How to Get Notes with the Endear API in Python
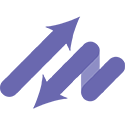
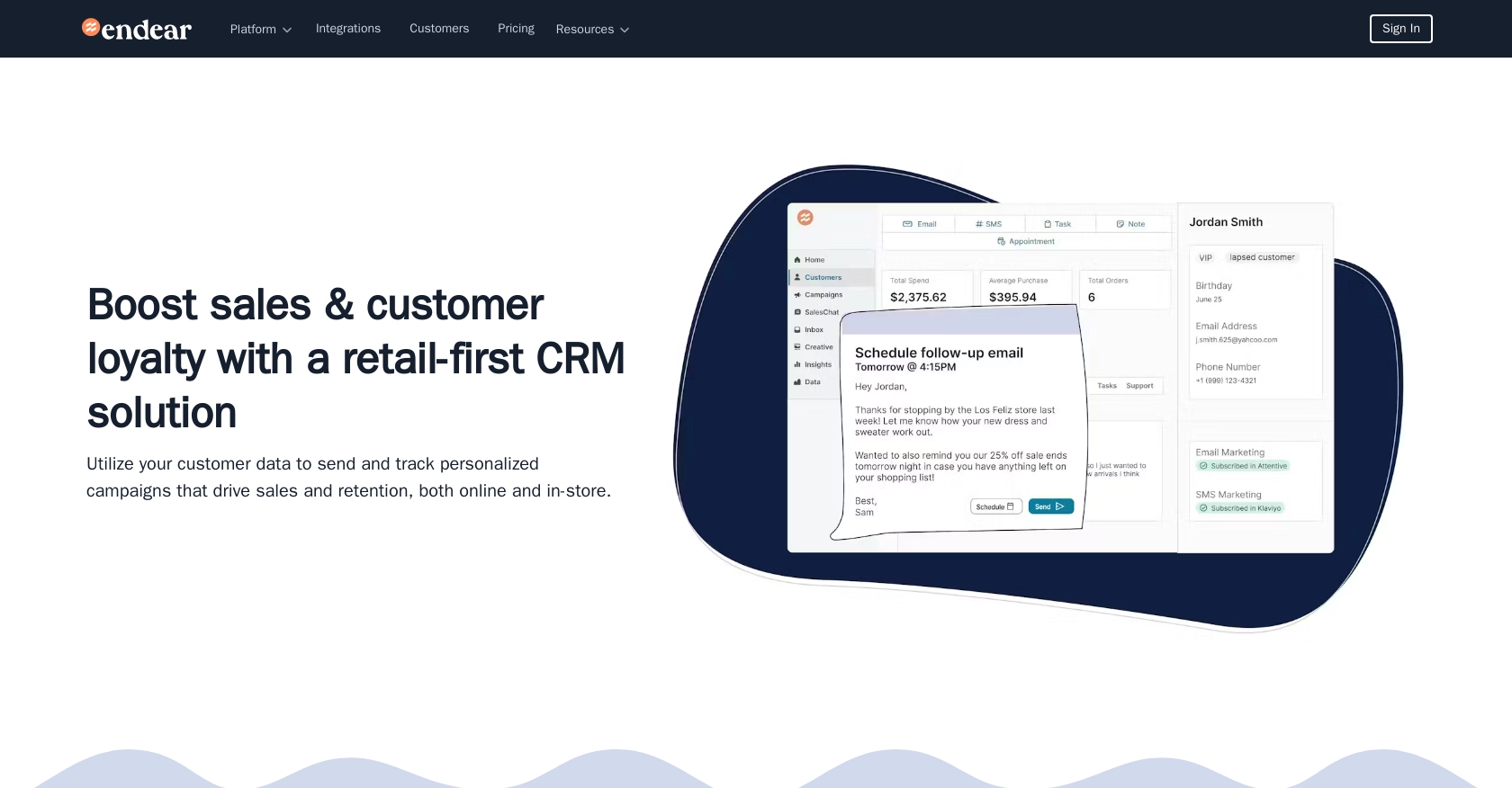
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationships by providing businesses with tools to manage and analyze customer interactions. Its robust features make it an ideal choice for brands looking to personalize their customer engagement strategies.
