Using the Pipedrive API to Create or Update Leads in PHP
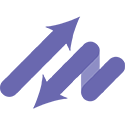
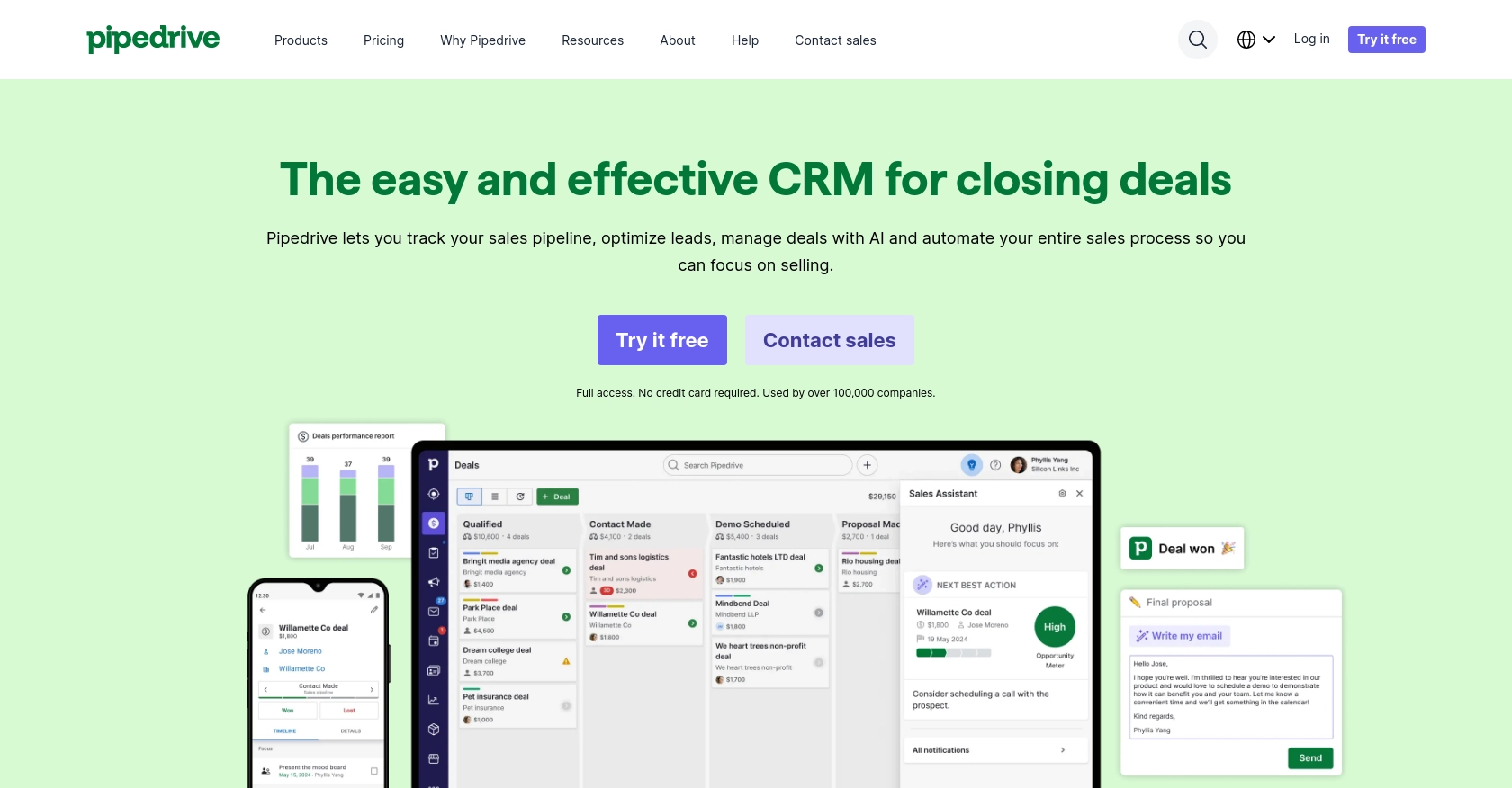
Introduction to Pipedrive CRM and API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage deals, and optimize their sales pipelines efficiently.
For developers, integrating with Pipedrive's API offers the opportunity to automate and enhance sales operations. By connecting with Pipedrive, developers can create or update leads programmatically, ensuring that sales teams have access to the most up-to-date information. For example, a developer might use the Pipedrive API to automatically update lead details from an external marketing platform, streamlining the sales process and improving data accuracy.
