Using the Zoho Books API to Get Purchase Orders (with Python examples)
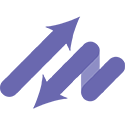
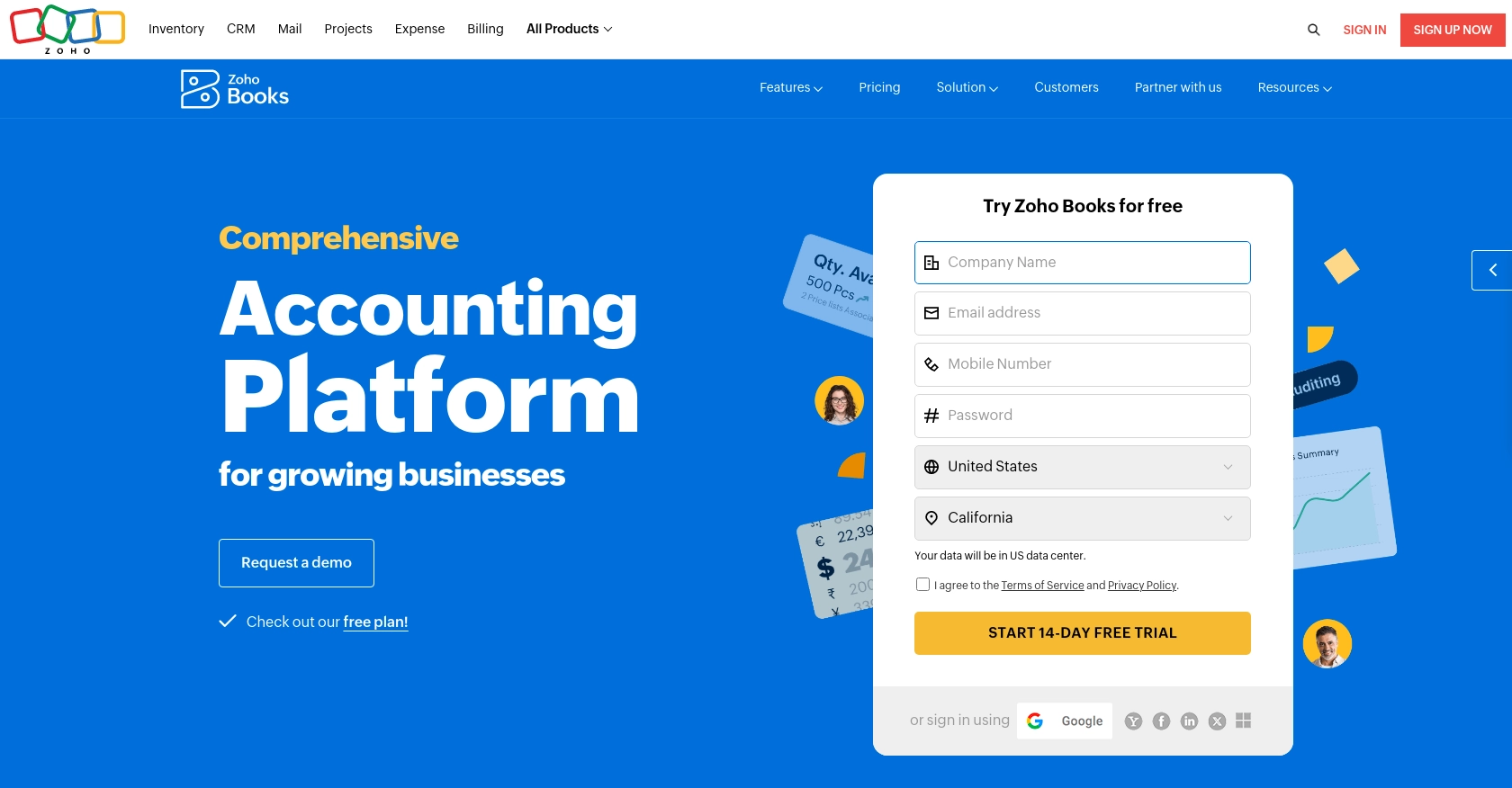
Introduction to Zoho Books API
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial management for businesses. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to simplify their accounting processes.
