How to Get Companies with the Copper API in Python
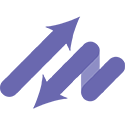
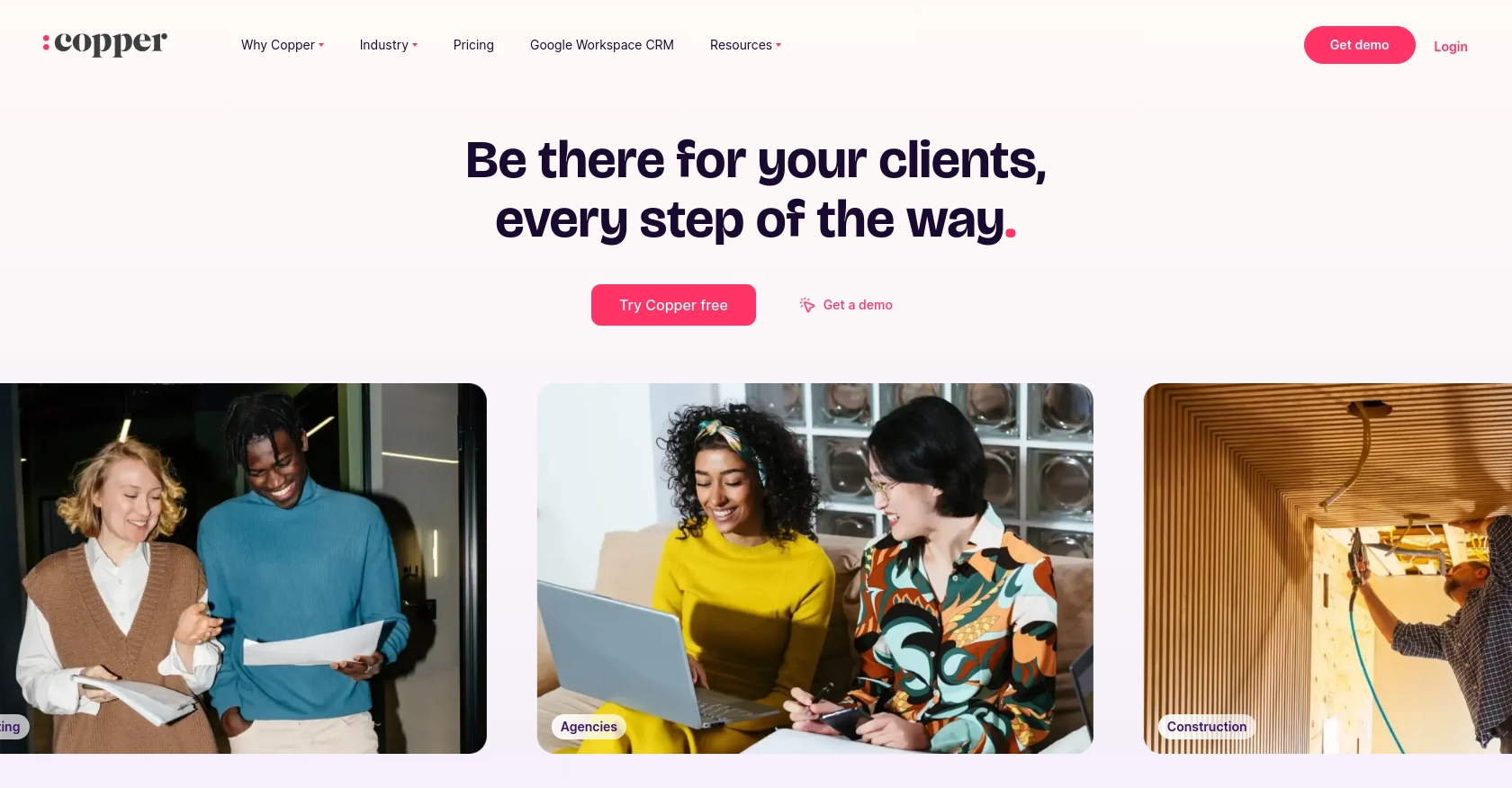
Introduction to Copper API for Company Data Integration
Copper is a powerful platform offering a comprehensive API designed to facilitate seamless integration with your applications. It provides a robust set of tools for managing various financial operations, including cryptocurrency transactions, portfolio management, and more.
