How to Create Sales Invoices with the Sage Accounting API in Python
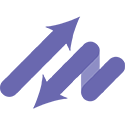
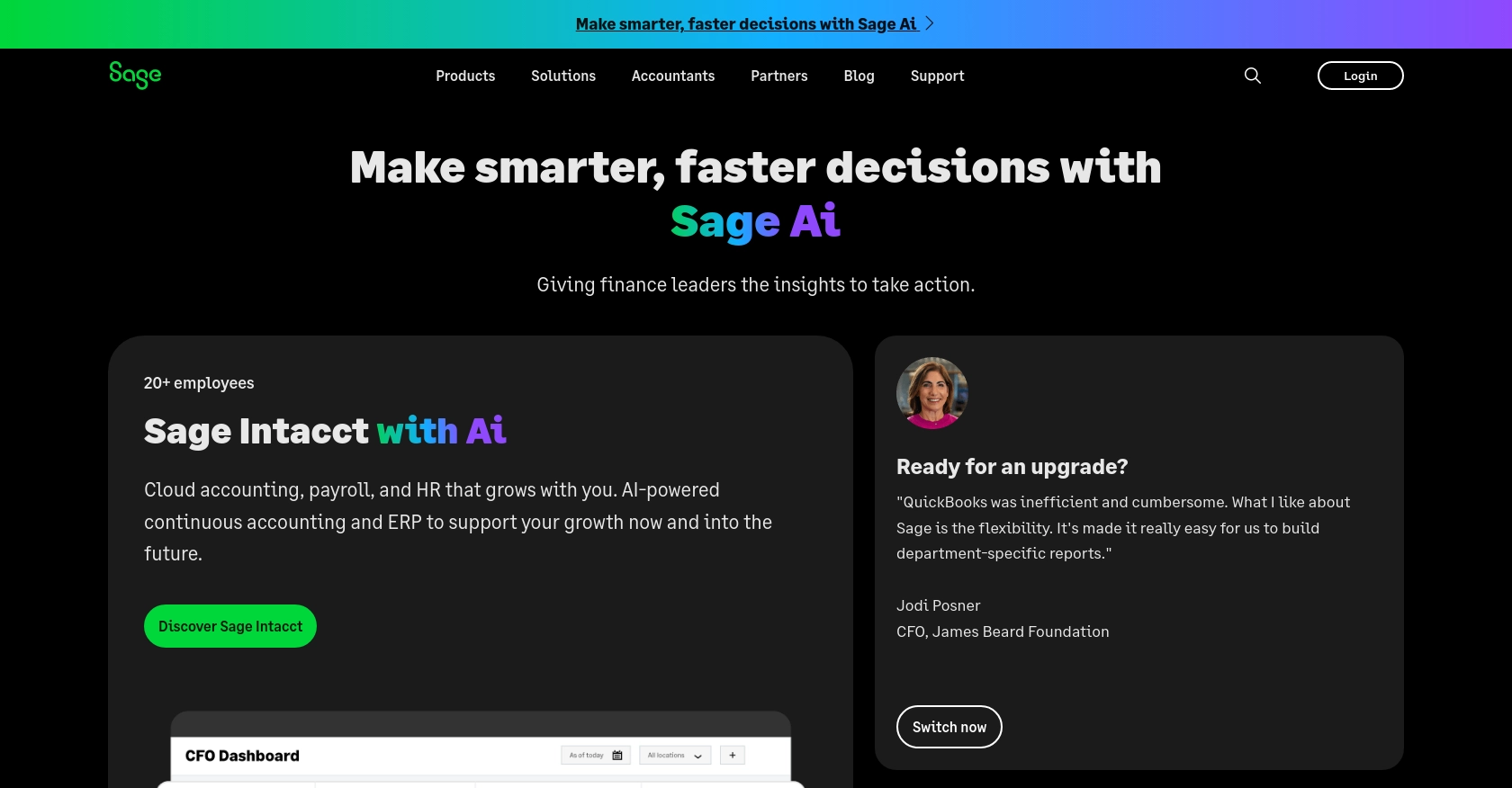
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage financial transactions, invoicing, and reporting, making it a popular choice for businesses seeking to streamline their accounting processes.
