Using the Odoo Online API to Get Records (with Javascript examples)
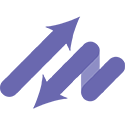
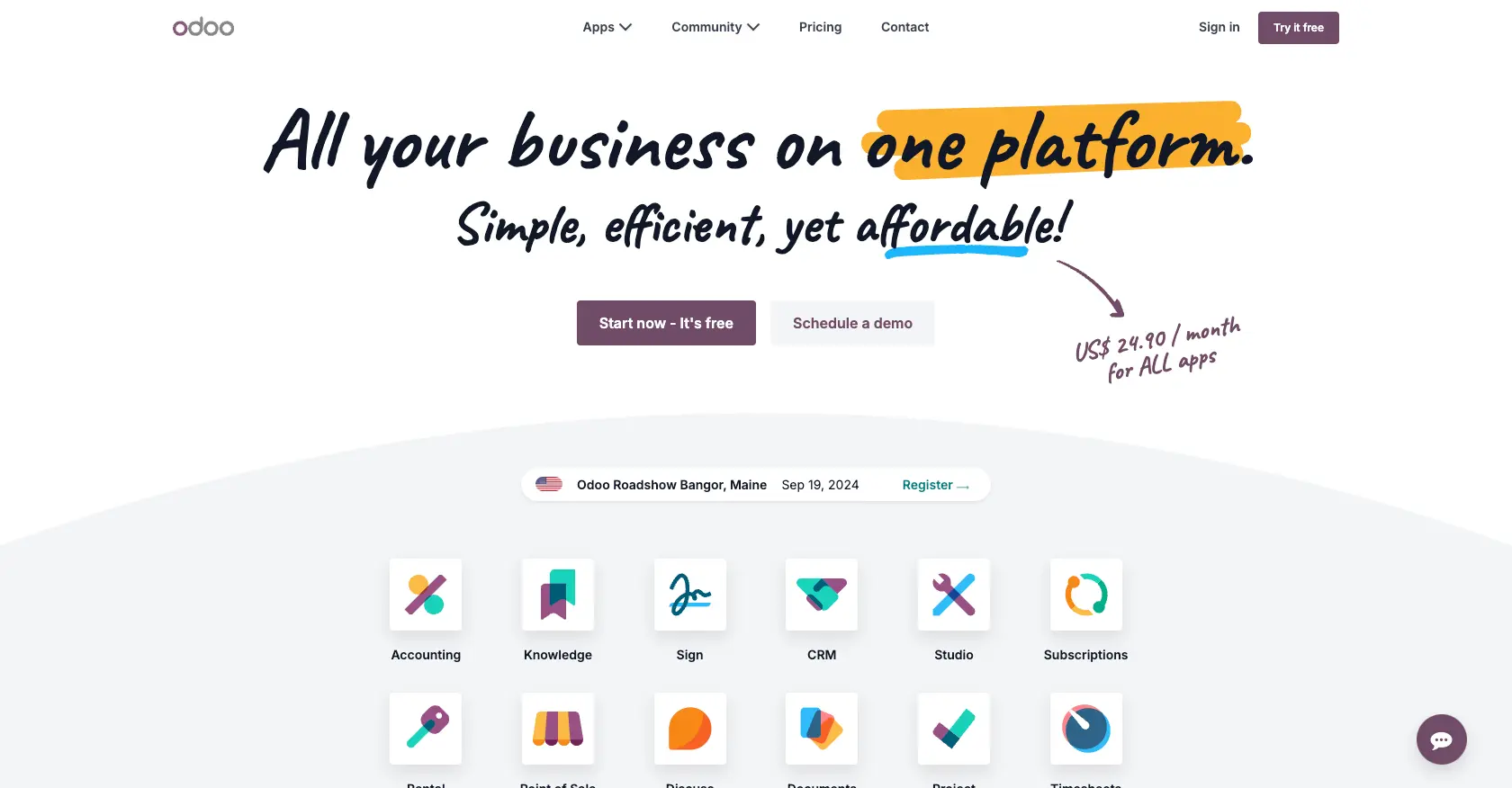
Introduction to Odoo Online API
Odoo Online is a comprehensive suite of business applications that covers various business needs, including CRM, e-commerce, accounting, inventory, and project management. Its modular design allows businesses to customize and scale their operations efficiently.
