How to Create Or Update Sales Orders with the Quickbooks API in Python
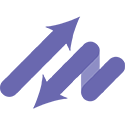
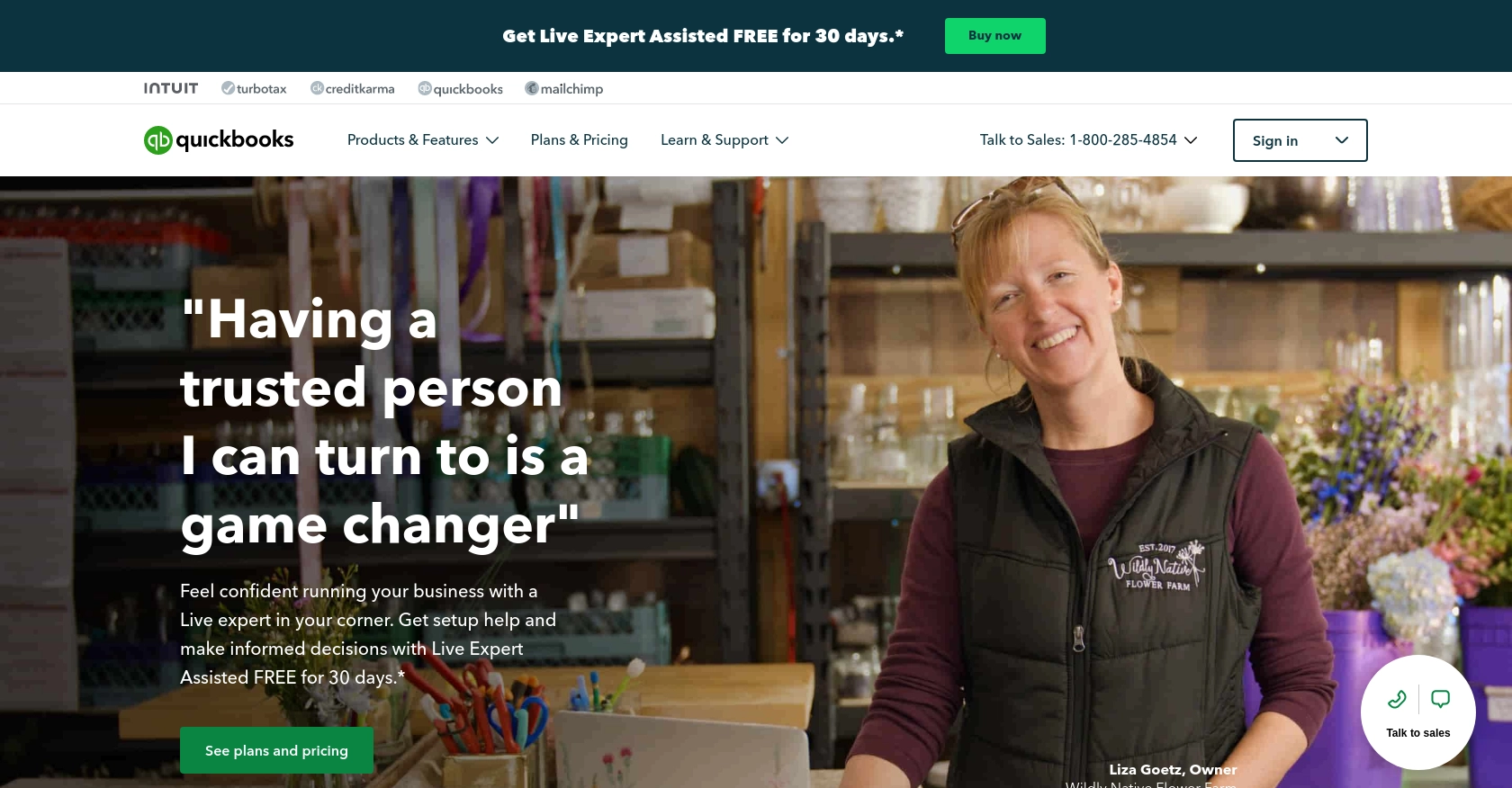
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that helps businesses manage their finances with ease. It offers a comprehensive suite of tools for invoicing, payroll, expense tracking, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate and streamline financial processes, such as creating or updating sales orders. For example, a developer might use the QuickBooks API to automatically generate sales orders from an e-commerce platform, ensuring that financial records are always up-to-date and accurate.