Integrating with the Sage Accounting API allows developers to automate and enhance financial operations. For example, creating sales invoices programmatically can save time and reduce errors, enabling businesses to focus on core activities. By using the Sage Accounting API, developers can seamlessly generate invoices, track payments, and manage customer accounts, all within a single platform.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start creating sales invoices using the Sage Accounting API, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Sign Up for a Sage Developer Account
To begin, you need a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the guide on creating an app to obtain your Client ID and Client Secret.
Create a Trial Business Account
Next, set up a trial business account for development purposes. Sage allows you to create trial businesses for all supported regions and multiple subscription tiers.
- Choose the appropriate region and subscription tier from the Sage Business Cloud Accounting options.
- Use email services that support aliasing to manage multiple environments from the same email account.
Register Your Application
Once your developer account and trial business account are ready, you need to register your application to interact with the Sage Accounting API.
- Log in to your Sage Developer account and click on "Create App."
- Enter a name and callback URL for your app. Optionally, provide an alternative email address and homepage URL.
- Save your app to receive your Client ID and Client Secret.
Upgrade to a Developer Account
To fully utilize the API, upgrade your trial account to a developer account, which provides 12 months of free access for testing.
- Submit the details of your trial account, including your name, email, app name, Client ID, and region, via the upgrade form.
- Wait for confirmation from the Sage team, which typically takes 3-5 working days.
With your Sage Accounting test/sandbox account set up, you're now ready to start integrating and creating sales invoices using the API.
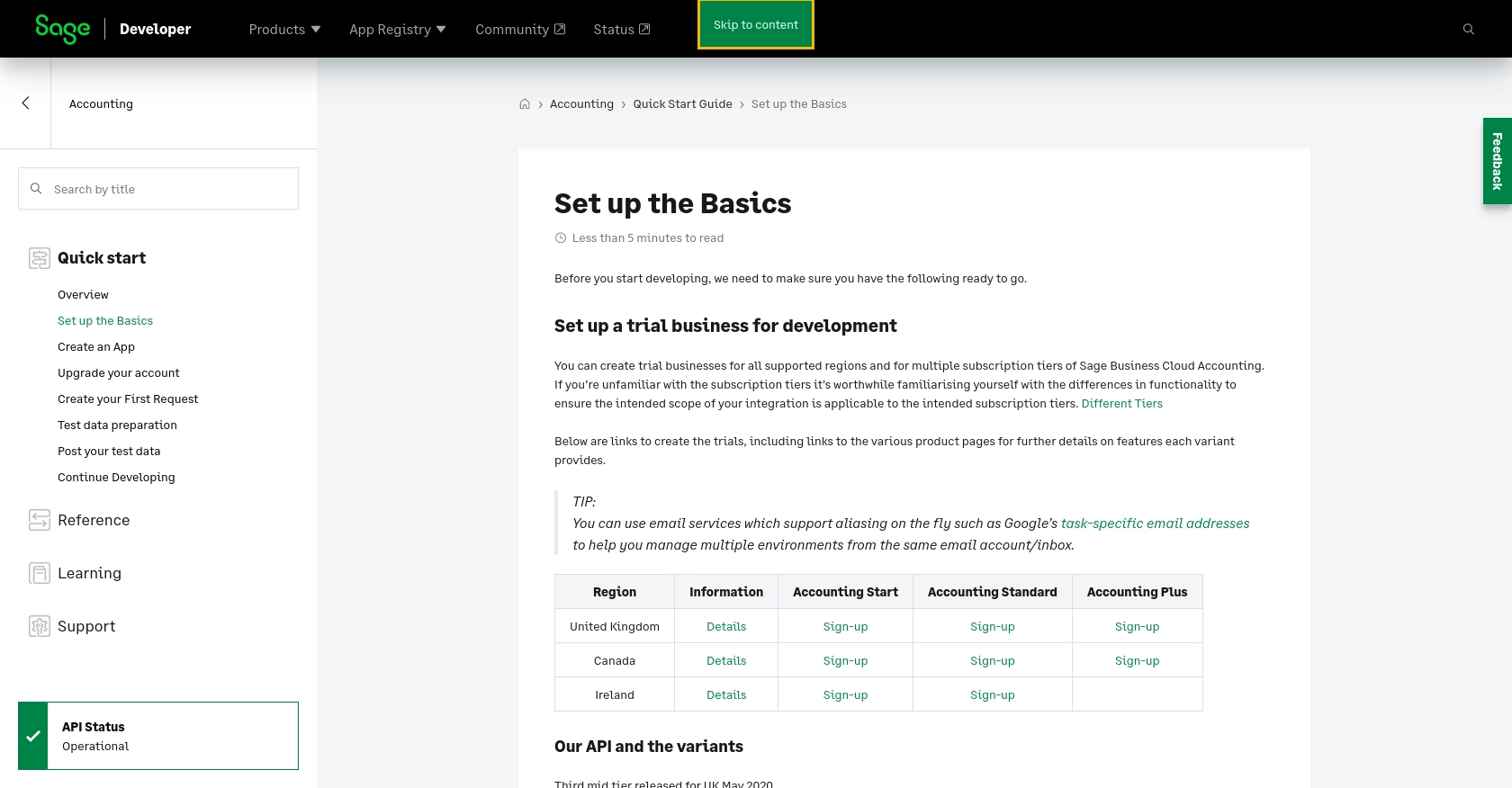
sbb-itb-96038d7
Making API Calls to Create Sales Invoices with Sage Accounting API in Python
To create sales invoices using the Sage Accounting API in Python, you'll need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up Python, installing dependencies, and executing the API call.
