How to Get Salespeople with the Sage 100 API in Javascript
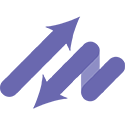
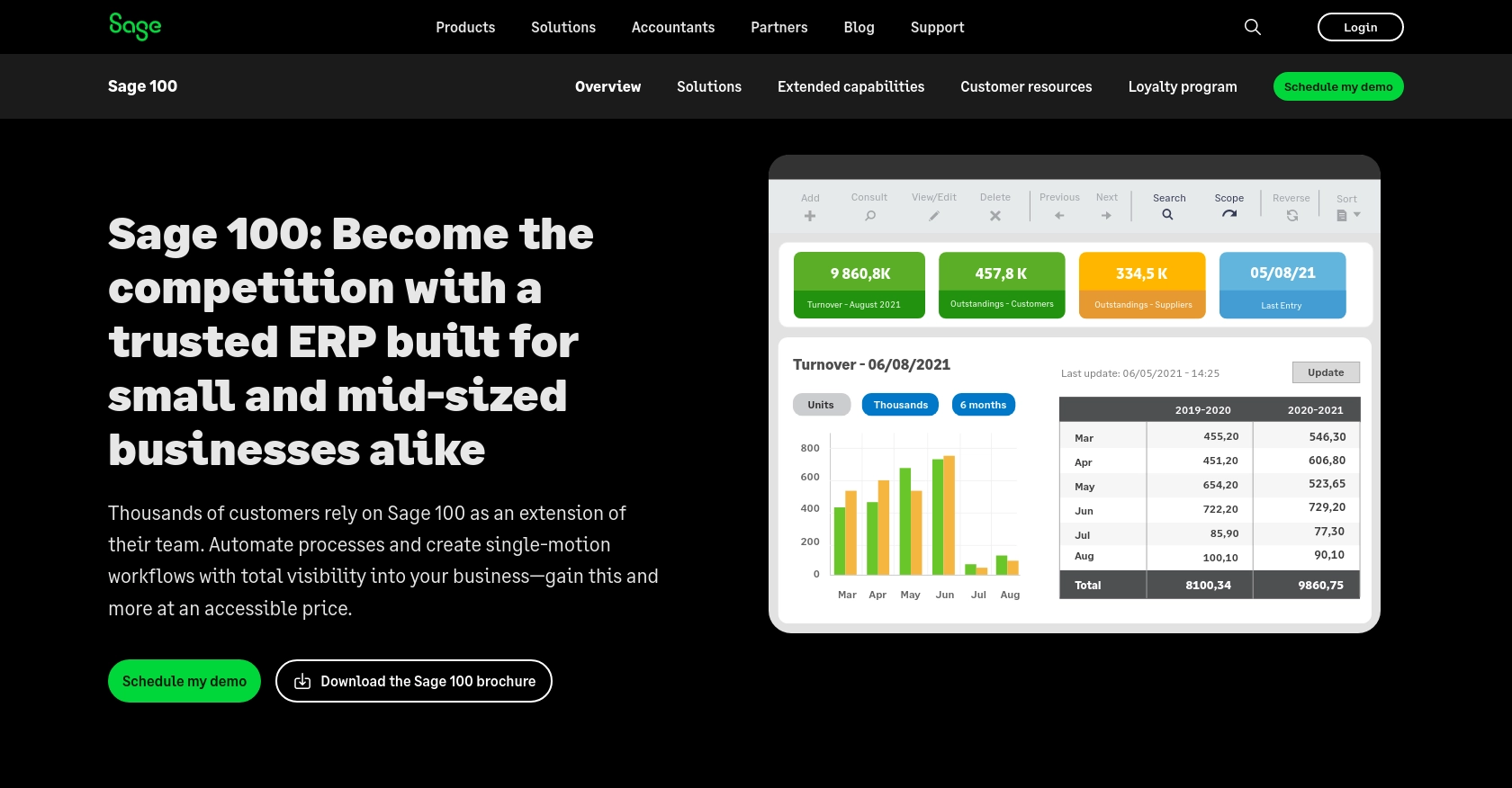
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution tailored for small to medium-sized businesses, offering robust features for accounting, inventory management, and customer relationship management. Its flexibility and scalability make it a popular choice for businesses looking to streamline their operations.
