docs API using Python, you'll need to ensure you have the right environment and dependencies set up. This section will guide you through the process of making API calls to retrieve document texts, including setting up Python, installing necessary libraries, and executing the API request.
Setting Up Python Environment for Google Docs API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You can download it from the official Python website.
Additionally, you'll need the google-auth
and google-auth-oauthlib
libraries to handle authentication, and the google-api-python-client
library to interact with the Google Docs API. Install these using pip:
pip install google-auth google-auth-oauthlib google-api-python-client
Executing the Google Docs API Call to Get Document Texts
Once your environment is set up, you can proceed to make the API call. Create a Python script named get_document_text.py
and add the following code:
from google.oauth2 import service_account
from googleapiclient.discovery import build
# Define the scope and credentials
SCOPES = ['https://www.googleapis.com/auth/documents.readonly']
SERVICE_ACCOUNT_FILE = 'path/to/your/service-account-file.json'
# Authenticate and build the service
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=SCOPES)
service = build('docs', 'v1', credentials=credentials)
# Specify the document ID
DOCUMENT_ID = 'your-document-id'
# Make the API call to get the document
document = service.documents().get(documentId=DOCUMENT_ID).execute()
# Extract and print the document text
doc_content = document.get('body').get('content')
for element in doc_content:
if 'paragraph' in element:
for text_run in element['paragraph']['elements']:
print(text_run['textRun']['content'])
Replace path/to/your/service-account-file.json
with the path to your service account JSON file and your-document-id
with the ID of the Google Doc you wish to access.
Verifying the API Call and Handling Errors
Run the script using the following command:
python get_document_text.py
If successful, the script will output the text content of the specified Google Doc. If there are any errors, ensure your document ID is correct and that your service account has the necessary permissions.
For error handling, you can catch exceptions and print error messages:
try:
# API call code here
except Exception as e:
print(f"An error occurred: {e}")
For more information on error codes and handling, refer to the Google Docs API documentation.
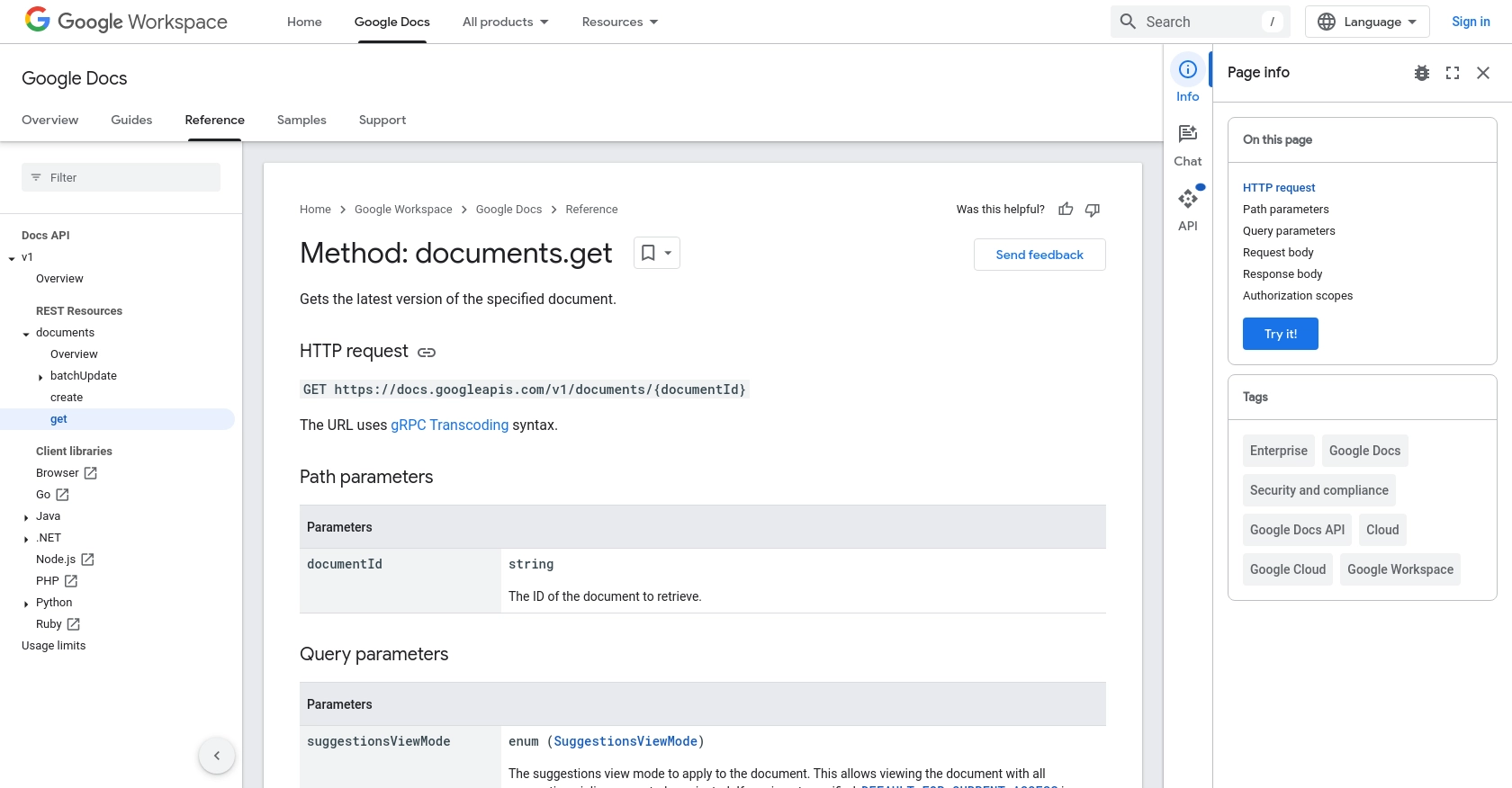
Conclusion and Best Practices for Using Google Docs API in Python
Integrating with the Google Docs API using Python provides developers with powerful capabilities to automate and manage document workflows. By following the steps outlined in this guide, you can efficiently retrieve document texts and leverage them for various applications, such as data analysis and report generation.
Best Practices for Secure and Efficient Google Docs API Integration
- Securely Store Credentials: Always keep your OAuth credentials and service account keys secure. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the Google Docs API rate limits to avoid disruptions. Implement exponential backoff strategies to handle retries gracefully.
- Optimize Data Processing: When dealing with large documents, consider processing data in chunks to improve performance and reduce memory usage.
- Regularly Update Dependencies: Keep your Python libraries up to date to benefit from the latest features and security patches.
Streamline Your Integration Process with Endgrate
While integrating with the Google Docs API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Docs. This allows you to build once for each use case and focus on your core product, saving time and resources.
Explore how Endgrate can enhance your integration experience by visiting Endgrate and discover how leading companies streamline their integrations efficiently.
Read More
- https://endgrate.com/provider/googledocs
- https://developers.google.com/docs/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/docs/api/reference/rest/v1/documents/get
Ready to get started?