How to Create or Update People with the Affinity API in Python
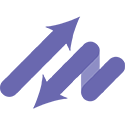
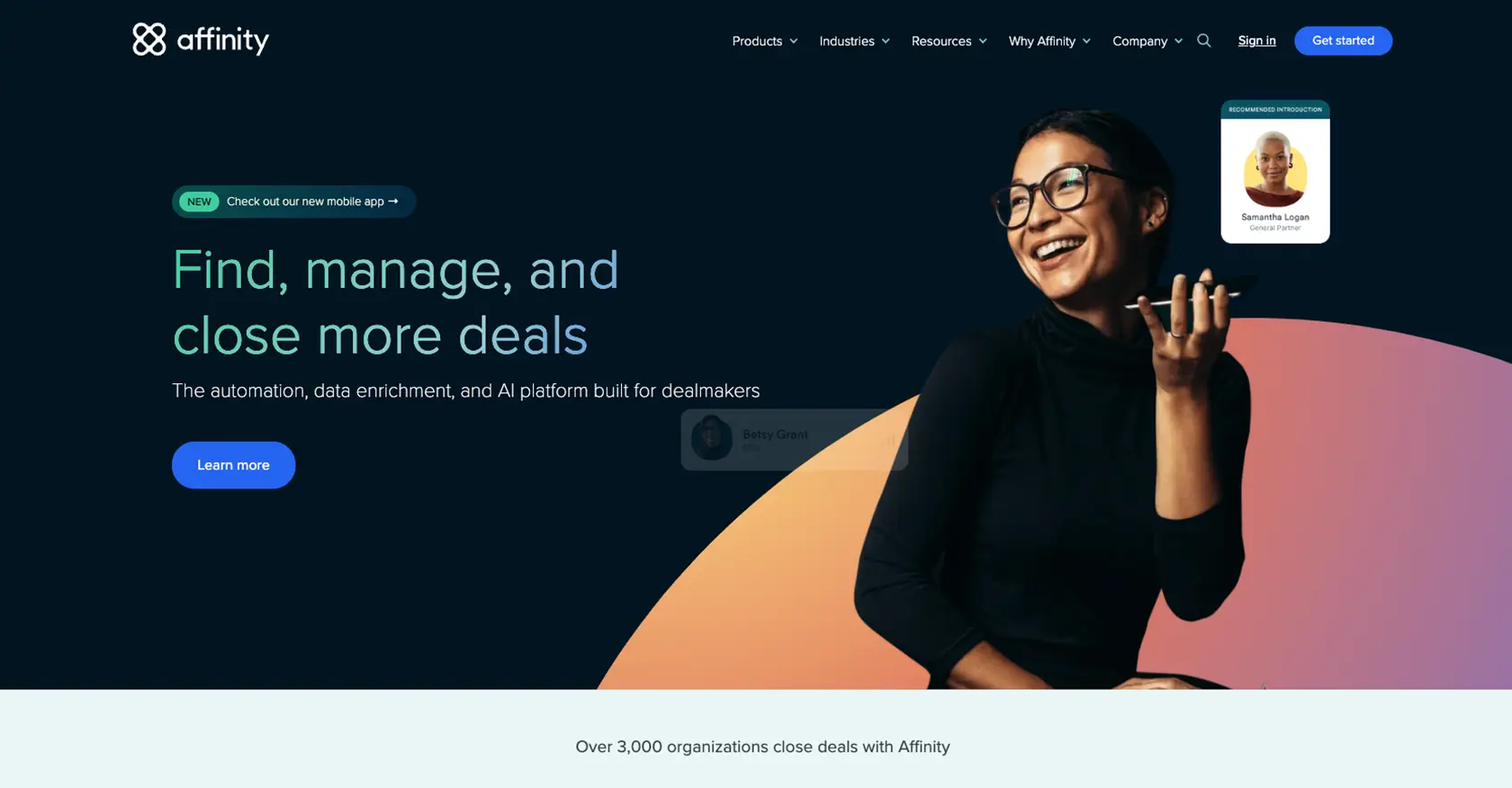
Introduction to Affinity API for People Management
Affinity is a powerful relationship intelligence platform designed to help businesses manage and leverage their professional networks. It provides tools for tracking interactions, managing contacts, and analyzing relationship strengths, making it an essential tool for organizations looking to enhance their networking capabilities.