Integrating with the Sage 100 API allows developers to access and manage critical business data, such as salespeople information, directly from their applications. For example, a developer might want to retrieve a list of salespeople to analyze sales performance or synchronize data with other systems.
This article will guide you through the process of using JavaScript to interact with the Sage 100 API, specifically focusing on retrieving salespeople data. By following these steps, you can efficiently access and utilize Sage 100's powerful features in your applications.
Setting Up Your Sage 100 Test Environment
Before you can start interacting with the Sage 100 API, it's essential to set up a test environment. This involves configuring the Sage 100 ODBC driver and creating a Data Source Name (DSN) to connect to the Sage 100 database. This setup allows you to safely test API interactions without affecting live data.
Installing and Configuring the Sage 100 ODBC Driver
To begin, ensure that the Sage 100 ODBC driver is installed on your system. This driver is crucial for establishing a connection to the Sage 100 database. Follow these steps to configure the driver:
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Refer to the official Sage 100 documentation for detailed instructions on configuring the ODBC driver.
Creating a Data Source Name (DSN)
Once the ODBC driver is installed, you need to create a DSN to facilitate the connection:
- Open the ODBC Data Source Administrator.
- Navigate to the 'User DSN' tab and click 'Add'.
- Select the Sage 100 ODBC driver from the list and click 'Finish'.
- In the setup window, enter the necessary connection details, including the server name and database credentials.
- Test the connection to ensure everything is configured correctly.
Configuring Sage 100 for API Access
After setting up the DSN, configure Sage 100 to allow API access:
- In Sage 100, navigate to Library Master > Setup > System Configuration.
- On the ODBC Driver tab, select the Enable C/S ODBC Driver checkbox.
- Enter the server name or IP address where the ODBC application or service is running.