Developers may want to integrate with Endear's API to access and manage customer notes, which can be crucial for maintaining detailed records of customer interactions. For example, a developer might use the Endear API to retrieve notes related to customer feedback, enabling the business to tailor its services based on customer insights.
Setting Up Your Endear Test Account
Before you can start interacting with the Endear API, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting live data. Endear provides a straightforward process to create an integration and obtain an API key, which is essential for authenticating your requests.
Creating an Endear Integration and Generating an API Key
- Log in to your Endear account. If you don't have one, you can sign up for a free trial on the Endear website.
- Navigate to the Settings section in your Endear dashboard.
- Click on Integrations and then select Add Integration.
- Choose API from the options provided.
- Fill in the required details to create your integration. Once completed, you will receive your API key.
Ensure you store your API key securely, as it will be used to authenticate your API requests. Remember, each API key is scoped to a specific brand, so you will need a separate key for each brand you work with.
Authenticating API Requests with Your API Key
With your API key in hand, you can now authenticate your requests to the Endear API. The API uses a simple API key-based authentication method. Here's how you can set it up:
import requests
# Define the API endpoint and headers
endpoint = "https://api.endearhq.com/graphql"
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Example request payload
payload = {
"query": "query { currentIntegration { id } }"
}
# Make a POST request to authenticate
response = requests.post(endpoint, headers=headers, json=payload)
# Check the response
if response.status_code == 200:
print("Authentication successful!")
else:
print("Authentication failed:", response.status_code)
Replace Your_API_Key
with the API key you generated earlier. This code snippet demonstrates how to authenticate your requests using Python's requests
library. A successful response indicates that your API key is valid and ready for use.
For more detailed information on authentication, you can refer to the Endear Authentication Documentation.