Setting Up Your Python Environment for Sage Accounting API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Create Sales Invoices with Sage Accounting API
Now that your environment is ready, you can write the Python code to create a sales invoice. Create a file named create_sales_invoice.py
and add the following code:
import requests
import json
# Set the API endpoint and headers
url = "https://api.sage.com/accounting/v3.1/sales_invoices"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the sales invoice data
invoice_data = {
"contact_id": "123456",
"date": "2023-10-01",
"due_date": "2023-10-15",
"invoice_lines": [
{
"description": "Consulting Services",
"quantity": 10,
"unit_price": 100
}
]
}
# Make a POST request to create the sales invoice
response = requests.post(url, headers=headers, data=json.dumps(invoice_data))
# Check if the request was successful
if response.status_code == 201:
print("Sales Invoice Created Successfully")
print(response.json())
else:
print("Failed to Create Sales Invoice")
print(response.status_code, response.text)
Replace Your_Access_Token
with the access token obtained from your Sage Developer account. The invoice_data
dictionary contains the necessary information to create a sales invoice, such as contact ID, date, due date, and invoice lines.
Executing the Python Script and Verifying the API Call
Run the script from your terminal or command prompt using the following command:
python create_sales_invoice.py
If the request is successful, you should see "Sales Invoice Created Successfully" along with the invoice details. If there is an error, the script will print the status code and error message.
Handling Errors and Verifying in Sage Accounting Sandbox
It's crucial to handle potential errors when making API calls. Common error codes include:
- 400 Bad Request: The request was invalid. Check the data format and required fields.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The endpoint URL is incorrect.
After running the script, log in to your Sage Accounting sandbox account to verify that the sales invoice appears as expected. This ensures that your integration is functioning correctly.
For more detailed information on error codes, refer to the Sage Accounting API documentation.