Setting Up Your QuickBooks Test/Sandbox Account
Before you can start integrating with the QuickBooks API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Sign In" if you already have one.
- Complete the registration process by providing the necessary details.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can create a sandbox company:
- Navigate to the "Dashboard" in your QuickBooks Developer account.
- Select "Sandbox" from the menu options.
- Click on "Add Sandbox" to create a new sandbox environment.
- Choose the type of company you want to simulate and follow the prompts to complete the setup.
Creating a QuickBooks App for OAuth Authentication
To interact with the QuickBooks API, you'll need to create an app to obtain the necessary OAuth credentials:
- Go to the App Management section in your developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required information, such as app name and scope of access.
- Once the app is created, navigate to the "Keys & OAuth" section to obtain your Client ID and Client Secret.
Configuring OAuth Settings
After creating your app, configure the OAuth settings:
- In the "Keys & OAuth" section, set the Redirect URI to a valid endpoint in your application.
- Ensure that the OAuth 2.0 settings are correctly configured to allow access to the necessary QuickBooks resources.
With your sandbox account and app set up, you're now ready to start making API calls to QuickBooks. This setup will enable you to test and develop your integration securely.
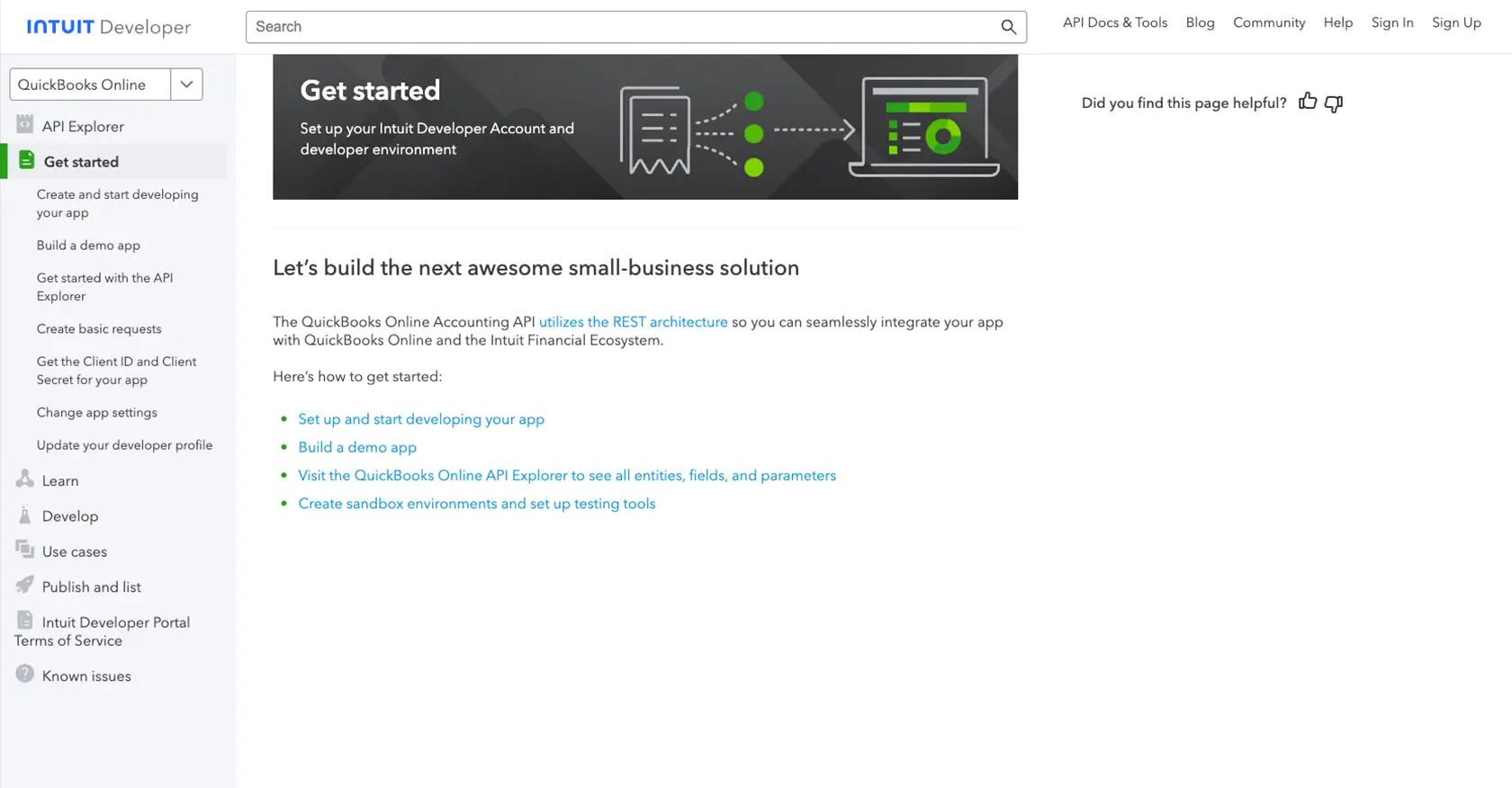
sbb-itb-96038d7
Making API Calls to QuickBooks for Sales Orders Using Python
With your QuickBooks sandbox account and app set up, you can now proceed to make API calls to create or update sales orders using Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code, and handling responses and errors.
Setting Up Your Python Environment for QuickBooks API Integration