Integrating with the Zoho Books API allows developers to automate and enhance various accounting tasks. For example, accessing purchase orders through the API can help businesses manage their procurement processes more efficiently. By retrieving purchase orders programmatically, developers can integrate this data into other systems, ensuring seamless operations and up-to-date financial records.
This article will guide you through using Python to interact with the Zoho Books API, specifically focusing on retrieving purchase orders. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your Zoho Books Test Account for API Integration
Before you can start interacting with the Zoho Books API to retrieve purchase orders, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. This trial will give you access to the features necessary for testing API interactions.
- Visit the Zoho Books website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your Zoho Books account.
Setting Up OAuth for Zoho Books API Access
Zoho Books uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth:
- Go to the Zoho Developer Console.
- Click on "Add Client ID" to register your application.
- Provide the required details, such as client name, domain, and redirect URI.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure.
Generating an OAuth Access Token
With your Client ID and Client Secret, you can generate an OAuth access token:
- Redirect users to the following authorization URL:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the access token securely for future API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.purchaseorders.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
import requests
url = "https://accounts.zoho.com/oauth/v2/token"
payload = {
'code': 'YOUR_CODE',
'client_id': 'YOUR_CLIENT_ID',
'client_secret': 'YOUR_CLIENT_SECRET',
'redirect_uri': 'YOUR_REDIRECT_URI',
'grant_type': 'authorization_code'
}
response = requests.post(url, data=payload)
print(response.json())
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
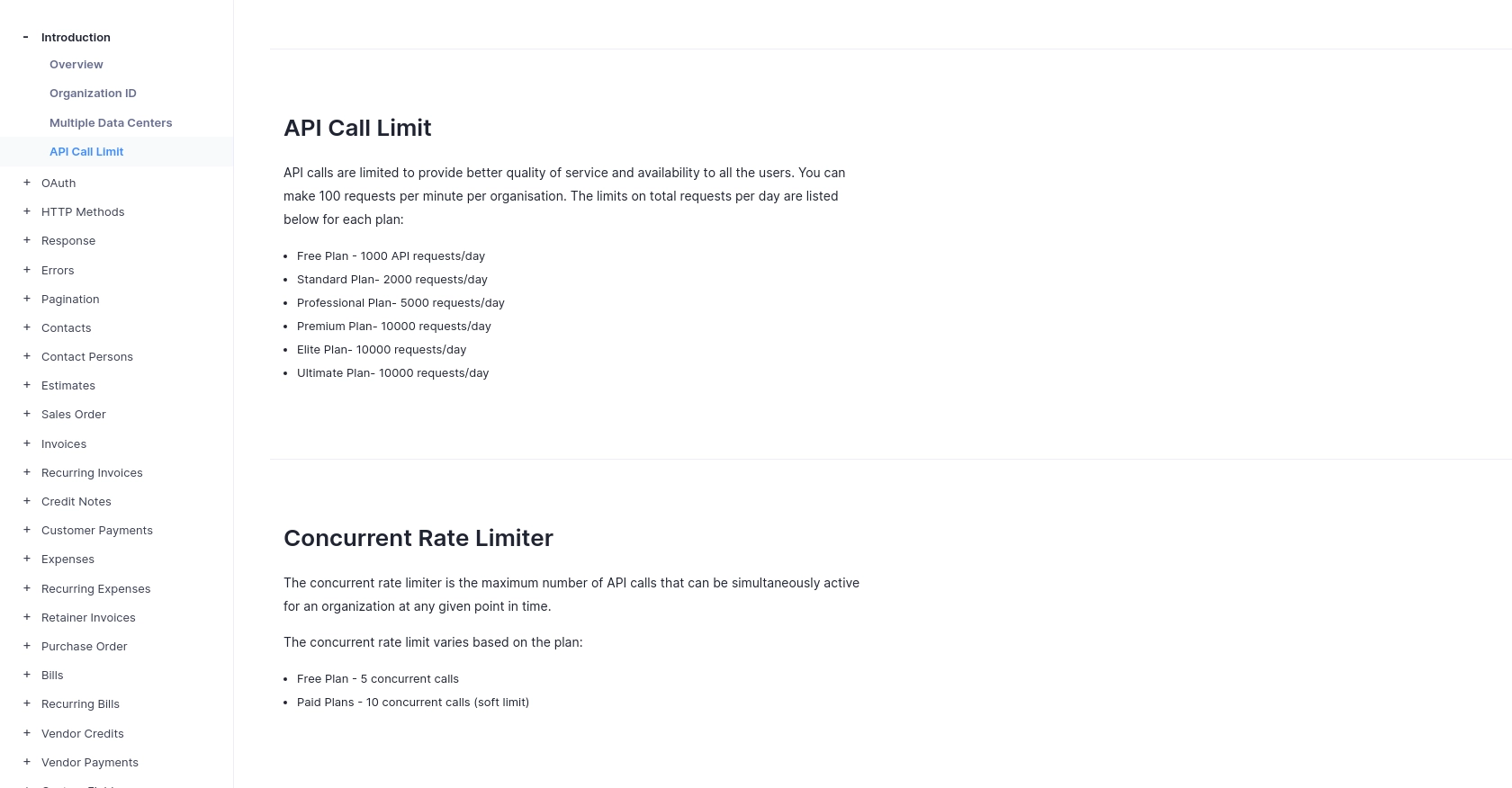
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from Zoho Books Using Python