For developers, integrating with the Odoo Online API offers the opportunity to automate and streamline business processes. For example, you might want to retrieve records from Odoo to synchronize data with another system, ensuring that your business operations remain seamless and up-to-date.
Setting Up Your Odoo Online Test/Sandbox Account
Before you can start interacting with the Odoo Online API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating an Odoo Online Account
If you don't already have an Odoo Online account, you can sign up for a free trial on the Odoo website. Follow the instructions to create your account and log in to the Odoo dashboard.
Accessing the Odoo Online Sandbox Environment
Odoo provides a sandbox environment where developers can test their integrations. To access this environment, navigate to the settings in your Odoo dashboard and look for the option to enable sandbox mode. This will allow you to test API interactions without impacting your production data.
Generating API Credentials for Odoo Online
To authenticate API requests, you'll need to generate API credentials. Follow these steps to create an app and obtain the necessary credentials:
- Go to the Developer Tools section in your Odoo dashboard.
- Select Create App and fill in the required information, such as the app name and description.
- Once the app is created, navigate to the API Credentials tab to find your client ID and client secret.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Understanding Custom Authentication in Odoo Online
Odoo Online uses a custom authentication method for API access. Ensure you have your client ID and client secret ready, as these will be used to generate an access token for making API calls.
For more detailed information on authentication, refer to the Odoo Online API documentation.
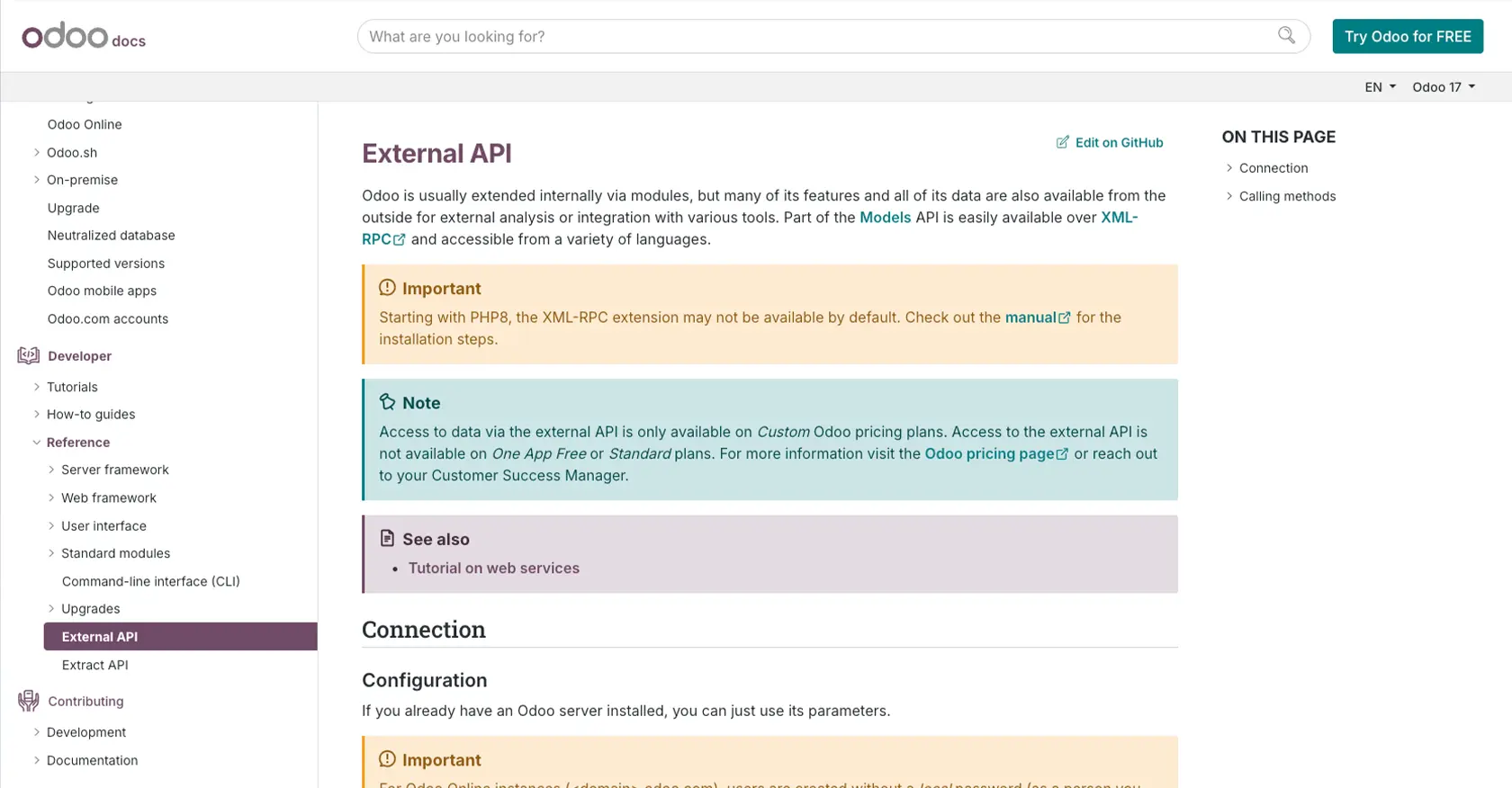
sbb-itb-96038d7
Making API Calls to Retrieve Records from Odoo Online Using JavaScript
To interact with the Odoo Online API and retrieve records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Odoo Online API
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based setup. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine.
You'll also need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Retrieve Odoo Online Records
Now, let's write the JavaScript code to make an API call to Odoo Online and retrieve records. Create a file named get_odoo_records.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-odoo-instance.com/api/endpoint';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Access_Token'
};
// Function to get records from Odoo Online
async function getOdooRecords() {
try {
const response = await axios.get(endpoint, { headers });
