Using the Capsule API to Create or Update People (with Python examples)
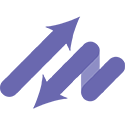
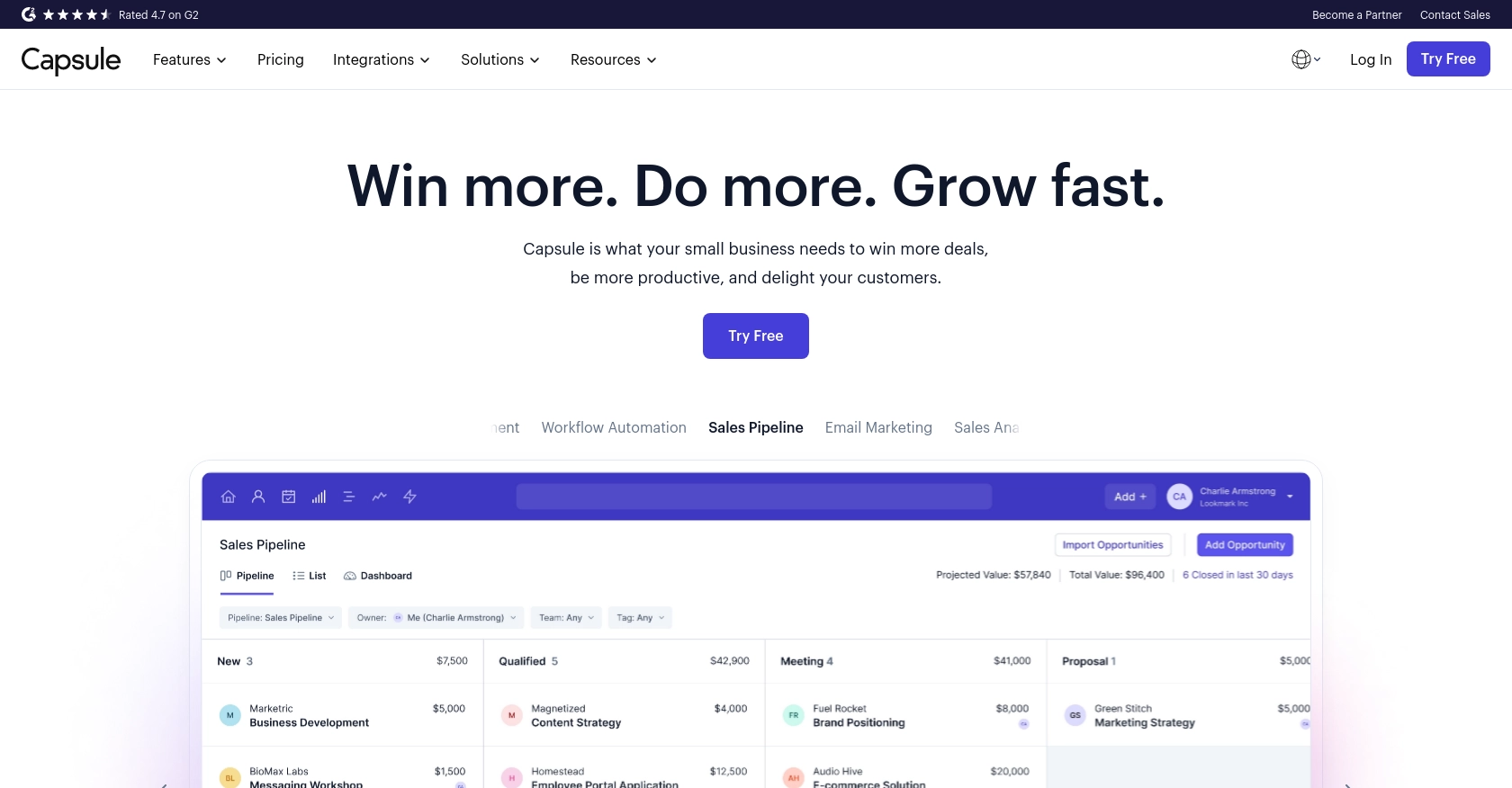
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform designed to help businesses manage their contacts, sales opportunities, and customer interactions effectively. Known for its simplicity and powerful features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Integrating with Capsule CRM's API allows developers to automate and enhance CRM functionalities, such as creating or updating contact information. For example, a developer might use the Capsule API to automatically update customer details from an external source, ensuring that the CRM data remains current and accurate.
