How to Create Or Update Sales Orders with the Quickbooks API in PHP
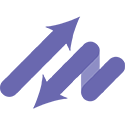
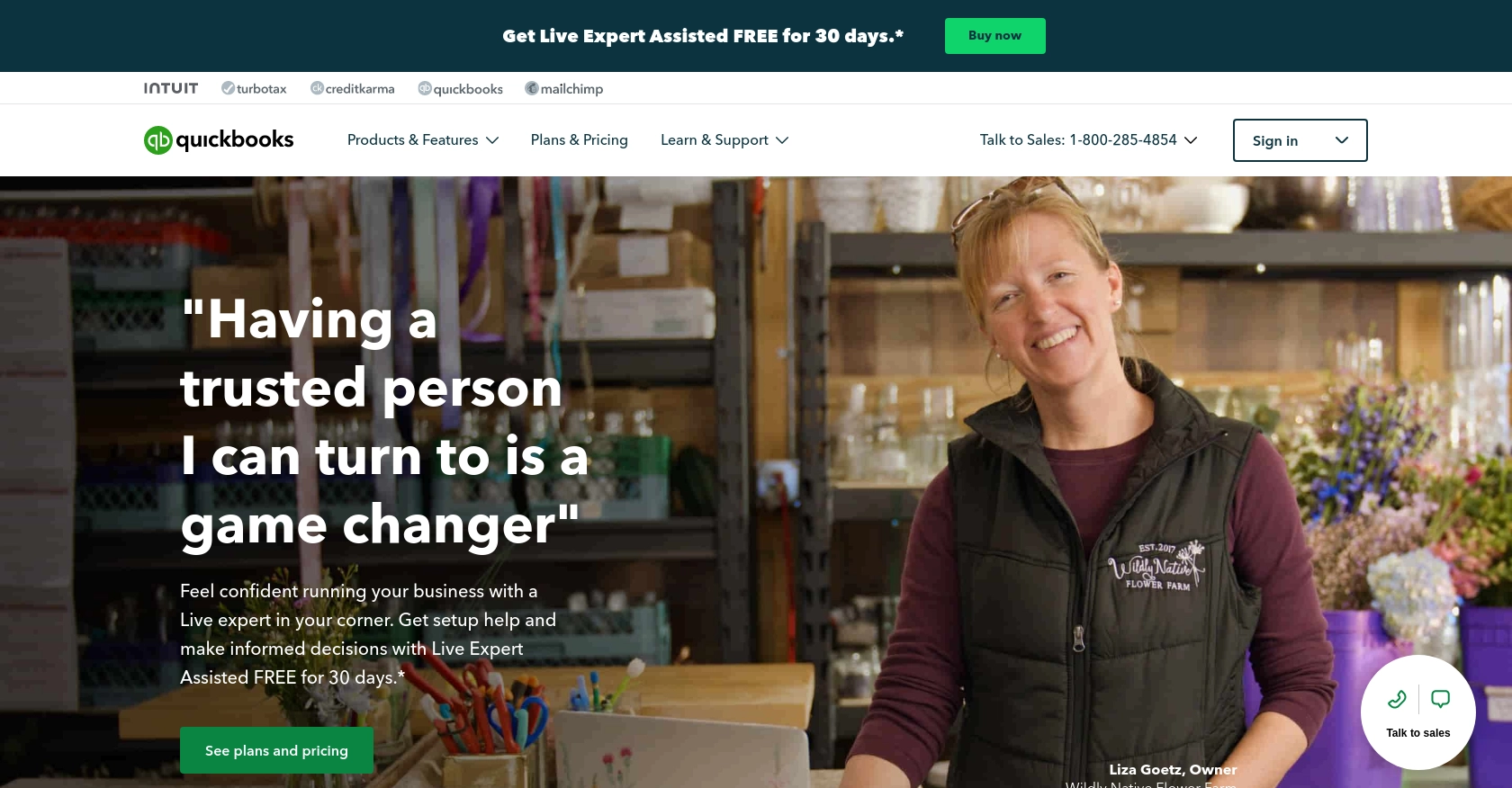
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers a robust set of tools for managing financial operations, including invoicing, payroll, and sales orders. It is a preferred choice for businesses looking to streamline their accounting processes and maintain accurate financial records.
