Using the Outlook API to Send Messages in Javascript
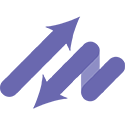
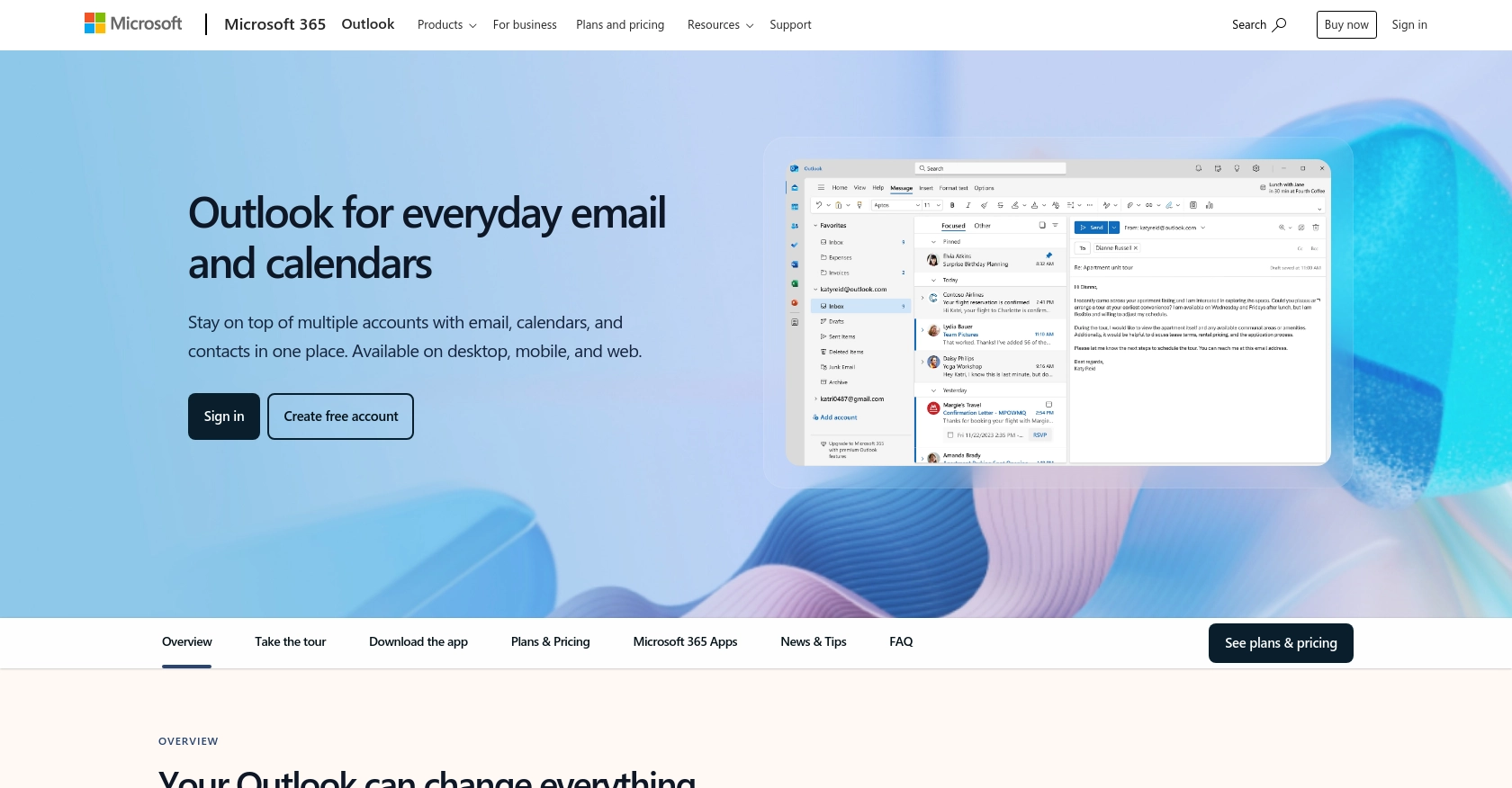
Introduction to Outlook API for Sending Emails
Outlook, part of the Microsoft 365 suite, is a widely used email and calendar service that helps businesses and individuals manage their communications and schedules efficiently. With its robust features and integration capabilities, Outlook is a preferred choice for many organizations.
