How to Create Or Update Vendors with the Quickbooks API in Javascript
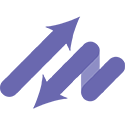
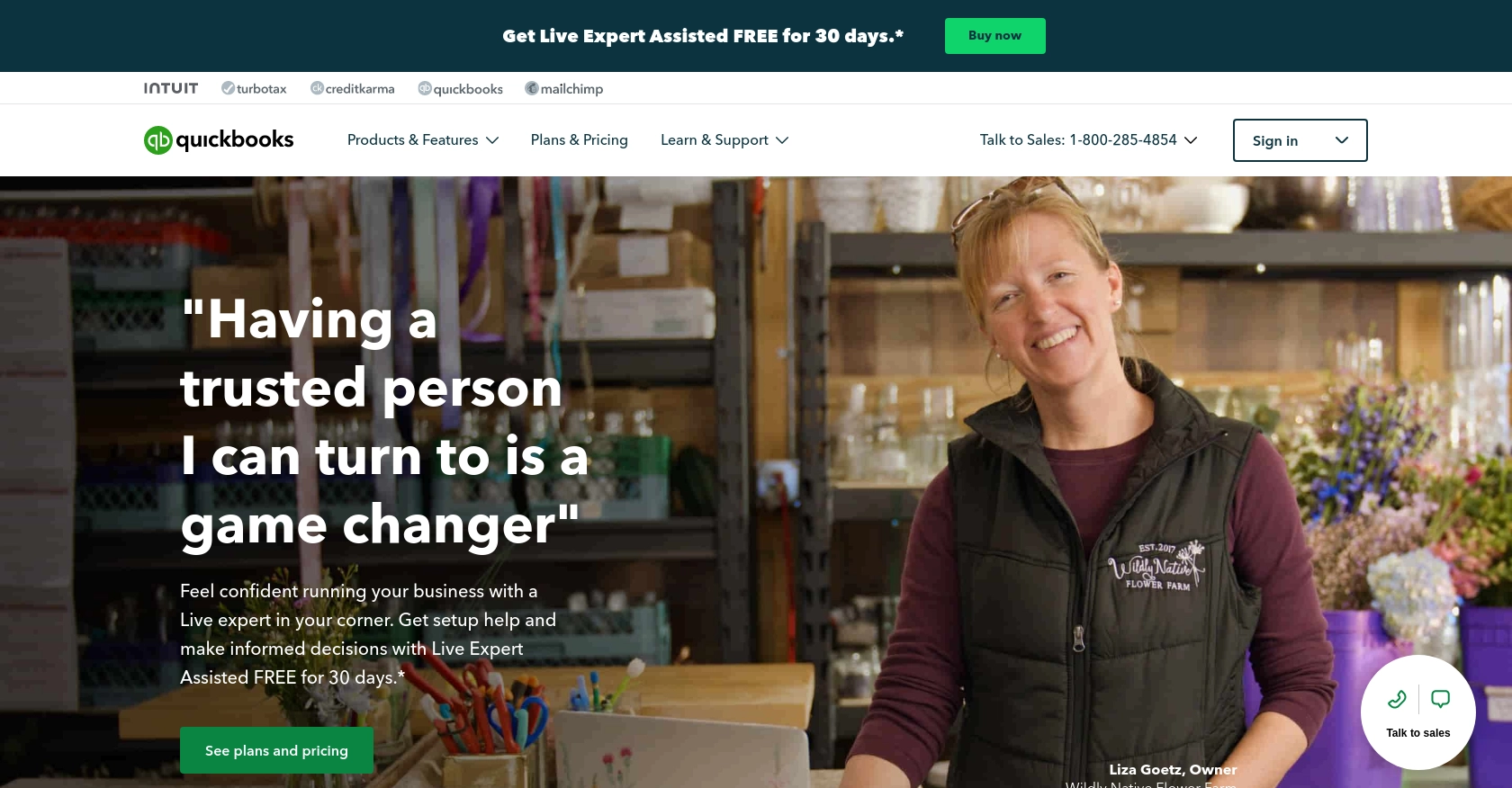
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers a comprehensive suite of tools for managing finances, invoicing, payroll, and more. It is a popular choice among small to medium-sized businesses due to its user-friendly interface and robust functionality.
Integrating with the QuickBooks API allows developers to automate and streamline financial processes, such as managing vendor information. For example, a developer might use the QuickBooks API to create or update vendor details directly from an external application, ensuring that financial records are always up-to-date and accurate.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start integrating with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test your application without affecting live data. QuickBooks provides a sandbox environment that mimics the production environment, giving you a safe space to develop and test your integrations.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the Intuit Developer Portal.
- Click on the "Sign Up" button and fill out the registration form with your details.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you can set up a sandbox company:
- Navigate to the "Dashboard" in your developer account.
- Click on "Sandbox" from the left-hand menu.
- Select "Add Sandbox" and choose the type of company you want to create.
- Follow the prompts to complete the setup of your sandbox company.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- In your developer dashboard, go to "My Apps" and click on "Create an App."
