How to Get Customers with the Stamped API in Javascript
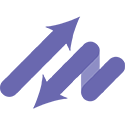
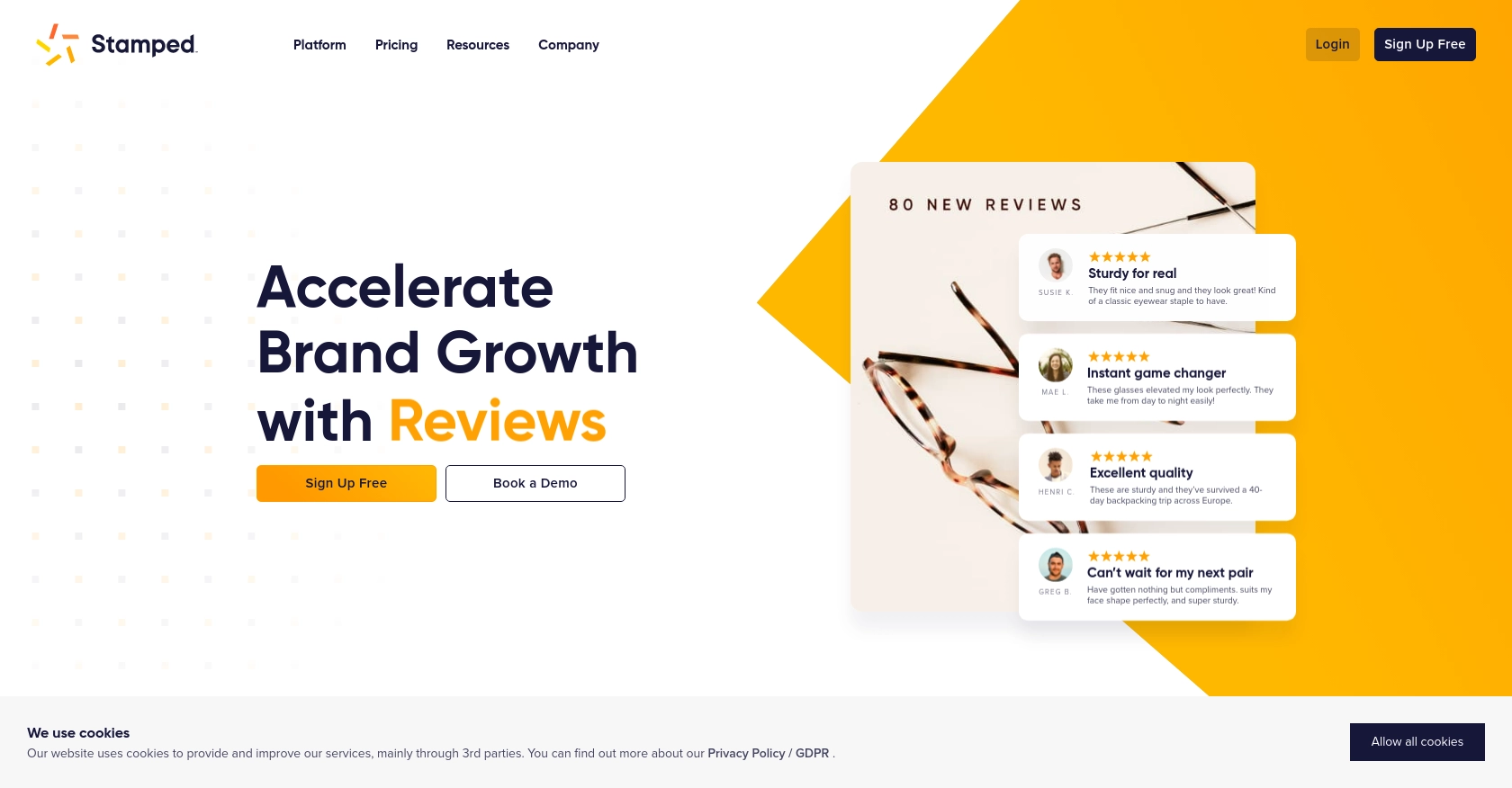
Introduction to Stamped API Integration
Stamped is a powerful platform that empowers over 75,000 brands to enhance their growth through customer reviews, loyalty programs, and more. It provides a comprehensive suite of tools designed to help businesses connect with their customers and drive engagement.