Setting Up Your Capsule CRM Test or Sandbox Account
Before you begin integrating with the Capsule API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Capsule CRM offers a straightforward process to get started with their API using OAuth 2 authentication.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on their website. This will give you access to all the features needed for testing and development.
- Visit the Capsule CRM signup page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Registering a Capsule CRM Application for OAuth 2 Authentication
To interact with the Capsule API, you'll need to register your application to obtain the necessary OAuth 2 credentials.
- Navigate to the "My Preferences" section in your Capsule account.
- Select "API Authentication Tokens" to create a new token.
- Register your application by providing the required details, such as application name and redirect URI.
- Once registered, you'll receive a client ID and client secret. Keep these credentials secure, as they are essential for API authentication.
Generating an OAuth 2 Bearer Token
With your application registered, you can now generate a bearer token to authenticate API requests.
- Direct users to the Capsule authorization URL:
https://api.capsulecrm.com/oauth/authorise
. - After user approval, Capsule will redirect to your specified redirect URI with an authorization code.
- Exchange this code for an access token by making a POST request to the token exchange URL:
https://api.capsulecrm.com/oauth/token
. - Include the following parameters in your request:
- Upon success, you'll receive a JSON response containing the access token, which you can use in API requests.
{
"code": "your_authorization_code",
"client_id": "your_client_id",
"client_secret": "your_client_secret",
"grant_type": "authorization_code"
}
For more detailed information on authentication, refer to the Capsule API Authentication documentation.
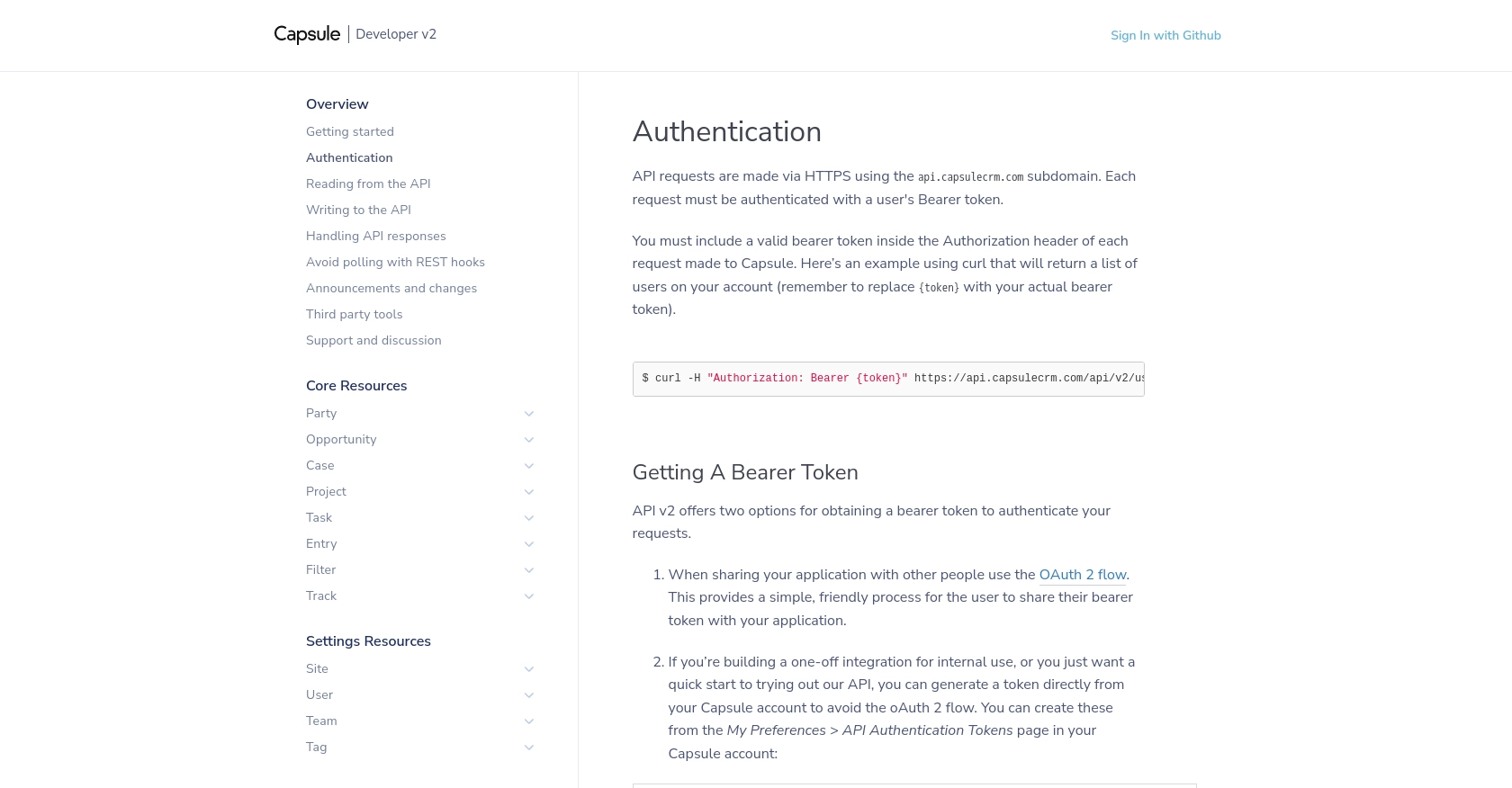
sbb-itb-96038d7
Making API Calls to Create or Update People in Capsule CRM Using Python
To interact with the Capsule API and manage people records, you'll need to make HTTP requests using Python. This section will guide you through the process of creating and updating people in Capsule CRM using Python's requests library.
Prerequisites for Capsule API Integration with Python
Before proceeding, ensure you have the following installed on your system:
- Python 3.11.1 or later
- pip, the Python package installer
Install the requests library by running the following command in your terminal:
pip install requests
Creating a New Person in Capsule CRM