const records = response.data;
console.log(records);
} catch (error) {
console.error('Error fetching records:', error.response ? error.response.data : error.message);
}
}
// Call the function
getOdooRecords();
Replace Your_Access_Token
with the access token you obtained during the authentication setup.
Understanding the JavaScript Code for Odoo Online API Calls
In the code above, we use the axios
library to make a GET request to the Odoo Online API. The endpoint URL and headers are specified, including the authorization token. The getOdooRecords
function is defined to handle the API call and log the retrieved records.
If the request is successful, the records are printed to the console. In case of an error, the error message is logged, providing insight into what went wrong.
Running the JavaScript Code and Verifying Odoo Online API Response
To execute the code, run the following command in your terminal:
node get_odoo_records.js
Upon successful execution, you should see the records retrieved from Odoo Online displayed in the console. Verify the data by cross-checking with your Odoo Online sandbox environment to ensure accuracy.
Handling Errors and Troubleshooting Odoo Online API Calls
When making API calls, it's crucial to handle potential errors gracefully. The code includes error handling that logs the error message. Common issues may include incorrect endpoint URLs, invalid access tokens, or network problems.
Refer to the Odoo Online API documentation for more information on error codes and troubleshooting tips.
Conclusion and Best Practices for Using Odoo Online API with JavaScript
Integrating with the Odoo Online API using JavaScript can significantly enhance your ability to automate and streamline business processes. By following the steps outlined in this guide, you can efficiently retrieve records and ensure seamless data synchronization across systems.
Best Practices for Secure and Efficient Odoo Online API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Odoo Online API to avoid exceeding request quotas. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from Odoo Online is transformed and standardized to match the requirements of your application or system.
- Error Handling: Implement robust error handling to capture and log errors effectively. This will help in diagnosing issues and maintaining a reliable integration.
Streamline Your Integration Process with Endgrate
While integrating with Odoo Online API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with various platforms, including Odoo Online. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing integrations to focus on what truly matters—your core product.
Read More
Ready to get started?