To interact with the QuickBooks API using Python, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- pip, the Python package installer
Next, install the requests
library, which will be used to make HTTP requests to the QuickBooks API:
pip install requests
Creating or Updating Sales Orders with QuickBooks API
Now, let's write the Python code to create or update sales orders in QuickBooks. Start by creating a file named quickbooks_sales_order.py
and add the following code:
import requests
# Set the API endpoint for creating or updating sales orders
url = "https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/salesorder"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Define the sales order data
sales_order_data = {
"Line": [
{
"Amount": 100.00,
"DetailType": "SalesItemLineDetail",
"SalesItemLineDetail": {
"ItemRef": {
"value": "1",
"name": "Sample Item"
}
}
}
],
"CustomerRef": {
"value": "1"
}
}
# Make a POST request to create a sales order
response = requests.post(url, json=sales_order_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Sales Order Created/Updated Successfully")
else:
print(f"Failed to Create/Update Sales Order: {response.status_code} - {response.text}")
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token obtained during the OAuth setup.
Verifying API Call Success in QuickBooks Sandbox
After running the script, verify the success of your API call by checking the QuickBooks sandbox environment. If the sales order was created or updated successfully, it should appear in your sandbox company's sales orders list.
Handling Errors and Common Error Codes
When making API calls, it's essential to handle potential errors gracefully. The QuickBooks API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
Ensure your application can handle these errors by checking the response status code and implementing appropriate error-handling logic.
For more detailed information on error codes, refer to the Intuit Developer documentation.