Developers might want to integrate with Copper's API to access and manage company data efficiently. For example, retrieving company information can be crucial for financial analysis, reporting, or integrating with other business intelligence tools. By leveraging the Copper API, developers can automate the process of fetching detailed company data, streamlining workflows and enhancing productivity.
Setting Up a Test or Sandbox Account for Copper API Integration
Creating a Copper Sandbox Account for API Testing
To begin integrating with the Copper API, you'll need to set up a sandbox account. This allows you to test API calls without affecting live data. Follow these steps to create your Copper sandbox account:
- Visit the Copper website and navigate to the developer section.
- Sign up for a sandbox account by providing the necessary details.
- Once registered, log in to your sandbox account to access the developer dashboard.
Generating API Keys for Copper API Authentication
Authentication with the Copper API requires an API key and secret. Here's how to generate them:
- In your Copper sandbox account, go to the API settings section.
- Click on "Create API Key" and provide a name for your key.
- Copy the generated API key and secret. Store them securely as they will be used for authenticating your API requests.
Configuring OAuth for Copper API Access
Copper API uses a custom authentication method. Follow these steps to configure OAuth:
- In the API settings, navigate to the OAuth configuration section.
- Create a new OAuth app by providing the required details such as app name, redirect URI, etc.
- Note down the client ID and client secret provided by Copper.
Testing Copper API Calls in the Sandbox Environment
With your API key, secret, and OAuth credentials ready, you can start testing API calls. Use the sandbox environment to ensure your integration works as expected:
import hashlib
import hmac
import requests
import time
ApiKey = 'your_api_key'
Secret = 'your_api_secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/platform/accounts"
body = ""
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
url = 'https://api.stage.copper.co' + path
response = requests.get(url, headers={
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
})
print(response.json())
Replace your_api_key
and your_api_secret
with the values you obtained earlier. This code snippet demonstrates how to authenticate and make a basic API call to retrieve account information.
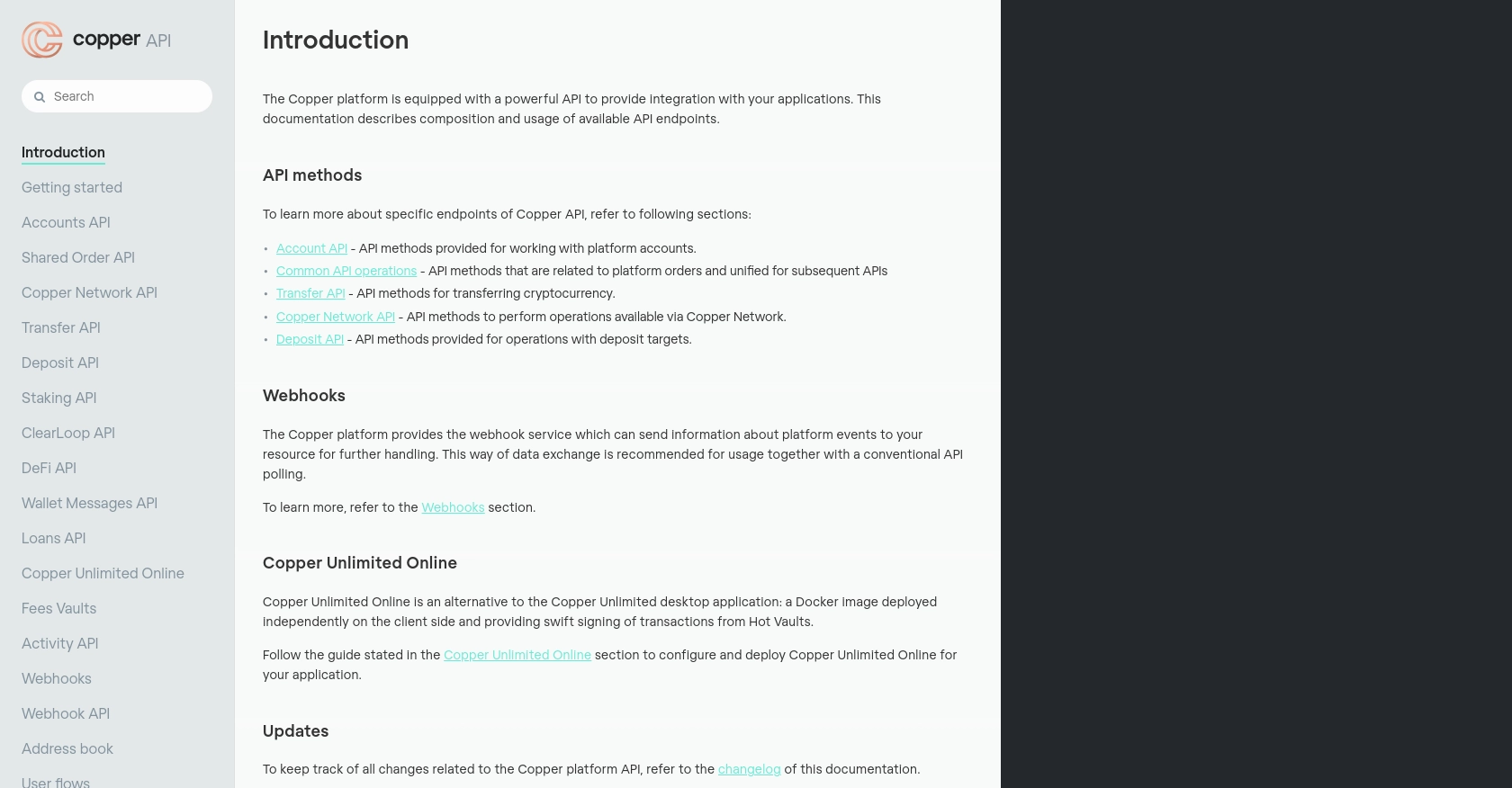
sbb-itb-96038d7
Making API Calls to Retrieve Company Data with Copper API in Python
Understanding Python Setup for Copper API Integration
To interact with the Copper API using Python, ensure you have Python 3.6 or later installed. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Fetch Company Data from Copper API
