Using the PipelineCRM API to Create Deals in Javascript
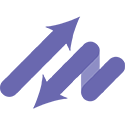
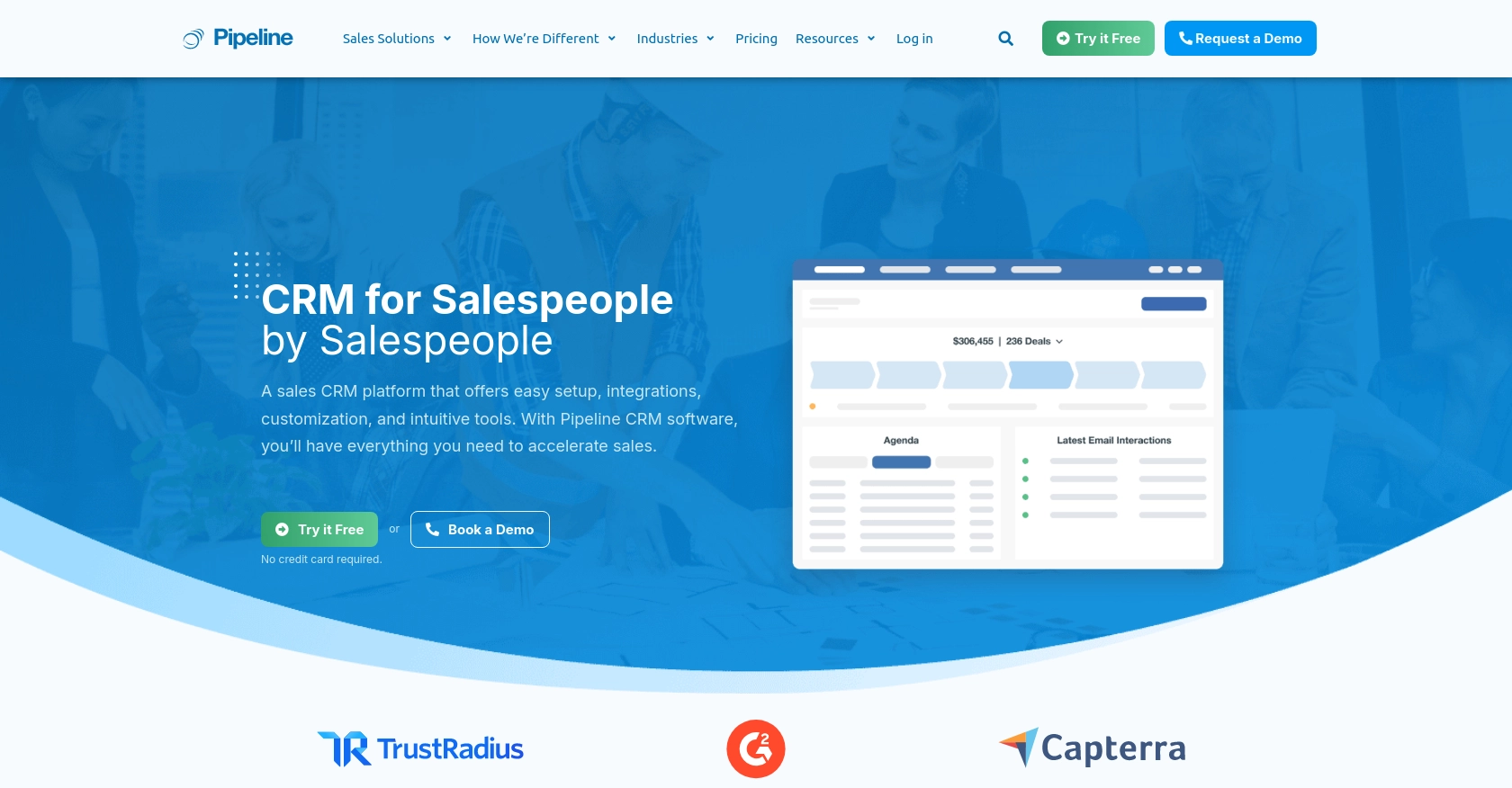
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features like deal tracking, contact management, and sales forecasting, PipelineCRM empowers sales teams to close deals faster and improve customer relationships.
