How to Get Customers with the Stripe API in Javascript
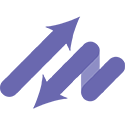
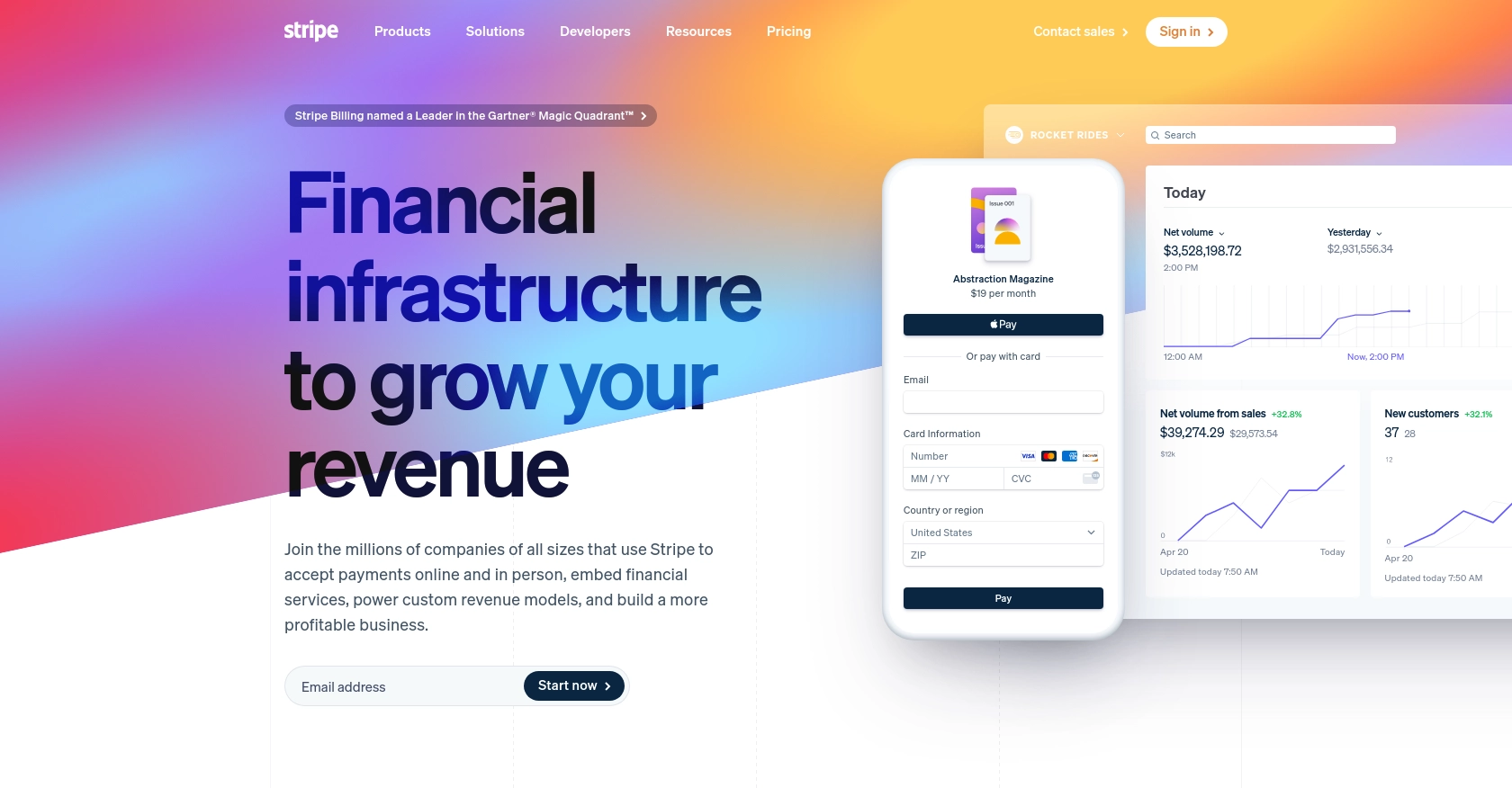
Introduction to Stripe API Integration
Stripe is a powerful payment processing platform that enables businesses to manage online transactions seamlessly. With its robust API, Stripe offers developers the flexibility to integrate payment solutions into their applications, providing a smooth and secure payment experience for users.
Connecting with Stripe's API allows developers to access a wide range of functionalities, including managing customers, processing payments, and handling subscriptions. For example, a developer might use the Stripe API to retrieve customer data and automate billing processes, enhancing operational efficiency and customer satisfaction.