To create a new person in Capsule CRM, you'll need to send a POST request to the Capsule API with the person's details. Here's a step-by-step guide:
- Create a new Python file named
create_person.py
and add the following code:
import requests
# Set the API endpoint
url = "https://api.capsulecrm.com/api/v2/parties"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the person data
person_data = {
"party": {
"type": "person",
"firstName": "John",
"lastName": "Doe",
"emailAddresses": [
{"type": "Work", "address": "john.doe@example.com"}
]
}
}
# Send the POST request
response = requests.post(url, json=person_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Person created successfully:", response.json())
else:
print("Failed to create person:", response.status_code, response.json())
Replace Your_Access_Token
with the access token obtained from the OAuth 2 process. Run the script using:
python create_person.py
Upon success, the response will include the details of the newly created person.
Updating an Existing Person in Capsule CRM
To update an existing person's details, you'll need to send a PUT request with the updated information. Follow these steps:
- Create a new Python file named
update_person.py
and add the following code:
import requests
# Set the API endpoint with the person's ID
person_id = "12345" # Replace with the actual person ID
url = f"https://api.capsulecrm.com/api/v2/parties/{person_id}"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the updated person data
update_data = {
"party": {
"firstName": "John",
"lastName": "Smith",
"emailAddresses": [
{"id": 12134, "type": "Work", "address": "john.smith@example.com"}
]
}
}
# Send the PUT request
response = requests.put(url, json=update_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Person updated successfully:", response.json())
else:
print("Failed to update person:", response.status_code, response.json())
Replace Your_Access_Token
and person_id
with the appropriate values. Run the script using:
python update_person.py
Upon success, the response will include the updated details of the person.
Handling Errors and Verifying API Requests in Capsule CRM
When making API calls, it's crucial to handle potential errors. Capsule API responses include status codes and error messages that can help diagnose issues:
- 401 Unauthorized: Check if the access token is valid and not expired.
- 403 Forbidden: Ensure the token has the necessary permissions.
- 422 Unprocessable Entity: Verify that all required fields are correctly formatted.
For more detailed error handling, refer to the Capsule API Handling API Responses documentation.