Integrating with the PipelineCRM API allows developers to automate and streamline sales operations. For example, a developer might want to create deals programmatically using JavaScript to ensure that new sales opportunities are captured and tracked in real-time, enhancing the overall sales workflow.
Setting Up a PipelineCRM Test Account and API Key
Before you can start creating deals using the PipelineCRM API with JavaScript, you need to set up a test account and obtain an API key. This will allow you to authenticate your requests and interact with the PipelineCRM platform programmatically.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial or use the free version available on the PipelineCRM website. Follow these steps to create your account:
- Visit the PipelineCRM website.
- Click on the "Sign Up" button and fill in the required information to create your account.
- Once your account is set up, log in to access the PipelineCRM dashboard.
Generating an API Key for Authentication
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account.
- Navigate to the "Settings" section from the dashboard.
- Find the "API Keys" option and click on it.
- Click on "Generate New API Key" and provide a name for your key to easily identify it later.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your API key ready, you can now proceed to make API calls to create deals in PipelineCRM using JavaScript. For more details on authentication, refer to the PipelineCRM API documentation.
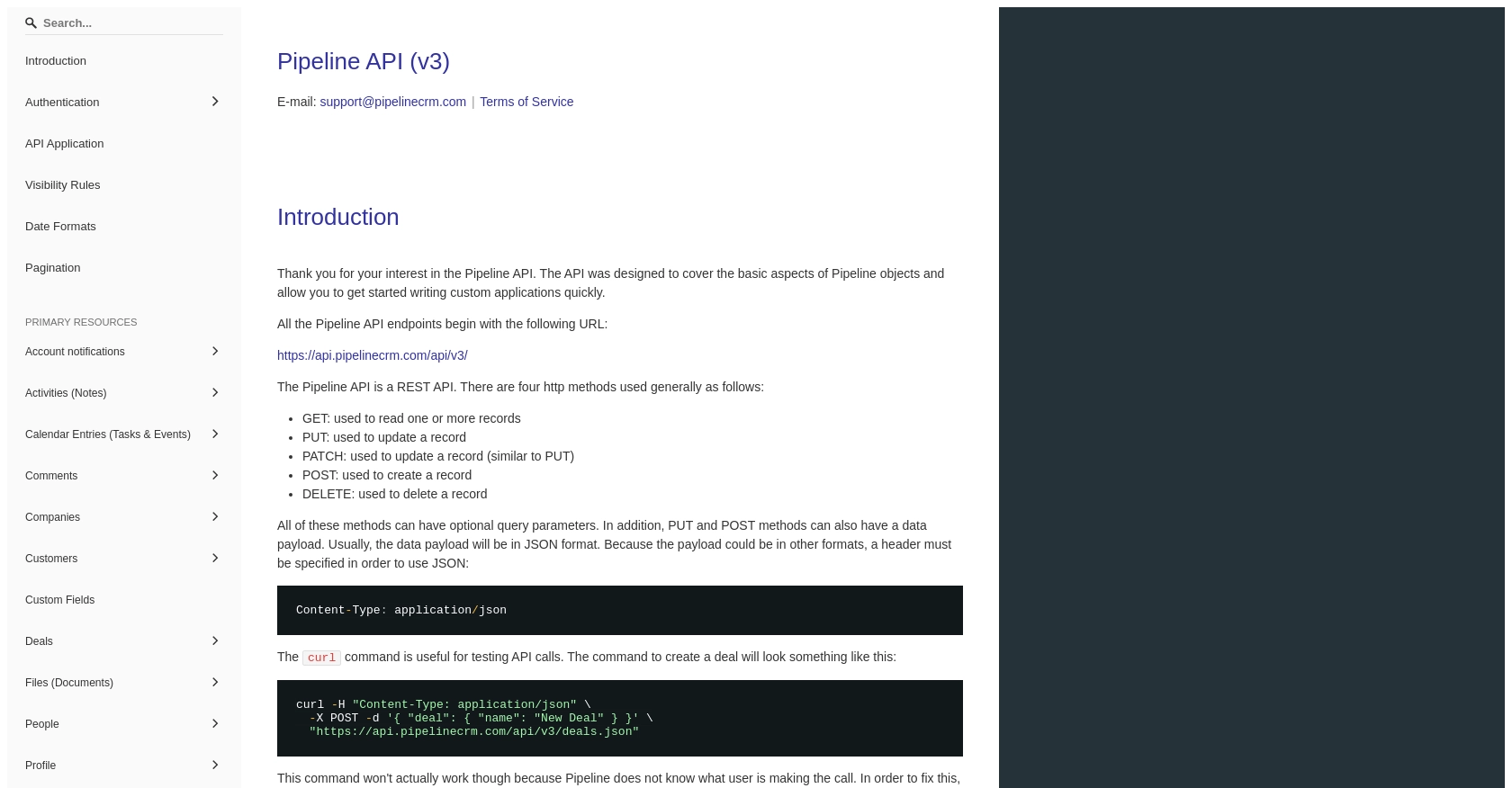
sbb-itb-96038d7
Making API Calls to Create Deals in PipelineCRM Using JavaScript
To create deals in PipelineCRM using JavaScript, you'll need to make HTTP requests to the PipelineCRM API. This section will guide you through the process of setting up your JavaScript environment, making the API call, and handling the response.
Setting Up Your JavaScript Environment
Before you begin, ensure you have the following installed on your machine:
- Node.js (preferably version 14.x or higher)
- NPM (Node Package Manager)
You'll also need to install the axios
library, which simplifies making HTTP requests. Run the following command in your terminal:
npm install axios
Creating a Deal in PipelineCRM with JavaScript
Now that your environment is set up, you can proceed to write the JavaScript code to create a deal in PipelineCRM. Create a new file named createDeal.js
and add the following code:
const axios = require('axios');
// Define the API endpoint and your API key
const endpoint = 'https://api.pipelinecrm.com/api/v3/deals';
const apiKey = 'Your_API_Key';