This article will guide you through the process of using JavaScript to interact with the Stripe API, specifically focusing on retrieving customer information. By following these steps, you can streamline your integration efforts and leverage Stripe's capabilities to enhance your application's payment features.
Setting Up Your Stripe Test Account for API Integration
Before you can start interacting with the Stripe API using JavaScript, you'll need to set up a test account. Stripe provides a test mode that allows developers to simulate transactions and API interactions without affecting live data. This is essential for testing and development purposes.
Creating a Stripe Account
If you don't already have a Stripe account, you can sign up for free on the Stripe website. Follow the instructions to create your account. Once your account is set up, you'll have access to the Stripe Dashboard, where you can manage your API keys and other settings.
Accessing the Stripe Dashboard
Log in to your Stripe account and navigate to the Dashboard. Here, you'll find all the tools you need to manage your API keys, monitor transactions, and configure your account settings.
Generating API Keys for Authentication
Stripe uses API keys to authenticate requests. You can find your test API keys in the Dashboard under the "Developers" section:
- Navigate to Developers > API keys.
- Locate the Publishable key and Secret key for test mode. These keys will have the prefix
sk_test_
for secret keys andpk_test_
for publishable keys. - Ensure you keep your secret key secure and do not expose it in client-side code.
Configuring API Key-Based Authentication
To authenticate your API requests, include your secret API key in the request headers. Here's an example of how to set up authentication in JavaScript:
const stripe = require('stripe')('sk_test_yourSecretKeyHere');
Replace sk_test_yourSecretKeyHere
with your actual test secret key from the Stripe Dashboard.
Testing in Sandbox Mode
Stripe's test mode allows you to simulate transactions and API calls without affecting live data. Use the test API keys to perform operations such as creating customers, processing payments, and more. This ensures that your integration works correctly before going live.
By following these steps, you'll be ready to start integrating with the Stripe API using JavaScript, allowing you to test and refine your application's payment features in a safe environment.
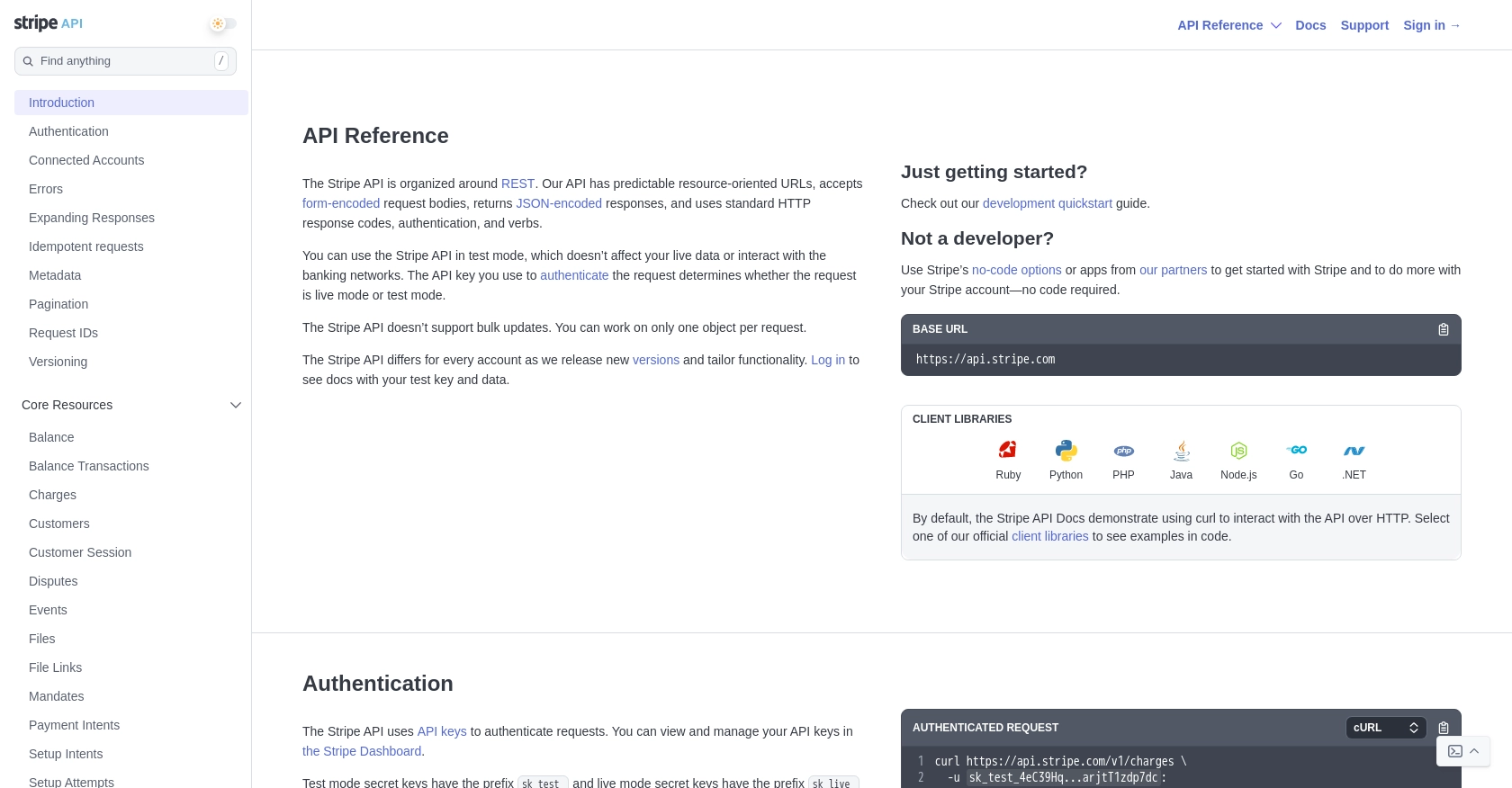
sbb-itb-96038d7
Making API Calls to Retrieve Customers with Stripe API in JavaScript
To interact with the Stripe API using JavaScript, you'll need to make HTTP requests to the appropriate endpoints. This section will guide you through the process of retrieving customer information using the Stripe API.
Setting Up Your JavaScript Environment for Stripe API Integration
Before making API calls, ensure you have Node.js installed on your machine. This tutorial uses Node.js to execute JavaScript code server-side. You can download Node.js from the official website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the Stripe library:
npm install stripe