With your environment set up, you can now write a Python script to retrieve company data using the Copper API. Below is a sample code snippet to get you started:
import hashlib
import hmac
import requests
import time
# Replace with your Copper API credentials
ApiKey = 'your_api_key'
Secret = 'your_api_secret'
# Set the current timestamp and API endpoint
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/platform/companies"
body = ""
# Generate the signature for authentication
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
# Construct the request URL
url = 'https://api.stage.copper.co' + path
# Make the API request
response = requests.get(url, headers={
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
})
# Print the response data
print(response.json())
Ensure you replace your_api_key
and your_api_secret
with your actual Copper API credentials. This script demonstrates how to authenticate and make a GET request to fetch company data.
Handling API Responses and Errors in Copper API Integration
After making an API call, it's crucial to handle the response correctly. Check the status code to verify if the request was successful:
if response.status_code == 200:
print("Success:", response.json())
else:
print("Error:", response.status_code, response.text)
Common error codes include 401 for unauthorized access and 429 for rate limit exceeded. Refer to the Copper API documentation for detailed error handling guidelines.
Verifying Data Retrieval in Copper Sandbox Environment
To ensure the data retrieval process is successful, verify the returned data against your Copper sandbox account. This step helps confirm that your API integration is functioning as expected.
By following these steps, you can efficiently integrate Copper's API into your Python applications, enabling seamless access to company data for enhanced business operations.
Conclusion and Best Practices for Copper API Integration in Python
Integrating with the Copper API using Python can significantly enhance your ability to manage and access company data efficiently. By following the steps outlined in this guide, you can streamline your workflows and improve productivity.
Best Practices for Secure and Efficient Copper API Integration
- Secure Storage of API Credentials: Always store your API key and secret securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Copper API enforces rate limits to ensure fair usage. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the Copper API is transformed and standardized to fit your application's requirements.
- Regularly Review API Documentation: Stay updated with the latest Copper API documentation to leverage new features and adhere to best practices.
Enhance Your Integration Strategy with Endgrate
For developers looking to simplify and scale their integration efforts, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your application's capabilities.
Read More
Ready to get started?