Integrating with the Stamped API allows developers to access and manage customer data seamlessly. For example, you might want to retrieve customer information to personalize marketing campaigns or analyze customer behavior. This article will guide you through the process of using JavaScript to interact with the Stamped API to get customer details efficiently.
Setting Up Your Stamped API Test Account
Before you can start interacting with the Stamped API using JavaScript, you'll need to set up a test account. This involves creating an account on Stamped and obtaining the necessary API keys for authentication.
Creating a Stamped Account
To begin, you'll need to sign up for a Stamped account. Note that using the Stamped API requires a Professional or Enterprise plan. Visit the Stamped website and follow the instructions to create an account. Once your account is set up, you can access the dashboard where you'll manage your API keys.
Generating API Keys for Stamped Integration
Stamped uses a combination of public and private API keys for authentication. Follow these steps to generate your API keys:
- Log in to your Stamped account and navigate to the Control Panel.
- Go to the API Keys section.
- Here, you'll find your public and private API keys. These keys are essential for authenticating your API requests.
Ensure you keep these keys secure, as they provide access to your Stamped account data.
Understanding Stamped API Authentication
Authentication to private endpoints in the Stamped API is done via HTTP Basic Auth. Use your public API key as the username and your private API key as the password. For public endpoints, you may only need the store hash or public key.
For more detailed information, refer to the Stamped REST API documentation.
Preparing Your Development Environment
With your API keys ready, you can now prepare your development environment to start making API calls. Ensure you have a JavaScript runtime environment set up, such as Node.js, to execute your code.
In the next section, we'll dive into making API calls to retrieve customer data using JavaScript.
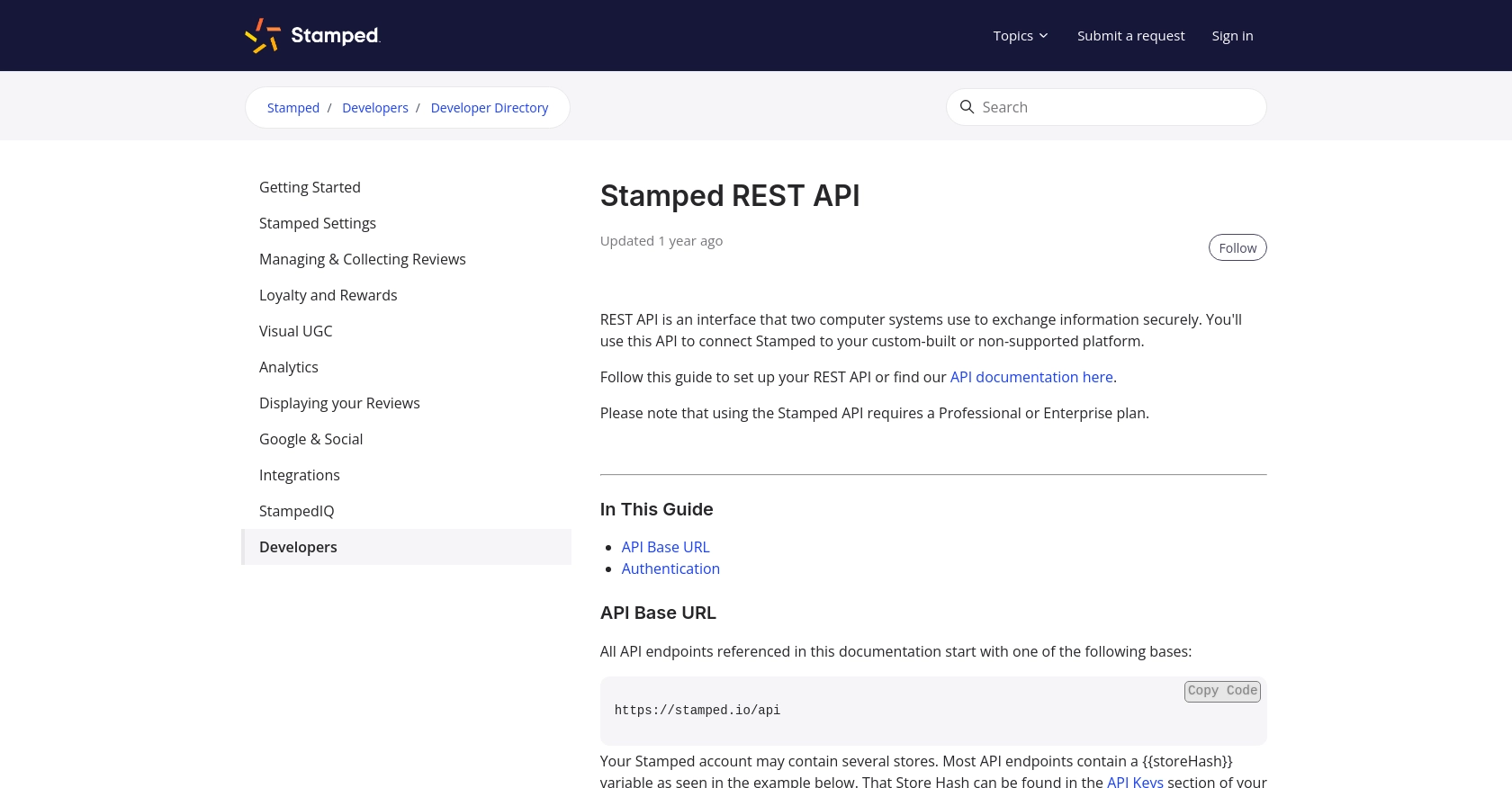
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data with Stamped API in JavaScript
Now that you have your Stamped API keys and development environment ready, it's time to make API calls to retrieve customer data. In this section, we'll guide you through the process of using JavaScript to interact with the Stamped API efficiently.
Setting Up Your JavaScript Environment for Stamped API Integration