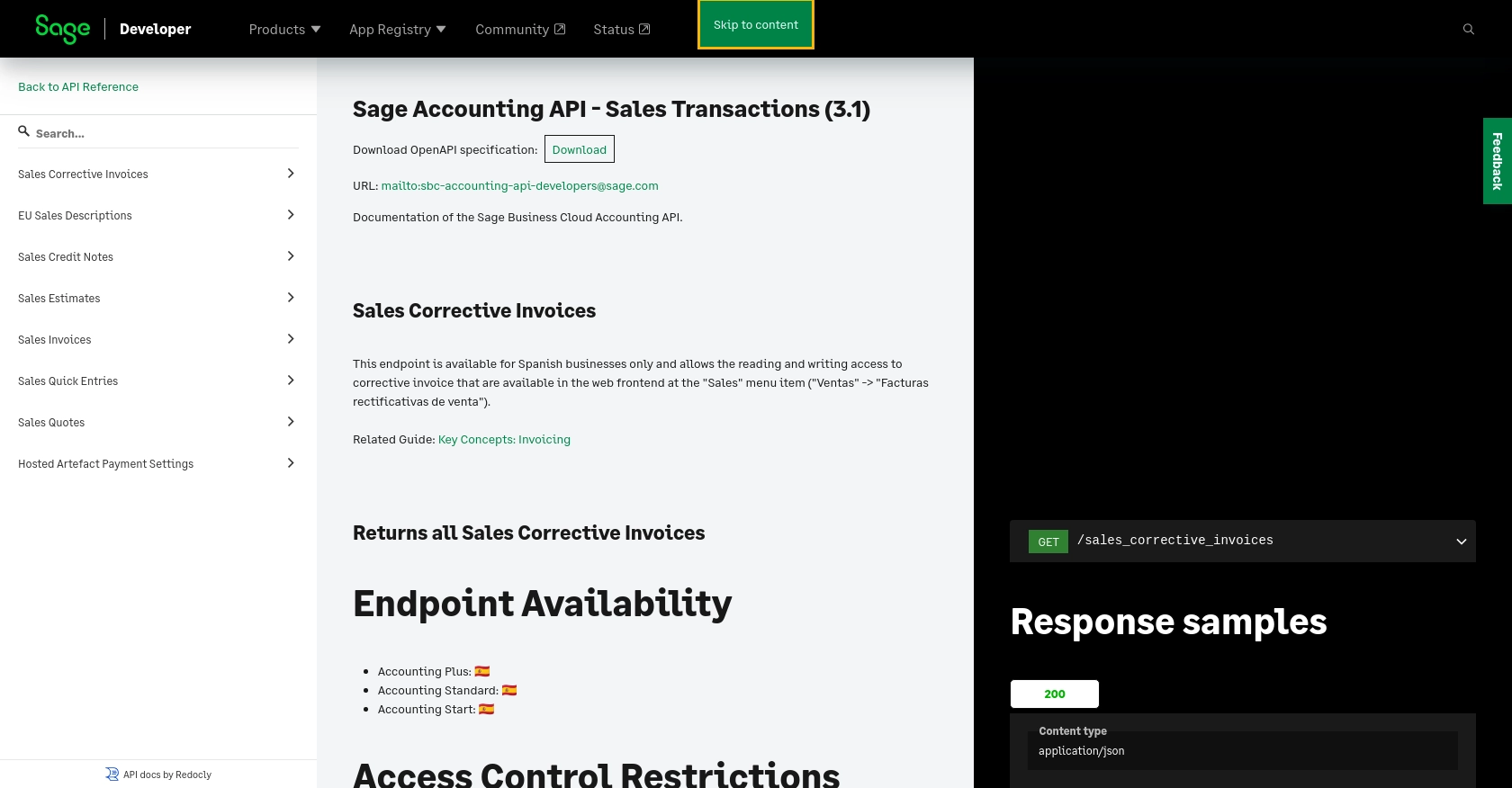
Conclusion and Best Practices for Integrating with Sage Accounting API
Integrating with the Sage Accounting API to create sales invoices in Python can significantly enhance your business's financial operations. By automating invoice creation, you save time, reduce errors, and allow your team to focus on core business activities. However, successful integration requires adherence to best practices to ensure security, efficiency, and reliability.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Validate Data: Ensure that all data sent to the API is validated and sanitized to prevent errors and maintain data integrity.
- Monitor API Usage: Regularly monitor your API usage and logs to detect any anomalies or unauthorized access attempts.
Transforming and Standardizing Data Fields
When integrating with Sage Accounting, it's essential to transform and standardize data fields to match the API's requirements. This ensures consistency and accuracy in your financial records. Consider creating utility functions to handle common data transformations, such as date formatting and currency conversions.
Leverage Endgrate for Seamless Integration
If managing multiple integrations becomes overwhelming, consider using Endgrate to streamline the process. Endgrate offers a unified API endpoint that connects to various platforms, including Sage Accounting, allowing you to build once and deploy across multiple services. This approach saves time and resources, enabling you to focus on your core product development.
By following these best practices and leveraging tools like Endgrate, you can ensure a smooth and efficient integration with the Sage Accounting API, enhancing your business's financial management capabilities.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/invoicing-sales/
Ready to get started?