// Define the deal data
const dealData = {
name: 'New Business Opportunity',
value: 10000,
currency: 'USD',
stage_id: 1
};
// Set the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
};
// Make a POST request to create a new deal
axios.post(endpoint, dealData, { headers })
.then(response => {
console.log('Deal Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Deal:', error.response ? error.response.data : error.message);
});
Replace Your_API_Key
with the API key you generated earlier.
Running the JavaScript Code and Verifying the Deal Creation
To execute the code, run the following command in your terminal:
node createDeal.js
If successful, you should see a confirmation message with the details of the newly created deal. You can verify the creation by checking the PipelineCRM dashboard for the new deal entry.
Handling Errors and Troubleshooting
When making API calls, it's essential to handle potential errors. The code above logs errors to the console, providing information about what went wrong. Common issues might include:
- Invalid API key: Ensure your API key is correct and has the necessary permissions.
- Network issues: Check your internet connection and ensure the API endpoint is reachable.
- Incorrect data format: Verify that the data being sent matches the expected format in the PipelineCRM API documentation.
For more detailed error information, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for Using PipelineCRM API with JavaScript
Integrating with the PipelineCRM API using JavaScript can significantly enhance your sales processes by automating deal creation and management. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and create deals programmatically.
Best Practices for Secure and Efficient API Integration
- Securely Store API Keys: Always keep your API keys confidential and avoid hardcoding them directly in your source code. Consider using environment variables or secure vaults to store sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the PipelineCRM API to avoid throttling. Implement retry logic with exponential backoff to manage rate limits effectively.
- Validate Data: Ensure that the data you send to the API is correctly formatted and validated to prevent errors and ensure successful API calls.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any potential issues or anomalies in your integration.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including PipelineCRM, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can help you save time and resources.
Read More
Ready to get started?