To interact with the Zoho Books API and retrieve purchase orders, you'll need to use Python. This section will guide you through setting up your environment, making the API call, and handling the response effectively.
Setting Up Your Python Environment for Zoho Books API Integration
Before making API calls, ensure you have the necessary tools and libraries installed:
- Python 3.11.1 or later
- The
requests
library for handling HTTP requests
Install the requests
library using pip:
pip install requests
Constructing the API Request to Retrieve Purchase Orders
With your environment ready, you can construct the API request to retrieve purchase orders. Use the following Python code as a template:
import requests
# Define the API endpoint and headers
organization_id = 'YOUR_ORGANIZATION_ID'
access_token = 'YOUR_ACCESS_TOKEN'
url = f"https://www.zohoapis.com/books/v3/purchaseorders?organization_id={organization_id}"
headers = {
'Authorization': f'Zoho-oauthtoken {access_token}'
}
# Make the GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
purchase_orders = response.json().get('purchaseorders', [])
for order in purchase_orders:
print(f"Purchase Order ID: {order['purchaseorder_id']}, Vendor: {order['vendor_name']}")
else:
print(f"Failed to retrieve purchase orders: {response.status_code} - {response.text}")
Understanding and Handling API Responses from Zoho Books
After making the API call, it's important to handle the response correctly:
- Check the HTTP status code to determine if the request was successful. A status code of
200
indicates success. - Parse the JSON response to extract purchase order details.
- Handle errors by checking for status codes such as
400
(Bad Request),401
(Unauthorized), and429
(Rate Limit Exceeded). Refer to the Zoho Books error documentation for more details.
Verifying API Call Success in Your Zoho Books Account
To ensure the API call was successful, log in to your Zoho Books account and verify the purchase orders retrieved match those in your system. This step helps confirm that your integration is working as expected.
For more information on API call limits, refer to the Zoho Books API call limit documentation.