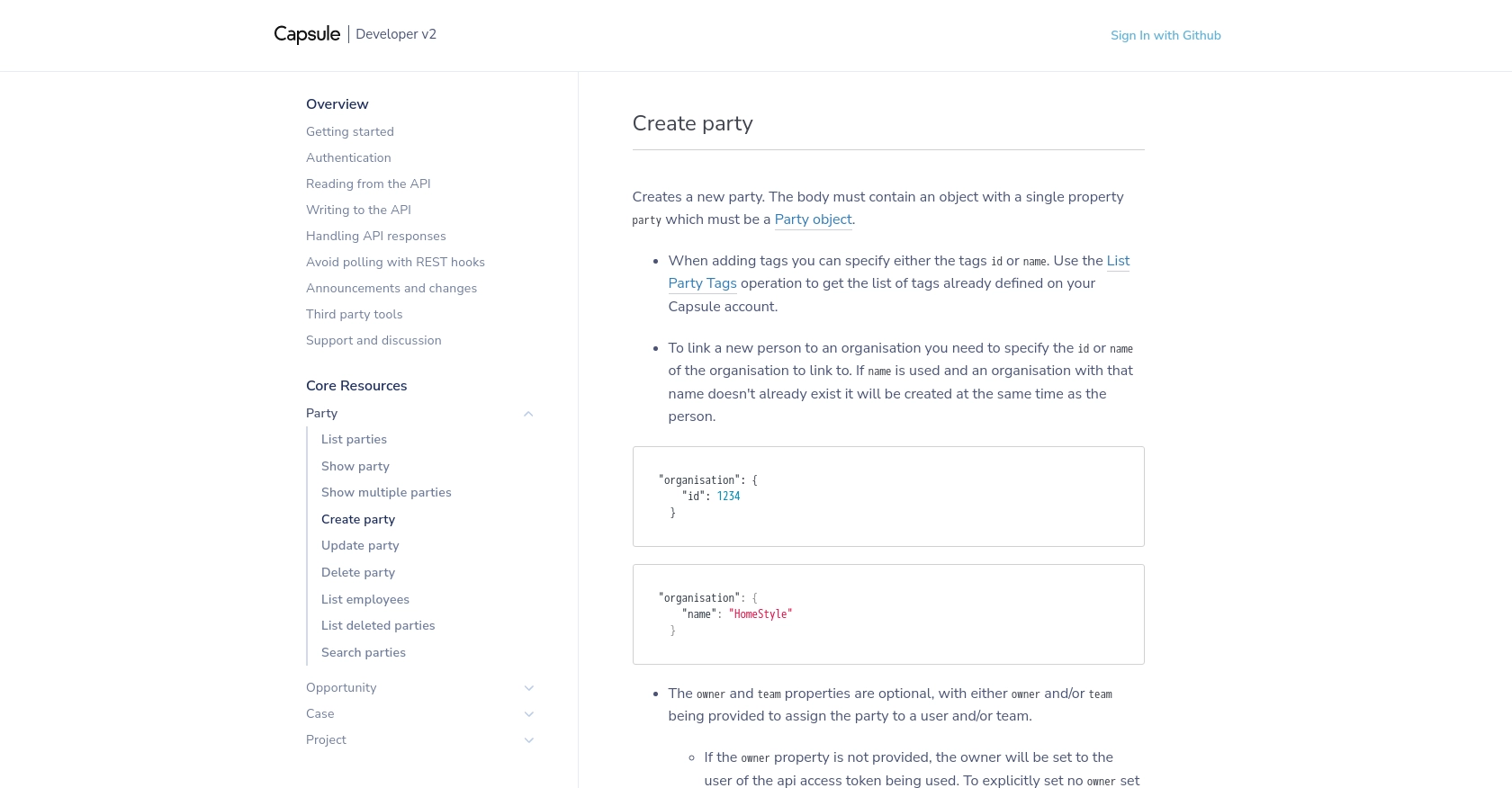
Conclusion and Best Practices for Capsule API Integration
Integrating with Capsule CRM's API offers significant advantages for automating and enhancing CRM functionalities. By following the steps outlined in this guide, you can efficiently create and update people records using Python, ensuring your CRM data is always up-to-date.
Best Practices for Secure and Efficient Capsule API Usage
- Securely Store Credentials: Always keep your client ID, client secret, and access tokens secure. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Capsule API allows up to 4,000 requests per hour. Monitor the
X-RateLimit-Remaining
header to avoid exceeding this limit. Implement exponential backoff strategies if you encounter rate limit errors. - Data Standardization: Ensure consistent data formats when creating or updating records. This helps maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage API response codes effectively. This includes retry mechanisms for transient errors and logging for debugging purposes.
Leverage Endgrate for Streamlined Integration Management
While integrating with Capsule CRM can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint for various integrations, including Capsule CRM. By using Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#createParty
Ready to get started?