Integrating with the Affinity API allows developers to automate and streamline the management of contacts, known as "people" within the platform. For example, you can create or update contact information programmatically, ensuring that your organization's database is always up-to-date with the latest information.
Using Python to interact with the Affinity API can significantly enhance your ability to manage contacts efficiently. This guide will walk you through the process of creating or updating people in Affinity using Python, providing a seamless integration experience.
Setting Up Your Affinity API Test Account
Before you can start integrating with the Affinity API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Affinity provides a straightforward process for obtaining an API key, which is essential for authentication and making requests.
Steps to Create an Affinity Test Account
- Sign Up for Affinity: If you don't already have an Affinity account, visit the Affinity website and sign up for a free trial or demo account.
- Access the Settings Panel: Once logged in, navigate to the Settings Panel, which can be found in the left sidebar of the Affinity web app.
- Generate an API Key: In the Settings Panel, locate the section for API keys. Follow the instructions to generate a new API key. This key will be used to authenticate your API requests.
- Secure Your API Key: Copy the generated API key and store it securely. You'll need this key to authenticate your API requests in Python.
Authenticating with the Affinity API Using an API Key
The Affinity API uses HTTP Basic Auth for authentication. Your API key will serve as the password, and you do not need to provide a username. Here's an example of how to authenticate using your API key in a Python script:
import requests
# Define the API endpoint
url = "https://api.affinity.co/api_endpoint"
# Your API key
api_key = "Your_API_Key"
# Make a request with HTTP Basic Auth
response = requests.get(url, auth=('', api_key))
# Check the response
if response.status_code == 200:
print("Authentication successful!")
else:
print("Failed to authenticate:", response.status_code)
Ensure that you replace Your_API_Key
with the API key you generated. This setup will allow you to make authenticated requests to the Affinity API, enabling you to create or update people within the platform.
For more detailed information on authentication, refer to the Affinity API documentation.
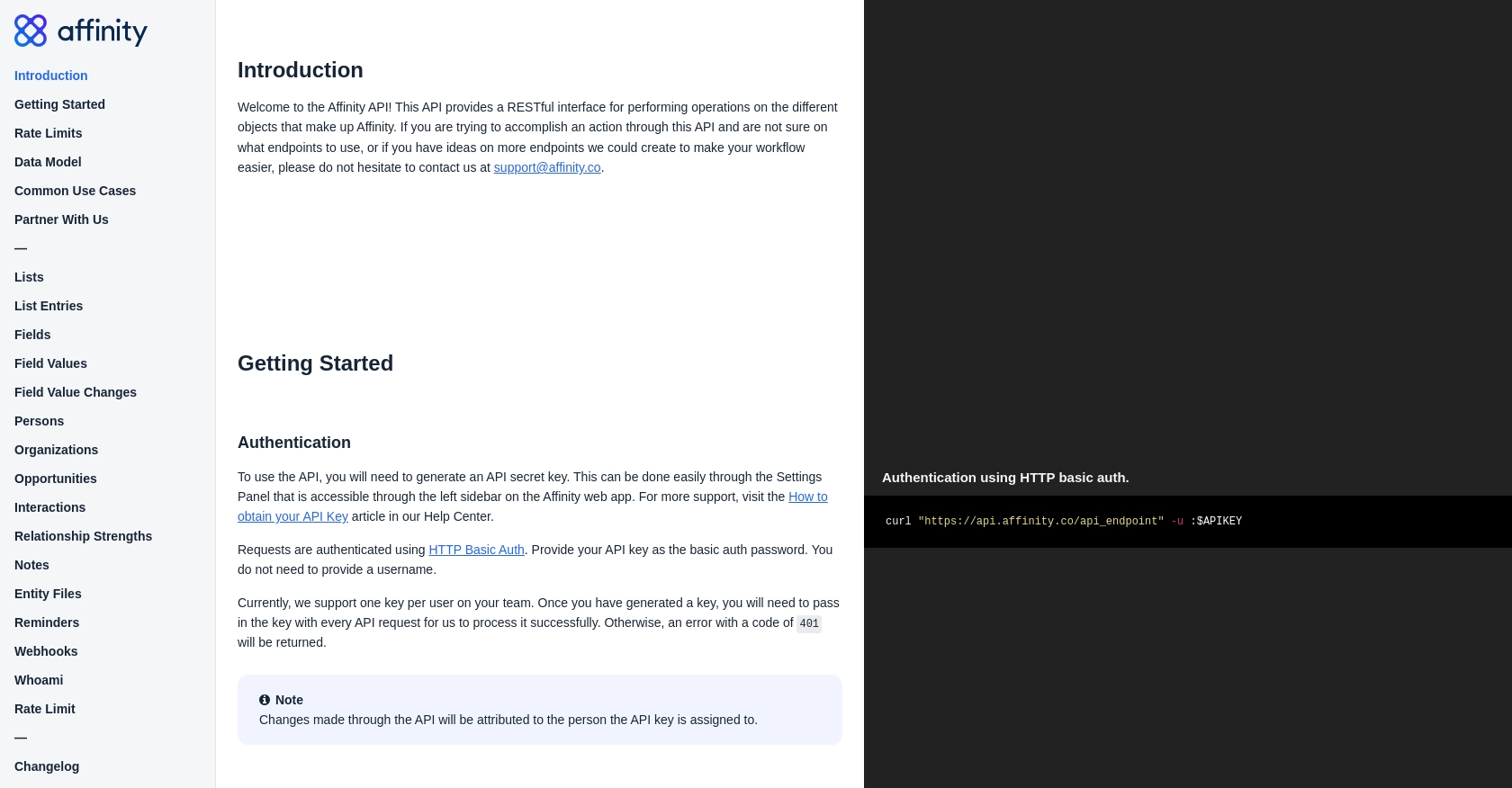
sbb-itb-96038d7
How to Make API Calls to Create or Update People with Affinity API in Python
To interact with the Affinity API for creating or updating people, you'll need to use Python to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Affinity API Integration
Before making API calls, ensure you have Python installed on your machine. This guide uses Python 3.11.1. You'll also need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Create or Update People in Affinity
With your environment ready, you can now write the Python script to interact with the Affinity API. Below is an example of how to create a new person:
import requests
# Define the API endpoint for creating a person
url = "https://api.affinity.co/persons"
# Your API key
api_key = "Your_API_Key"
# Person data to be created
person_data = {
"first_name": "Alice",
"last_name": "Doe",
"emails": ["alice@example.com"]
}
# Make a POST request to create a new person
response = requests.post(url, json=person_data, auth=('', api_key))
# Check the response
if response.status_code == 200:
print("Person created successfully:", response.json())
else:
print("Failed to create person:", response.status_code, response.json())
Replace Your_API_Key
with your actual API key. This script sends a POST request to the Affinity API to create a new person with the specified details.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. The Affinity API returns JSON responses, which you can parse to check the status of your request. If the request is successful, you should see the details of the created or updated person. If not, handle errors based on the status code:
- 401 Unauthorized: Check your API key.
- 403 Forbidden: Ensure you have the necessary permissions.
- 404 Not Found: Verify the endpoint URL.
- 422 Unprocessable Entity: Check the request parameters.
- 429 Too Many Requests: Implement rate limiting strategies.
For more details on error codes, refer to the Affinity API documentation.
Verifying API Call Success in Affinity
To confirm that your API call was successful, check the Affinity platform to see if the person was created or updated as expected. This verification step ensures that your integration is working correctly.
Conclusion and Best Practices for Using Affinity API in Python
Integrating with the Affinity API using Python provides a robust solution for managing your organization's contacts efficiently. By automating the creation and updating of people, you can ensure that your database remains accurate and up-to-date, enhancing your networking capabilities.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Implement Rate Limiting: Be mindful of the Affinity API's rate limits, which allow up to 900 requests per user per minute. Implement strategies to handle
429 Too Many Requests
errors gracefully. - Data Standardization: Ensure that data fields are standardized across your applications to maintain consistency and improve data quality.
- Error Handling: Implement comprehensive error handling to manage different response codes effectively, ensuring a smooth user experience.
Enhance Your Integration Experience with Endgrate
While integrating with the Affinity API can significantly streamline your contact management processes, leveraging a tool like Endgrate can further enhance your integration experience. Endgrate allows you to build once for each use case, offering a unified API endpoint that connects to multiple platforms, including Affinity.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development while providing an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?