Developers may want to integrate with the Outlook API to automate email sending processes, enhancing productivity and streamlining communication workflows. For example, a developer could use the Outlook API to send automated notifications or newsletters to a list of subscribers, ensuring timely and consistent communication.
Setting Up Your Outlook API Test Account
Before you can start sending emails using the Outlook API, you'll need to set up a test account. This involves creating a Microsoft Entra ID tenant and registering your application with the Microsoft identity platform. This setup will allow you to authenticate and authorize API requests securely.
Create a Microsoft Entra ID Tenant
If you don't already have a Microsoft Entra ID tenant, you can create one by signing up for a free Azure account. This will give you access to the necessary resources to register and manage your application.
Register Your Application with Microsoft Identity Platform
- Sign in to the Microsoft Entra admin center.
- Navigate to Identity > Applications > App registrations and select New registration.
- Enter a display name for your application.
- Choose the supported account types. For most integrations, select Accounts in any organizational directory and personal Microsoft accounts.
- Click Register to complete the initial registration.
Configure Platform Settings for Your Outlook API App
- Under Manage, select Authentication.
- Under Platform configurations, select Add a platform and choose Single-page application.
- Enter a Redirect URI for your app. This URI is where the Microsoft identity platform will send responses after authentication.
- Click Configure to save your settings.
Add Credentials to Your Outlook API App
To authenticate your application, you'll need to add credentials:
- Select Certificates & secrets and then Client secrets.
- Click New client secret and add a description.
- Select an expiration period and click Add.
- Copy the client secret value as it will be used in your application code.
Set Up Permissions for Outlook API Access
To send emails using the Outlook API, you need to configure permissions:
- Go to API permissions and click Add a permission.
- Select Microsoft Graph and then Delegated permissions.
- Search for and select Mail.Send permission.
- Click Add permissions to apply the changes.