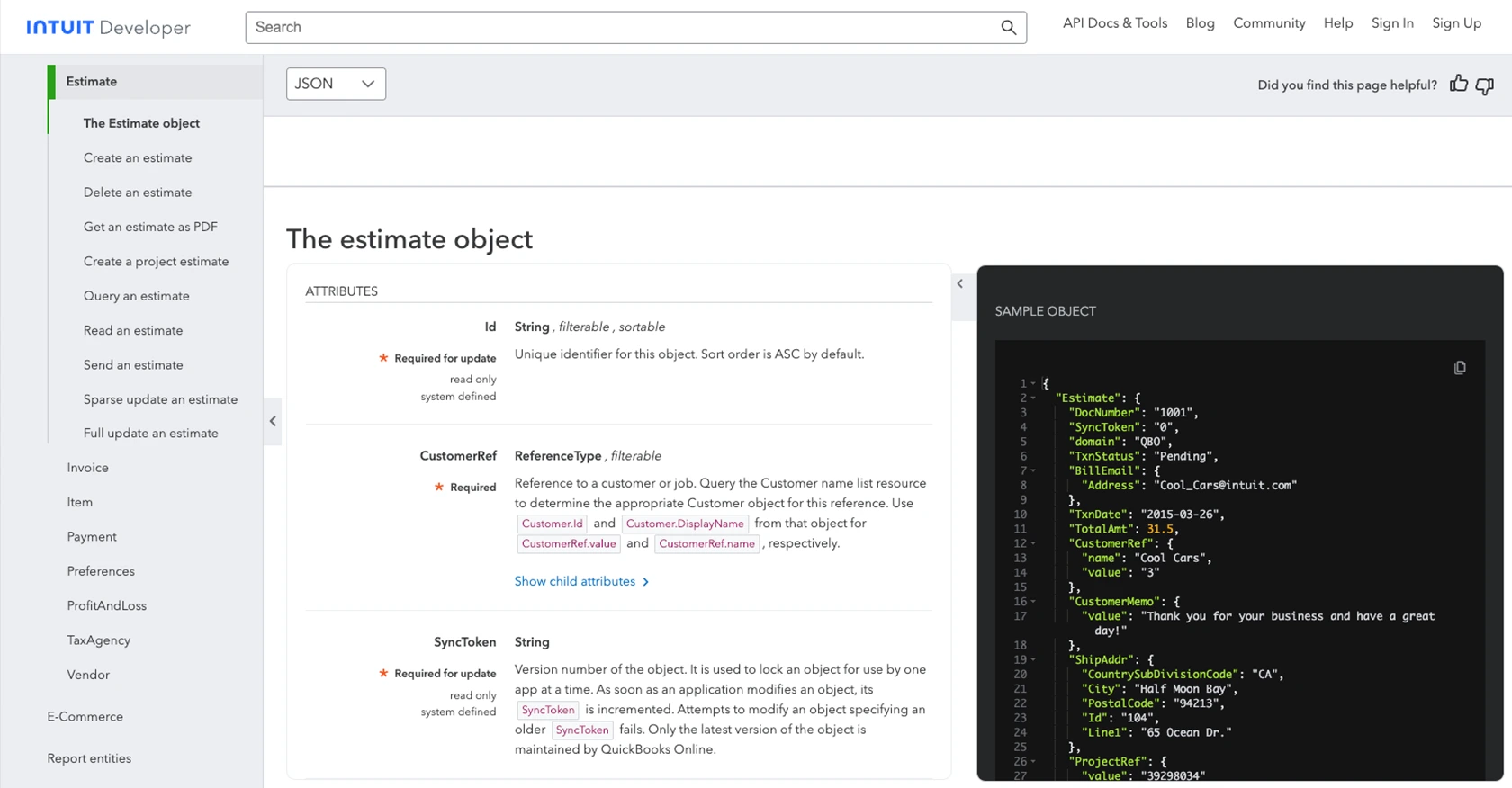
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to create or update sales orders can significantly enhance your business's financial management processes. By automating these tasks, you can ensure accuracy and efficiency, allowing your team to focus on more strategic activities.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your systems to maintain consistency and accuracy in your financial records.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for troubleshooting and provide user-friendly messages where applicable.
Streamlining Integrations with Endgrate
While integrating with QuickBooks API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including QuickBooks.
With Endgrate, you can save time and resources by building integrations once and reusing them across different platforms. This allows you to focus on your core product while delivering a seamless integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover the benefits of a streamlined integration strategy.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/estimate
Ready to get started?