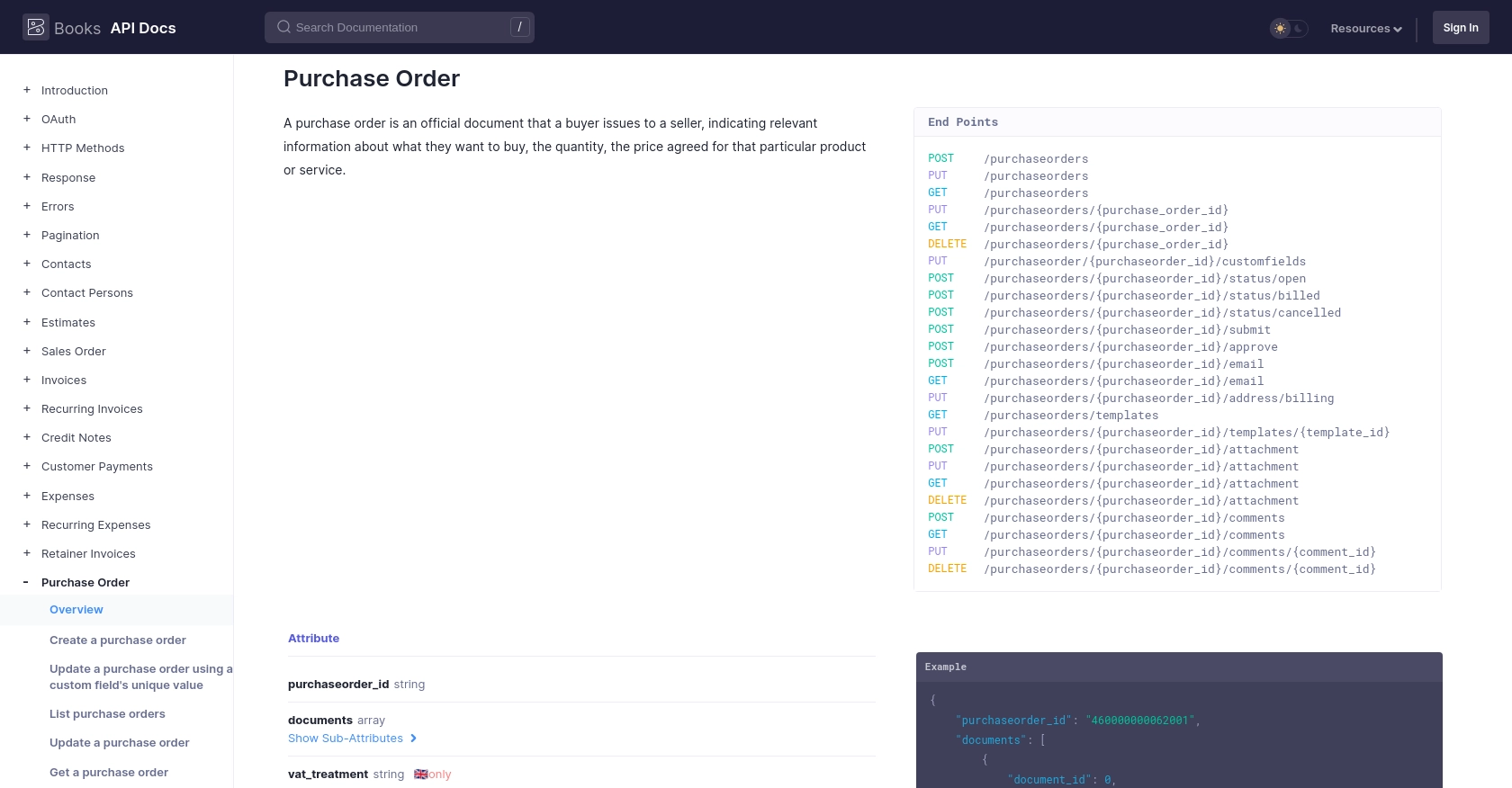
Best Practices for Using Zoho Books API in Python
When working with the Zoho Books API, it's essential to follow best practices to ensure secure and efficient integration. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books API has rate limits. For instance, you can make up to 100 requests per minute per organization. Be sure to implement logic to handle
429
status codes, which indicate rate limit exceedance. Refer to the API call limit documentation for more details. - Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your systems. This can help maintain data integrity across platforms.
- Error Handling: Implement robust error handling to manage different HTTP status codes. For example, handle
400
for bad requests and401
for unauthorized access. Refer to the error documentation for guidance.
Streamlining Integration with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to multiple platforms, including Zoho Books. With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of integration.
- Build Once, Use Everywhere: Develop a single integration that works across various platforms, reducing redundancy.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/purchase-order/#overview
Ready to get started?