- Specify the server port or leave it blank to use the default port, 20222.
Once these steps are completed, your Sage 100 test environment is ready for API interactions.
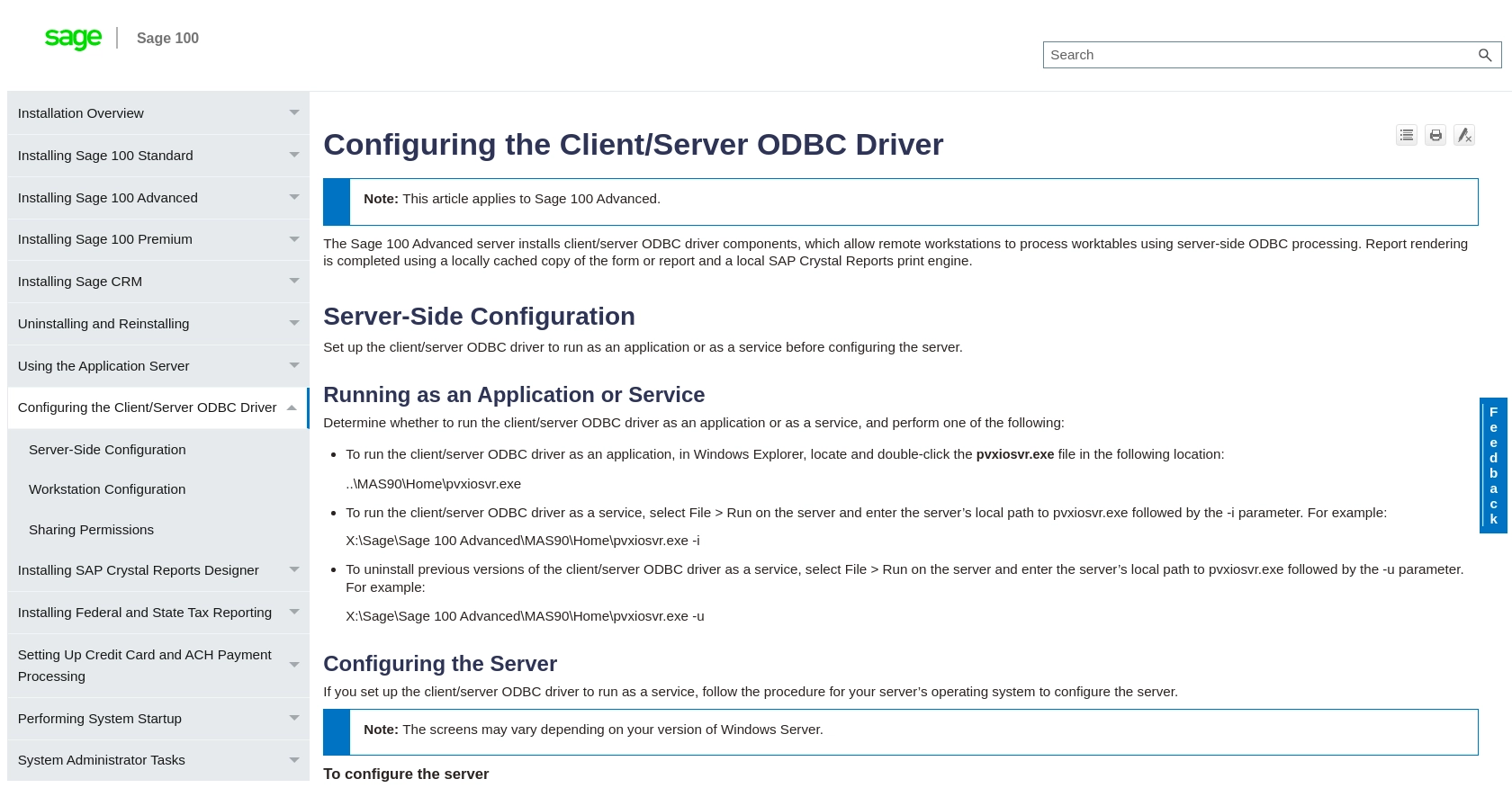
sbb-itb-96038d7
Making API Calls to Retrieve Salespeople Data from Sage 100 Using JavaScript
To interact with the Sage 100 API and retrieve salespeople data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Sage 100 API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a JavaScript runtime environment that allows you to execute JavaScript code outside of a web browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions. - Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the
axios
library for making HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Access Sage 100 API
With your environment set up, you can now write the JavaScript code to retrieve salespeople data from the Sage 100 API.
const axios = require('axios');
// Define the API endpoint and DSN
const endpoint = 'https://your-sage-100-api-endpoint/salespeople';
const dsn = 'Your_DSN_Name';
// Function to get salespeople data
async function getSalespeople() {
try {
const response = await axios.get(endpoint, {
headers: {
'Content-Type': 'application/json',
'DSN': dsn
}
});
console.log('Salespeople Data:', response.data);
} catch (error) {
console.error('Error fetching salespeople data:', error);
}
}
// Call the function
getSalespeople();
Replace https://your-sage-100-api-endpoint/salespeople
with the actual API endpoint for your Sage 100 instance and Your_DSN_Name
with your Data Source Name.
Verifying API Call Success and Handling Errors
After executing the code, verify the success of your API call by checking the console output. If the call is successful, you should see the salespeople data printed in the console.
In case of errors, the catch
block will log the error details. Common issues might include incorrect endpoint URLs, network problems, or authentication failures. Ensure your DSN and API endpoint are correctly configured.
For more detailed information on error codes and handling, refer to the Sage 100 API documentation.