Writing JavaScript Code to Retrieve Customers from Stripe
Now, let's write the code to fetch customer data from Stripe. Create a new JavaScript file named get_customers.js
and add the following code:
const stripe = require('stripe')('sk_test_yourSecretKeyHere');
// Function to retrieve customers
async function getCustomers() {
try {
const customers = await stripe.customers.list({ limit: 10 });
console.log('Customers:', customers.data);
} catch (error) {
console.error('Error fetching customers:', error);
}
}
// Execute the function
getCustomers();
Replace sk_test_yourSecretKeyHere
with your actual test secret key from the Stripe Dashboard.
Understanding the JavaScript Code for Stripe API Calls
- Importing the Stripe Library: The
require('stripe')
function imports the Stripe library, allowing you to interact with the API. - Authenticating Requests: The secret key is passed to the Stripe library to authenticate your API requests.
- Retrieving Customers: The
stripe.customers.list()
method fetches a list of customers. Thelimit
parameter specifies the number of customers to retrieve. - Error Handling: The
try-catch
block handles any errors that occur during the API call.
Running Your JavaScript Code to Fetch Stripe Customers
To execute your code, run the following command in your terminal:
node get_customers.js
You should see a list of customers printed to the console, confirming that your API call was successful.
Verifying API Call Success and Handling Errors
After running the code, check the output in your terminal. If successful, the customer data will be displayed. If there are errors, ensure your API key is correct and that your network connection is stable.
Stripe uses conventional HTTP response codes to indicate the success or failure of an API request. Refer to the Stripe API documentation for detailed error code explanations and handling strategies.
Conclusion and Best Practices for Integrating Stripe API with JavaScript
Integrating the Stripe API with JavaScript provides a powerful way to manage customer data and streamline payment processes within your application. By following the steps outlined in this guide, you can efficiently retrieve customer information and enhance your application's functionality.
Best Practices for Secure and Efficient Stripe API Integration
- Secure API Keys: Always keep your secret API keys secure and never expose them in client-side code. Use environment variables to manage sensitive information.
- Handle Errors Gracefully: Implement robust error handling to manage API exceptions and provide meaningful feedback to users. Refer to the Stripe API documentation for detailed error handling strategies.
- Manage Rate Limits: Be aware of Stripe's rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests when necessary.
- Optimize Data Handling: Use pagination to manage large datasets efficiently and avoid overloading your application with unnecessary data.
Enhancing Your Integration with Endgrate
While integrating with Stripe directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Stripe. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
Ready to get started?