Setting Up Your Pipedrive Developer Sandbox Account
Before diving into the integration process with Pipedrive, it's essential to set up a developer sandbox account. This environment allows you to test and develop your application without affecting live data, providing a risk-free space to experiment with the Pipedrive API.
Creating a Pipedrive Developer Sandbox Account
To get started, you'll need to create a developer sandbox account. Follow these steps:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account but is limited to five seats for development and testing purposes.
- Once your account is approved, you'll receive access to the Developer Hub, where you can manage your apps and integrations.
Generating OAuth Credentials for Pipedrive API
Pipedrive uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Here's how to do it:
- Log in to your Pipedrive Developer Hub.
- Navigate to the "Apps" section and click on "Create an App."
- Fill in the required details, such as the app name and description.
- Once the app is created, you'll receive a Client ID and Client Secret. These credentials are crucial for the OAuth flow.
Configuring OAuth Scopes and Permissions
When setting up your app, you'll need to define the scopes and permissions it requires. This ensures that your app only accesses the necessary data:
- Select the scopes that align with your app's functionality, such as accessing leads or managing deals.
- Ensure that the scopes are minimal and specific to your use case to enhance security and user trust.
Testing Your Pipedrive Integration
With your sandbox account and OAuth credentials ready, you can now test your integration:
- Use tools like Postman or Insomnia to simulate API requests and verify responses.
- Ensure that your app handles OAuth flows correctly, including token generation and refresh.
- Test various API endpoints to confirm that your app interacts with Pipedrive as expected.
By setting up a Pipedrive developer sandbox account and configuring OAuth credentials, you're well-prepared to start integrating with Pipedrive's API and enhancing your sales operations.
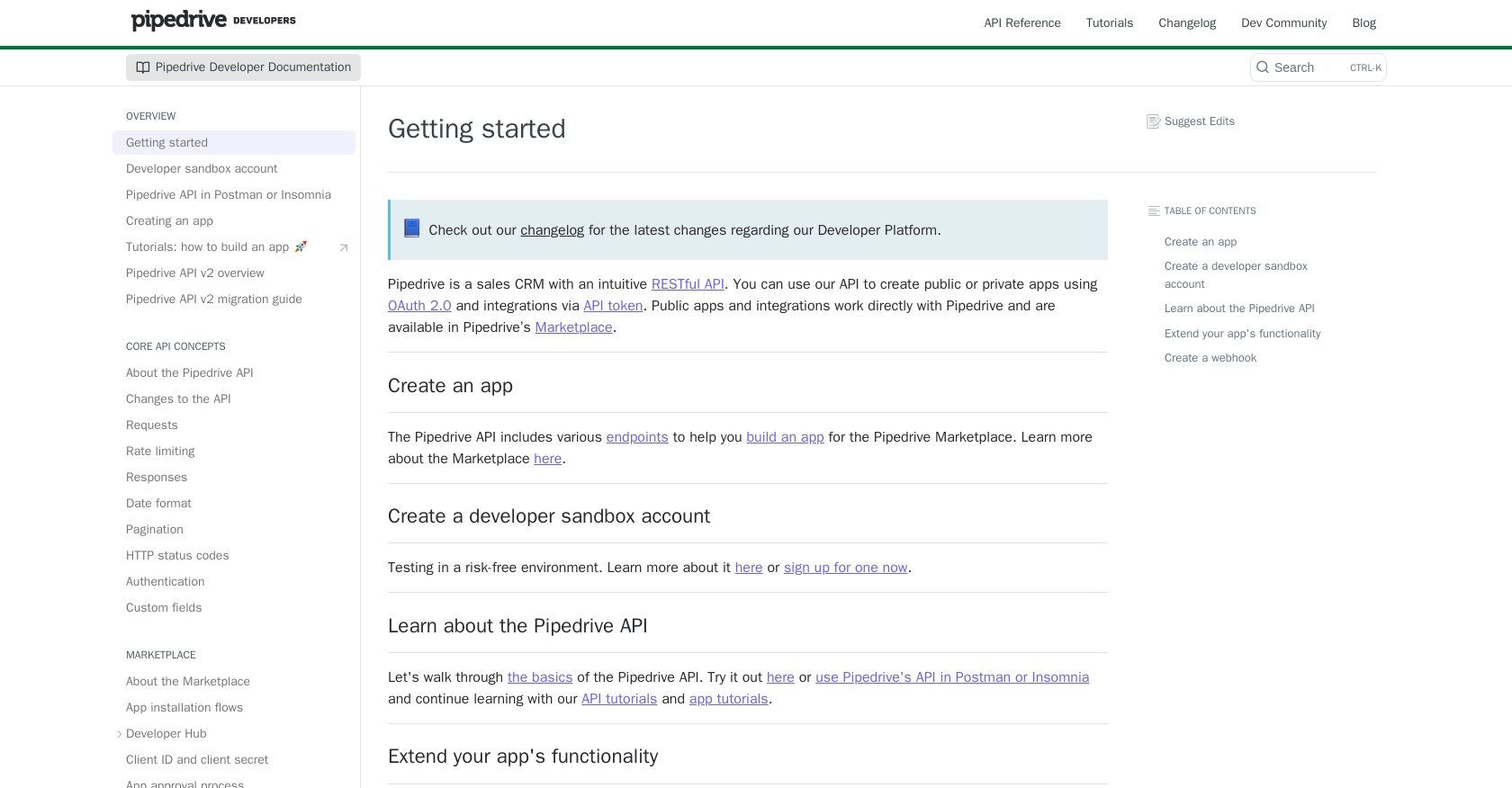
sbb-itb-96038d7
Making API Calls to Pipedrive for Creating or Updating Leads in PHP
To interact with the Pipedrive API using PHP, you need to ensure your environment is properly set up. This section will guide you through the process of making API calls to create or update leads in Pipedrive using PHP.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites installed on your system:
- PHP 7.4 or higher
- cURL extension for PHP
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Creating a Lead in Pipedrive Using PHP
To create a lead in Pipedrive, you'll need to make a POST request to the Pipedrive API. Here's a step-by-step guide:
<?php
$client_id = 'Your_Client_ID';
$client_secret = 'Your_Client_Secret';
$access_token = 'Your_Access_Token';
// API endpoint for creating a lead
$url = 'https://api.pipedrive.com/v1/leads';
// Lead data
$data = [
'title' => 'New Lead from PHP',
'person_id' => 12345,
'organization_id' => 67890,
'value' => [
'amount' => 1000,
'currency' => 'USD'
]
];
// Initialize cURL
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the response
$result = json_decode($response, true);
if ($result['success']) {
echo 'Lead created successfully!';
} else {
echo 'Failed to create lead: ' . $result['error'];
}
?>
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Access_Token
with your actual credentials.
Updating a Lead in Pipedrive Using PHP
To update an existing lead, you need to make a PATCH request. Here's how you can do it:
<?php
$lead_id = 'Lead_ID_To_Update';
// API endpoint for updating a lead
$url = 'https://api.pipedrive.com/v1/leads/' . $lead_id;
// Data to update
$data = [
'title' => 'Updated Lead Title',
'value' => [
'amount' => 1500,
'currency' => 'EUR'
]
];
// Initialize cURL
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PATCH');
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the response
$result = json_decode($response, true);
if ($result['success']) {
echo 'Lead updated successfully!';
} else {
echo 'Failed to update lead: ' . $result['error'];
}
?>
Ensure you replace Lead_ID_To_Update
with the actual ID of the lead you wish to update.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and errors effectively:
- Check the
success
field in the response to determine if the request was successful. - Handle error codes such as 400 (Bad Request) or 401 (Unauthorized) by checking the
error
field in the response. - Refer to the Pipedrive API documentation for a comprehensive list of HTTP status codes and their meanings: Pipedrive HTTP Status Codes.
Verifying API Call Success in Pipedrive
To verify that your API calls have succeeded, log into your Pipedrive sandbox account and check the Leads section. You should see the newly created or updated lead reflected there.
By following these steps, you can efficiently create or update leads in Pipedrive using PHP, enhancing your sales operations through automation.