To begin, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside of a browser. You can download and install it from the official Node.js website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the necessary dependencies. For this tutorial, we'll use the popular axios
library to make HTTP requests. Run the following command in your terminal to install it:
npm install axios
Writing JavaScript Code to Get Customer Data from Stamped API
With your environment set up, you can now write the JavaScript code to interact with the Stamped API. Create a new file named getCustomer.js
and add the following code:
const axios = require('axios');
// Replace with your actual store hash, public key, and private key
const storeHash = 'your_store_hash';
const publicKey = 'your_public_key';
const privateKey = 'your_private_key';
// Set the API endpoint
const endpoint = `https://stamped.io/api/v3/merchant/shops/${storeHash}/customers/{customerId}`;
// Function to get customer data
async function getCustomer(customerId) {
try {
const response = await axios.get(endpoint.replace('{customerId}', customerId), {
auth: {
username: publicKey,
password: privateKey
}
});
console.log('Customer Data:', response.data);
} catch (error) {
console.error('Error fetching customer data:', error.response ? error.response.data : error.message);
}
}
// Replace with the actual customer ID you want to retrieve
getCustomer('your_customer_id');
In this code, we use the axios
library to make a GET request to the Stamped API. We authenticate using HTTP Basic Auth, providing the public API key as the username and the private API key as the password. The getCustomer
function retrieves customer data by replacing {customerId}
with the actual customer ID you want to fetch.
Running the JavaScript Code to Fetch Customer Data
To execute the code, run the following command in your terminal:
node getCustomer.js
If successful, you should see the customer data printed in your terminal. If there's an error, the error message will help you diagnose the issue.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log any errors that occur during the request. Common errors might include authentication failures or invalid customer IDs.
To verify that your request succeeded, you can cross-check the returned data with the customer information in your Stamped dashboard. This ensures that the API call is functioning as expected.
For more detailed information on error codes and handling, refer to the Stamped API documentation.