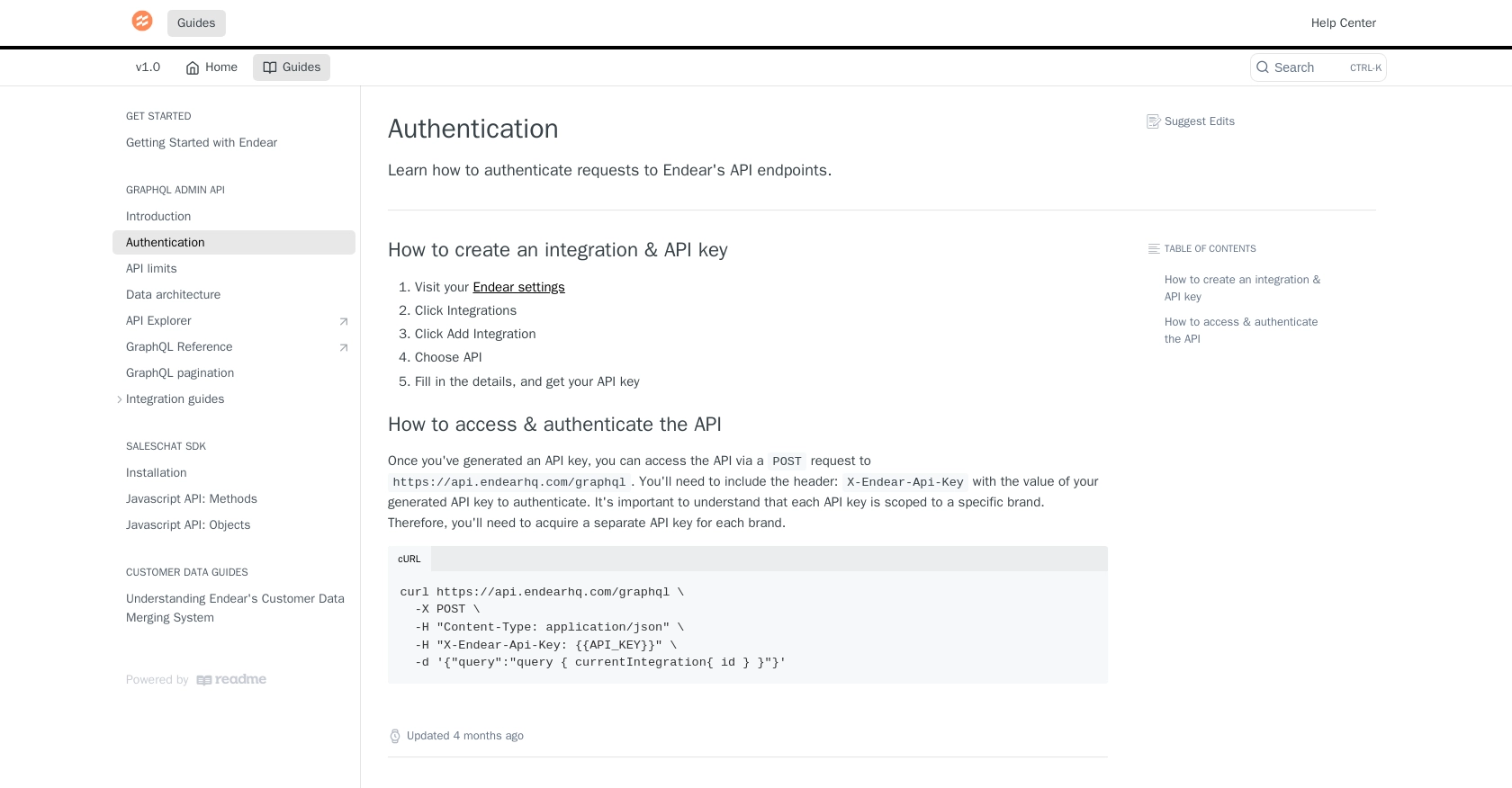
sbb-itb-96038d7
Making API Calls to Retrieve Notes with Endear API in Python
To interact with the Endear API and retrieve notes, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your environment, making the API call, and handling the response.
Setting Up Your Python Environment for Endear API
Before making any API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Writing Python Code to Retrieve Notes from Endear API
With your environment set up, you can now write the Python code to fetch notes from Endear. Create a file named get_endear_notes.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.endearhq.com/graphql"
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Define the GraphQL query to retrieve notes
query = """
query {
searchNotes {
edges {
node {
id
description
title
creator {
full_name
}
}
}
pageInfo {
endCursor
hasNextPage
}
}
}
"""
# Make a POST request to the API
response = requests.post(endpoint, headers=headers, json={"query": query})
# Check if the request was successful
if response.status_code == 200:
notes = response.json()
for edge in notes['data']['searchNotes']['edges']:
note = edge['node']
print(f"ID: {note['id']}, Title: {note['title']}, Description: {note['description']}, Creator: {note['creator']['full_name']}")
else:
print("Failed to retrieve notes:", response.status_code)
Replace Your_API_Key
with the API key you obtained earlier. This script sends a GraphQL query to the Endear API to fetch notes, including their ID, title, description, and creator's full name.
Running the Python Script and Verifying Results
Execute the script from your terminal or command line:
python get_endear_notes.py
If successful, the script will print out the details of the notes retrieved from your Endear account. You can verify the results by checking the notes in your Endear dashboard.
Handling Errors and Understanding Endear API Rate Limits
When making API calls, it's crucial to handle potential errors. The Endear API may return various status codes, such as 429 for rate limit errors. Endear's API allows up to 120 requests per minute. If you exceed this limit, you'll receive an HTTP 429 error. For more details, refer to the Endear Rate Limits Documentation.
Implement error handling in your script to manage these scenarios effectively and ensure a smooth integration experience.