Integrating with the QuickBooks API allows developers to automate and enhance financial workflows by creating or updating sales orders directly from their applications. For example, a developer might use the QuickBooks API to automatically generate sales orders from an e-commerce platform, ensuring seamless order management and accurate inventory tracking.
Setting Up Your QuickBooks Test/Sandbox Account for API Integration
Before you can start creating or updating sales orders with the QuickBooks API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your integration without affecting live data.
Step 1: Sign Up for a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. If you don't have one, visit the Intuit Developer Portal and sign up. This account will give you access to the QuickBooks sandbox environment and other developer resources.
Step 2: Create a QuickBooks Sandbox Company
Once your developer account is set up, create a sandbox company. This sandbox acts as a testing ground where you can simulate real-world scenarios without impacting actual business data.
- Log in to your QuickBooks Developer account.
- Navigate to the "Sandbox" section.
- Click on "Create Sandbox" and follow the prompts to set up your sandbox company.
Step 3: Obtain OAuth Credentials
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to get your client ID and client secret. Follow these steps:
- Go to the App Management section in your developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required details and save your app.
- Navigate to the "Keys & OAuth" tab to find your client ID and client secret.
Step 4: Configure App Settings
Ensure your app is configured correctly to interact with the QuickBooks API:
- In the "App Settings" section, set the redirect URI to a valid endpoint in your application.
- Enable the necessary scopes for accessing sales orders and other relevant data.
For more detailed instructions, refer to the QuickBooks App Settings Documentation.
With your sandbox account and OAuth credentials ready, you're all set to start integrating with the QuickBooks API using PHP. In the next section, we'll walk through making API calls to create or update sales orders.
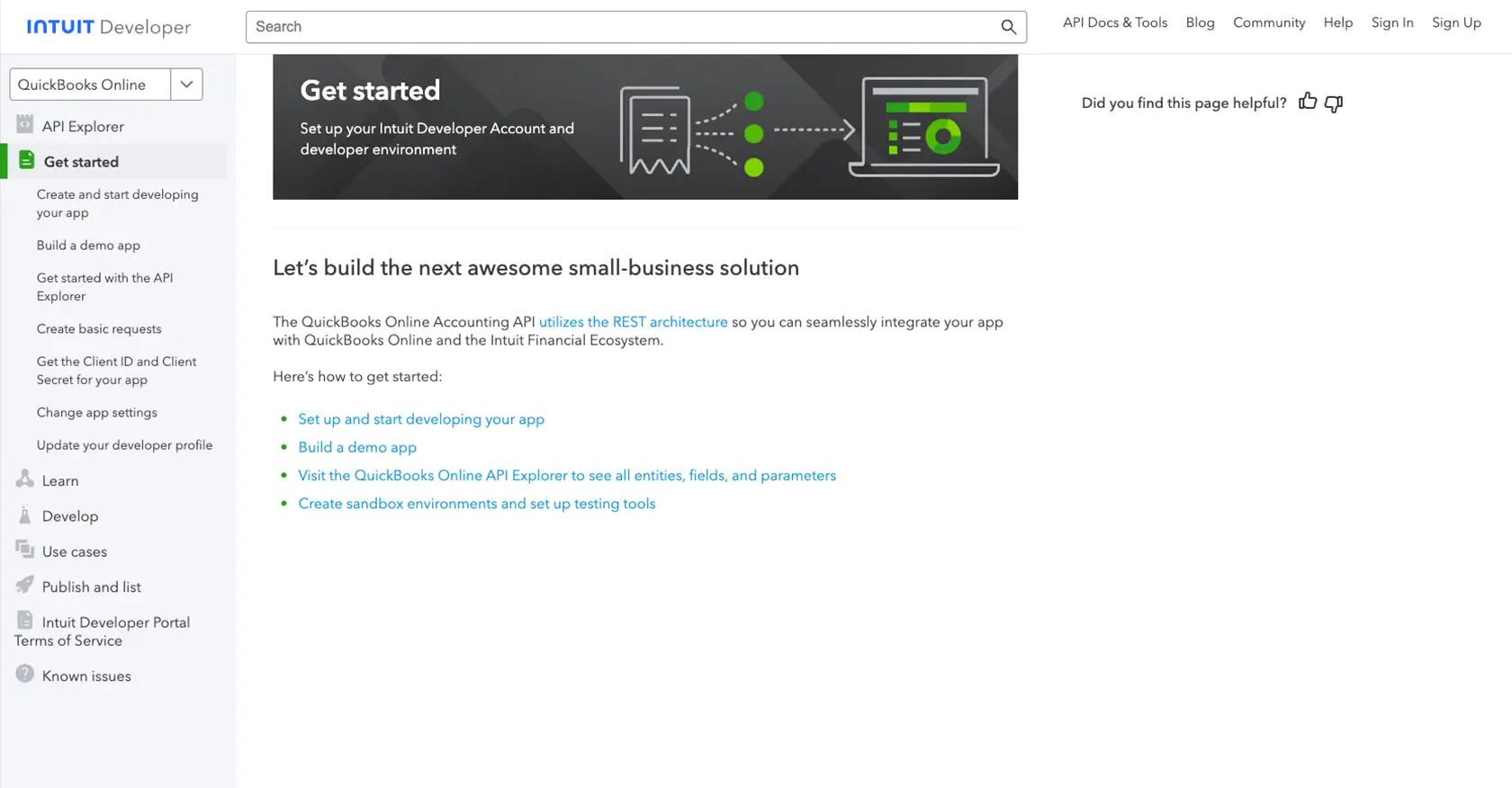