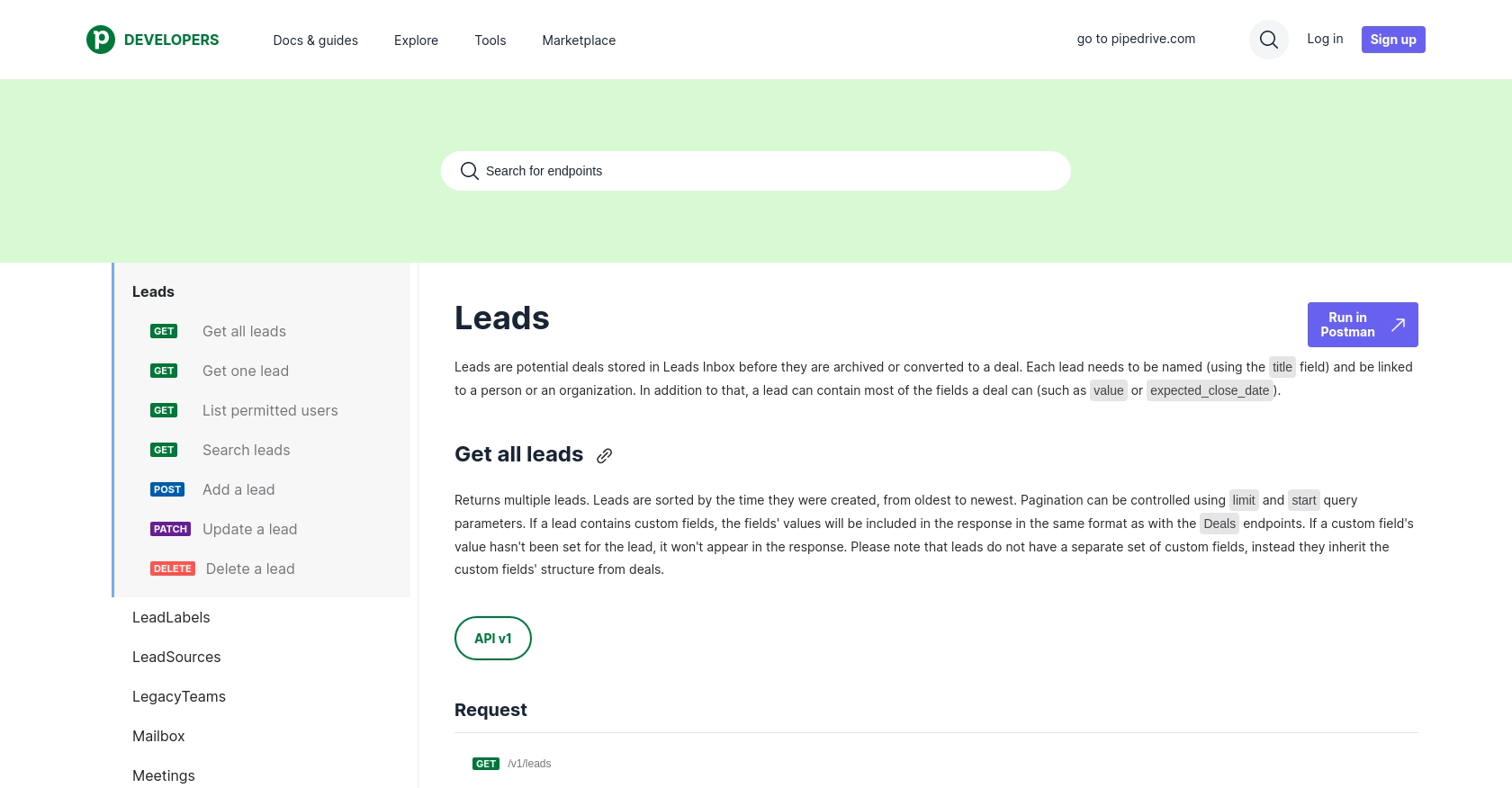
Conclusion: Best Practices for Pipedrive API Integration in PHP
Integrating with the Pipedrive API using PHP can significantly enhance your sales operations by automating lead management processes. To ensure a successful integration, consider the following best practices:
Securely Storing OAuth Credentials
Always store your OAuth credentials, such as the Client ID, Client Secret, and Access Token, securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Pipedrive API Rate Limits
Be mindful of Pipedrive's API rate limits to avoid disruptions. The rate limit for OAuth apps is up to 480 requests per 2 seconds, depending on your plan. Implement logic to handle HTTP 429 "Too Many Requests" responses gracefully by retrying after a delay.
Efficient Error Handling and Logging
Implement robust error handling to manage API responses effectively. Log errors and responses to monitor the integration's performance and troubleshoot issues promptly. Refer to the Pipedrive HTTP Status Codes for guidance on handling different error scenarios.
Data Transformation and Standardization
Ensure that data exchanged between your application and Pipedrive is correctly formatted and standardized. This includes handling custom fields and ensuring data consistency across platforms.
Leverage Endgrate for Streamlined Integrations
Consider using Endgrate to simplify your integration efforts. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint for multiple platforms, offering an intuitive integration experience for your customers.
By following these best practices, you can optimize your use of the Pipedrive API in PHP, enhancing your sales processes and driving business success.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Leads
Ready to get started?