With these steps completed, your application is now set up to interact with the Outlook API. You can proceed to implement the API calls in your JavaScript code.
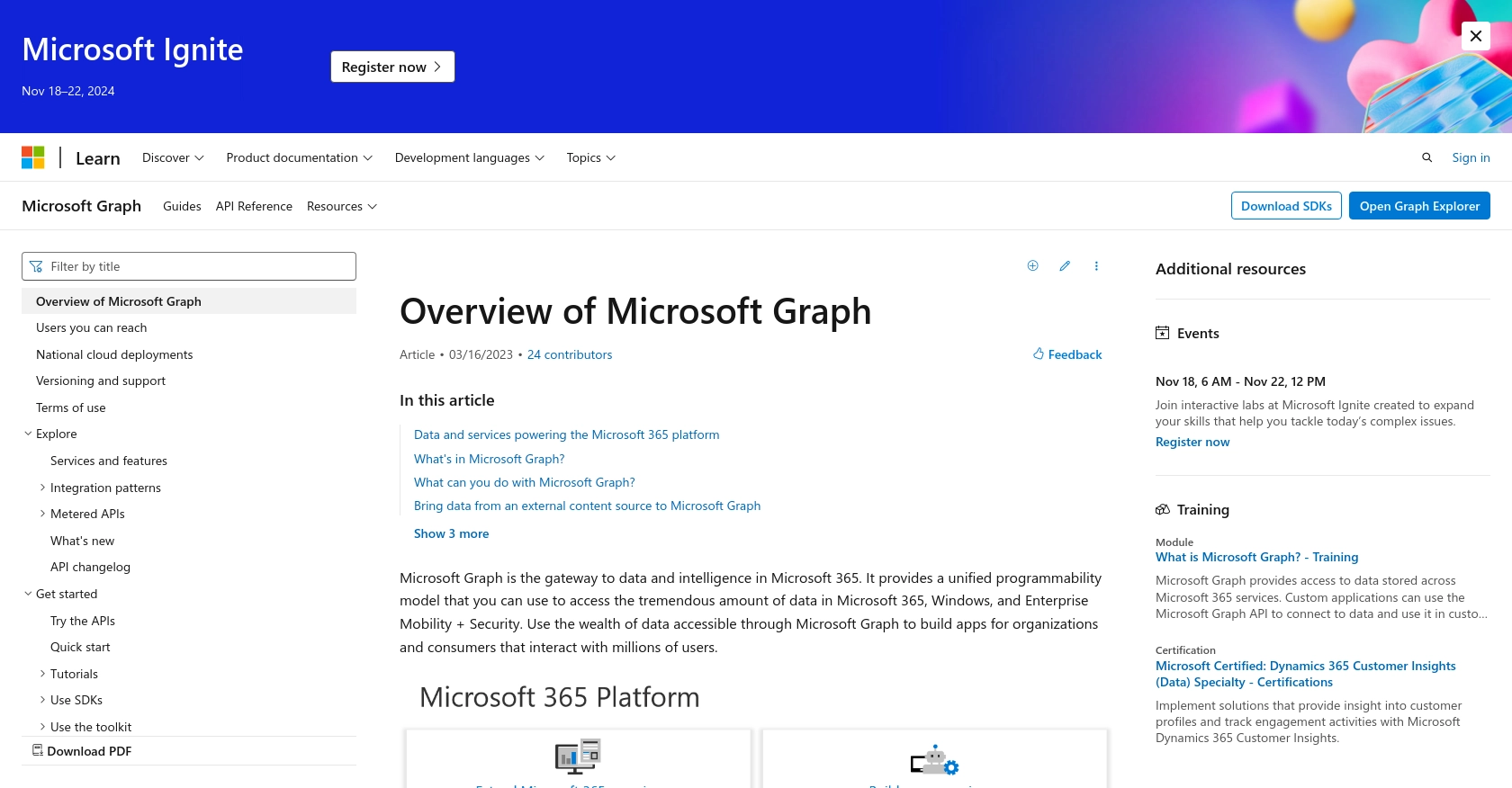
sbb-itb-96038d7
How to Make API Calls to Send Emails Using Outlook API in JavaScript
Setting Up Your JavaScript Environment for Outlook API Integration
To begin sending emails using the Outlook API in JavaScript, ensure you have Node.js installed on your machine. This tutorial uses Node.js for server-side JavaScript execution. You will also need the axios
library to handle HTTP requests.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library by runningnpm install axios
.
Implementing the Outlook API Call to Send Emails
With your environment set up, you can now write the JavaScript code to send emails using the Outlook API. The following example demonstrates how to send an email using the axios
library.
const axios = require('axios');
// Define the API endpoint and access token
const endpoint = 'https://graph.microsoft.com/v1.0/me/sendMail';
const accessToken = 'YOUR_ACCESS_TOKEN';
// Create the email message payload
const emailData = {
message: {
subject: 'Hello from Outlook API',
body: {
contentType: 'Text',
content: 'This is a test email sent using the Outlook API.'
},
toRecipients: [
{
emailAddress: {
address: 'recipient@example.com'
}
}
]
},
saveToSentItems: 'true'
};
// Send the email using axios
axios.post(endpoint, emailData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log('Email sent successfully:', response.status);
})
.catch(error => {
console.error('Error sending email:', error.response.data);
});
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the authentication process. Also, update the toRecipients
field with the desired recipient's email address.
Verifying the Email Sent via Outlook API
After running the script, check the recipient's inbox to verify the email was sent successfully. Additionally, you can log into your Outlook account and check the Sent Items folder to confirm the email was saved there.
Handling Errors and Debugging API Requests
When making API calls, it's crucial to handle potential errors. The example code includes a catch
block that logs any errors encountered during the request. Common errors include invalid access tokens or incorrect request payloads.
Refer to the Microsoft Graph API documentation for detailed information on error codes and troubleshooting tips.