sbb-itb-96038d7
Making API Calls to Create or Update Sales Orders with QuickBooks API in PHP
With your QuickBooks sandbox account and OAuth credentials set up, you can now proceed to make API calls to create or update sales orders using PHP. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for QuickBooks API Integration
Before diving into the code, ensure your development environment is ready:
- Install PHP version 7.4 or later.
- Ensure you have Composer installed for managing dependencies.
- Install the
guzzlehttp/guzzle
package for making HTTP requests by running the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Sales Orders in QuickBooks
Now, let's write the PHP code to interact with the QuickBooks API. This example demonstrates how to create a sales order:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$realmId = 'Your_Realm_ID';
$url = "https://sandbox-quickbooks.api.intuit.com/v3/company/$realmId/salesreceipt";
$headers = [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json',
'Content-Type' => 'application/json'
];
$salesOrderData = [
'Line' => [
[
'Amount' => 100.00,
'DetailType' => 'SalesItemLineDetail',
'SalesItemLineDetail' => [
'ItemRef' => [
'value' => '1',
'name' => 'Sample Item'
]
]
]
],
'CustomerRef' => [
'value' => '1'
]
];
$response = $client->post($url, [
'headers' => $headers,
'json' => $salesOrderData
]);
if ($response->getStatusCode() == 200) {
echo "Sales Order Created Successfully";
} else {
echo "Failed to Create Sales Order";
}
Replace Your_Access_Token
and Your_Realm_ID
with the actual values obtained from your OAuth setup.
Verifying API Call Success and Handling Errors
After running the script, you should verify the success of the API call by checking the QuickBooks sandbox environment. If the sales order is created successfully, it should appear in your sandbox company.
In case of errors, QuickBooks API provides detailed error codes and messages. Handle these errors gracefully in your application. For more information on error codes, refer to the QuickBooks API Documentation.
By following these steps, you can efficiently create or update sales orders in QuickBooks using PHP, streamlining your financial operations and enhancing your application's capabilities.