- Select "QuickBooks Online and Payments" as the platform.
- Fill in the required details for your app and click "Create App."
- Once your app is created, navigate to the "Keys & OAuth" section.
- Here, you'll find your Client ID and Client Secret. Keep these credentials secure as they are essential for API authentication.
For more detailed instructions, refer to the Intuit Developer Documentation.
Configuring App Settings for API Access
Ensure your app has the correct settings to access the QuickBooks API:
- In the "App Settings" section, configure the Redirect URIs to match your application's callback URL.
- Set the scopes required for accessing vendor data, such as "com.intuit.quickbooks.accounting."
- Save your settings to apply the changes.
For further guidance, visit the App Settings Documentation.
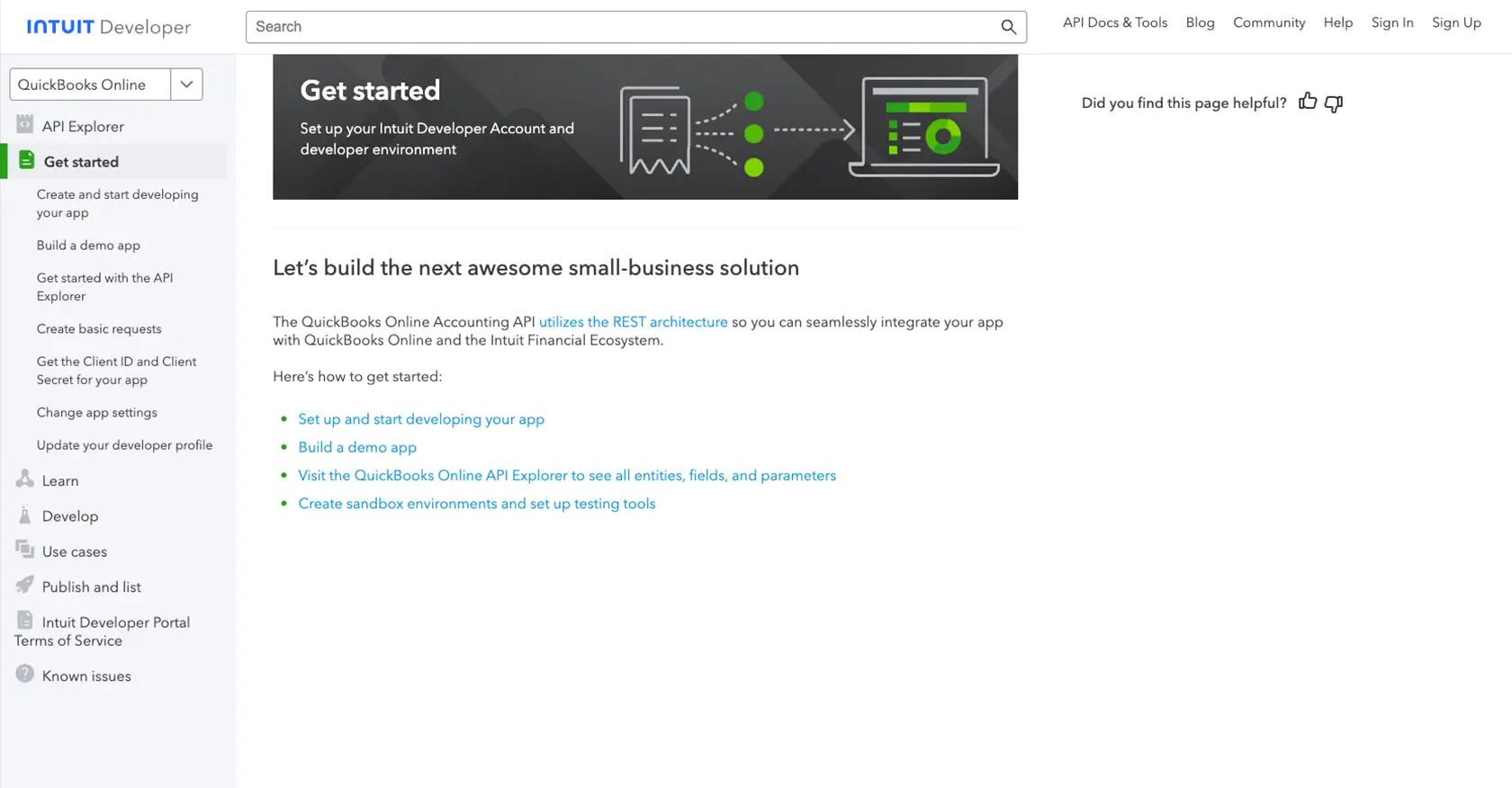
sbb-itb-96038d7
Making API Calls to Create or Update Vendors with QuickBooks API in JavaScript
To interact with the QuickBooks API using JavaScript, you'll need to set up your environment and write code to make API calls. This section will guide you through the process of creating or updating vendor information using the QuickBooks API.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites installed:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it:
mkdir quickbooks-vendor-api
cd quickbooks-vendor-api
npm init -y
Installing Required Dependencies
You'll need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Create or Update Vendors
Now, create a file named vendor.js
and add the following code to make API calls to QuickBooks:
const axios = require('axios');
// Set your QuickBooks API credentials
const clientId = 'Your_Client_ID';
const clientSecret = 'Your_Client_Secret';
const accessToken = 'Your_Access_Token';
// Set the API endpoint for creating or updating vendors
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/vendor';
// Function to create or update a vendor
async function createOrUpdateVendor(vendorData) {
try {
const response = await axios.post(endpoint, vendorData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'Accept': 'application/json'
}
});
console.log('Vendor created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating vendor:', error.response.data);
}
}
// Sample vendor data
const vendorData = {
DisplayName: 'Sample Vendor',
PrimaryPhone: {
FreeFormNumber: '(555) 555-5555'
},
PrimaryEmailAddr: {
Address: 'vendor@example.com'
}
};
// Call the function to create or update the vendor
createOrUpdateVendor(vendorData);
Replace Your_Client_ID
, Your_Client_Secret
, Your_Access_Token
, and YOUR_COMPANY_ID
with your actual QuickBooks credentials and company ID.