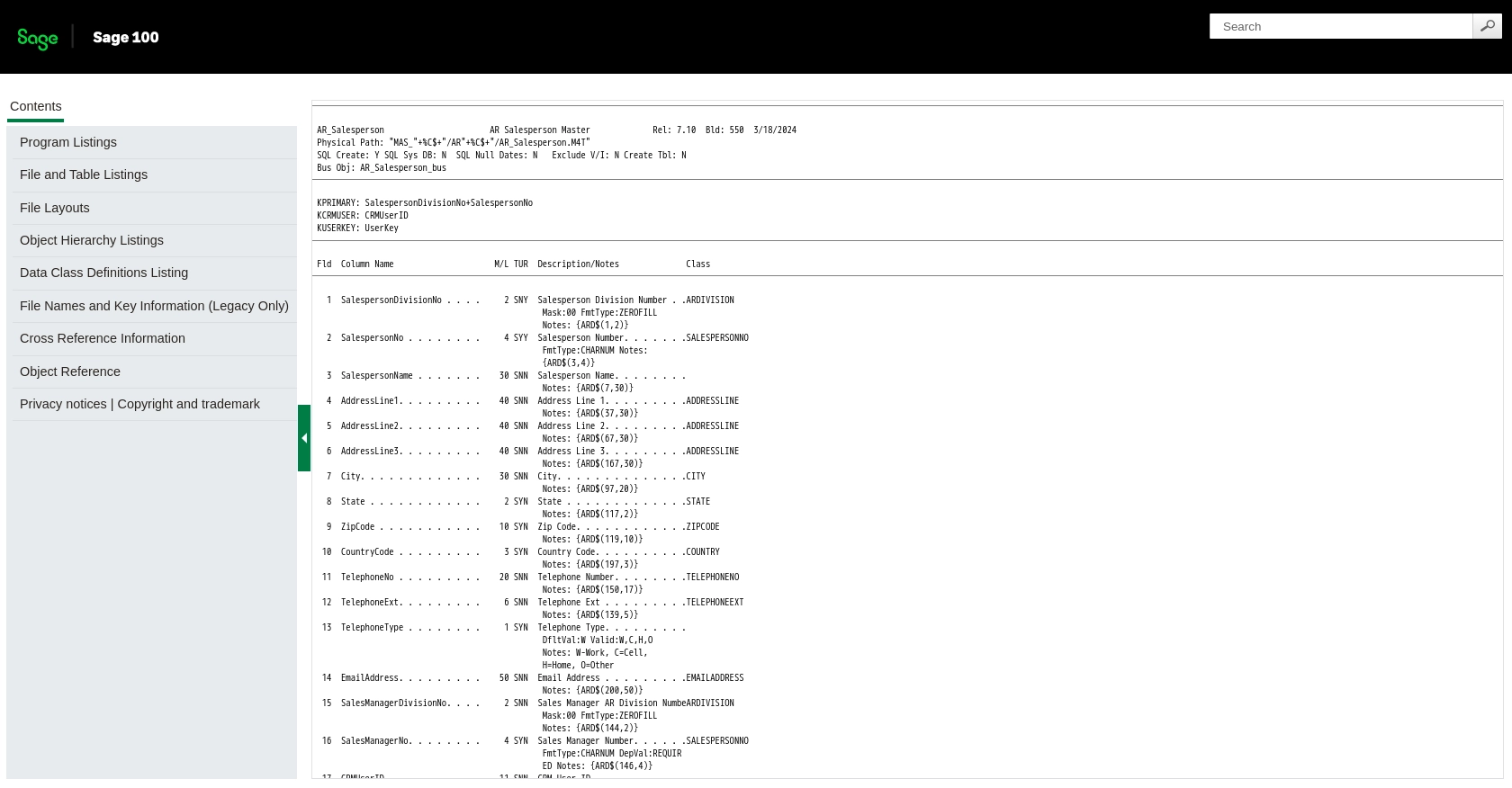
Conclusion and Best Practices for Sage 100 API Integration Using JavaScript
Integrating with the Sage 100 API using JavaScript provides a powerful way to access and manage business data, such as salespeople information, directly from your applications. By following the steps outlined in this guide, you can efficiently retrieve and utilize Sage 100's features to enhance your business operations.
Best Practices for Secure and Efficient Sage 100 API Usage
- Securely Store Credentials: Ensure that your DSN and any other sensitive information are stored securely. Consider using environment variables or secure vaults to manage credentials.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: When retrieving data, consider transforming and standardizing it to fit your application's data model. This will help maintain consistency across different systems.
- Error Handling: Implement comprehensive error handling to manage potential issues such as network failures or incorrect configurations. Use logging to capture error details for troubleshooting.
Streamlining Integrations with Endgrate
While integrating with the Sage 100 API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Sage 100.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Whether you're building once for each use case or aiming for an intuitive integration experience for your customers, Endgrate simplifies the process.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration approach.
Read More
Ready to get started?