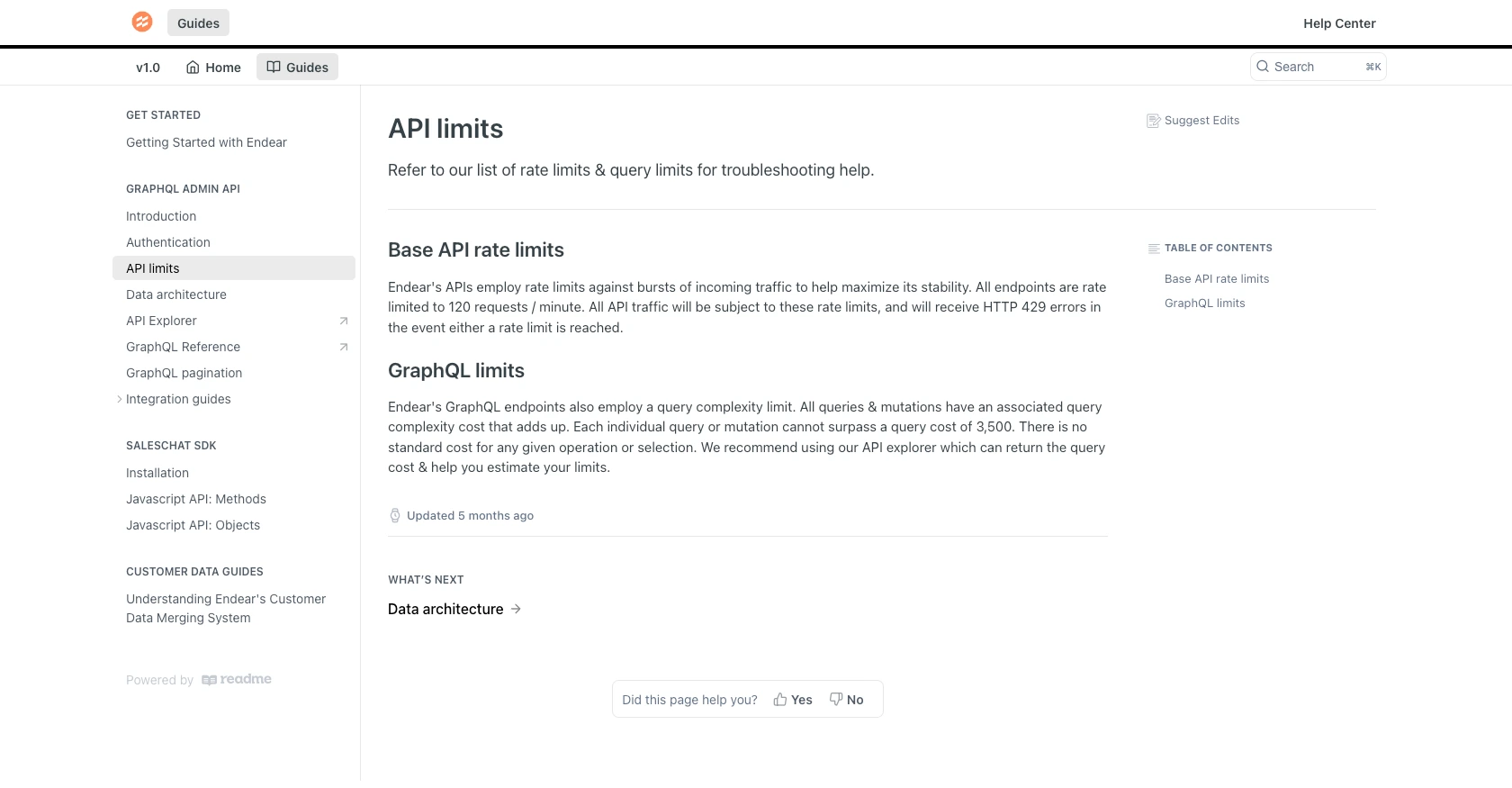
Conclusion and Best Practices for Using Endear API in Python
Integrating with the Endear API allows developers to efficiently manage and retrieve customer notes, enhancing the ability to personalize customer interactions. By following the steps outlined in this guide, you can seamlessly authenticate and interact with the Endear API using Python.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement retry logic and exponential backoff strategies to handle HTTP 429 errors gracefully.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to maintain consistency across your applications.
- Error Handling: Implement robust error handling to manage different status codes and ensure a smooth user experience.
Streamlining Integration Development with Endgrate
While integrating with APIs like Endear can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Endear. This allows you to focus on your core product while outsourcing integration development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Note
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?