Running the JavaScript Code
To execute the code, run the following command in your terminal:
node vendor.js
If successful, you should see a message indicating that the vendor was created or updated. Verify the changes by checking your QuickBooks sandbox account.
Handling Errors and Debugging
When making API calls, it's essential to handle potential errors. The code above logs errors to the console, providing details from the API response. Common error codes include:
- 401 Unauthorized: Check your access token and credentials.
- 400 Bad Request: Verify the request payload and endpoint URL.
For more error codes and their meanings, refer to the Intuit Developer Documentation.
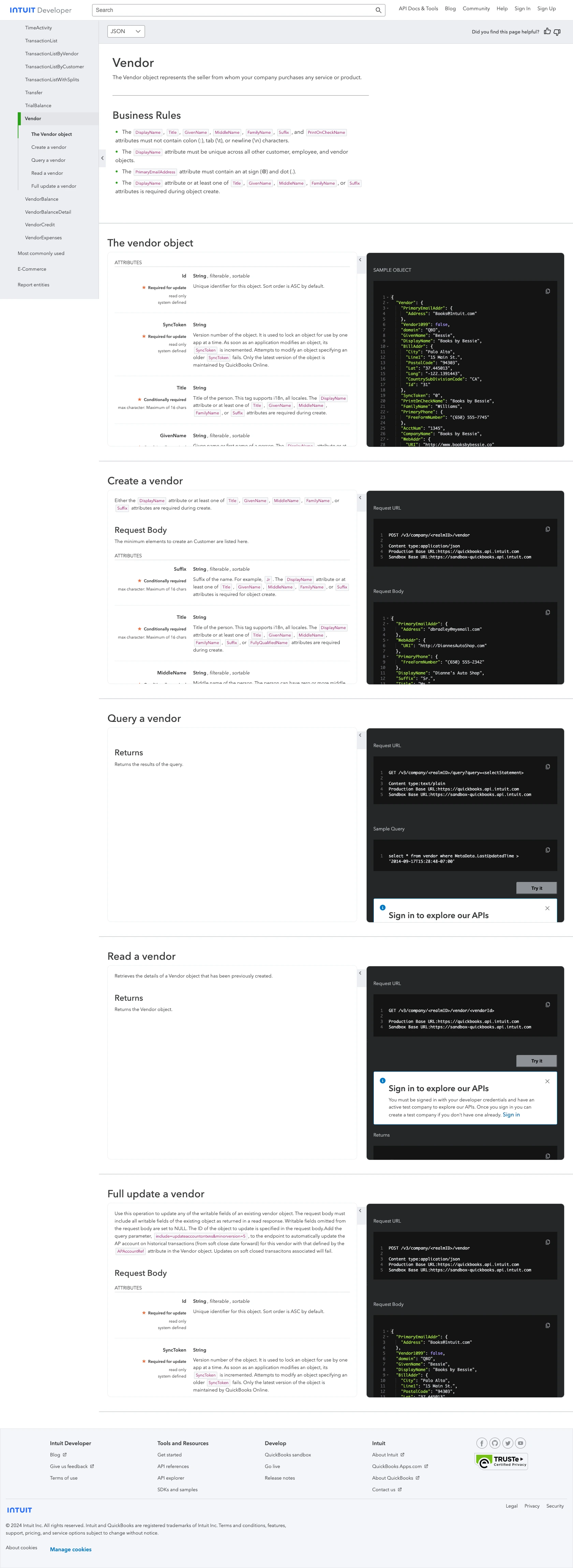
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API using JavaScript allows developers to efficiently manage vendor data, ensuring that financial records are accurate and up-to-date. By following the steps outlined in this guide, you can create or update vendor information seamlessly within your QuickBooks sandbox environment.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and Access Token securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits in place. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send to QuickBooks is standardized and validated to prevent errors and maintain consistency across your applications.
Streamline Your Integrations with Endgrate
While integrating with QuickBooks API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including QuickBooks.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/vendor
Ready to get started?