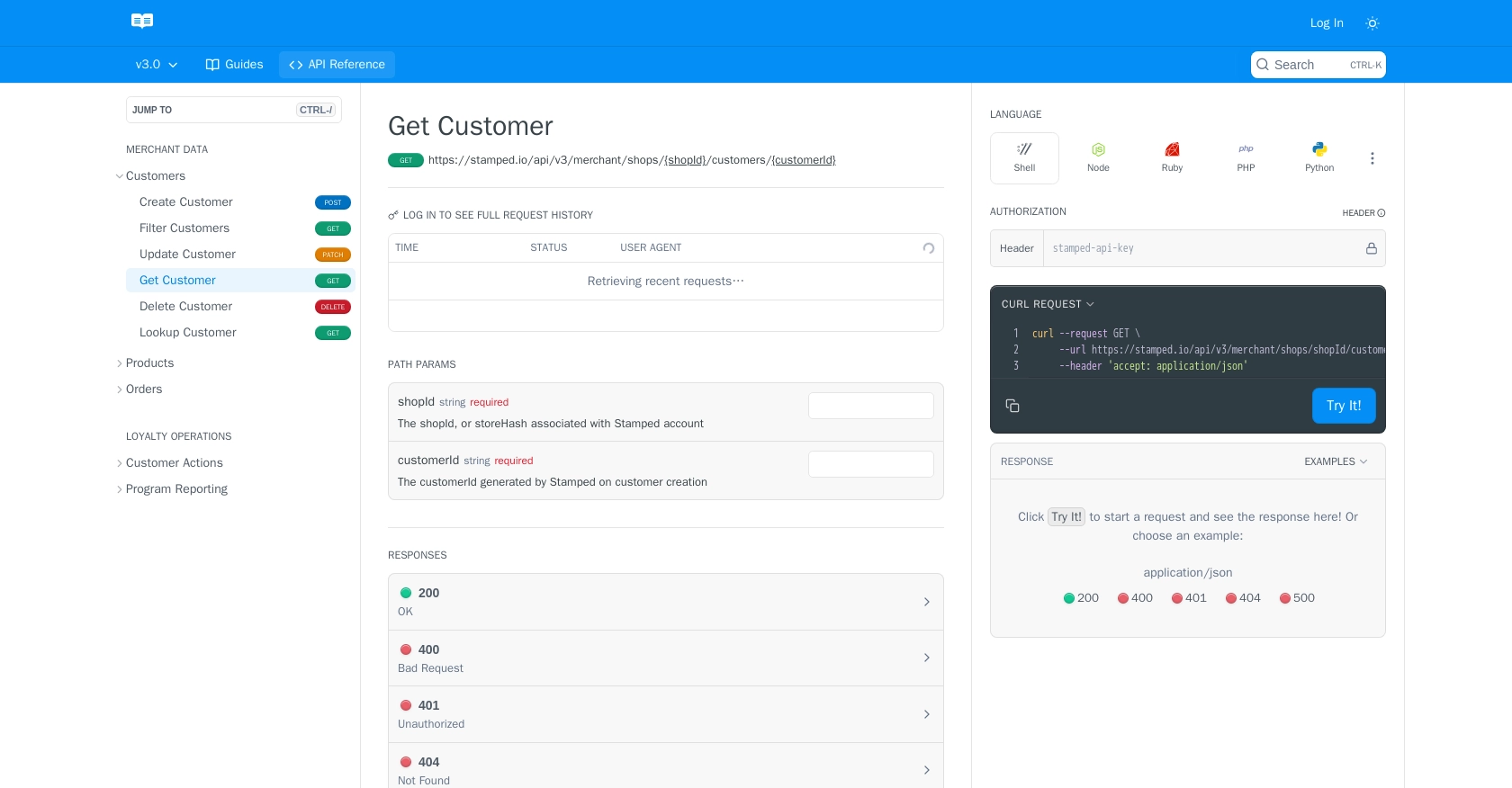
Conclusion and Best Practices for Using Stamped API with JavaScript
Integrating with the Stamped API using JavaScript provides a powerful way to manage and analyze customer data, enhancing your ability to personalize marketing efforts and improve customer engagement. By following the steps outlined in this guide, you can efficiently retrieve customer information and handle API interactions smoothly.
Best Practices for Stamped API Integration
- Secure Your API Keys: Always keep your public and private API keys secure. Avoid hardcoding them directly in your source code. Instead, use environment variables or secure vaults to store sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Stamped API. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Refer to the Stamped API documentation for specific rate limit details.
- Error Handling: Implement comprehensive error handling in your API calls. Use the error response data to diagnose issues and provide meaningful feedback to users or logs for debugging.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed for your application. This helps maintain consistency and improves data usability.
Streamlining Integrations with Endgrate
While integrating with the Stamped API directly can be effective, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including Stamped.
With Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while providing an easy and intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration approach.
Read More
Ready to get started?