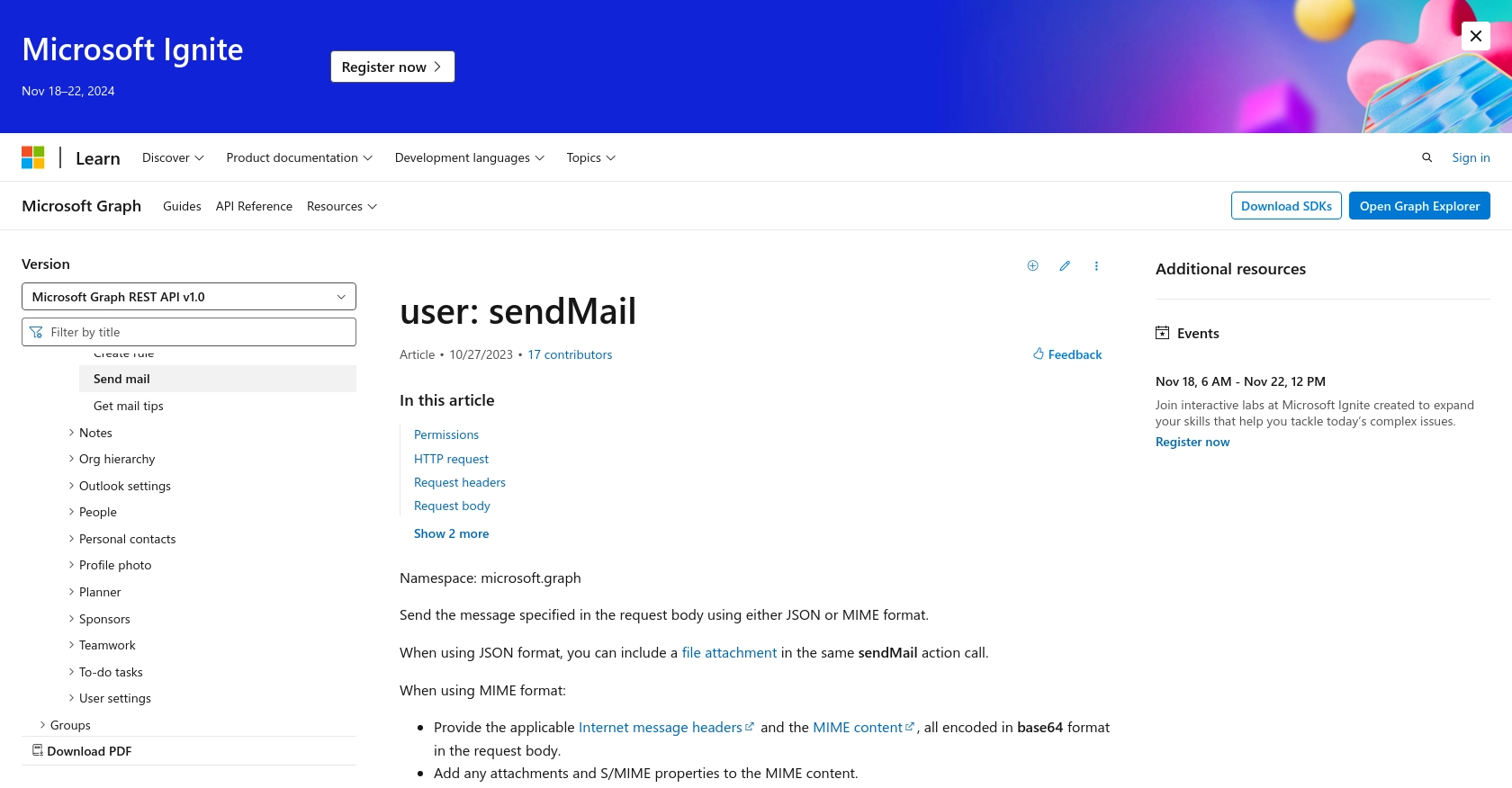
Conclusion and Best Practices for Using Outlook API in JavaScript
Integrating with the Outlook API to send emails using JavaScript can significantly enhance your application's communication capabilities. By automating email processes, you can improve efficiency and ensure timely delivery of important messages.
Best Practices for Secure and Efficient Outlook API Integration
- Securely Store Credentials: Always store your client secrets and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Validate API Responses: Always check the status codes and response data to ensure that your API calls are successful. Implement error handling to manage exceptions and provide meaningful feedback to users.
- Keep Access Tokens Updated: Access tokens are short-lived. Ensure your application can refresh tokens as needed to maintain uninterrupted service.
Transforming and Standardizing Data for Outlook API
When sending emails, ensure that the data, such as email addresses and message content, is validated and standardized. This helps prevent errors and ensures that your communications are consistent and professional.
Leverage Endgrate for Streamlined Integration Solutions
For developers looking to simplify their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing complex integrations, allowing you to focus on your core product. Endgrate provides a unified API experience, enabling you to build once and deploy across multiple platforms effortlessly.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover a more efficient way to manage your API integrations.
Read More
- https://endgrate.com/provider/outlook
- https://docs.microsoft.com/en-us/graph/overview
- https://learn.microsoft.com/en-us/graph/auth/auth-concepts
- https://learn.microsoft.com/en-us/graph/auth-register-app-v2
- https://learn.microsoft.com/en-us/graph/auth-v2-user?tabs=http
- https://learn.microsoft.com/en-us/graph/api/user-sendmail?view=graph-rest-1.0&tabs=http
Ready to get started?