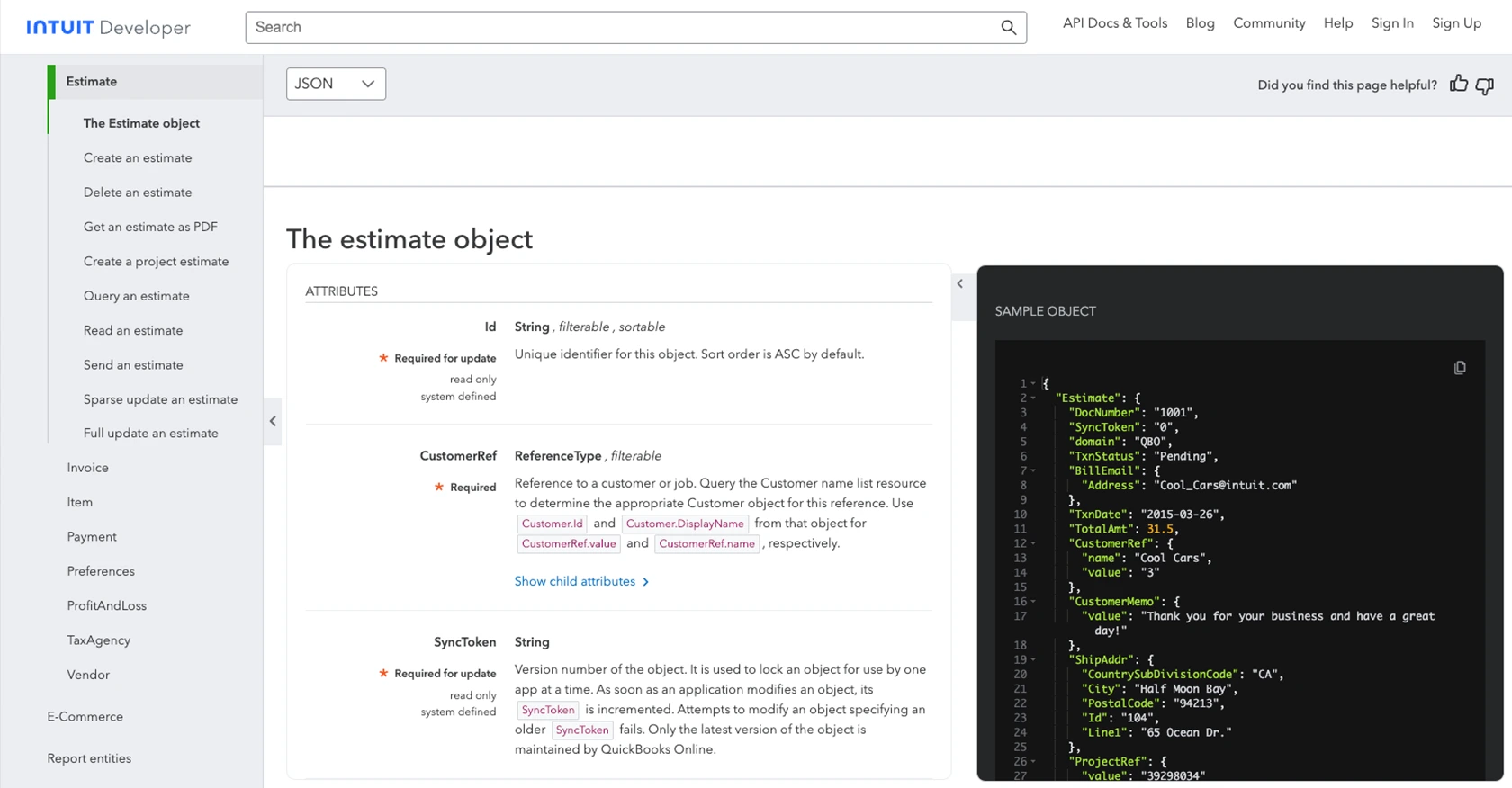
Best Practices for QuickBooks API Integration in PHP
Successfully integrating with the QuickBooks API requires attention to detail and adherence to best practices. Here are some recommendations to ensure a smooth and efficient integration process:
- Securely Store OAuth Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API imposes rate limits to ensure fair usage. Monitor your API calls and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data you send to QuickBooks is consistent and standardized. This helps in maintaining data integrity and reduces the chances of errors.
- Error Handling: Implement robust error handling in your application. Use the error codes and messages provided by QuickBooks API to diagnose and resolve issues effectively.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including QuickBooks.
Endgrate allows you to focus on your core product by outsourcing integrations. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/estimate
Ready to get started?